In C++, an array vector is a dynamic array that can grow or shrink in size and provides the benefits of both arrays and linked lists, allowing easy management of collections of elements.
Here’s a simple example of using a `std::vector` in C++:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
numbers.push_back(6); // Add an element
for(int num : numbers) {
std::cout << num << " "; // Output the elements
}
return 0;
}
Understanding C++ Arrays
What is an Array?
In C++, an array is a collection of elements that are of the same data type. Arrays allow you to store multiple items together under a single name, providing a convenient way to manage a fixed amount of data. When you declare an array, memory is allocated contiguously, meaning all elements are stored next to each other in memory.
Here’s an example of how to declare and initialize an array:
int numbers[5] = {1, 2, 3, 4, 5};
In this case, `numbers` can hold up to five integers.
Characteristics of Arrays
- Fixed size: Arrays have a fixed size once declared. You cannot change the size of an array during runtime, which can limit flexibility in certain applications.
- Type safety: All elements in an array must be of the same type. This means that you cannot store a mixture of integers and floats in a single array.
- Memory efficiency: Arrays are typically allocated on the stack, which can be more efficient for memory access. However, this can lead to limits on the maximum array size because stack space is generally limited.
Accessing Array Elements
Accessing elements in an array is done using indices. The first element is at index `0`, and the last element can be accessed using `size - 1`. Modifying elements is straightforward:
numbers[2] = 10; // Changes the third element to 10
To iterate through the array, you can use a loop:
for (int i = 0; i < 5; i++) {
std::cout << numbers[i] << " ";
}
Limitations of Arrays
Arrays have several limitations to consider:
- Fixed size limitation: You must know the array size at compile time. If the required size exceeds the allocated space, it can lead to undefined behavior.
- Lack of built-in methods: Unlike vectors, arrays do not provide built-in functions for common operations such as size checking, inserting, or deleting elements. To perform these tasks, you would need to implement additional logic.
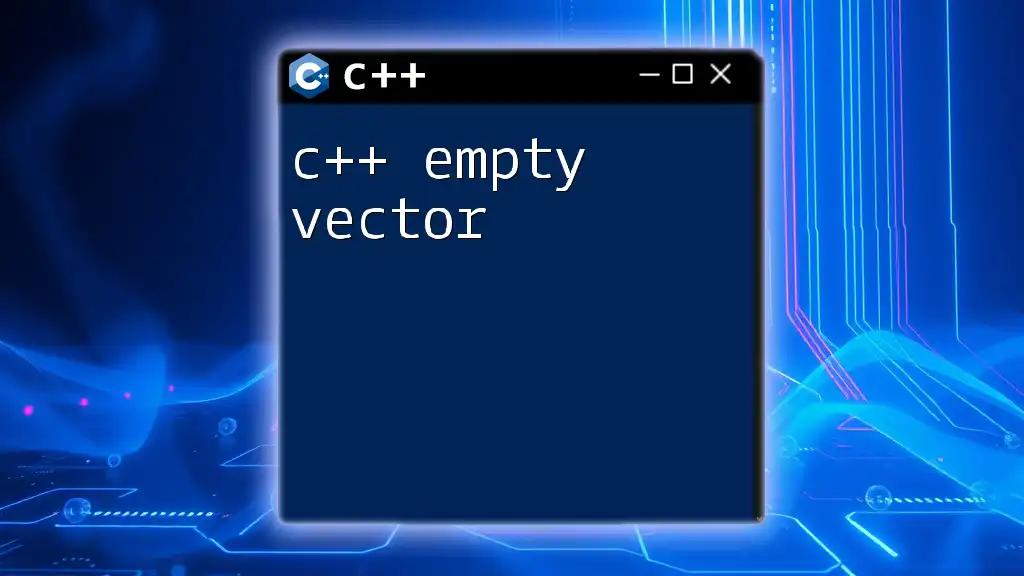
Exploring C++ Vectors
What is a Vector?
A vector is a part of the C++ Standard Template Library (STL) and represents a dynamic array. This means that vectors can change their size during runtime, allowing better flexibility than traditional arrays. Here's a simple declaration and initialization of a vector:
std::vector<int> numbers_vector = {1, 2, 3, 4, 5};
Advantages of Vectors over Arrays
Dynamic Size
One of the significant advantages of vectors is their ability to resize dynamically. You can add or remove elements as needed without worrying about exceeding a fixed size:
numbers_vector.push_back(6); // Adds 6 to the end
Built-in Methods
Vectors offer numerous built-in methods that simplify data manipulation. Commonly used methods include:
- `size()`: Returns the number of elements in the vector.
