A C++ web development framework provides tools and libraries that simplify the creation of web applications using C++, enabling developers to build dynamic and scalable server-side solutions efficiently.
Here’s a simple example of a C++ web server using the Crow framework:
#include "crow_all.h"
int main()
{
crow::SimpleApp app;
CROW_ROUTE(app, "/")([](){
return "Hello, World!";
});
app.port(18080).multithreaded().run();
}
Understanding C++ Web Frameworks
What is a C++ Web Framework?
A C++ web framework is a software framework specifically designed to assist in the development of web applications using the C++ programming language. These frameworks provide developers with tools for handling common tasks such as routing, session management, and database interaction, thereby allowing them to focus on creating unique application features instead of reinventing the wheel.
When comparing C++ web frameworks to frameworks in other programming languages (like Python or JavaScript), C++ frameworks often provide greater control over system resources and performance optimization. This control can be critical in scenarios where high efficiency and low-level system interaction are required.
Why Use C++ for Web Development?
Choosing C++ for web development comes with significant performance advantages. The compiled nature of C++ means that applications can run significantly faster than interpreted ones. For industries that demand high-performance applications such as gaming, finance, or real-time data processing, C++ can be an appealing choice.
Furthermore, C++ gives developers fine-tuned control over memory management. This can lead to lower memory usage and less overhead, which is particularly beneficial in large-scale applications where resources are a premium.
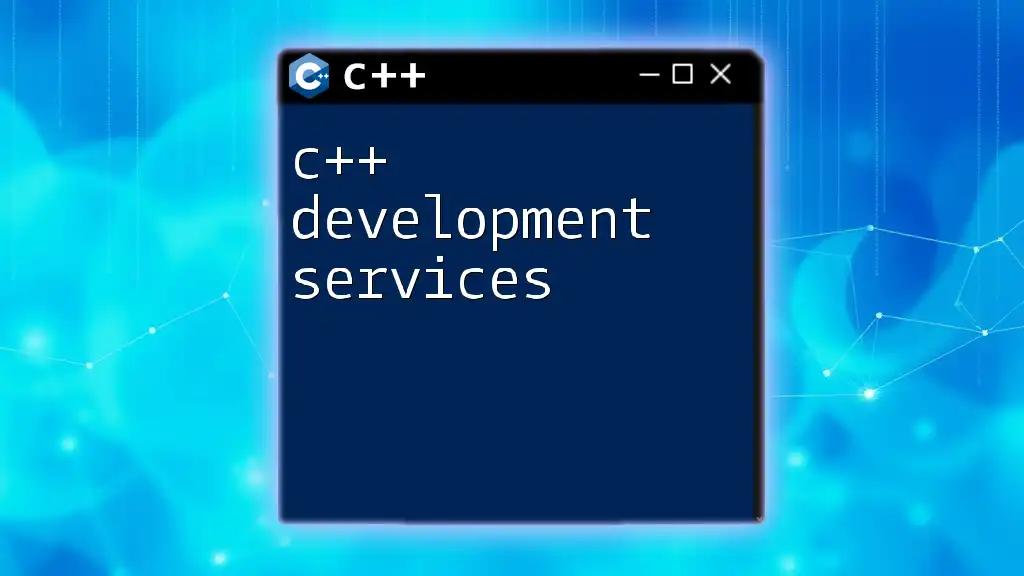
Popular C++ Web Frameworks
Overview of Key C++ Web Frameworks
Numerous C++ web frameworks have carved out a niche in the development landscape. Here are a few of the most notable ones:
CPPCMS CPPCMS is designed for building high-performance web applications. It's known for its ability to support web applications requiring high concurrency.
