C++ desktop development involves creating applications that run on desktop operating systems using the C++ programming language, allowing for efficient system-level programming and high-performance applications.
Here's a simple "Hello, World!" example in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is Desktop Development?
Desktop development refers to the process of creating applications that run on desktop operating systems, like Windows, macOS, and Linux. These applications are designed to interact directly with the end-user via a graphical user interface (GUI), allowing for a rich user experience. Desktop applications are prevalent because they often require more resources and provide richer features than web applications.
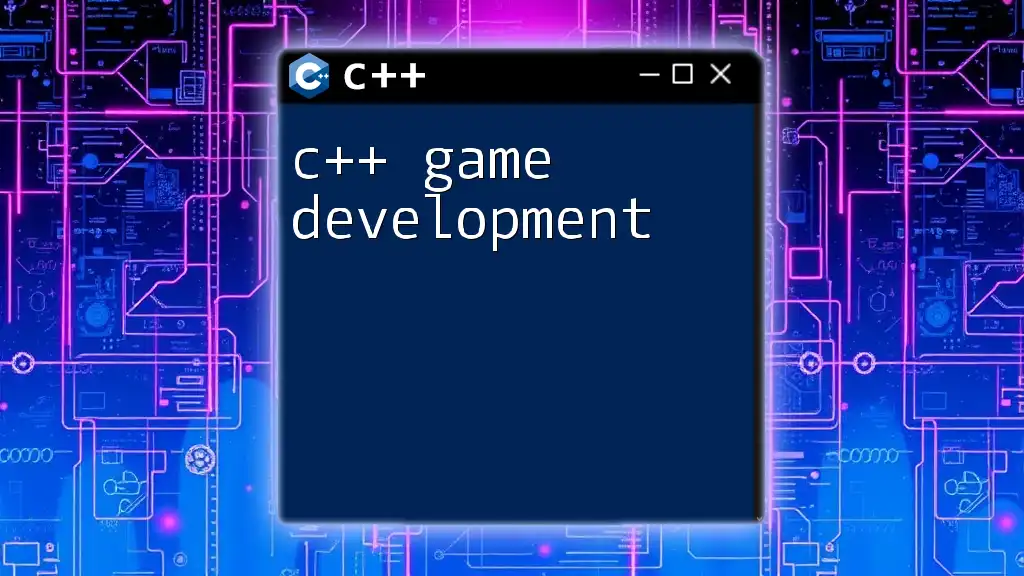
Why Use C++ for Desktop Development?
C++ is a powerful language widely used in desktop development due to its performance, control over system resources, and cross-platform capabilities. It compiles to native code, allowing applications to run efficiently. Additionally, C++ offers low-level memory management features, enabling developers to optimize their applications for resource-intensive tasks.
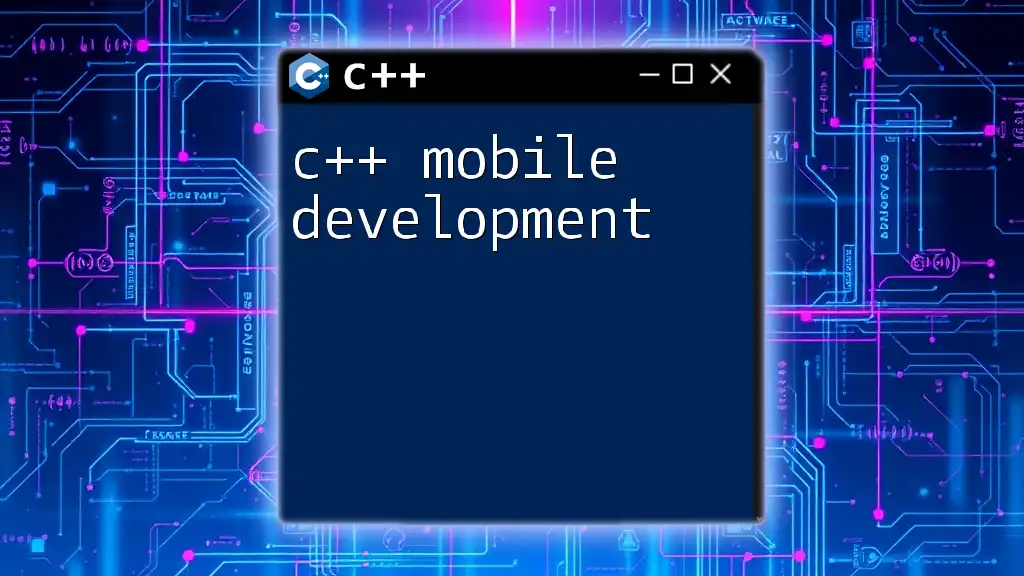
Setting Up Your Development Environment
Choosing an IDE for C++ Development
Selecting the right Integrated Development Environment (IDE) is crucial for productivity in C++ development. Some popular IDEs include:
- Visual Studio: Offers a robust debugger and excellent integration for Windows applications.
