C++ code practice involves hands-on exercises that enhance programming skills by allowing students to implement various C++ commands and constructs.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Getting Started with C++ Practice
Setting Up Your C++ Environment
Choosing the Right Development Tools
When embarking on your C++ code practice journey, selecting an appropriate development environment is crucial. You can choose from popular IDEs such as Visual Studio, Code::Blocks, and Eclipse, each offering unique features tailored to your coding needs. IDEs provide a user-friendly interface, debugging tools, and compilation assistance, all of which can significantly enhance your coding experience.
Compiling and Running Your First C++ Program
Before diving into practice problems, creating and running your first C++ program is an essential step. Here's how to write a simple "Hello, World!" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
To compile and run this code, follow these steps:
- Open your chosen IDE and create a new project.
- Copy and paste the above code into your C++ source file.
- Compile the code (look for a build or compile option).
- Run the compiled program to see the output.
Congratulations! You've successfully set up your C++ development environment.
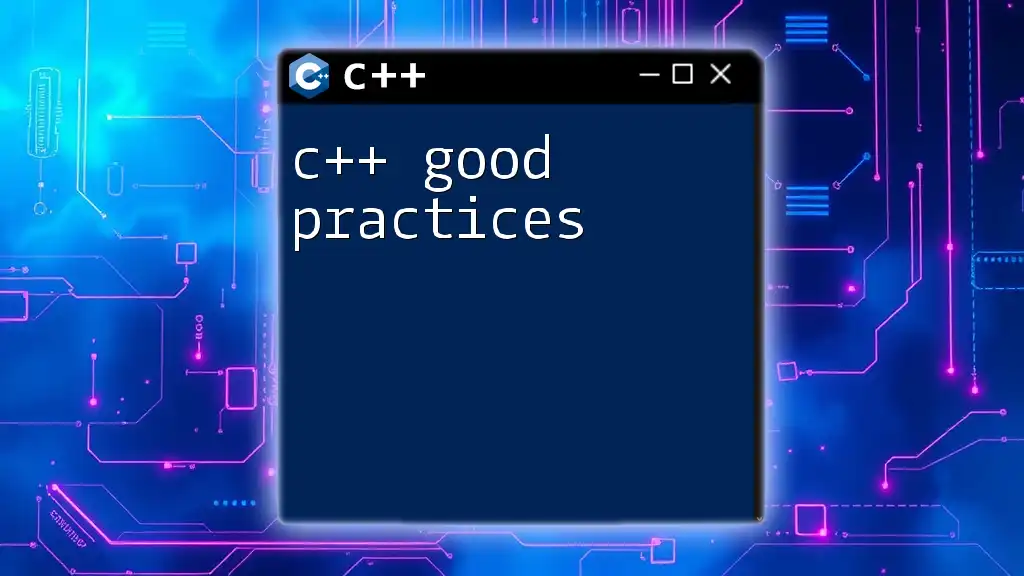
C++ Practice Problems for Beginners
Understanding Basic Concepts
Data Types and Variables
In C++, data types determine the kind of data a variable can hold. The most common data types include:
- int: for integers.
- float: for single-precision floating-point numbers.
- char: for characters.
Understanding how to declare and use variables is foundational for C++ practice.
Control Structures
Control structures like conditional statements (if, switch) and loops (for, while) allow your program to make decisions and repeat actions. Grasping these concepts aids in problem-solving.
Foundational C++ Practice Problems
Problem 1: Simple Calculator
Creating a simple calculator is a fantastic beginner practice exercise. The key functionalities should include addition, subtraction, multiplication, and division. Here’s a concise example of how you might implement this:
#include <iostream>
using namespace std;
int main() {
float num1, num2;
char operation;
cout << "Enter first number: ";
cin >> num1;
cout << "Enter second number: ";
cin >> num2;
cout << "Enter operation (+, -, *, /): ";
cin >> operation;
switch(operation) {
case '+':
cout << "Result: " << num1 + num2 << endl;
break;
case '-':
cout << "Result: " << num1 - num2 << endl;
break;
case '*':
cout << "Result: " << num1 * num2 << endl;
break;
case '/':
if (num2 != 0)
cout << "Result: " << num1 / num2 << endl;
else
cout << "Cannot divide by zero!" << endl;
break;
default:
cout << "Invalid operation!" << endl;
break;
}
return 0;
}
This example encourages users to practice handling user input and executing different functionalities based on that input.
