C++ code coverage tools are essential for assessing the effectiveness of your tests by analyzing which parts of your codebase are exercised during execution.
Here’s a simple example showcasing how you can use the `gcov` tool to generate a coverage report for a C++ program:
// sample.cpp
#include <iostream>
void hello() {
std::cout << "Hello, World!" << std::endl;
}
int main() {
hello();
return 0;
}
To compile and generate the coverage data, you would use:
g++ -fprofile-arcs -ftest-coverage sample.cpp -o sample
./sample
gcov sample.cpp
Understanding Code Coverage
What is Code Coverage?
Code coverage is a critical metric in software testing that reflects the extent to which your source code is executed while running tests. In the context of C++, it helps developers identify which parts of their codebase have been tested and, importantly, which parts have not. The primary types of code coverage include statement coverage, branch coverage, function coverage, and line coverage:
- Statement Coverage measures whether each executable statement in the code has been executed at least once during tests.
- Branch Coverage goes a step further, ensuring that every possible branch (true/false paths) in control structures like if-statements or loops has been tested.
- Function Coverage checks if every function in the code has been invoked during testing.
- Line Coverage is a detailed measure that assesses whether each line of code has been executed.
Why is Code Coverage Important?
The importance of code coverage in C++ development cannot be overstated. High code coverage indicates a well-tested codebase, which reduces the likelihood of hidden bugs and enhances the maintainability of the code. When changes are made or new features are added, comprehensive test coverage allows developers to feel confident that they are not inadvertently introducing defects in untouched areas of the code.
Moreover, most software development methodologies advocate for continuous testing and integration. C++ code coverage tools play a critical role in supporting these practices, helping ensure that all parts of the code are actively tested throughout the development lifecycle.
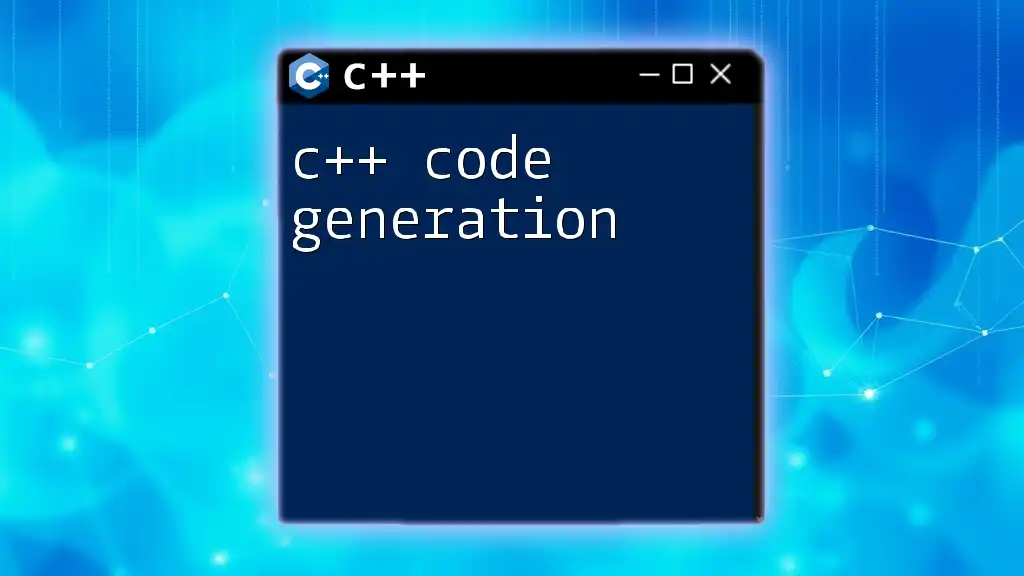
Overview of C++ Code Coverage Tools
Popular C++ Code Coverage Tools
There are numerous tools available for measuring code coverage in C++, each with its unique features and advantages:
-
gcov: This tool is part of the GNU Compiler Collection (GCC) and provides basic code coverage analysis. It generates reports that show which parts of your code were executed during testing, alongside the number of times they were executed. To integrate gcov with your C++ project, simply compile your code with flags enabling instrumented code coverage:
g++ -fprofile-arcs -ftest-coverage -o my_program my_program.cpp ./my_program gcov my_program.cpp
-
lcov: Built on top of gcov, lcov offers enhanced visualization for coverage reports, allowing for both summary information and detailed HTML reports. This can greatly aid in interpreting complex data. To generate an HTML report, you would typically proceed as follows:
lcov --capture --directory . --output-file coverage.info genhtml coverage.info --output-directory out
-
codecov: This is a cloud-based service that provides seamless integration with CI/CD pipelines. It supports various code coverage formats and provides comprehensive visualization of your coverage statistics, making it easier for teams to monitor their test effectiveness.
