C++ code beautification refers to the process of formatting and organizing C++ source code for better readability and maintainability.
Here's a simple example of poorly formatted C++ code followed by its beautified version:
#include<iostream>using namespace std;int main(){cout<<"Hello World";return 0;}
And here's the beautified version:
#include <iostream>
using namespace std;
int main() {
cout << "Hello World" << endl;
return 0;
}
Understanding C++ Code Beautification
What is Code Beautification?
Code beautification is a vital process in programming that enhances the appearance of source code. This involves arranging the code's layout, spacing, indentation, and syntax to make it more readable and maintainable. Well-beautified code has significant importance in collaborative environments and long-term projects, where multiple developers interact with the same codebase.
Why Use a C++ Code Beautifier?
Utilizing a C++ code beautifier offers numerous benefits:
-
Enhances Readability: Beautifully formatted code is easier for developers to read and understand. This clarity helps when returning to code after a period of time or when other developers need to work with it.
-
Standardizes Coding Style: A beautifier can enforce a consistent coding style throughout the entire codebase, which is crucial when working as part of a team to maintain uniformity in aesthetics and structure.
-
Reduces Bugs and Errors: Properly formatted code minimizes the chances of introducing syntax errors caused by misalignments or misinterpretations of the code structure.
-
Increases Collaboration: Clean, readable code encourages collaboration among team members, as they may find it easier to discuss and implement changes in the code.
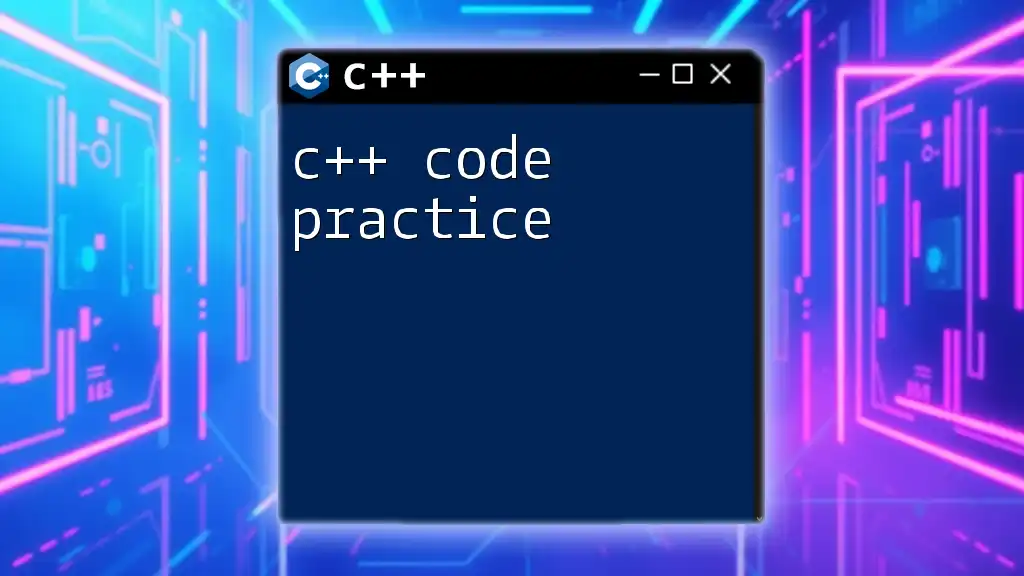
Popular C++ Code Beautifiers and Formatters
Overview of Top C++ Code Beautifiers
Several popular tools are available to help with C++ code beautification, each with its unique set of features and functionalities. The notable ones include ClangFormat, Astyle, and Uncrustify.
ClangFormat
Features of ClangFormat
ClangFormat offers a comprehensive and flexible way to format C++ code. It supports a wide range of options, including specifying indentation styles, spacing around operators, and line length constraints.
How to Install and Use ClangFormat
To start using ClangFormat, follow these installation steps:
-
Installation: Install Clang via your package manager or download it from the official LLVM website.