Problem 2: Checking Prime Numbers
Understanding prime numbers is a great way to practice loops and conditional statements. The basic idea is to check if a number is divisible only by 1 and itself.
Here’s a simple approach:
#include <iostream>
using namespace std;
bool isPrime(int num) {
if (num < 2) return false;
for (int i = 2; i <= num / 2; i++) {
if (num % i == 0) return false;
}
return true;
}
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
if (isPrime(number))
cout << number << " is a prime number." << endl;
else
cout << number << " is not a prime number." << endl;
return 0;
}
This problem enhances your algorithmic thinking by focusing on efficient number checks.
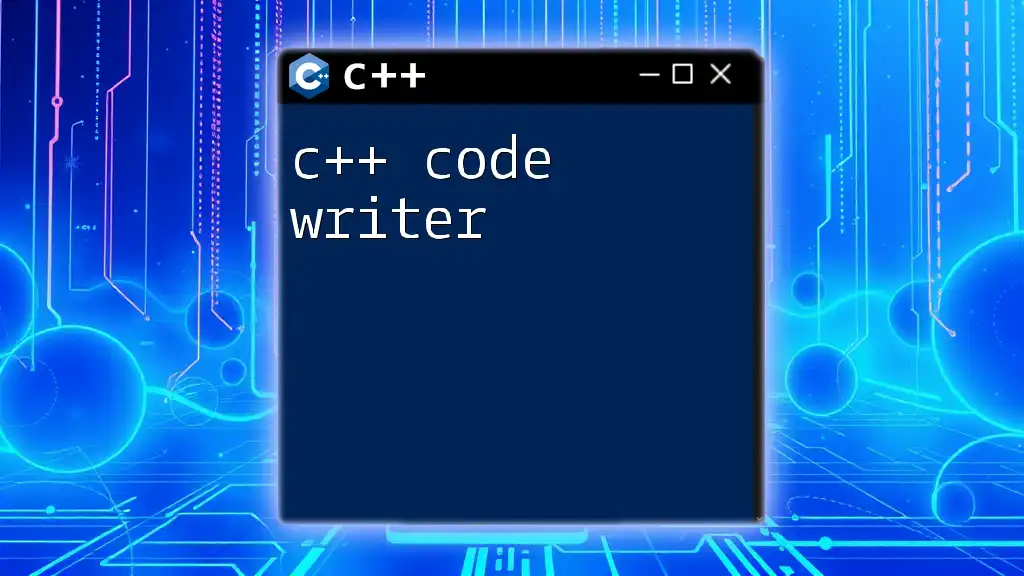
Intermediate C++ Coding Problems
Exploring Functions and Arrays
Functions in C++
Functions are essential for creating reusable code segments, allowing you to break down complex problems into manageable parts. Understanding how to declare and call functions is vital for your C++ code practice.
Problem 3: Reverse a String
Reversing a string is a common task in programming. This problem involves using a function to take a string input and return its reversed version.
Here’s a code snippet for reference:
#include <iostream>
#include <string>
std::string reverse_string(const std::string &str) {
std::string reversed = "";
for (int i = str.length() - 1; i >= 0; i--) {
reversed += str[i];
}
return reversed;
}
int main() {
std::string input;
std::cout << "Enter a string: ";
std::getline(std::cin, input);
std::cout << "Reversed string: " << reverse_string(input) << std::endl;
return 0;
}
By practicing this problem, you will not only strengthen your function use but also handle strings in C++ more effectively.