- Code::Blocks: A free, open-source IDE that is highly customizable.
- Qt Creator: Tailored for applications using the Qt framework, it's easy to use with powerful features.
Each of these IDEs has its strengths, so consider your project requirements when making a choice.
Installing Required Libraries
Most desktop applications depend on libraries to provide GUI elements or other essential features. Commonly used libraries include Qt, wxWidgets, and GTK+. Here’s a simple example of installing Qt on a Debian-based Linux system:
sudo apt install qt5-default
By leveraging these libraries, you can streamline your development process while adding functionality to your applications.
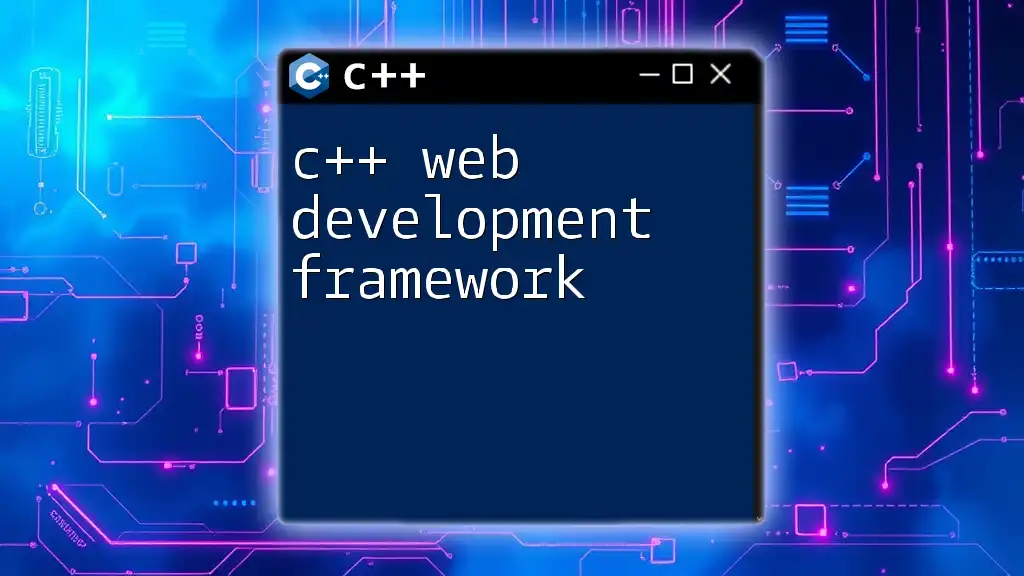
Fundamentals of C++ Desktop Application Design
Understanding the MVC Pattern
The Model-View-Controller (MVC) design pattern is fundamental in desktop application development. In MVC, the Model represents the data and business logic, the View displays the user interface, and the Controller mediates user input, updating the model or view as necessary. This separation of concerns enhances maintainability and testability of desktop applications.
Event-Driven Programming in C++
Graphic user interfaces are primarily built around event-driven programming, where the application reacts to user actions (like mouse clicks and keyboard input). Understanding how to create an event loop and handle events is crucial in developing responsive applications. Here’s a simple example of an event loop structure in a C++ application:
while (isRunning) {
processEvents();
render();
}
This loop will continuously check for events and update the GUI accordingly.