- `empty()`: Checks whether the vector is empty.
- `clear()`: Clears all elements from the vector.
Here’s an example of resizing a vector:
numbers_vector.resize(10); // Resizes the vector to hold 10 elements
Type Safety and Flexibility
Vectors also provide a templated interface, allowing storage of any data type. You can create a vector of vectors (2D vector):
std::vector<std::vector<int>> matrix = {{1, 2}, {3, 4}};
Accessing Vector Elements
Accessing elements in a vector is similar to arrays. You can employ the indexing operator `[]`:
numbers_vector[2] = 10; // Modifies the third element
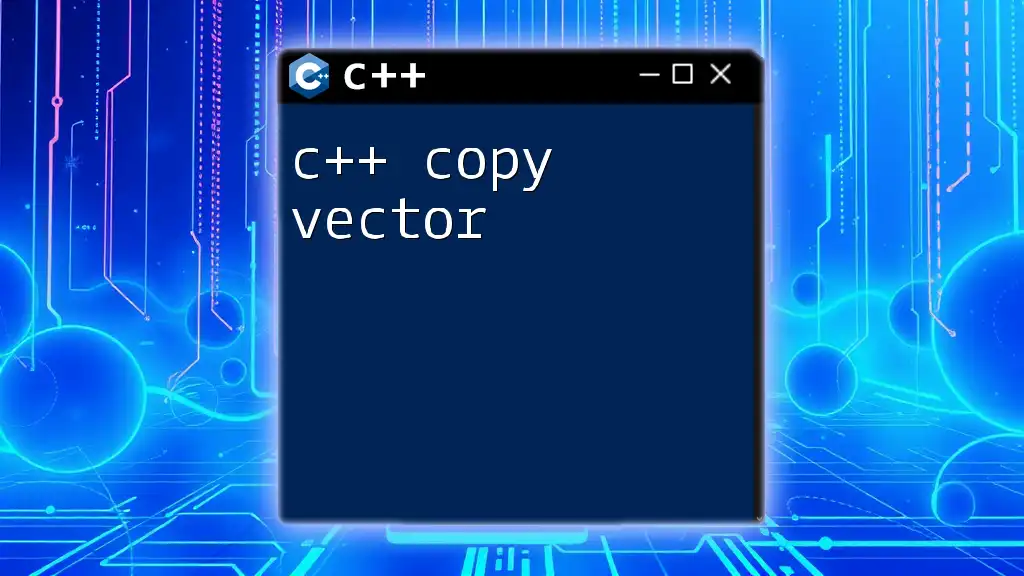
Key Comparisons Between Array and Vector
Memory Management
Arrays are usually allocated on the stack, which can be limited. If an array becomes too large, you may face a stack overflow. On the other hand, vectors use heap memory, which allows for dynamic allocation as necessary. This makes them more suitable for dynamic arrays.
Performance Considerations
While arrays provide slightly faster access times since their size is fixed, vectors are optimized for resizing and managing memory. Access speed for both structures is generally comparable. When considering time complexity:
- Accessing an element in an array: O(1)
- Accessing an element in a vector: O(1)
- Inserting or deleting an element in an array is often O(n), while in a vector, it can vary from O(1) (when appending) to O(n) (when resizing or deleting from the front).
When to Use Each
When deciding between arrays and vectors, consider the following guidelines:
- Use arrays when the size of the data is known at compile time and remains constant.
- Use vectors when you require dynamic sizing, built-in methods, or need to perform various operations effortlessly.