Working with Pointers and Dynamic Memory
Understanding Pointers
Pointers are a powerful feature in C++, allowing you to directly manipulate memory addresses. Learning bounds, pointer operations, and memory allocation is crucial at this stage of C++ code practice.
Problem 4: Dynamic Array Allocation
Dynamic memory management is another key aspect of C++. For this problem, you’ll create a program to dynamically allocate an array based on user input.
Example:
#include <iostream>
using namespace std;
int main() {
int size;
cout << "Enter the size of the array: ";
cin >> size;
int *arr = new int[size]; // Dynamic array allocation
cout << "Enter " << size << " elements:\n";
for (int i = 0; i < size; i++) {
cin >> arr[i];
}
cout << "You entered:\n";
for (int i = 0; i < size; i++) {
cout << arr[i] << " ";
}
cout << endl;
delete[] arr; // Free memory
return 0;
}
This exercise teaches essential C++ concepts involving pointers and dynamic memory management, preparing you for more complex applications.
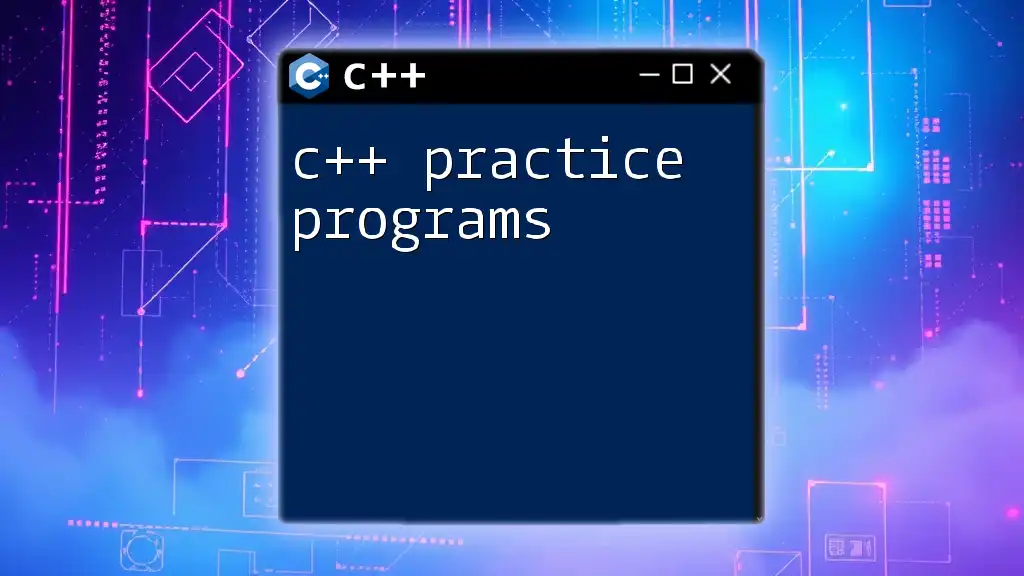
C++ Advanced Coding Problems
Introduction to Object-Oriented Programming (OOP)
Understanding OOP is a game-changer in programming. C++ is an object-oriented language, emphasizing the use of classes and objects to create structured programs.
Problem 5: Class-Based Bank Account Management
Designing a bank account management system is an excellent application of OOP principles. You’ll define a class to manage attributes and methods associated with a bank account.
