Coverity is a static analysis tool that helps developers identify and fix security vulnerabilities and defects in C++ code before it is deployed.
Here’s a simple code snippet demonstrating how to analyze a C++ program with Coverity:
// Example C++ code for Coverity analysis
#include <iostream>
void checkDivision(int a, int b) {
if (b != 0) {
std::cout << "Result: " << a / b << std::endl;
} else {
std::cout << "Error: Division by zero" << std::endl;
}
}
int main() {
checkDivision(10, 0);
return 0;
}
This snippet includes a function that ensures safe division, which can be analyzed by Coverity for potential issues.
What is Coverity?
Coverity is a powerful static code analysis tool designed to identify and remediate software defects early in the development lifecycle. By scanning source code without executing it, Coverity helps developers find potential security vulnerabilities, performance issues, and coding errors that might lead to expensive fixes later. This is particularly important for C++ developers, who often navigate complex memory management issues and intricate syntax that can introduce subtle bugs.
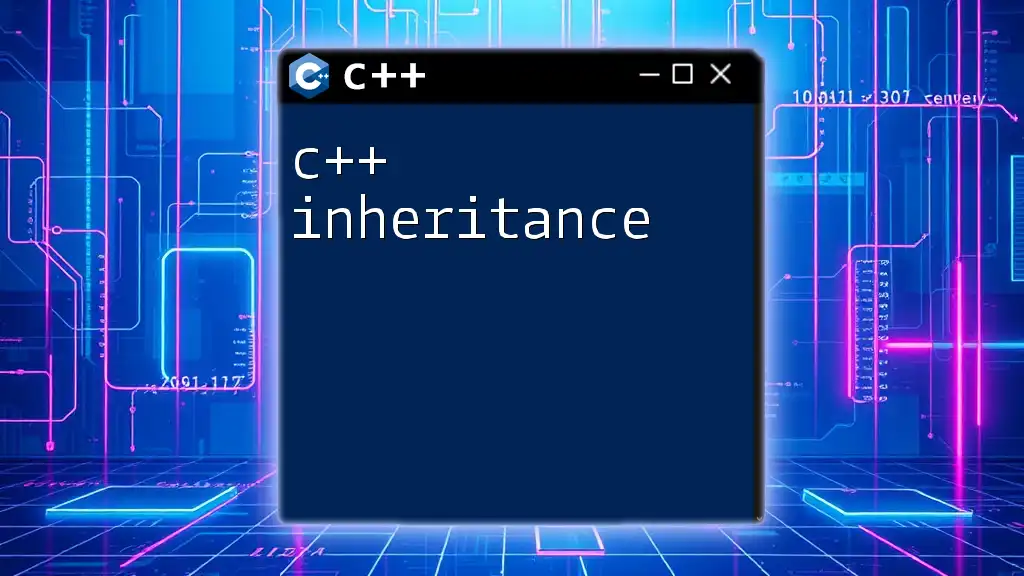
Why Use Coverity for C++?
C++ is a language known for its performance and flexibility, but it also comes with inherent risks. Common vulnerabilities such as buffer overflows, null pointer dereferences, and memory leaks can plague C++ projects. Coverity excels at detecting these types of issues, providing developers with detailed insights into potential weaknesses within their code. By using Coverity, C++ developers can ensure improved code quality and security compliance.