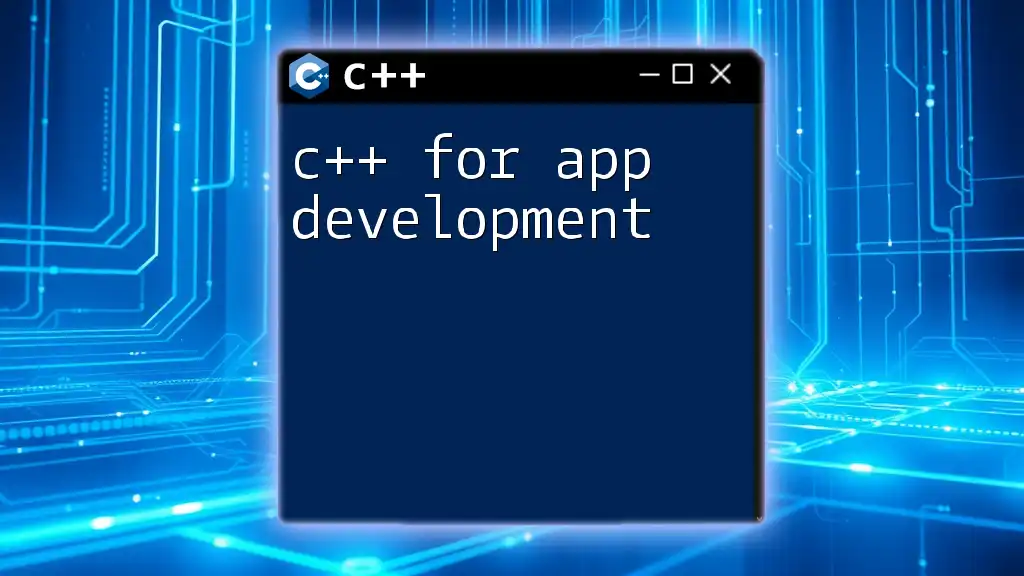
Creating Your First Desktop Application
Setting Up the Project Structure
Every C++ desktop project should begin with a structured directory layout. A typical project might include folders for:
- Source files (src): Your C++ files containing application logic.
- Headers (include): Header files for function declarations and class definitions.
- Resources: Any assets needed, such as images or configuration files.
Writing Your First C++ GUI Application
Let’s create a simple "Hello, World!" application using the Qt framework. Below is a step-by-step guide:
-
Set up the project in your IDE and create a new Qt Widgets Application.
-
Write the main code in your main.cpp file:
#include <QApplication>
#include <QPushButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Hello, World!");
button.resize(200, 100);
button.show();
return app.exec();
}
This code initializes a Qt application, creates a button that displays "Hello, World!", and runs the application until the user closes it.
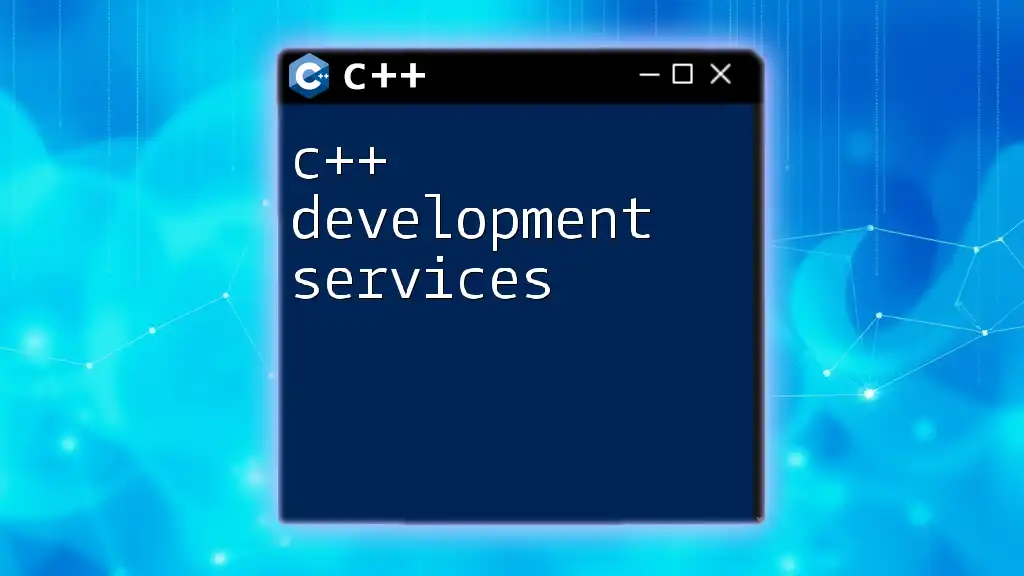
Introduction to GUI Libraries
Overview of Popular C++ GUI Libraries
- Qt: A comprehensive framework that supports complex UI elements and is cross-platform.
- wxWidgets: Provides a native look and feel across different platforms with a simpler API compared to Qt.
- GTK+: Mainly used in Linux, it's known for its speed and ease of integration with GNOME applications.
