In C++, an array iterator allows you to traverse an array using pointer arithmetic or the standard `begin()` and `end()` functions to access elements efficiently.
Here's a simple code snippet demonstrating the use of an array iterator:
#include <iostream>
int main() {
int arr[] = {10, 20, 30, 40, 50};
for (int* it = arr; it < arr + 5; ++it) {
std::cout << *it << " ";
}
return 0;
}
What is an Iterator?
An iterator is a design pattern that allows for sequential access to the elements in a collection without exposing the underlying structure of that collection. It acts as a generalized pointer that enables traversal through items such as arrays, lists, or even custom collections.
Types of Iterators
C++ categorizes iterators based on their capabilities:
- Input Iterators: They allow reading data from a collection one element at a time.
- Output Iterators: They enable writing data into a collection one element at a time.
- Forward Iterators: These allow the traversal of data in a single direction (forwards).
- Bidirectional Iterators: They extend forward iterators by supporting traversal in both directions—forward and backward.
- Random Access Iterators: They provide all the features of bidirectional iterators along with the ability to access any element directly, making them very versatile, especially for complex algorithms.
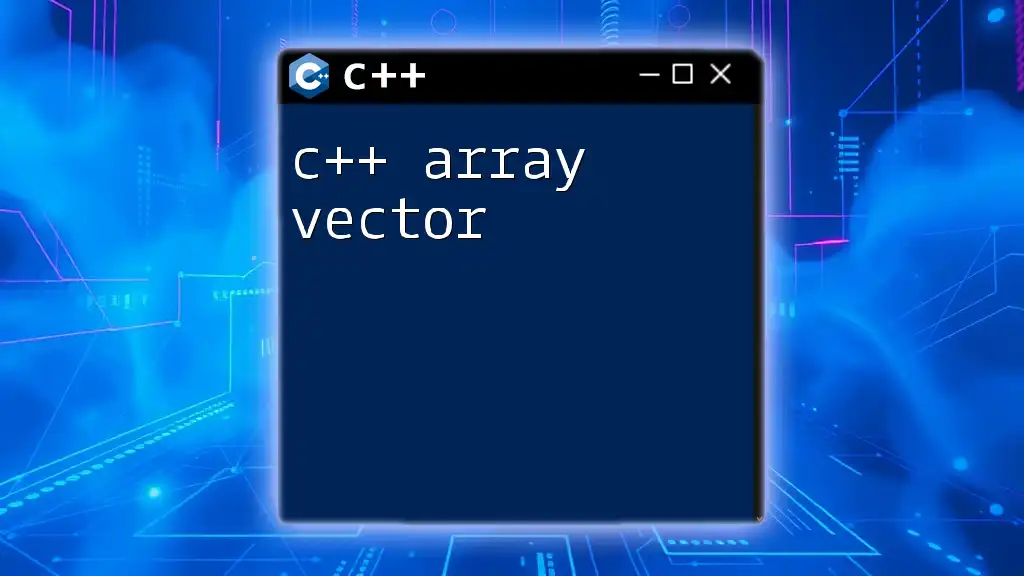
Understanding C++ Arrays
An array in C++ is a collection of elements of the same data type, stored in contiguous memory locations. They offer a way to hold multiple items under a single name and can be accessed using indices.
Characteristics of Arrays
- Fixed Size: The size of the array is determined at compile time, making it less flexible than dynamic data structures.
- Homogeneous Data Types: All elements must be of the same type, which enables efficient storage and access.
- Memory Allocation: Arrays require a contiguous block of memory, which makes accessing elements quick but may lead to inefficient memory usage if not resized properly.