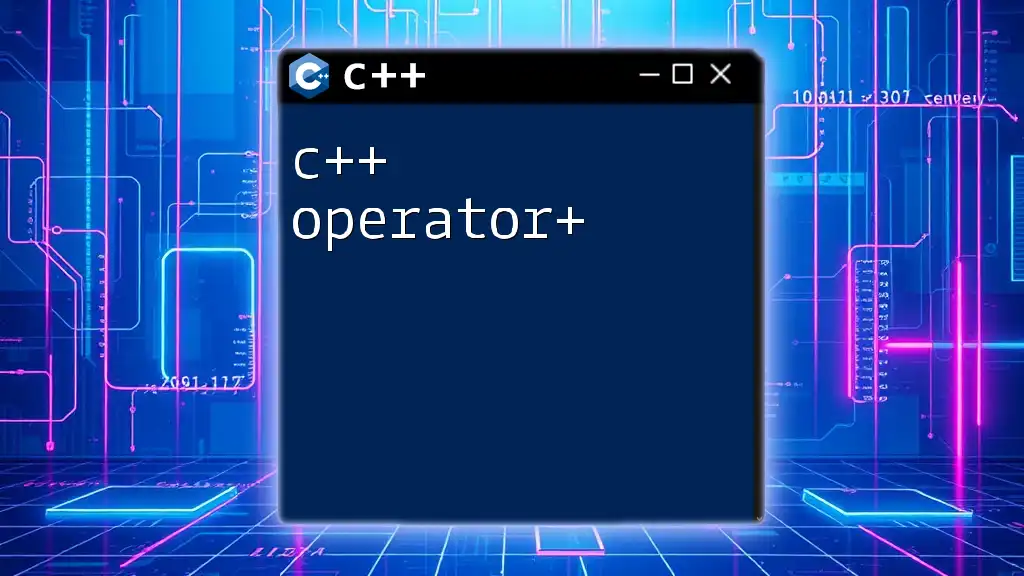
Understanding Static Code Analysis
What is Static Code Analysis?
Static code analysis is a method of examining source code for potential vulnerabilities, bugs, and code quality issues without running the program. Unlike dynamic analysis, which requires code execution, static analysis can be performed on uncompiled code, offering developers insights much earlier in the software development lifecycle.
How Coverity Performs Static Analysis
Coverity employs advanced algorithms, including data flow analysis and control flow analysis, to examine the structure and execution paths of code. This allows it to pinpoint vulnerabilities that traditional testing methods might miss. For instance, Code that appears valid might inadvertently lead to security holes; Coverity helps illuminate these hidden dangers.
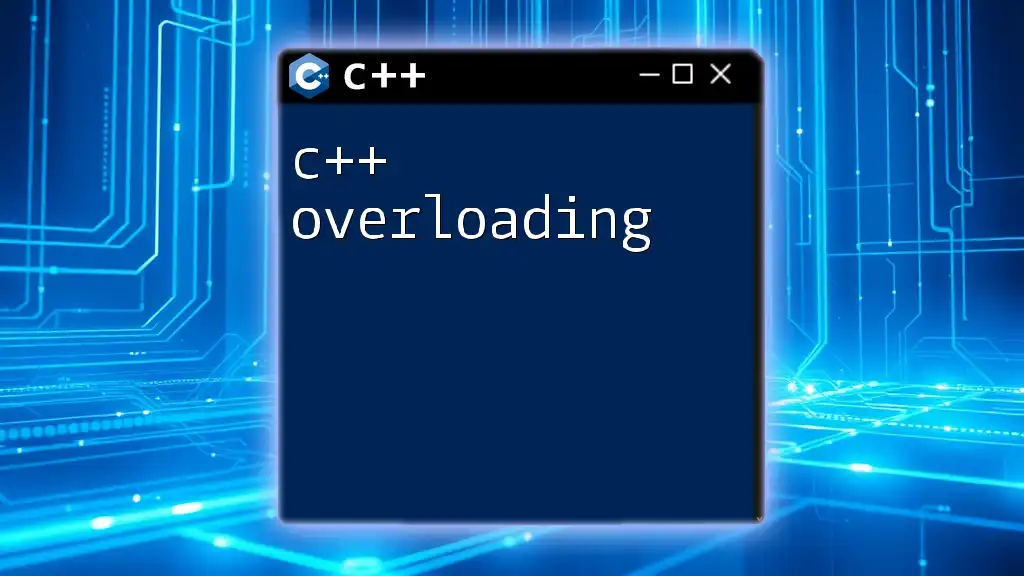
Setting Up Coverity for C++
Initial Requirements
Before getting started with Coverity, ensure your development environment meets the necessary prerequisites. This includes having a compatible operating system (Windows or Linux), a supported C++ compiler, and sufficient hardware resources to run the analyses.