Each of these libraries has distinct characteristics, making them suitable for different types of projects.
Comparing C++ GUI Libraries
Here is a quick comparison of the libraries mentioned:
Feature | Qt | wxWidgets | GTK+ |
---|---|---|---|
Platform Support | Cross-Platform | Cross-Platform | Primarily Linux |
Learning Curve | Moderate | Low | Moderate |
Community Support | Large | Moderate | Large |
Choosing the right GUI library often depends on the specific requirements of your application, such as the target operating system and desired user experience.
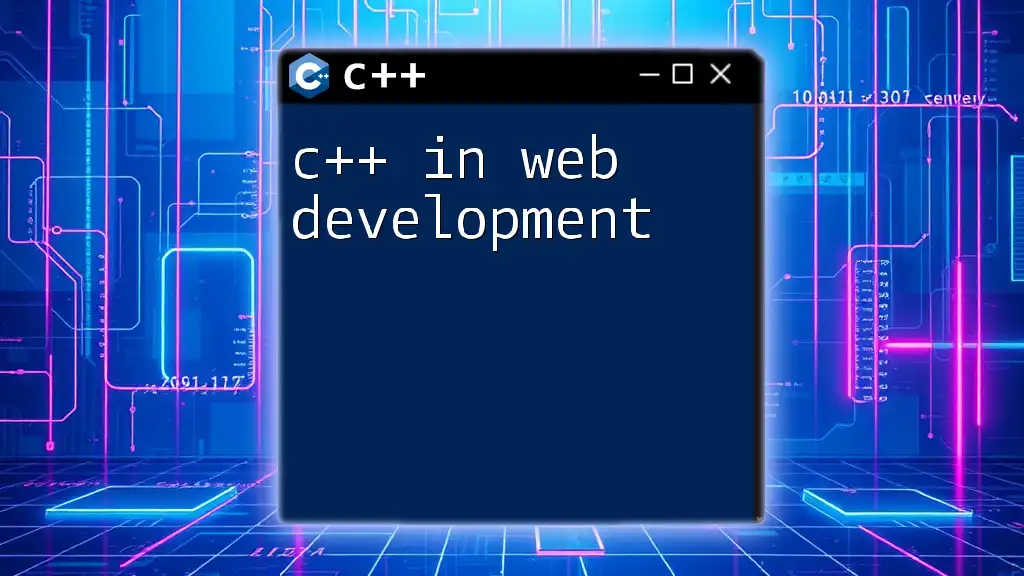
Advanced Desktop Development Topics
Implementing Multithreading in Desktop Applications
For more interactive applications, you can implement multithreading using C++11’s threading features. Multithreading is critical for maintaining responsive applications, especially when handling time-consuming tasks. Here’s a simple example of creating a thread:
#include <thread>
#include <iostream>
void ThreadFunction() {
std::cout << "Thread is running.\n";
}
int main() {
std::thread myThread(ThreadFunction);
myThread.join(); // Wait for the thread to finish
return 0;
}
Handling File Operations
Desktop applications often require file I/O for data persistence. C++ provides robust standard libraries to manage file operations efficiently. Here’s an example of reading from a file:
#include <fstream>
#include <iostream>
#include <string>
int main() {
std::ifstream inputFile("data.txt");
std::string line;
if (inputFile.is_open()) {
while (getline(inputFile, line)) {
std::cout << line << '\n';
}
inputFile.close();
}
return 0;
}
This snippet reads a file line-by-line and prints its contents on the console.