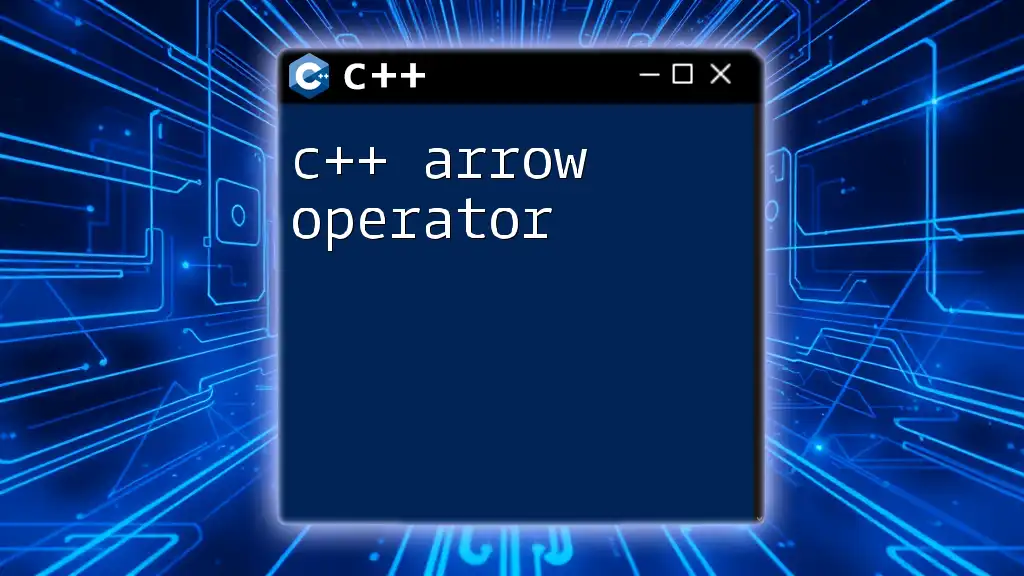
Introduction to C++ Array Iterators
Using C++ array iterators promotes better programming practices by enhancing readability and maintainability. They allow you to simplify your code and make it less prone to errors by abstracting away the complexity of the underlying data structure.
Why Use Array Iterators?
- Simplifies Code: Writing loops using iterators can make the code cleaner and easier to understand compared to traditional indexing.
- Improves Readability: Well-named iterator variables can convey intent better than array indices.
- Encourages Abstraction: By utilizing iterators, programmers can focus on what they are doing rather than how the data is structured.
Basic Syntax of Array Iterators
In C++, array iterators can often be implemented using pointers. Here's a basic example:
int arr[] = {1, 2, 3, 4, 5};
int* begin = arr; // pointing to the first element
int* end = arr + sizeof(arr) / sizeof(arr[0]); // pointing to one past the last element
In this code snippet, `begin` points to the first element of the array, while `end` points to one past the last element, facilitating easy iteration over the array.
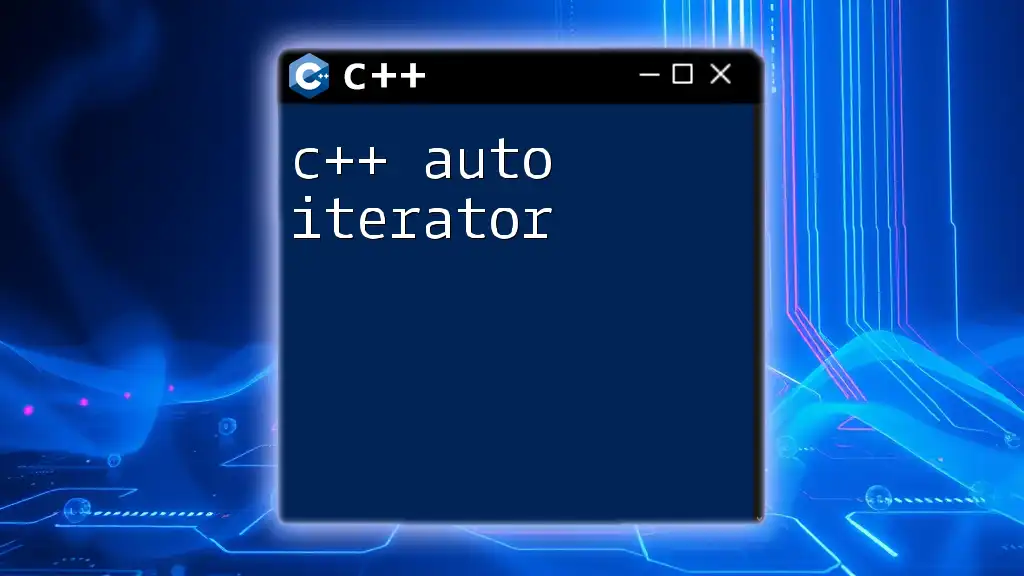
Using Array Iterators in C++
Iterating through an Array
Example 1: Basic Iteration
With pointers acting as iterators, you can traverse through an array elegantly. Here’s a simple code example:
for (int* ptr = arr; ptr < end; ++ptr) {
std::cout << *ptr << " ";
}
In this loop, we redefine `ptr` to point to each element in the array from `begin` to `end`, outputting the value at that position. This method avoids the complexity of using array indices directly.