Installation Process
Installing Coverity can vary slightly depending on your operating system. Here’s a basic guide for both Windows and Linux:
For Windows:
- Download the Coverity installer from the official website.
- Run the installer and follow the on-screen instructions.
For Linux:
- Use the terminal to download the Coverity installation package.
- Extract the files and run the installation script:
sudo ./install_script.sh
Configuration for C++ Projects
Once installed, configuring Coverity to analyze your C++ project is crucial. This process includes specifying the project structure and compiler paths in the configuration file. Below is a simple example of how this might look:
# Coverity Configuration
[project]
name = MyCPlusPlusProject
language = cpp
[compiler]
path = /usr/bin/g++
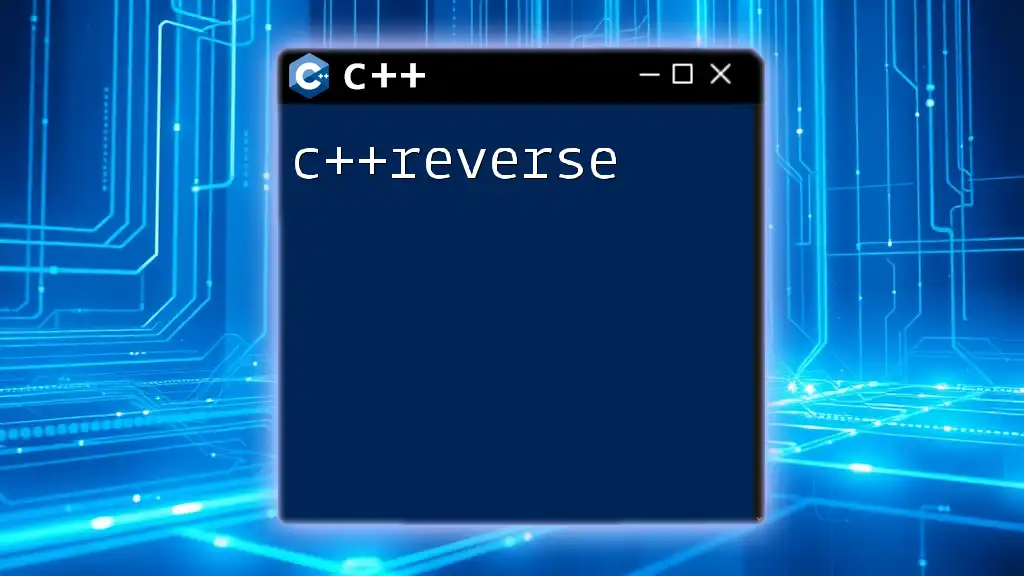
Analyzing C++ Code with Coverity
Running Analysis
To initiate a code analysis in Coverity, navigate to your project directory in the terminal and execute the following command:
cov-build --dir cov-int make
This command compiles the project while collecting necessary information for the analysis. After the build process, you can run the analysis itself using:
cov-analyze --dir cov-int
Interpreting Results
After the analysis completes, Coverity generates a report detailing any identified issues. Understanding this output is essential; it will categorize findings into critical defects, potential issues, and informational notices. This helps developers prioritize their fixes, addressing the most severe problems first.
Categorizing Defects
Coverity classifies defects according to their severity and type. For example, critical defects may include buffer overflows, while lesser issues might involve style violations. Each defect reported contains detailed information, including the location within the code and potential fixes.