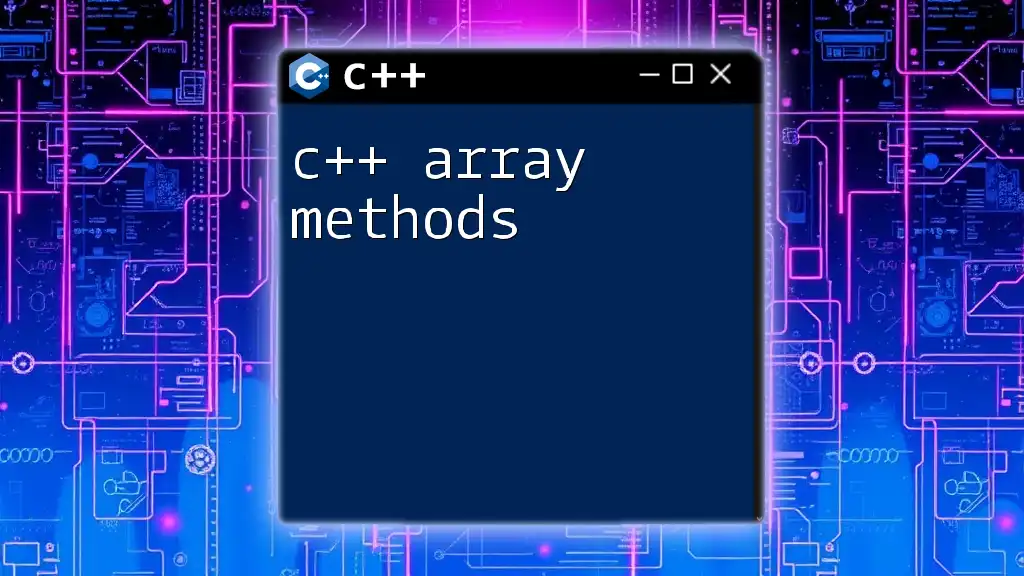
Conclusion
Understanding the differences between C++ arrays and vectors is crucial for effective programming in C++. Each data structure has its strengths and weaknesses, and mastering them will significantly enhance your coding capabilities.
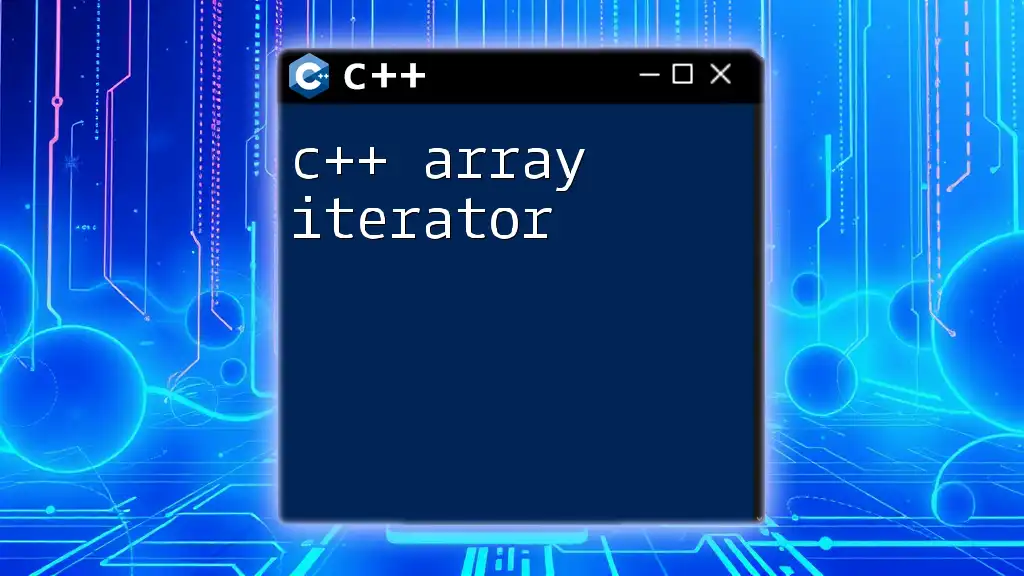
Additional Resources
To further your understanding of C++ data structures, check out the C++ official documentation and explore tutorials focused on C++ command usage. Practical coding sites will also provide hands-on opportunities for learning.