Advanced Iteration Techniques
Example 2: Reverse Iteration
You may sometimes want to iterate through the array in reverse order. This can be achieved easily with pointer arithmetic:
for (int* ptr = end - 1; ptr >= arr; --ptr) {
std::cout << *ptr << " ";
}
This loop demonstrates how you can traverse the array backward, starting from the last element and moving toward the first.
Example 3: Using `std::begin` and `std::end`
With the advent of C++11, we have the convenience of using functions like `std::begin` and `std::end` to create modern and cleaner code:
#include <iostream>
#include <iterator>
int main() {
int arr[] = {1, 2, 3, 4, 5};
for (auto it = std::begin(arr); it != std::end(arr); ++it) {
std::cout << *it << " ";
}
}
In this case, `std::begin(arr)` returns a pointer to the first element, while `std::end(arr)` returns a pointer to one past the last element. This approach enhances standardization and code readability.
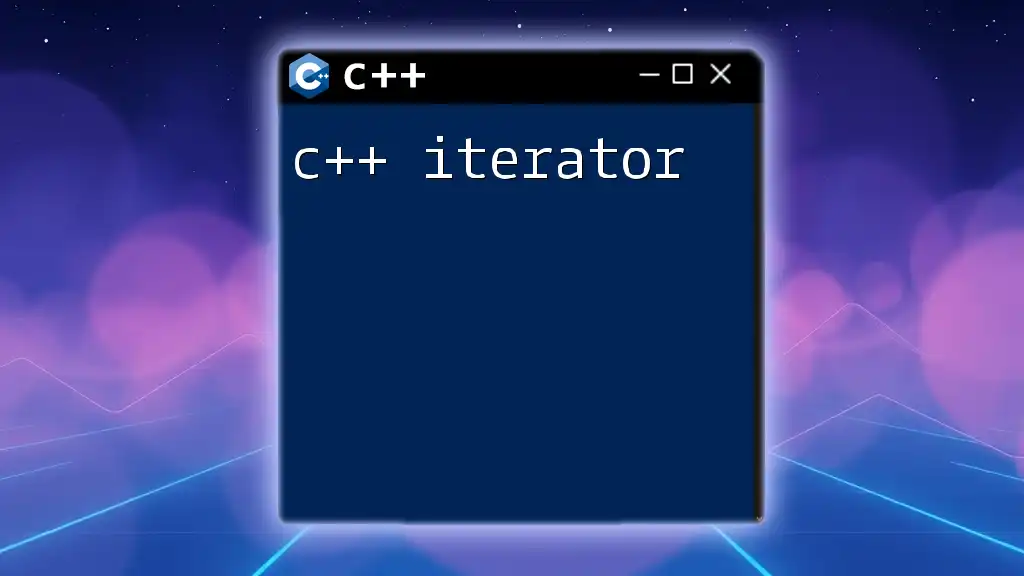
Performance Considerations
Efficiency of Array Iterators
When comparing array iterators to traditional indexing, iterators are often more efficient in terms of code maintainability and ease of use. However, remember that pointer arithmetic requires careful management to prevent going out of bounds.
Avoiding Common Pitfalls
While iterators greatly enhance code expressiveness, they come with potential pitfalls:
- Pointer Arithmetic Pitfalls: Miscalculations can lead to accessing invalid memory.
- Out-of-Bounds Access: Always ensure that iterator operations are performed within array bounds to prevent undefined behavior.