Example: A common defect might be a null pointer dereference:
void myFunction(MyObject* obj) {
if (obj->someMethod()) { // Potential null pointer dereference
// Code logic here
}
}
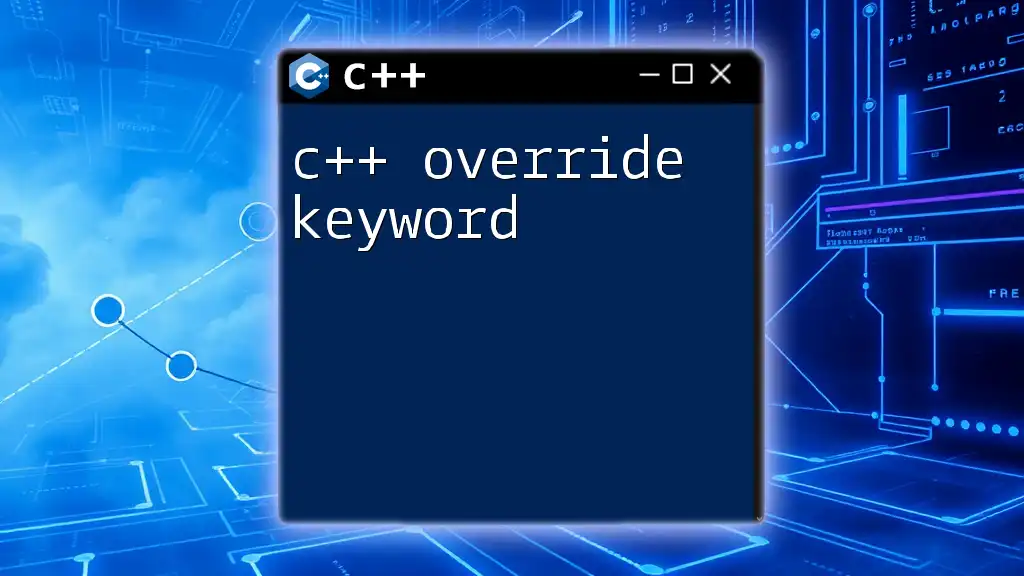
Best Practices for Using Coverity
Integrating Coverity into Your Development Workflow
To maximize the benefits of Coverity, integrate it into your CI/CD pipeline. This ensures that every commit to your codebase undergoes review, facilitating immediate detection of issues.
Example: Setting up Coverity with Jenkins can be done by adding a build step in your Jenkins job, specifying the command to run Coverity analysis.
Routine Code Reviews with Coverity
Conduct regular code reviews using Coverity findings. By combining automated analysis with human oversight, teams can uncover complex issues that automated tools may overlook.
Fixing Defects Reported by Coverity
When addressing defects identified by Coverity, it’s important to not only resolve the issue but also understand the root cause. For instance, if you encounter a memory leak, review how memory is allocated and freed in your code.
Code Snippet: Before and after a fix for a memory leak:
Before:
void memoryLeakExample() {
MyObject* obj = new MyObject(); // Memory allocated
// usage of obj
// obj is never deleted
}
After:
void memoryLeakExample() {
MyObject* obj = new MyObject(); // Memory allocated
// usage of obj
delete obj; // Memory properly freed
}
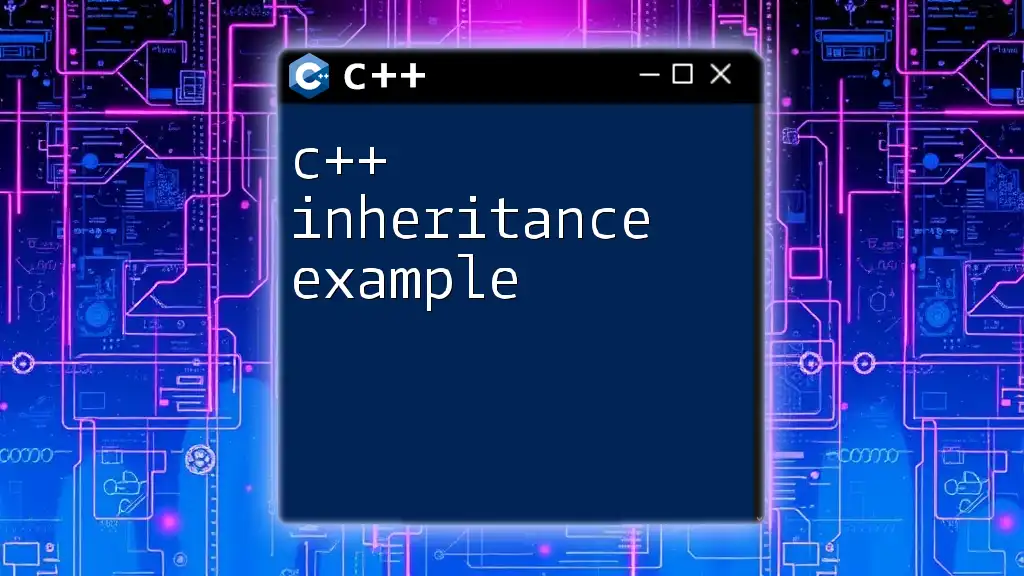
Advanced Features of Coverity
Customizing Coverity Analysis
Coverity allows developers to customize their analysis settings based on project needs. Creating custom rules tailored to specific coding standards or organizational policies can enhance the effectiveness of the tool.