Here’s a code structure you may follow:
#include <iostream>
using namespace std;
class BankAccount {
private:
string accountName;
double balance;
public:
BankAccount(string name, double initialBalance) {
accountName = name;
balance = initialBalance;
}
void deposit(double amount) {
balance += amount;
cout << "Deposited: $" << amount << " | New Balance: $" << balance << endl;
}
void withdraw(double amount) {
if (amount <= balance) {
balance -= amount;
cout << "Withdrawn: $" << amount << " | Remaining Balance: $" << balance << endl;
} else {
cout << "Insufficient funds!" << endl;
}
}
void displayBalance() {
cout << "Account Holder: " << accountName << " | Balance: $" << balance << endl;
}
};
int main() {
BankAccount account("John Doe", 1000.0); // Sample account
account.displayBalance();
account.deposit(500);
account.withdraw(300);
account.displayBalance();
return 0;
}
This design practice not only emphasizes OOP concepts but also facilitates a deeper engagement with C++ coding skills.
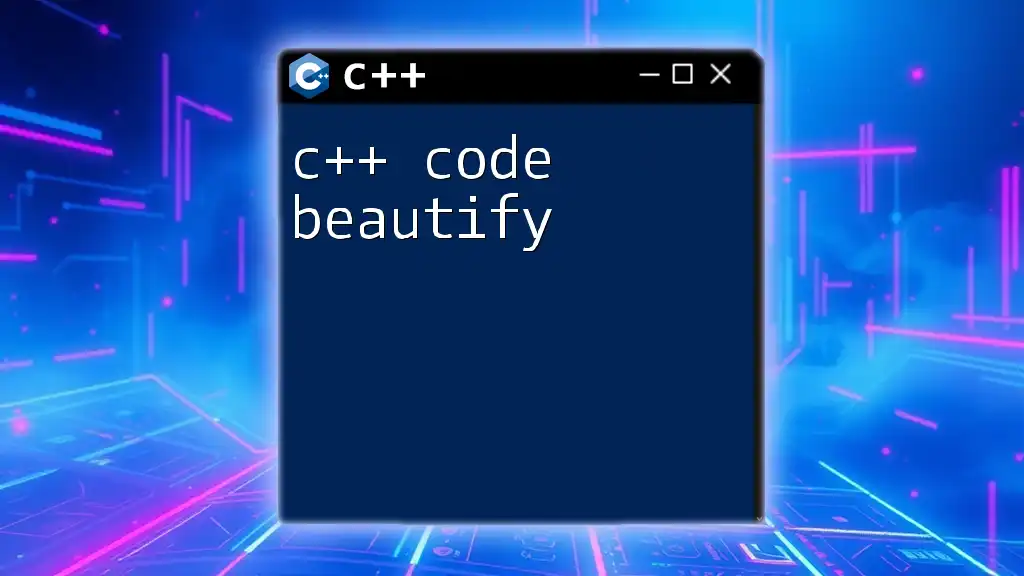
Tips for Successful C++ Coding Practice
Effective Problem-Solving Strategies
Breaking Down Problems into Smaller Steps
One of the most effective approaches in programming is breaking complex problems into smaller, manageable tasks. This method not only clarifies your coding path but also boosts confidence as each smaller task is completed.
Utilizing Online Resources and Platforms
Where to Find C++ Practice Problems
Numerous online platforms can aid in your C++ code practice. Websites like LeetCode, HackerRank, and Codewars offer a plethora of coding challenges that cater to all skill levels. Engaging with these platforms allows you to refine your skills and learn new techniques.
