A C++ copy constructor is a special constructor that initializes a new object as a copy of an existing object, typically used for creating a new instance with the same values as another object.
class MyClass {
public:
int value;
// Copy constructor
MyClass(const MyClass &obj) {
value = obj.value;
}
};
What is a Copy Constructor?
A copy constructor in C++ is a special type of constructor used to create a new object as a copy of an existing object. Its primary purpose is to ensure that when an object is duplicated, all its resources are appropriately managed and copied, especially when dealing with dynamic resources like memory allocations. In essence, a copy constructor defines how an object should be duplicated.
Understanding Object Copies
When we talk about object copying, it's crucial to differentiate between shallow and deep copies:
- Shallow Copy: This type of copy duplicates only the values of the object's members, which means that if the object has pointers to dynamically allocated memory, both the original and the copied object will point to the same memory location. This can lead to issues such as double deletion and memory leaks.
- Deep Copy: In contrast, a deep copy duplicates not only the values but also the underlying data structures. This means that if an object references dynamic memory, the copy constructor will allocate new memory and copy the content to ensure that the copied object has its independent copy.
Understanding these concepts is fundamental before defining and implementing a copy constructor.

Creating a Simple Copy Constructor
The syntax of a copy constructor is relatively straightforward. It is defined like any other constructor but takes a single parameter of the same class type, passed by reference, typically prefixed by `const` to avoid modifying the source object.
Here’s an example of a simple copy constructor:
class Example {
public:
int value;
Example(int v) : value(v) {} // Parameterized constructor
Example(const Example &obj) : value(obj.value) { // Copy constructor
// additional logic can be added here
}
};
In this snippet, the `Example` class includes a parameterized constructor to initialize the `value` member. The copy constructor takes a constant reference to another `Example` object and initializes the new object's `value` with the one from the `obj`.
How the Copy Constructor Works
When you create an object and want to copy it, C++ automatically calls the appropriate constructor. For instance:
Example obj1(10); // Calls the parameterized constructor
Example obj2 = obj1; // Calls the copy constructor
In this scenario, `obj2` is created as a copy of `obj1`, which invokes the copy constructor, copying the value from `obj1` to `obj2`.
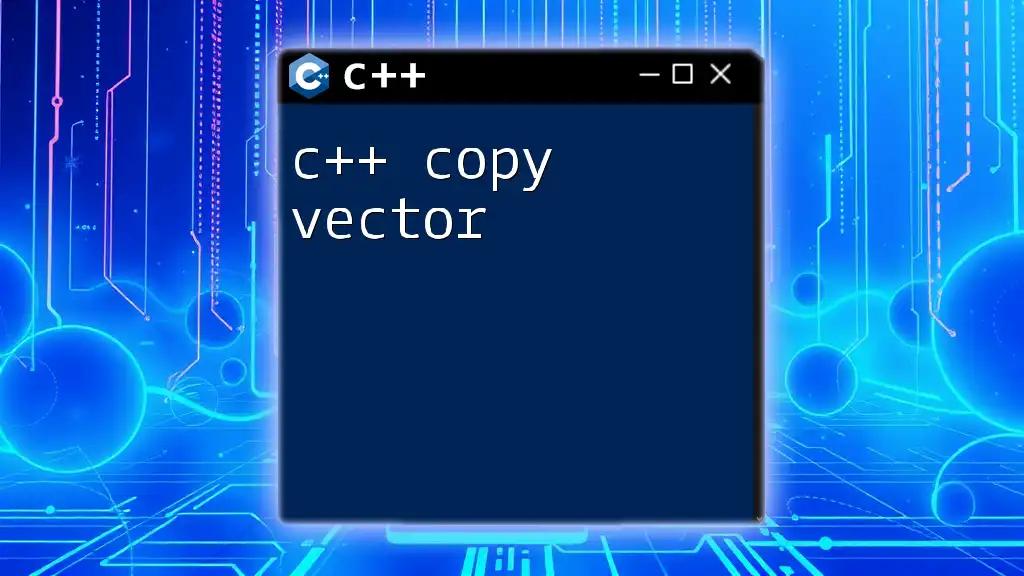
The Role of Copy Constructors in Class Design
When to Define a Copy Constructor
You should explicitly define a copy constructor when:
- Your class manages resources, such as dynamic memory or file handles.
- The default copy behavior provided by the compiler would lead to undesirable outcomes or bugs (e.g., shallow copies).
Copy Constructor vs. Default Copy Constructor
C++ automatically generates a copy constructor for you if you do not provide one. However, the default copy constructor performs a shallow copy. This behavior can lead to significant issues when objects have member variables that allocate resources dynamically.
For example, consider this scenario:
class Shallow {
public:
int* data;
Shallow(int size) {
data = new int[size]; // dynamically allocated memory
}
~Shallow() {
delete data; // Cleaning up
}
};
If a `Shallow` object is copied, the default copy constructor will only copy the pointer `data` to the new object. Consequently, when either object is destroyed, they both call `delete` on the same memory, leading to undefined behavior due to double deletion.

