The `operator delete` function in C++ is used to deallocate memory previously allocated for an object, ensuring proper cleanup and memory management.
Here's a code snippet demonstrating its usage:
#include <iostream>
class MyClass {
public:
MyClass() { std::cout << "Object created\n"; }
~MyClass() { std::cout << "Object destroyed\n"; }
};
int main() {
MyClass* obj = new MyClass(); // Allocating memory for an object
delete obj; // Deallocating memory using operator delete
return 0;
}
Understanding Dynamic Memory Allocation
What is Dynamic Memory Allocation?
Dynamic memory allocation allows a program to request memory as needed during its execution, rather than relying on pre-defined, static memory sizes. This flexibility is crucial for handling data structures like linked lists, trees, and more. Unlike stack memory, which is automatically managed via function calls, heap memory must be managed manually, giving programmers control but also introducing complexity.
How Memory Allocation Works in C++
In C++, memory allocation is accomplished primarily through two operators: `new` and `delete`. When you use the `new` operator, you allocate memory on the heap, which persists until it's explicitly released using the `delete` operator. This separation allows for dynamic data structures, but it also means that programmers must be vigilant about freeing memory to avoid issues like memory leaks.
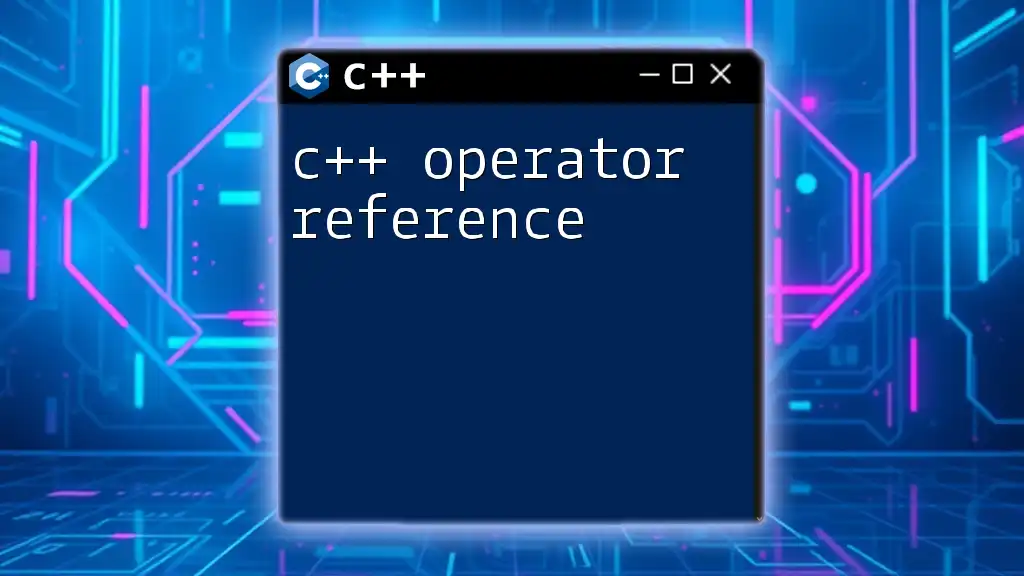
The `delete` Operator in C++
What is the `delete` Operator?
The `delete` operator in C++ is essential for deallocating memory that was previously allocated using the `new` operator. Unlike the `free` function in C, which releases memory allocated by `malloc`, `delete` also calls the destructor of the object (if it is a class type) before deallocating the memory. This ensures that any cleanup operations defined in the class destructor are performed.
Syntax of the `delete` Operator
The syntax for `delete` is straightforward. You simply follow the `delete` keyword with the pointer to the allocated memory. For example:
int* ptr = new int(10);
delete ptr; // This releases the allocated memory
This line of code allocates memory for an integer, initializes it to `10`, and then properly frees that memory when it is no longer needed.
The `delete[]` Operator
When allocating an array with `new`, you must use `delete[]` to release that memory. Using `delete` instead of `delete[]` can lead to undefined behavior.