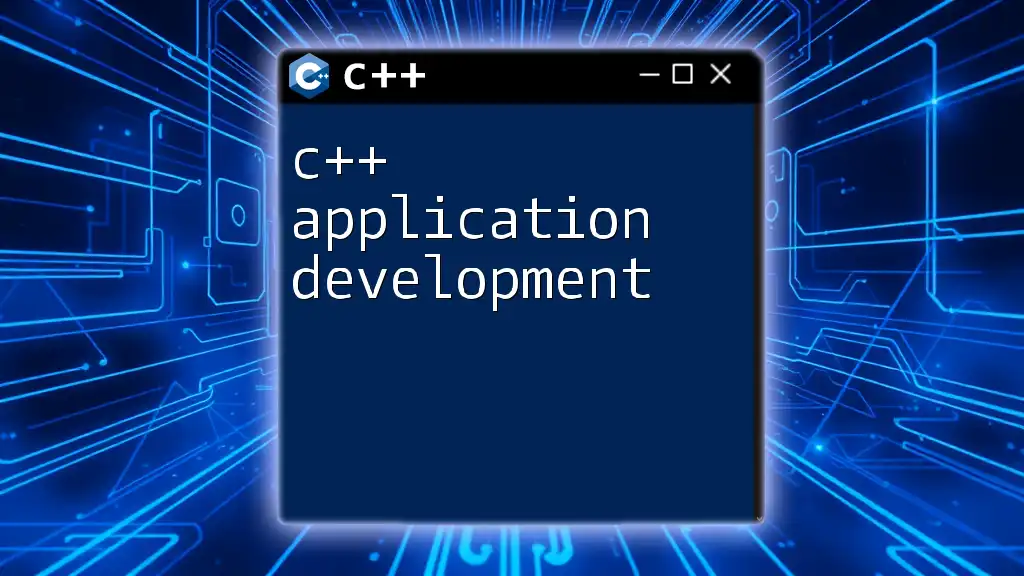
Testing and Debugging Your Desktop Application
Unit Testing in C++
Unit testing helps ensure that your application behaves as expected. Google Test is a popular framework for writing unit tests in C++. Here’s a simple test case example:
#include <gtest/gtest.h>
int Add(int a, int b) {
return a + b;
}
TEST(AddTest, PositiveNumbers) {
EXPECT_EQ(Add(1, 2), 3);
}
Debugging Techniques
Debugging is a critical part of desktop development. Utilizing the built-in debugging tools provided by your IDE can help you set breakpoints, inspect variables, and step through your code. Additionally, logging can serve as a useful technique for tracking application behavior without halting execution.
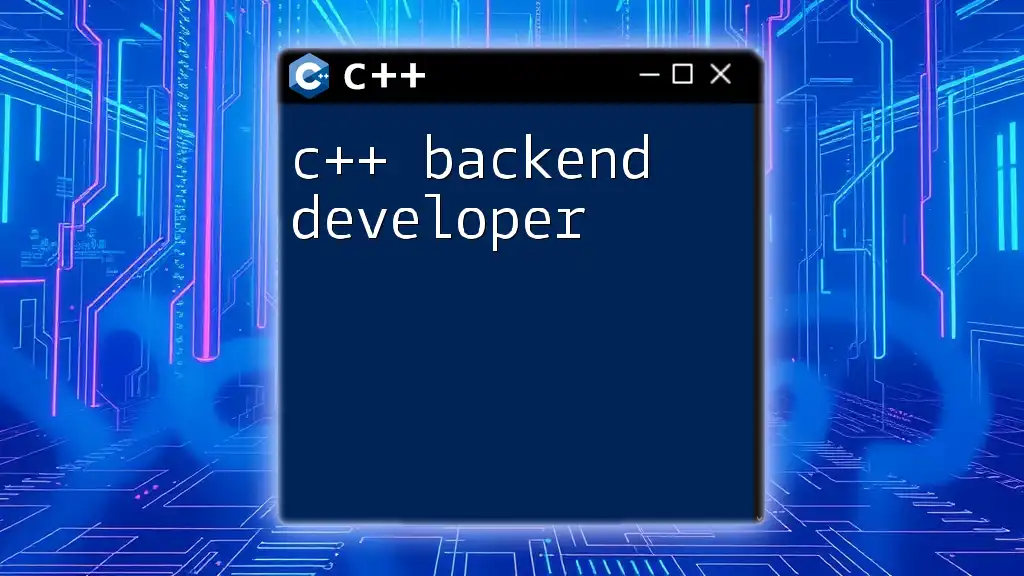
Deploying Your C++ Desktop Application
Building Executables for Various Platforms
When your application is complete, building it for different platforms is essential. Each OS may have specific requirements or needs different builds. Familiarize yourself with the platform's compile and link processes.
Creating Installation Packages
Packaging your application for distribution enhances the user experience. Tools like NSIS for Windows allow you to craft an installation script to guide users through setup. Here’s a simple installation script example:
!define PRODUCT_NAME "MyApp"
OutFile "MyAppInstaller.exe"
InstallDir "$PROGRAMFILES\MyApp"
Section "MainSection"
SetOutPath "$INSTDIR"
File "MyApp.exe"
SectionEnd
This script creates an installer for your application that installs it in the Program Files directory.