-
Basic Usage: To format your C++ files, you can use the following command:
clang-format -i yourfile.cpp
Here, the `-i` flag tells ClangFormat to edit the file in place. You can also use configurations to specify styles, like:
clang-format -i -style=Google yourfile.cpp
Astyle
Key Features of Astyle
Astyle (Artistic Style) is another powerful C++ code formatter that is highly customizable. It provides options for formatting function definitions, comments, and different brace styles, making it a versatile choice for developers.
Using Astyle
To get started with Astyle, follow these steps:
-
Installation: You can usually install Astyle via your package manager or download it from the official website.
-
Command for Beautification: To beautify your C++ code using Astyle, you can run:
astyle --style=allman yourfile.cpp
This command applies the Allman style of braces, which is a popular choice among many developers.
Uncrustify
Overview of Uncrustify
Uncrustify stands out for its capacity to support various coding standards and gives developers unparalleled flexibility with complex formatting configurations.
Installation and Basic Usage
To start using Uncrustify, follow these guidelines:
-
Installation: Install Uncrustify just like the other tools via your package manager or from its source.
-
Formatting Code: After configuring `uncrustify.cfg`, you can format your C++ file as follows:
uncrustify -c config.cfg --replace yourfile.cpp
This command uses your configuration file to determine how to format the input C++ file.
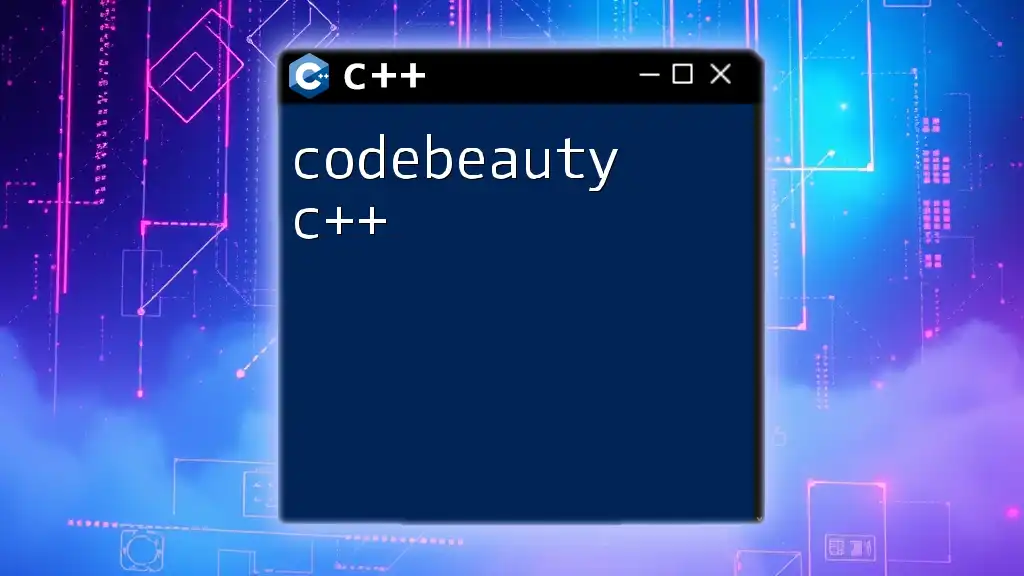
How to Beautify Your C++ Code
Conventional Code Style Guidelines
Adhering to conventional code style guidelines is essential for producing high-quality C++ code. Standards such as the Google C++ Style Guide and the LLVM Coding Standards offer invaluable insights and rules to follow.
These guidelines typically cover aspects like:
- Indentation: Use spaces or tabs consistently for indentation.
- Bracket Placement: Decide whether to place opening braces on the same line or on a new line.
- Naming Conventions: Establish consistent naming for variables, functions, and classes.
Basic Formatting Techniques
Indentation and Spacing
Proper indentation and spacing are vital for promoting clarity in code. This includes spacing around operators, after commas, and before and after braces.