Here's how to correctly delete an array:
int* arr = new int[5];
delete[] arr; // This releases the allocated array memory
Utilizing `delete[]` ensures that the destructors of each element in the array (if applicable) are called.
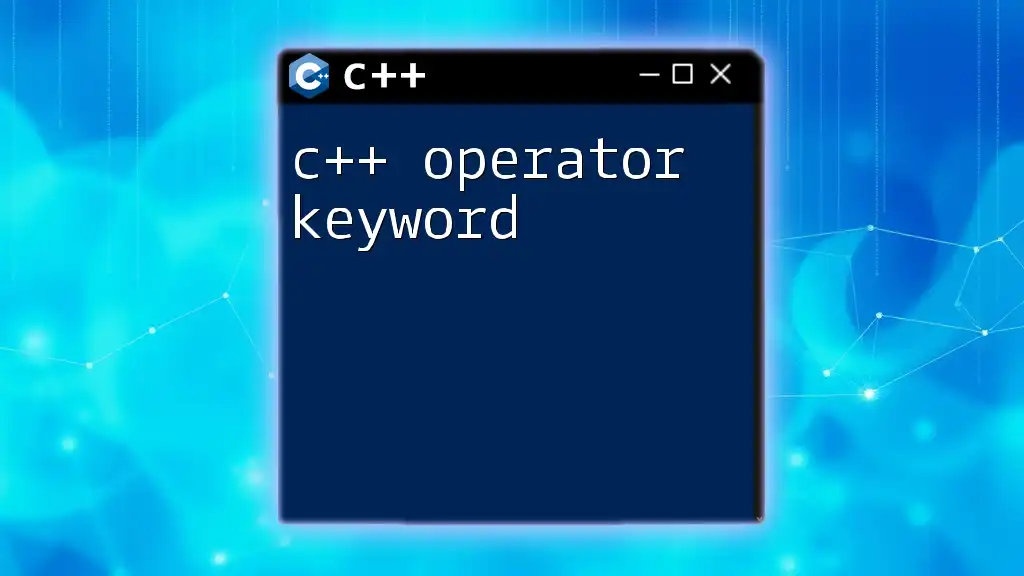
Manual Memory Management with `delete`
The Risks of Manual Memory Management
Manual memory management with `new` and `delete` can introduce several risks, primarily memory leaks and fragmentation. A memory leak occurs when you allocate memory but fail to release it, leading to a gradual decrease in available memory in your application. Fragmentation happens when memory is allocated and deallocated in such a way that it causes discontinuity, which can lead to inefficient memory usage.
Best Practices for Using `delete`
To maintain clean code and effective memory management, adhere to these best practices:
- Always pair `new` with `delete`, and `new[]` with `delete[]`. This ensures proper deallocation of resources.
- Set pointers to `nullptr` after deletion. This prevents dangling pointers, which can lead to undefined behavior if accessed after deletion.
Here's an example demonstrating these practices:
int* num = new int(5);
delete num;
num = nullptr; // Prevents dangling pointer
By setting `num` to `nullptr`, you can safely check before any further operations.