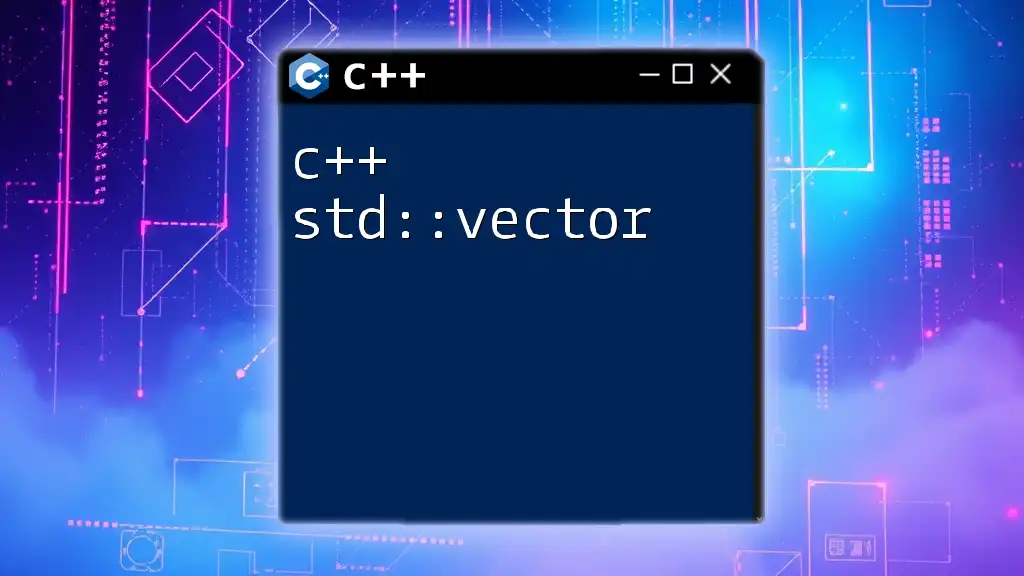
Frequently Asked Questions (FAQ)
Can a vector replace an array in every scenario?
While vectors provide flexibility and convenience, there are scenarios where arrays are more suitable, especially when managing low-level system resources or specific performance optimizations.
What are multi-dimensional arrays vs. multi-dimensional vectors?
Multi-dimensional arrays are arrays of arrays, with fixed sizes, whereas multi-dimensional vectors can be resized dynamically. Each has its use cases, depending on your requirements.
How do I convert an array to a vector?
You can create a vector from an array easily with the following code snippet:
std::vector<int> vec(std::begin(numbers), std::end(numbers));
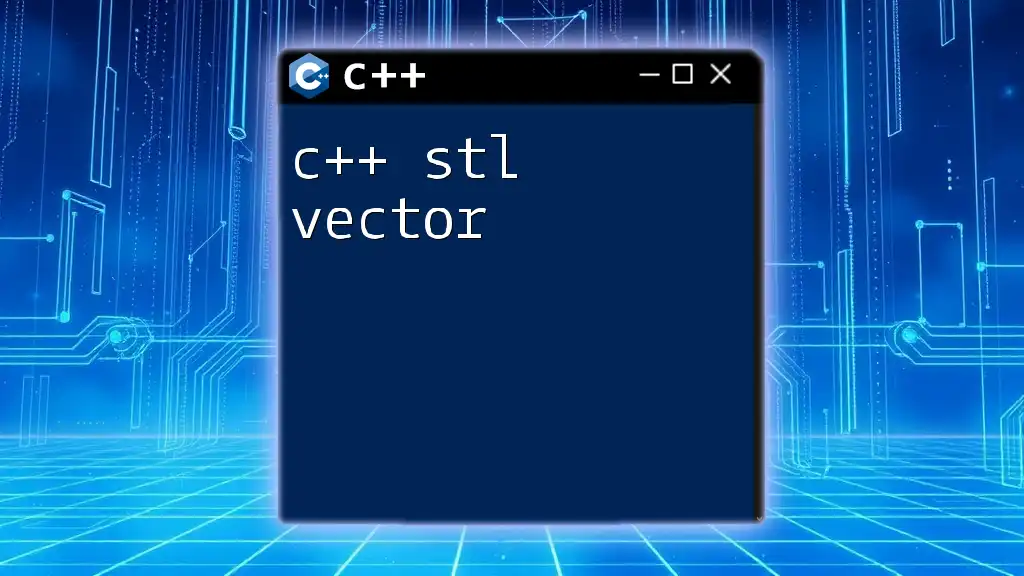
Call to Action
Take your C++ skills to the next level by signing up for our tutorials on using C++ commands efficiently. Mastering arrays and vectors will provide you with essential tools for better programming in C++.