Copying Complex Objects
Handling Dynamic Memory in Copy Constructors
To handle situations like this, you need to implement a deep copy in your copy constructor. Here’s how you would modify the `Shallow` class:
class DeepCopy {
public:
int* data;
DeepCopy(int size) {
data = new int[size]; // allocate memory
}
DeepCopy(const DeepCopy &obj) {
data = new int[*obj.data]; // create new memory for copy
// copy actual values
}
~DeepCopy() {
delete[] data; // clean up
}
};
In this code, the copy constructor not only allocates new memory but also copies the contents from `obj` to the new `data`. This ensures that when either instance is destroyed, it has its own memory to manage, eliminating issues of double deletions.
Copying Objects with Pointers
When working with pointers in a class, consider using smart pointers like `std::shared_ptr` or `std::unique_ptr` where feasible. These automatically handle memory cleanup, reducing the risks associated with manual memory management.

Understanding the Copy-and-Swap Idiom
What is the Copy-and-Swap Idiom?
The copy-and-swap idiom is an efficient and robust way to manage resources and implement the assignment operator based on copy constructors. It simplifies the assignment logic and helps prevent resource leaks or corruption.
Here’s an example implementation:
class SwapExample {
public:
std::vector<int> data;
SwapExample(const std::vector<int>& vec) : data(vec) {}
// Copy constructor
SwapExample(const SwapExample &other) : data(other.data) {}
// Swap function
void swap(SwapExample &other) noexcept {
std::swap(data, other.data);
}
// Assignment operator using copy-and-swap
SwapExample& operator=(SwapExample other) {
swap(other);
return *this;
}
};
In this example, the assignment operator takes its parameter by value, relying on the copy constructor to create a copy. Then, the `swap` method efficiently exchanges the resources between the current object and the copy, ensuring that the original object's resources are safely released once the copy goes out of scope.