Example of Poor vs. Beautified Code:
// Poorly formatted code
if(x<y){doSomething();}
// Beautified version
if (x < y) {
doSomething();
}
The beautified version not only is more readable but also clearly indicates the conditional structure to any developer reviewing it.
Line Length and Wrapping
Managing line length is crucial for the readability of your code. A common standard is to limit your lines to 80-120 characters.
Example of Properly Wrapped Lines:
// Long line
std::cout << "This is an example of a long line that needs to be wrapped properly.";
// Beautified with correct line wrapping
std::cout << "This is an example of a long line that "
"needs to be wrapped properly.";
By wrapping long lines, you create cleaner, more manageable code that is easier to navigate.
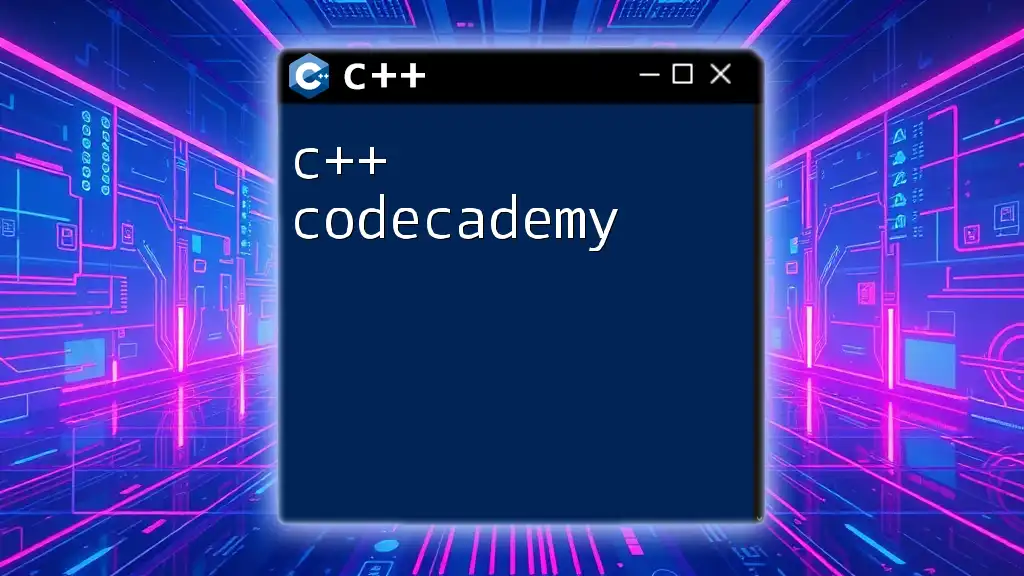
Automating Code Beautification
Integrating Beautification Tools into Your Workflow
To ensure that your C++ code is consistently beautified, you can integrate beautification tools into your development environment (IDE) or build process. This step provides automatic formatting whenever you save or compile your project, significantly improving code quality.
Using Pre-Commit Hooks
Implementing pre-commit hooks is an effective method to ensure that no poorly formatted code makes it into your version control system. Here’s how to set up a basic pre-commit hook for a Git repository:
Create a file named `pre-commit` in the `.git/hooks` directory and add the following script:
#!/bin/sh
clang-format -i *.cpp
This script will automatically format all C++ files in your repository whenever you attempt to commit changes, ensuring that all code adheres to your specified style.
