A C++ custom iterator is a user-defined object that enables users to traverse a container through a specific set of rules, similar to built-in iterators.
Here's a simple example of a custom iterator for a collection of integers:
#include <iostream>
#include <vector>
class IntCollection {
std::vector<int> data;
public:
void add(int value) { data.push_back(value); }
// Custom iterator class
class Iterator {
std::vector<int>::iterator it;
public:
explicit Iterator(std::vector<int>::iterator iterator) : it(iterator) {}
int operator*() { return *it; }
Iterator& operator++() { ++it; return *this; }
bool operator!=(const Iterator& other) const { return it != other.it; }
};
Iterator begin() { return Iterator(data.begin()); }
Iterator end() { return Iterator(data.end()); }
};
int main() {
IntCollection collection;
collection.add(1);
collection.add(2);
collection.add(3);
for (auto value : collection) {
std::cout << value << std::endl;
}
return 0;
}
Understanding Iterators
What is an Iterator?
An iterator is an object that enables a programmer to traverse through a container, such as an array or list, without exposing the underlying details of that container. In C++, iterators are widely used in the Standard Template Library (STL) to operate with various data structures, providing a standard interface for iteration.
Types of Iterators
C++ provides several types of iterators, each catering to different use cases and functionality:
- Input Iterators: These allow for reading data from a container sequentially.
- Output Iterators: These permit writing data into a container sequentially.
- Forward Iterators: These support multiple passes over a collection; they can read but only move forward.
- Bidirectional Iterators: These can move forwards and backwards, offering greater flexibility.
- Random Access Iterators: These allow direct access to any element in a container, providing functionalities similar to pointers, such as addition and subtraction of integer offsets.
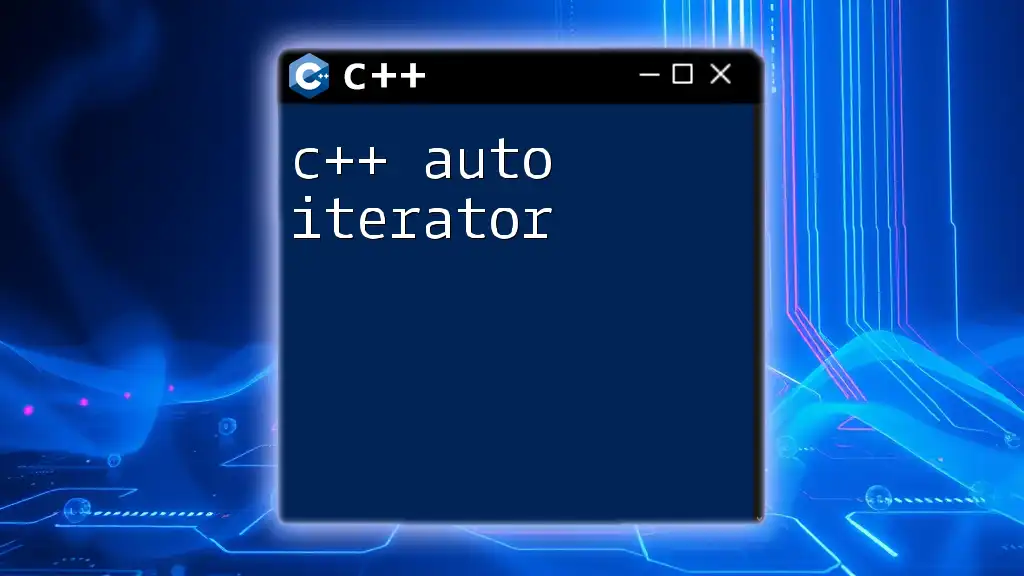
Why Create Custom Iterators?
While built-in C++ iterators fulfill many common needs, they can be limiting when it comes to specialized data structures or requirements. Here are several scenarios where custom iterators shine:
- Custom Data Structures: If you develop a unique data structure that doesn't fit the mold of existing containers, a custom iterator can provide the necessary traversal methods.