Example: You can define custom rules in a separate configuration file and inform Coverity to apply these during analysis.
Using Coverity’s Reporting Tools
Coverity provides robust reporting features, allowing teams to generate reports and track metrics over time. This capability fosters transparency among stakeholders and helps illustrate improvements in code quality.
Example: You might generate a report showing reduced defect density over several iterations:
cov-format-errors --output myReport.txt
Integrating with Other Development Tools
Integrating Coverity with version control systems like Git or issue trackers like Jira can streamline the defect management process. For instance, upon identifying a defect, Coverity can automate ticket creation in Jira, assigning it to relevant team members.
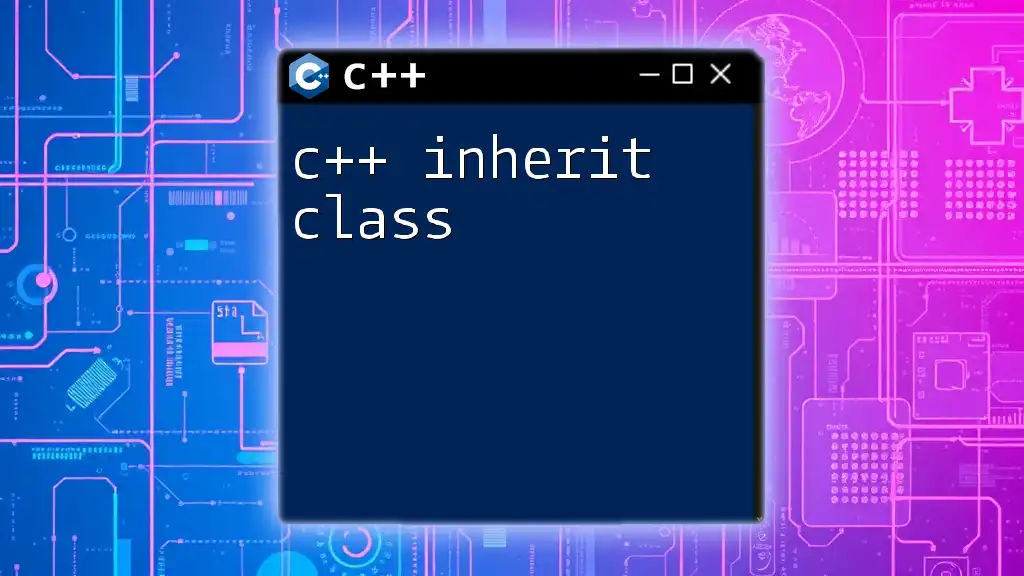
Case Studies: Successful Implementation of Coverity in C++ Projects
Industry Examples
Several industries have successfully implemented Coverity to enhance their software development processes. Notable companies in finance and gaming have reported significant reductions in defects by leveraging this tool.
Analysis of Results
These case studies often demonstrate quantifiable improvements, such as a decrease in the number of high-severity defects, shorter test cycles, and higher overall software security compliance.