-
BullseyeCoverage: Although it's a commercial product, BullseyeCoverage provides in-depth coverage analysis, especially suited for larger teams and enterprise projects. The reports generated are highly detailed and accessible, which helps in understanding and improving code coverage.
Comparison of C++ Code Coverage Tools
When choosing the right tool for measuring code coverage, consider the following features:
- Ease of Integration: Does the tool fit naturally into your existing workflow? Both gcov and lcov are easy to set up with GCC projects, while codecov might require additional steps for CI/CD integration.
- Level of Detail in Reporting: Assess the granularity of the coverage reports. Tools like BullseyeCoverage may provide more detailed insights than gcov.
- Support for Various Platforms: Ensure the tool you choose works well with your build system—whether that’s Makefiles, CMake, or others—and supports the platforms your software is targeting.
Evaluating these factors will help you select a tool that seamlessly fits into your development process.
Pros and Cons
While each tool comes with its strengths, they also have certain limitations:
-
gcov:
- Pros: It's open-source and a part of GCC, making it widely available and straightforward to use.
- Cons: Its functionality is primarily limited to code compiled with GCC.
-
lcov:
- Pros: Enhances gcov’s output by generating colorful HTML reports.
- Cons: Requires an additional installation and configuration process.
-
codecov:
- Pros: Provides excellent integration with various CI/CD platforms and supports multiple programming languages.
- Cons: The free tier may not be sufficient for private repositories.
-
BullseyeCoverage:
- Pros: Comprehensive support and advanced reporting features ideal for large teams.
- Cons: It involves licensing costs, which may not be suitable for all budgets.
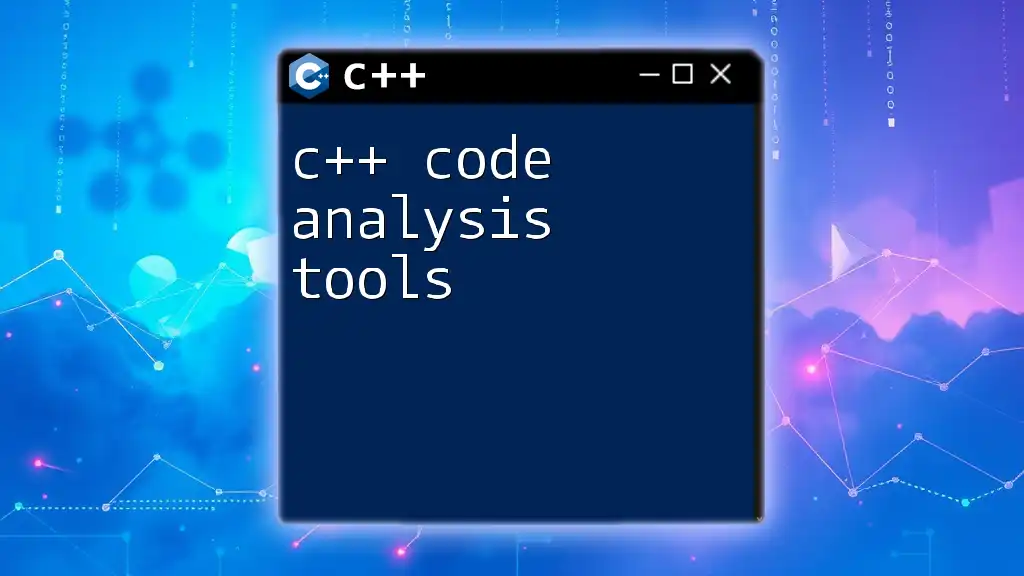
Integrating Code Coverage Tools into Your C++ Workflow
Setting Up gcov
To effectively utilize gcov in your C++ projects, follow these steps:
-
Compile your code with the appropriate flags to enable coverage data collection:
g++ -fprofile-arcs -ftest-coverage -o my_program my_program.cpp
-
Run your program, which will generate data files needed by gcov:
./my_program
-
Generate coverage reports using gcov:
gcov my_program.cpp
This will produce a `.gcov` file alongside your source file, containing detailed coverage metrics.