- Specialized Iteration Requirements: Certain situations may require non-linear traversal or custom filtering during iteration that standard iterators cannot provide.
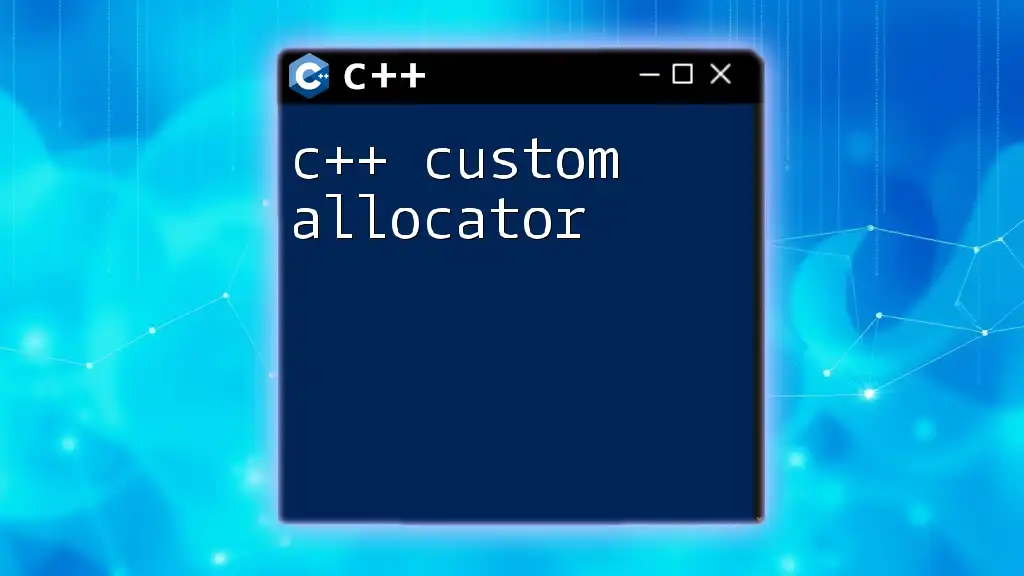
Creating a Custom Iterator
Step-by-Step Guide to Implementing a Custom Iterator
When implementing a custom iterator in C++, follow these steps to create an iterator class:
Defining the Iterator Class
The iterator class must encapsulate all the necessary member functions for it to operate effectively with your container. At a minimum, it should include:
- A constructor for initializing the iterator state.
- Overloaded operators (`operator++`, `operator*`, `operator!=`) for control and value access.
Here is a basic structure to get started:
class MyIterator {
public:
// Constructor and member variables
MyIterator(/* parameters */);
// Overload operator *
value_type& operator*();
// Overload operator ++
MyIterator& operator++();
// Overload operator !=
bool operator!=(const MyIterator& other);
};
Detailed Explanation of Each Member Function
Constructor: This initializes the internal state of the iterator—such as pointing to the beginning of the data structure.
operator*: This provides access to the value that the iterator currently points to. It should return a reference to the value to enable modification.
operator++: This function is crucial as it defines how the iterator moves to the next element. The logic here should consider the underlying structure and define what "next" means.
operator!=: This function checks whether two iterators point to different locations within the same container. This comparison enables loops and conditionals to exit gracefully.
Usage of Custom Iterators in a Container
To effectively demonstrate the usage of a custom iterator, let’s create a simple dynamic array container that employs our custom iterator.
Example: Custom Container Implementation
class MyContainer {
public:
MyContainer();
~MyContainer();
using iterator = MyIterator; // Hooking up custom iterator
// Various member functions to manipulate container
iterator begin();
iterator end();
// Further functionality such as adding elements, size etc.
};
In this example, `MyContainer` serves as a simple dynamic array. Its `begin()` and `end()` methods return iterators pointing to the first and one past the last elements of the container, permitting the use of range-based for loops and standard or custom algorithms.