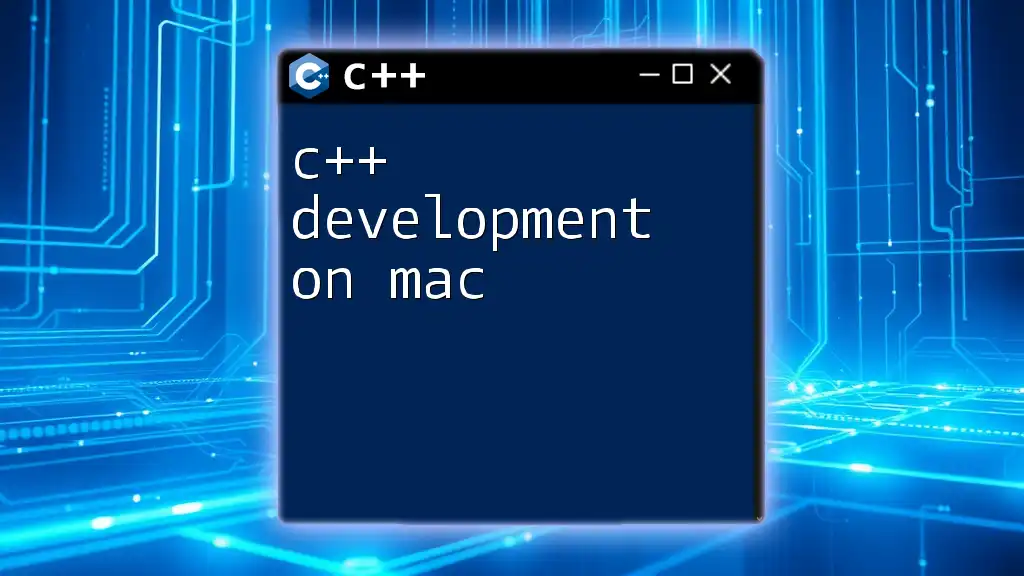
Conclusion
Exploring C++ desktop development opens up vast opportunities for creating powerful, efficient applications suited to desktop environments. By understanding fundamental principles like MVC pattern, event-driven programming, and utilizing libraries, you can create rich, interactive applications that leverage the full power of C++. Continued learning through practice, exploring advanced topics, and understanding best practices will prepare you to tackle more significant projects in the future.
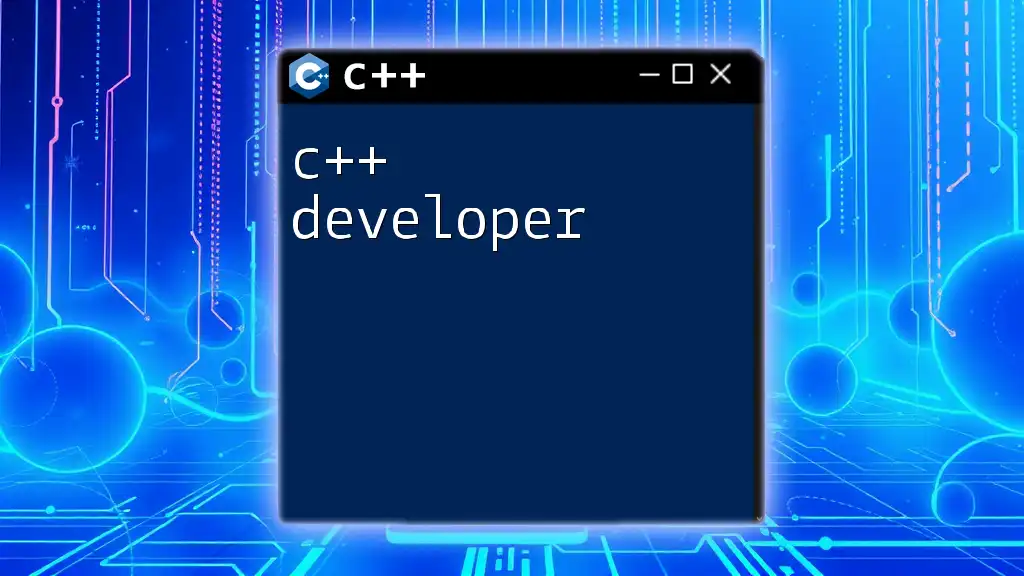
Next Steps and Resources
To further your knowledge in C++ desktop development, consider diving deeper into advanced concepts like graphics programming, exploring various C++ frameworks, or contributing to open-source projects. Engaging with communities and forums can provide support and inspiration as you create your digital solutions.