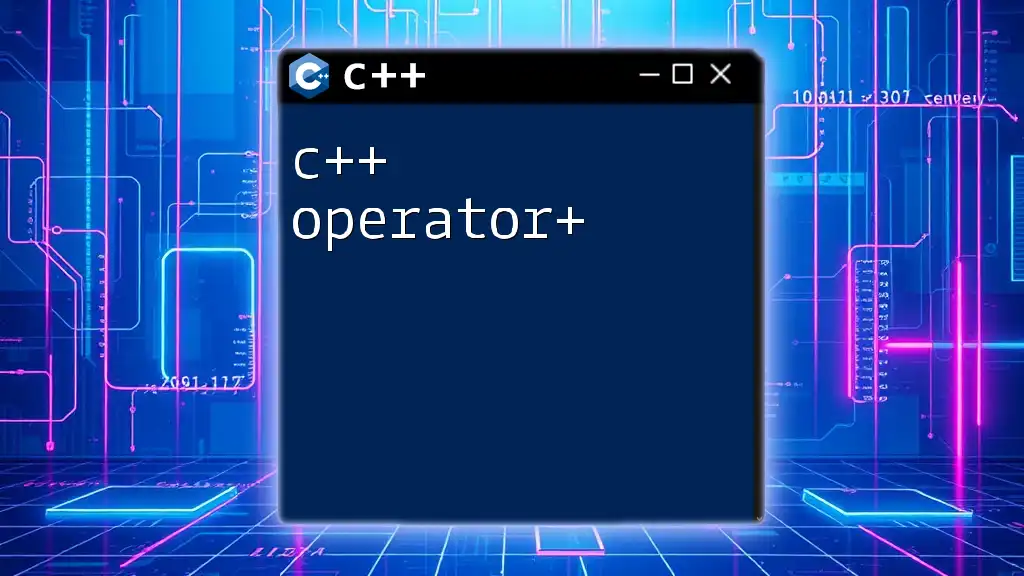
Implementing Custom `delete` Operators
Overloading the `delete` Operator
C++ allows for the overloading of the `delete` operator, which can be useful for tracking memory usage or implementing custom deallocation strategies. To overload the `delete` operator, you define it as a member function within a class.
Here’s a simple example:
class MyClass {
public:
void* operator new(size_t size) {
return ::operator new(size); // Custom allocation logic (if any)
}
void operator delete(void* pointer) {
// Custom deallocation logic (if any)
::operator delete(pointer);
}
};
Use Cases for Custom Delete Operators
Custom delete logic might be useful in scenarios such as:
- Logging: You might want to log each deallocation for debugging.
- Tracking Memory Usage: Keeping track of memory allocations and deallocations could help in resource management, especially in large applications with dynamic behavior.
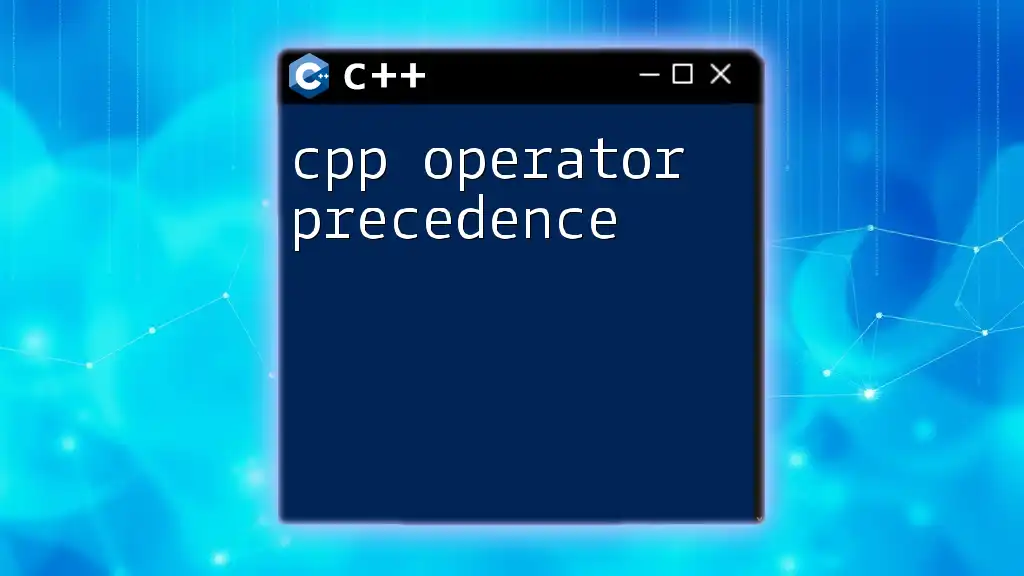
The Relationship Between Constructors, Destructors, and `delete`
How Constructors and Destructors Work
In C++, every class can have a constructor (called when an object of the class is created) and a destructor (called when the object goes out of scope or is deallocated). These special member functions are critical for managing resources, including dynamic memory.