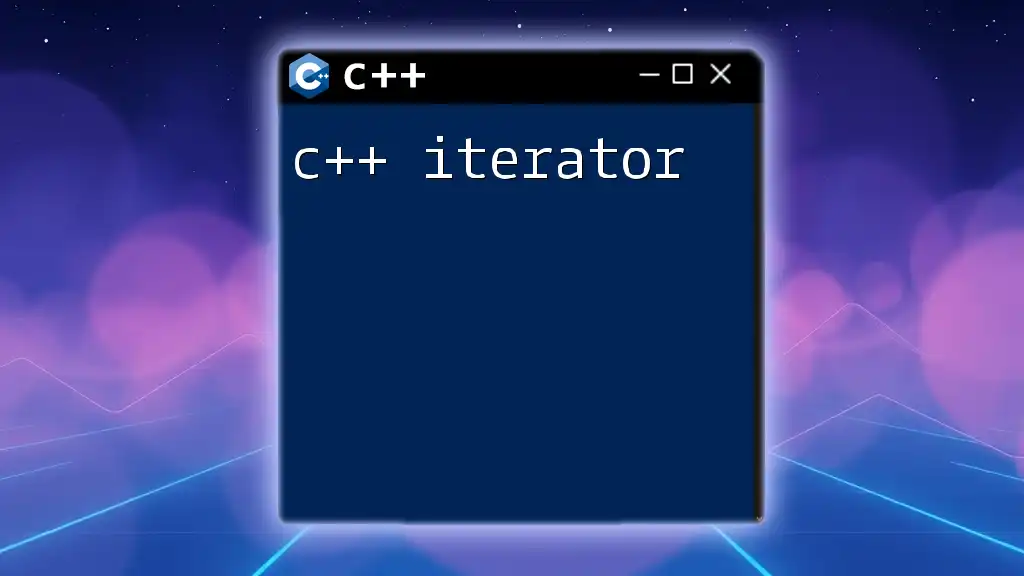
Best Practices for Writing Custom Iterators
Ensuring Iterator Validity
One of the key aspects of implementing custom iterators is managing the lifecycle and scope of both the iterators and the underlying collections. Ensure that your iterator does not outlive the container it is accessing, or else you'll run into undefined behavior.
Performance Considerations
Minimize overhead in custom iterator operations to ensure that they operate efficiently. Understanding and implementing copy and move semantics appropriately can lead to substantial performance improvements, especially for larger data sets.
Testing Your Custom Iterator
Unit tests are essential in verifying the behavior of your iterator. Cover several test cases, including:
- Basic access to elements (ensuring `operator*` is functional).
- Successful incrementing through the entire container (ensuring `operator++` behaves correctly).
- Boundary conditions like end-of-container behavior and iterator comparisons.
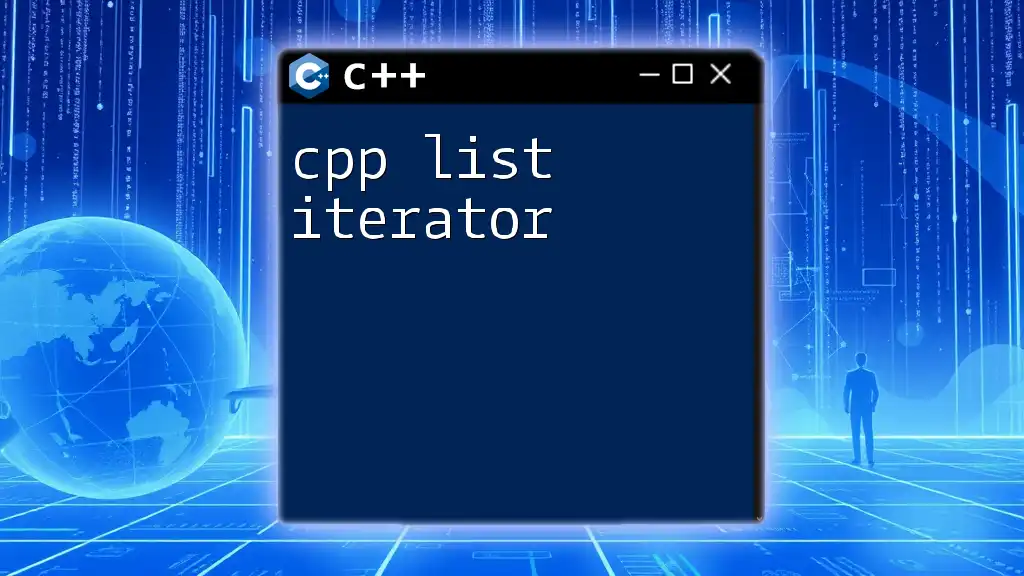
Common Pitfalls When Working with Custom Iterators
When building custom iterators, developers encounter some frequent pitfalls:
- Mistakenly Returning by Value: Returning iterators by value can lead to performance issues, especially for complex types. Always prefer returning by reference or using move semantics where appropriate.