Visualizing with lcov
Once you have gcov output, lcov can create a more user-friendly HTML report. Here’s how to do it:
-
Capture coverage data:
lcov --capture --directory . --output-file coverage.info
-
Generate an HTML output to visualize the coverage results:
genhtml coverage.info --output-directory out
This report can be opened in any web browser, making it easy to analyze your code coverage visually.
Integrating codecov in CI/CD
To streamline code coverage with your CI/CD pipeline, you can integrate codecov, which automates many steps. Here’s an example setup for GitHub Actions:
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Install dependencies
run: sudo apt-get install g++ lcov
- name: Run tests with coverage
run: |
g++ -fprofile-arcs -ftest-coverage -o my_program my_program.cpp
./my_program
lcov --capture --directory . --output-file coverage.info
bash <(curl -s https://codecov.io/bash) -f coverage.info
This setup ensures that every time you commit code, it automatically tests and reports coverage to codecov.
Implementing BullseyeCoverage
If you're in a Windows environment and prefer a more comprehensive solution, BullseyeCoverage is an excellent choice. To implement it, follow these steps:
- Purchase and install BullseyeCoverage on your system following the official guidelines.
- Configure your project settings in Visual Studio to enable coverage collection.
- Execute your tests, and BullseyeCoverage will generate intuitive reports that can help identify untested code.
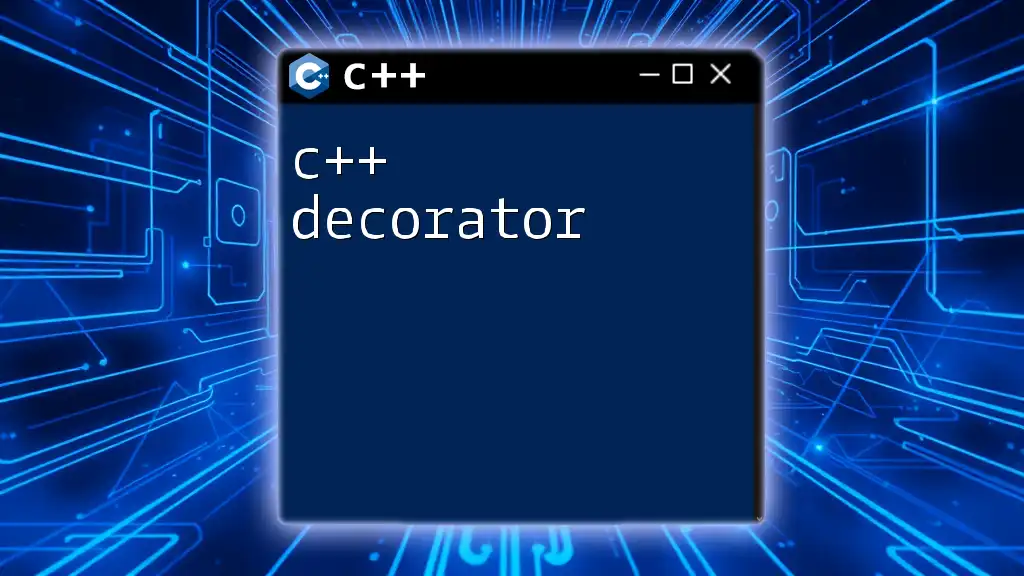
Best Practices for Achieving High Code Coverage
Writing Test-Driven Code
Embrace methodologies like Test-Driven Development (TDD), where you write tests before writing the actual implementation code. This approach ensures every piece of code has associated tests and promotes better design.
Regularly Monitor Code Coverage
Make code coverage an ongoing concern throughout the development lifecycle. Automate coverage reporting in your CI/CD pipeline to always have up-to-date insights regarding the parts of your codebase that might require additional testing.
Interpreting Coverage Reports
Analyzing coverage reports is just as important as generating them. Focus on areas with low coverage and prioritize writing tests for high-risk code paths. Remember, high code coverage does not necessarily equate to a bug-free or high-quality codebase; it merely indicates that the code has undergone some testing. Thus, strive for meaningful test coverage rather than merely aiming for high numbers.