Using `delete` in Constructor/Destructor
It's common to release resources in a class's destructor using `delete`. For example:
class Example {
int* data;
public:
Example() : data(new int[10]) {} // Allocate memory in constructor
~Example() { delete[] data; } // Release memory in destructor
};
In this code snippet, `data` is allocated when an `Example` object is created, and the memory is automatically released when the object is destructed.
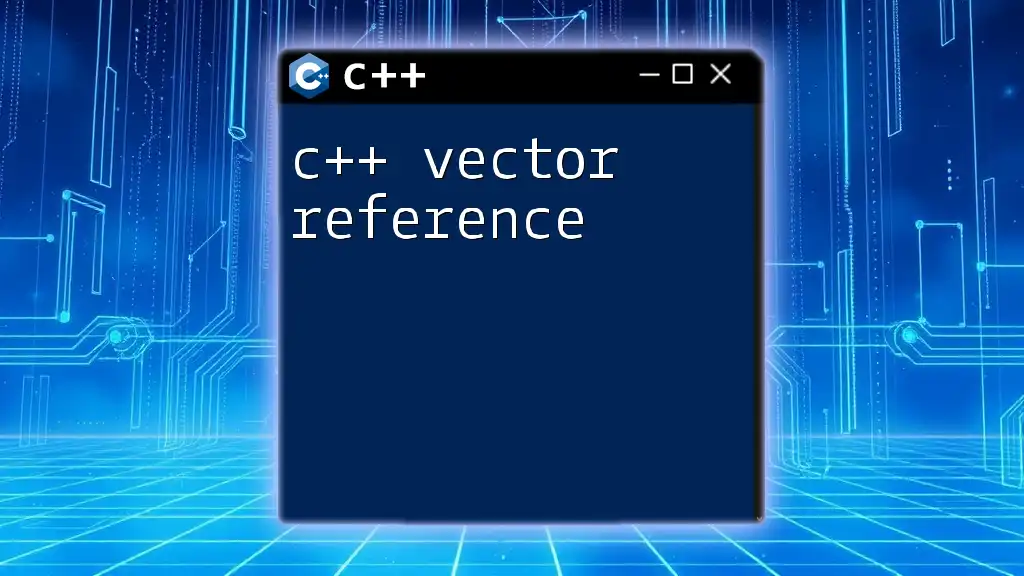
Common Pitfalls and How to Avoid Them
Forgetting to Call `delete`
Forgetting to release dynamically allocated memory is a common mistake that can lead to memory leaks. These leaks can accumulate over time, impacting performance and leading to potential crashes. Being diligent about calling `delete` when memory is no longer needed is crucial in managing resources effectively.
Double Deleting Pointers
A more severe mistake is double deleting a pointer. This occurs when the same memory is deallocated more than once, resulting in undefined behavior. To avoid this problem, ensure that pointers are not accidentally deleted twice:
int* ptr = new int(10);
delete ptr;
// delete ptr; // This causes undefined behavior
To safeguard against this, always set pointers to `nullptr` after deletion.