Copy Constructor Best Practices
Rule of Three in C++
The Rule of Three states that if your class requires a user-defined copy constructor, destructor, or copy assignment operator, it likely requires all three. This rule emphasizes the importance of resource management in C++:
- Copy Constructor: To define how to copy the object.
- Destructor: To clean up resources.
- Copy Assignment Operator: To handle the assignment of one object to another.
Avoiding Common Pitfalls
Be cautious of self-assignment issues, particularly in your assignment operator. A simple check at the start can prevent potential problems:
if (this == &other) return *this; // Prevent self-assignment
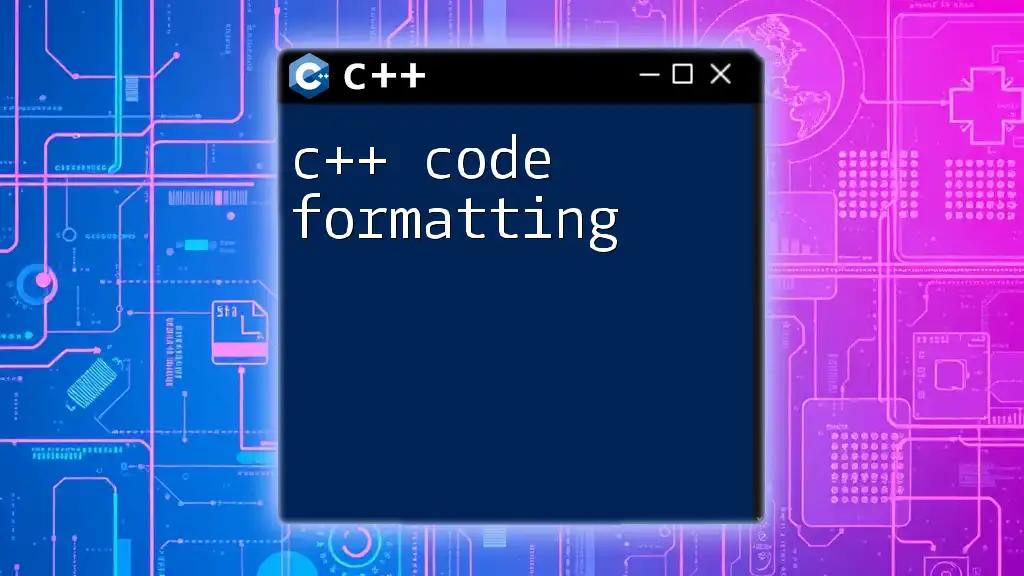
Performance Considerations
Impact of Copy Constructors on Performance
Copy constructors can introduce overhead, particularly in performance-critical applications, due to the time taken to copy resources.
Using Move Semantics as an Alternative
Introduced in C++11, move semantics allows for more efficient resource management by transferring ownership of resources instead of copying them. For classes managing resources, implementing a move constructor can significantly boost performance:
class MoveExample {
public:
int* data;
MoveExample(int size) : data(new int[size]) {}
// Move constructor
MoveExample(MoveExample&& other) noexcept : data(other.data) {
other.data = nullptr; // Null out the source
}
~MoveExample() {
delete[] data;
}
};
In this code, the move constructor transfers the pointer from `other` and clears it, avoiding any unnecessary copying and enhancing efficiency.
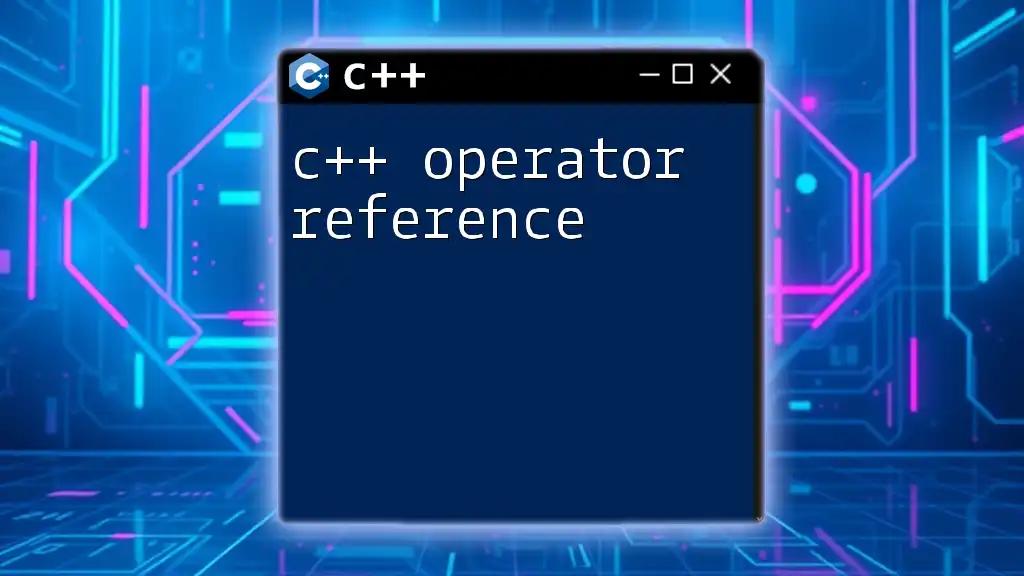
Conclusion
Copy constructors are a vital aspect of C++ programming, particularly when it comes to managing resources effectively. By understanding and implementing copy constructors correctly, especially in conjunction with the Rule of Three and move semantics, developers can create robust and efficient classes that avoid common pitfalls associated with object copying.
As you continue your journey in C++ programming, consider exploring related concepts such as the Rule of Five, which extends the Rule of Three by incorporating move semantics, to deepen your understanding of resource management in modern C++.

FAQs about Copy Constructors
What happens if I don't implement a copy constructor?
If you don’t implement a copy constructor, C++ generates a default copy constructor that performs a shallow copy. This may lead to issues if your class manages resources, such as dynamic memory.
Can a copy constructor be declared private?
Yes, a copy constructor can be declared private. This is often done to prevent copying of instances, particularly for classes that should not allow copying due to resource management concerns (e.g., singletons).
What is the significance of the `const` keyword in a copy constructor?
The `const` keyword indicates that the object passed into the copy constructor should not be modified. This is crucial because it protects the original object from unintentional changes during the copying process, ensuring the integrity of the source object.