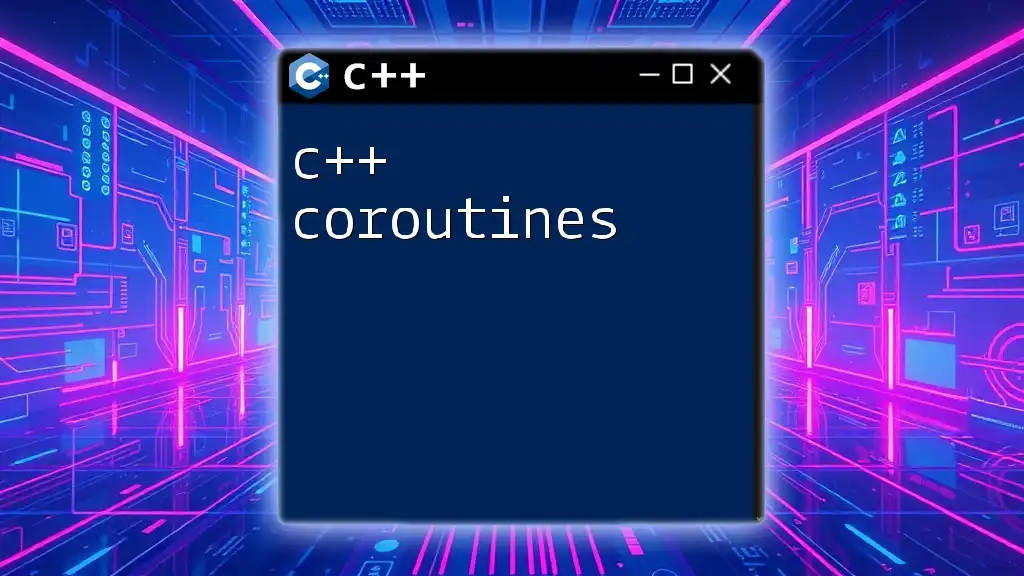
Conclusion
Recap of C++ Code Beautification
In summary, taking advantage of C++ code beautification not only enhances the readability of your code but also standardizes coding styles, reduces bugs, and fosters collaborative work among developers.
Encouragement to Adopt Best Practices
As you continue your journey with C++, embracing these beautification practices will lead you to write better and maintainable code, contributing positively to your workflow and development projects.
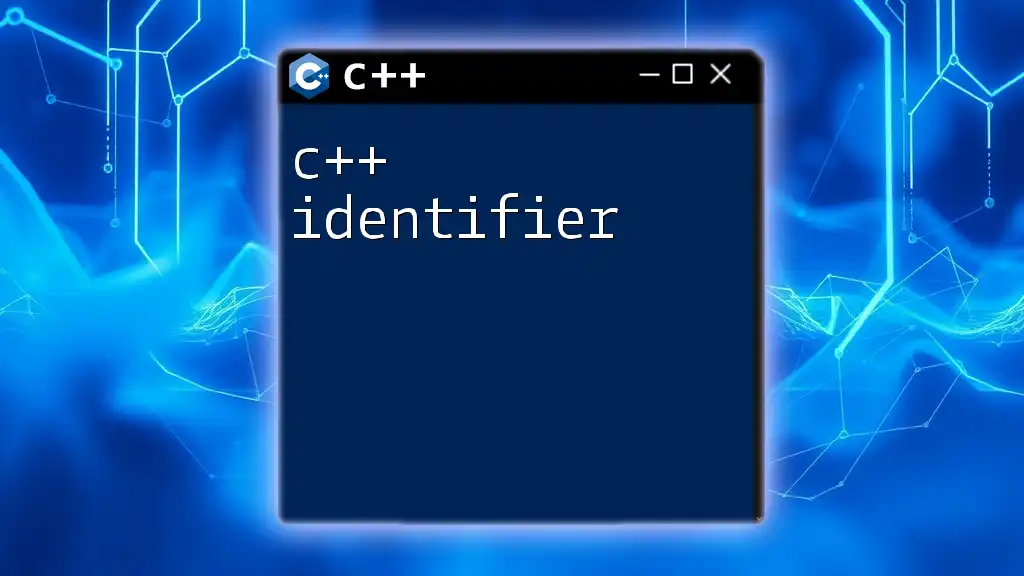
Additional Resources
Where to Learn More
Take some time to delve into prominent C++ resources. Documentation for ClangFormat, Astyle, and Uncrustify will provide additional insights into how each tool can be utilized effectively.
Join the Community
Engaging with community forums and groups for C++ developers will empower you with knowledge sharing and best practices that further enrich your C++ coding experience.