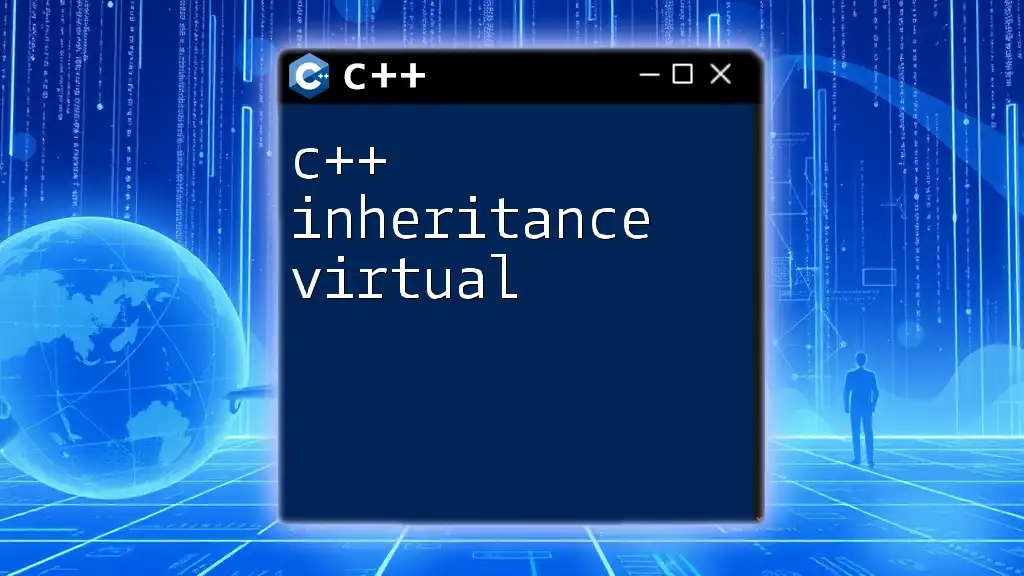
Conclusion
Utilizing C++ Coverity can dramatically enhance your code quality and security posture. By integrating static code analysis early into your development workflow, you not only save time on debugging later but also foster a culture of quality within your development team. As C++ continues to evolve, so too will the tools and practices we use to write secure and efficient code.
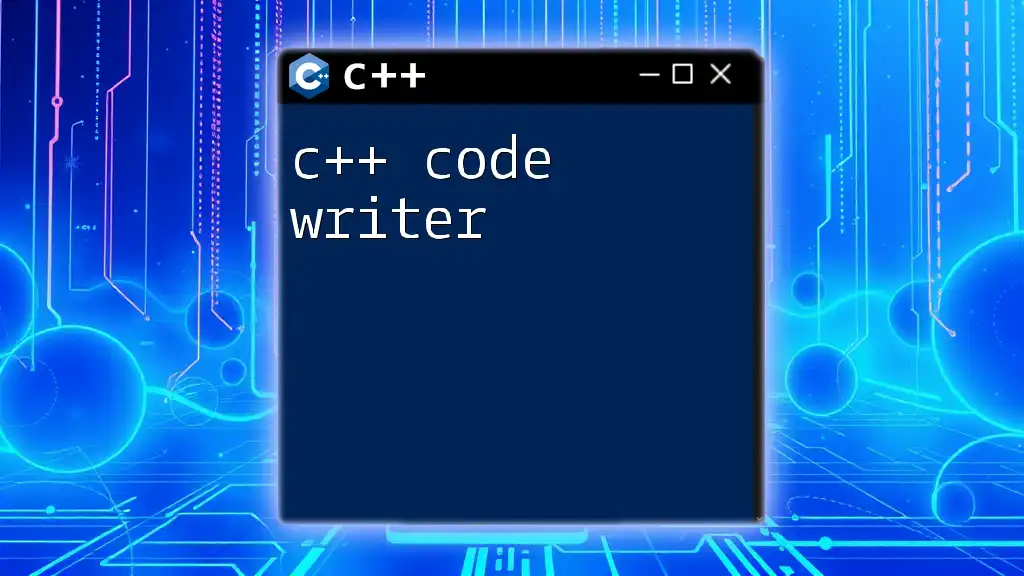
Additional Resources
Links to Documentation
For further reading and to access the official Coverity documentation, you can visit Coverity's website.
Further Reading
Consider exploring books and articles on C++ best practices and secure coding standards to elevate your understanding beyond static analysis tools.