- Incorrect Increment Logic: Failing to implement the increment logic correctly can lead to infinite loops. Always validate iterator movement logic against the container's actual data.
- Memory Management Issues: Be vigilant about memory ownership rules, particularly with dynamically allocated memory. Improper handling can lead to memory leaks or dangling pointers.
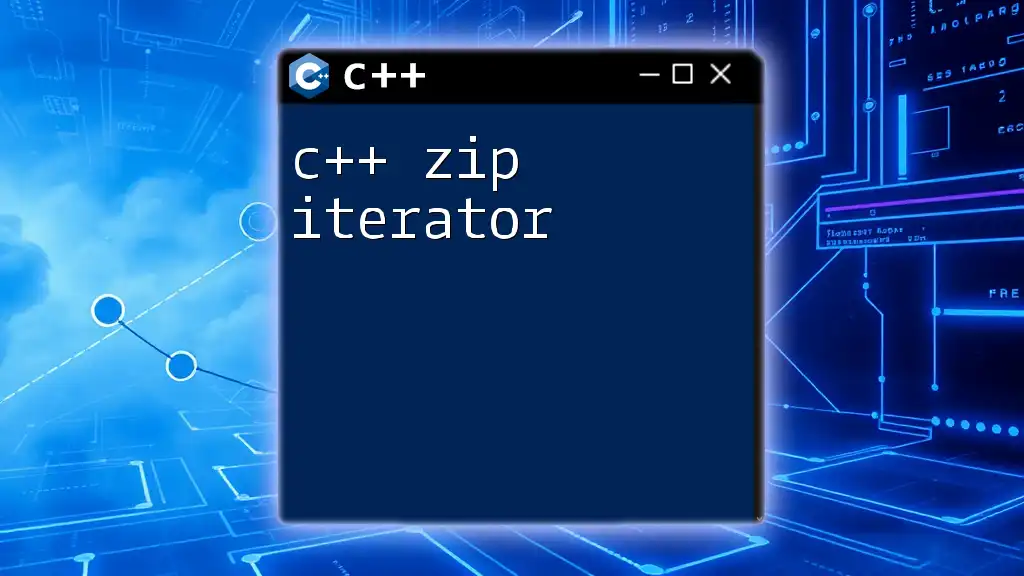
Conclusion
Understanding how to implement and utilize C++ custom iterators can significantly enhance your programming toolkit, allowing for greater flexibility and functionality in your code. By following the outlined steps and paying close attention to best practices, you can create custom iterators tailored to your unique data structures and requirements. Don’t hesitate to experiment with your creations and push the boundaries of what's possible with iteration in C++.