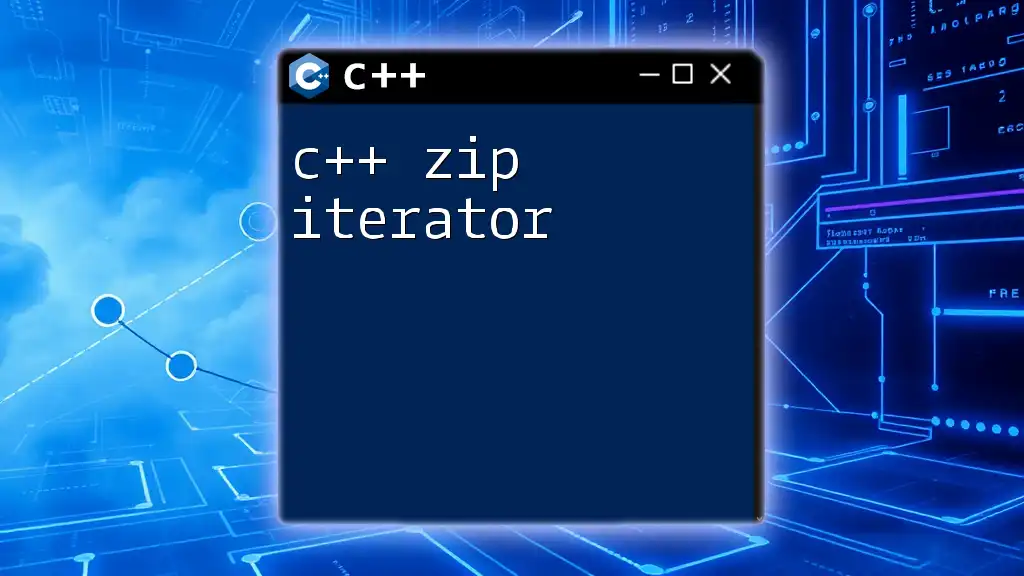
Real-World Applications
Use Cases for Array Iterators
In real-world scenarios, array iterators can play critical roles in various contexts:
- Exploring Data Structures: Use iterators for analyzing and manipulating the contents of data structures like arrays.
- Implementing Algorithms: Iterators facilitate the implementation of essential algorithms, such as searching or sorting, allowing you to work with collections abstractly.
Best Practices for Implementing Iterators
- Encapsulation: Keep the iterator logic encapsulated in functions or classes when possible.
- Abstraction: Use iterators to separate the logic of data access from data storage for better scalability.
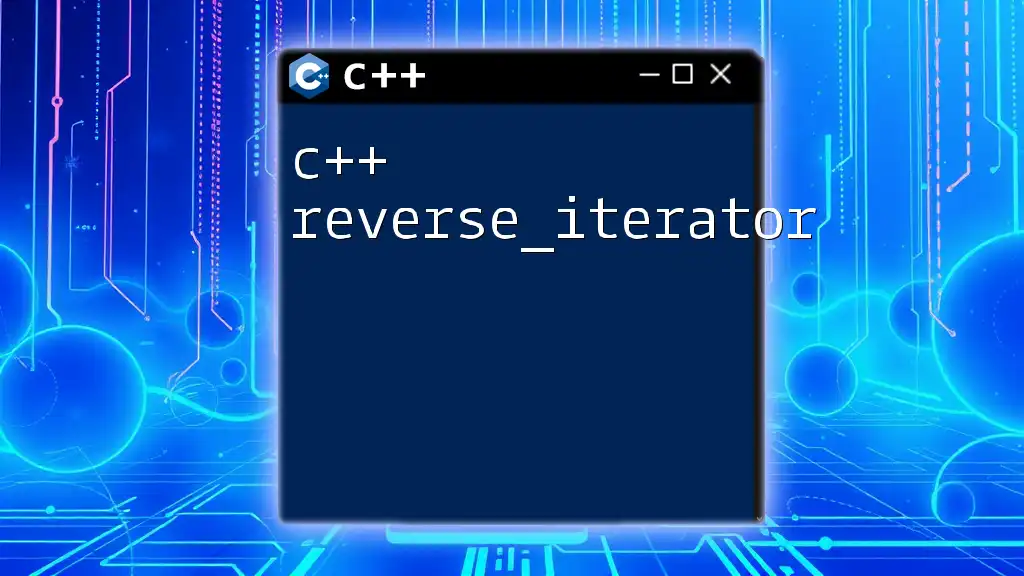
Conclusion
C++ array iterators greatly enhance code quality by simplifying iterative processes and promoting a more abstract way of programming. By effectively utilizing array iterators, programmers can achieve cleaner, more maintainable, and efficient code. Remember that understanding how to properly implement and use iterators is crucial in taking full advantage of their capabilities in the C++ programming landscape.