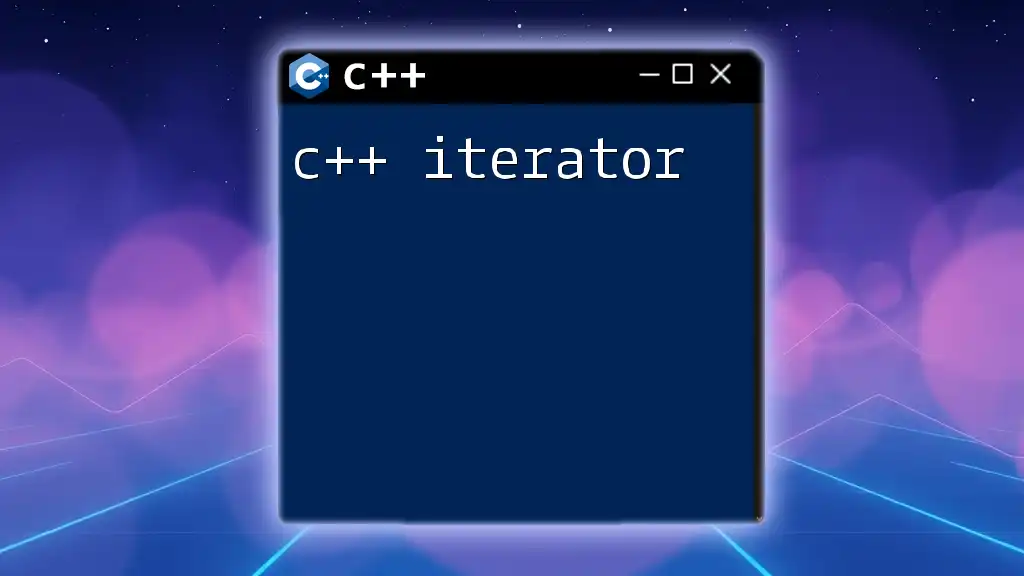
Modern Alternatives to Manual Memory Management
Smart Pointers: A Modern C++ Approach
In modern C++, the introduction of smart pointers, such as `std::unique_ptr` and `std::shared_ptr`, represents a significant advancement in managing dynamic memory. These smart pointers automatically handle memory deallocation when they go out of scope, reducing the chances of memory leaks and dangling pointers.
Here’s how to utilize a `std::unique_ptr`:
#include <memory>
std::unique_ptr<int> smartPtr(new int(100)); // Automatically deallocates memory
With `smartPtr`, memory is released automatically when the pointer is no longer needed, which makes memory management safer and easier for developers.
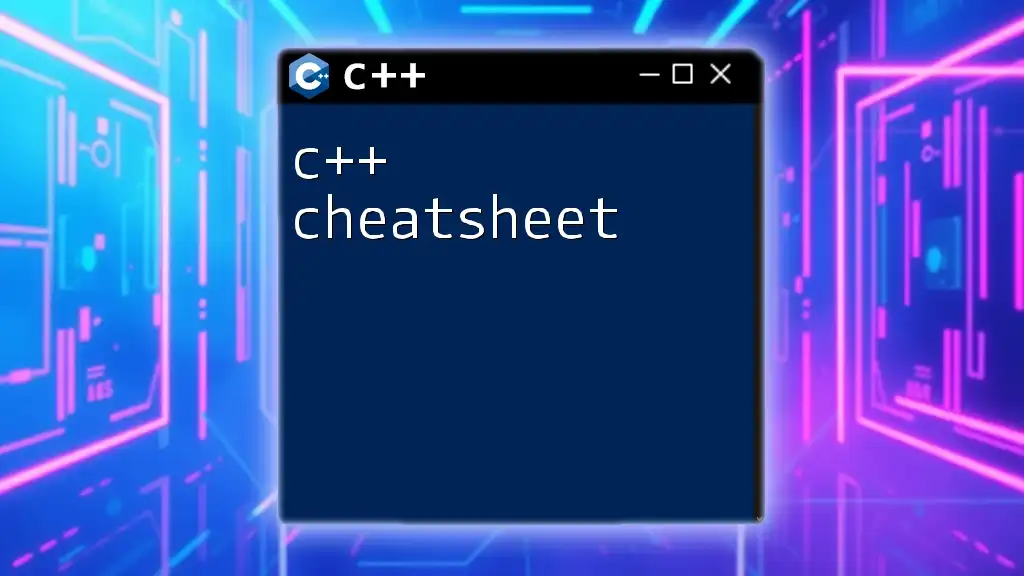
Conclusion
In conclusion, understanding the C++ operator delete is essential for effective memory management in your applications. By adhering to best practices, avoiding common mistakes, and leveraging modern alternatives like smart pointers, you can build safer, more efficient C++ programs. Proper handling of dynamic memory will not only improve the performance of your applications but also enhance their stability and correctness.