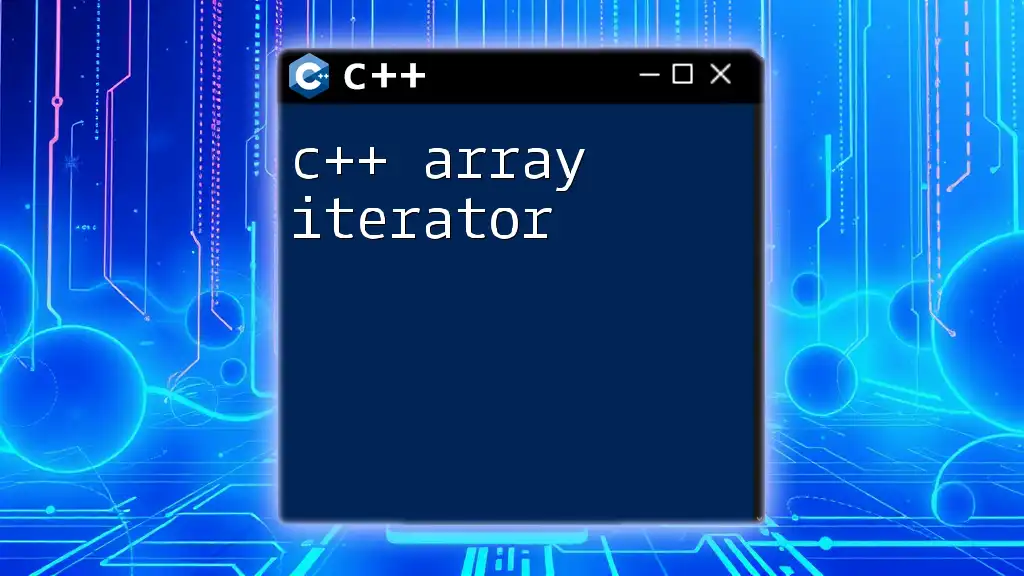
Call to Action
Now that you're equipped with the knowledge of creating custom iterators, why not give it a try? Create your own custom iterator for a data structure and share your experience in the comments. Your insights could benefit others in the learning journey!
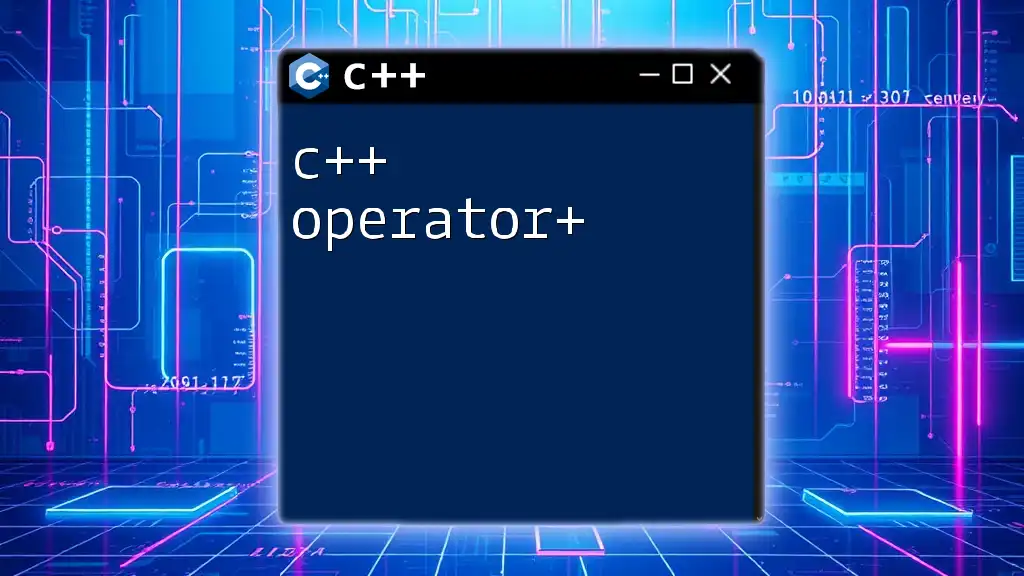
Additional Resources and References
Make sure to check out official C++ documentation for iterators, as well as recommended books and online tutorials to further deepen your understanding and enhance your skills in C++ programming.