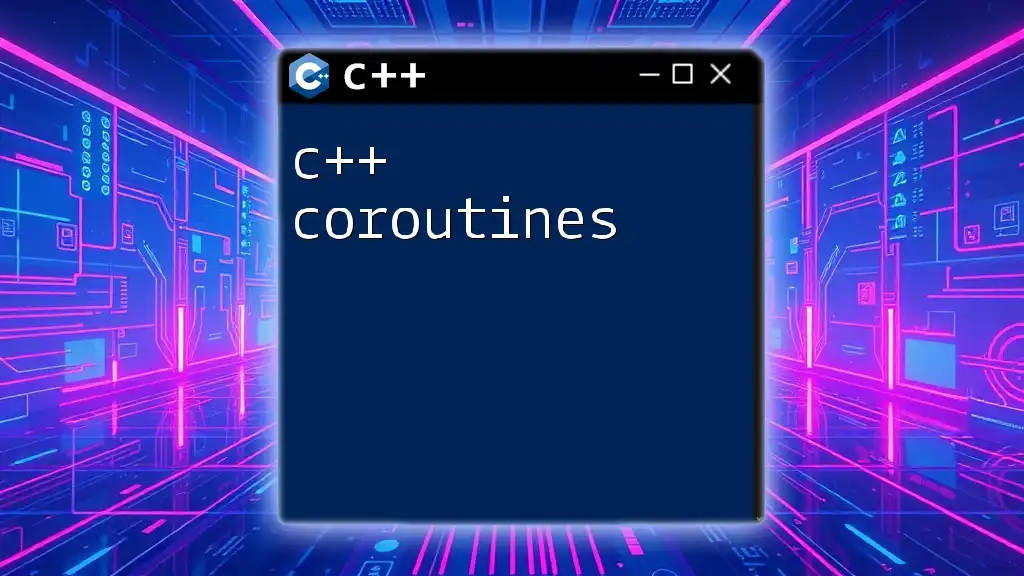
Conclusion
Moving forward with C++ code practice is vital to becoming a proficient programmer. This article has provided a structured path filled with practical problems and examples to boost your understanding of C++ programming. Remember, programming is a journey; consistent practice and problem-solving will help you grow in your coding abilities.
Through deliberate practice using basic to advanced C++ problems, you’ll develop both foundational knowledge and sophisticated skill levels necessary for tackling real-world programming challenges. Make it a habit to regularly engage with coding problems, participate in online forums, and never hesitate to seek help or collaborate with other coders on your journey. Happy coding!
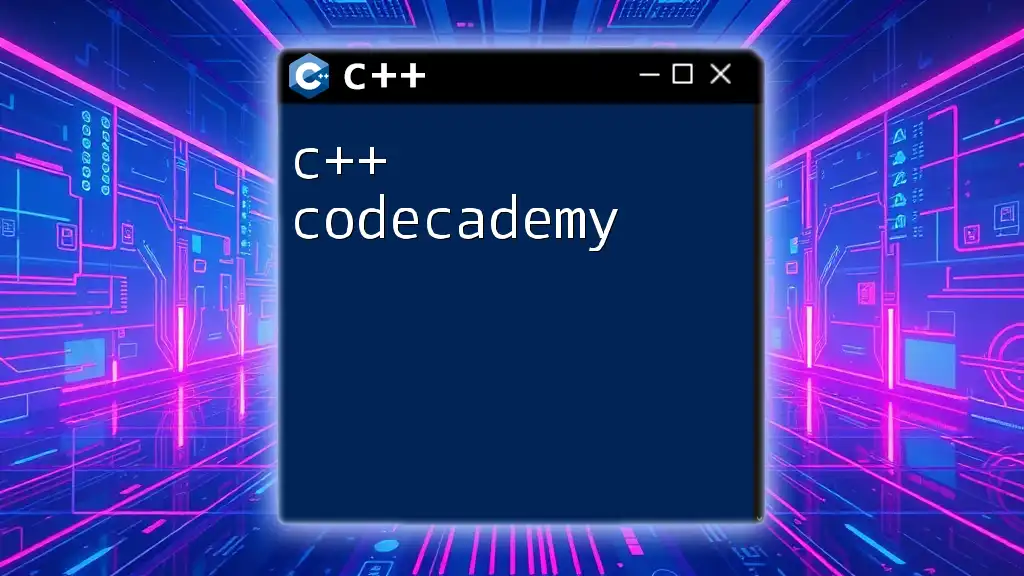
Additional Resources
- Books: “C++ Primer” by Stanley B. Lippman.
- Websites: TutorialsPoint, GeeksforGeeks.
- Communities: Stack Overflow, C++ Reddit forums.
Engage actively in these resources for a well-rounded learning experience.