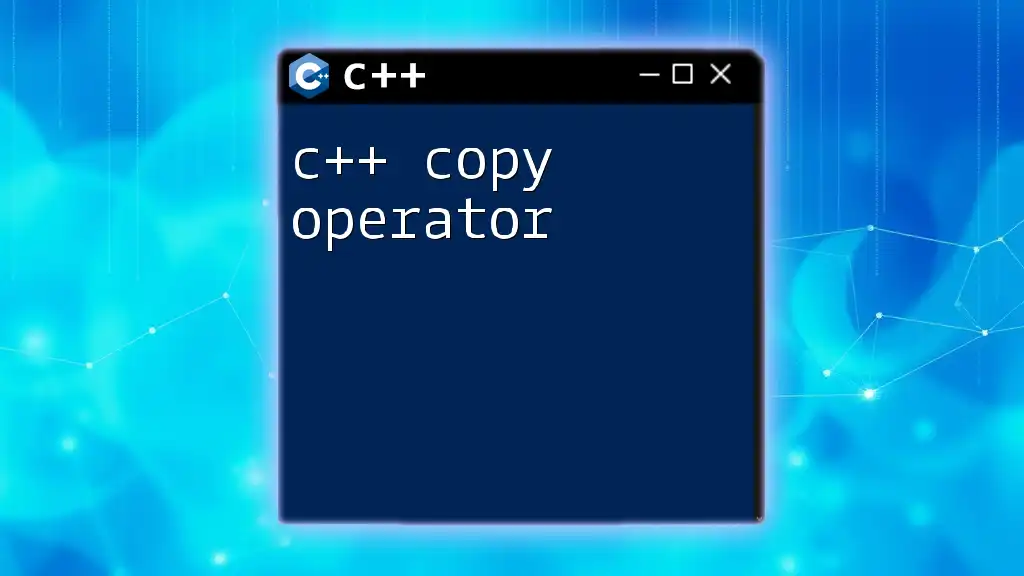
Conclusion
Incorporating C++ code coverage tools into your development workflow is essential for delivering robust and maintainable software. By understanding how to effectively utilize tools like gcov, lcov, codecov, and BullseyeCoverage, you can ensure your C++ code is well-tested and any gaps are identified quickly. Maintaining high code coverage will not only improve software quality but also instill confidence in your codebase as you make changes and enhancements over time.
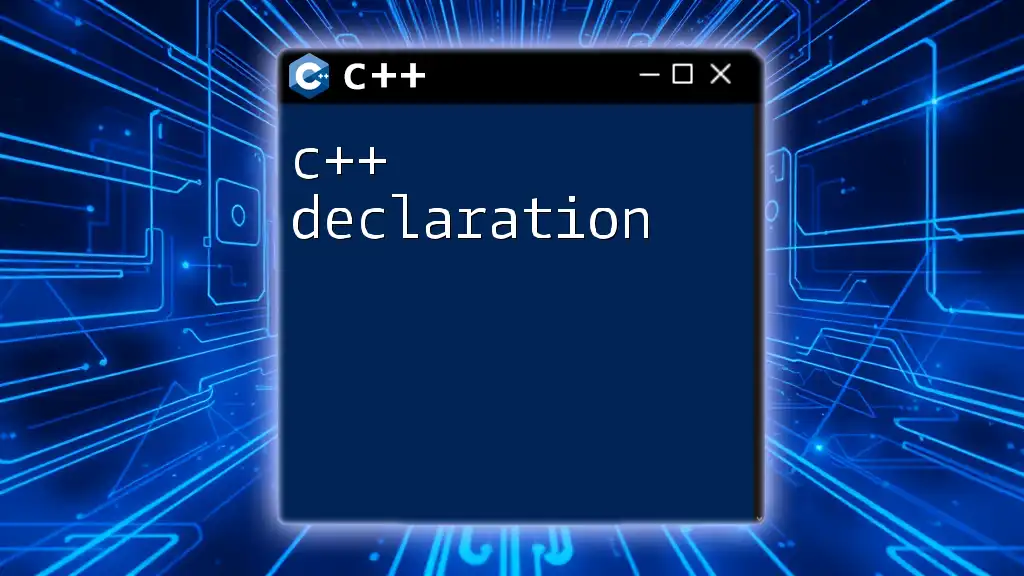
Additional Resources
For those eager to delve deeper into the world of code coverage, consider exploring the tools’ official documentation—for instance, gcov or codecov. Additionally, many online courses and community forums are available where you can further expand your knowledge and connect with other developers passionate about enhancing their C++ development practices.