#include <cppcms/application.h>
#include <cppcms/service.h>
#include <cppcms/http_response.h>
#include <cppcms/json.h>
class HelloWorld : public cppcms::application {
public:
HelloWorld(cppcms::service &srv) : cppcms::application(srv) {}
virtual void main(std::string url) {
response().out() << "Hello World!";
}
};
int main(int argc, char* argv[]) {
cppcms::service srv(argc, argv);
srv.applications_pool().mount(cppcms::create_pool<HelloWorld>("hello"));
srv.run();
}
Wt (pronounced "witty")
Wt is another prominent C++ framework that allows developers to create complex web applications in a manner similar to desktop applications. Wt encourages UI-driven designs, making it an excellent choice for developers accustomed to traditional GUI applications.
#include <Wt/WApplication.h>
#include <Wt/WText.h>
class MyApp : public Wt::WApplication {
public:
MyApp(const Wt::WEnvironment& env) : Wt::WApplication(env) {
setTitle("My Wt Application");
root()->addWidget(std::make_unique<Wt::WText>("Hello Wt!"));
}
};
int main(int argc, char **argv) {
return Wt::WRun(argc, argv, &MyApp);
}
Crow
Crow is celebrated for its lightweight and easy-to-use nature. It focuses on modern C++ as well as taking advantage of C++11 features for better syntax and performance.
#include "crow_all.h"
int main() {
crow::SimpleApp app;
CROW_ROUTE(app, "/")([]{
return "Hello, Crow!";
});
app.port(18080).multithreaded().run();
}
Pistache
Pistache is a modern C++ framework aimed at helping developers build HTTP servers and REST APIs quickly. It’s designed to be straightforward while leveraging the full power of C++.
#include <pistache/endpoint.h>
using namespace Pistache;
class HelloServer : public Http::Endpoint {
public:
HelloServer(Address addr) : Http::Endpoint(addr) {}
void init(size_t threads = 1) {
Http::Endpoint::init();
// Other initializations
}
void start() {
Http::Endpoint::serve();
}
};
int main() {
Address addr(Ipv4::any(), Port(9080));
HelloServer server(addr);
server.init();
server.start();
}
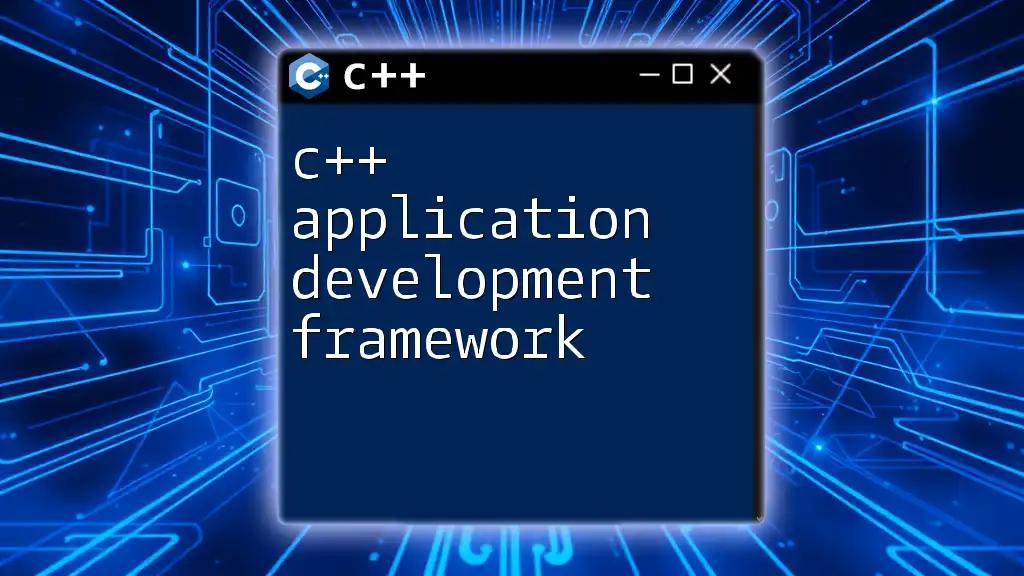
Setting Up a C++ Web Development Environment
Tools and Prerequisites
Before diving into C++ web development, ensure you have the necessary tools at your disposal. Popular software includes:
- IDE: Visual Studio, CLion, or Eclipse CDT.
- Compiler: GCC or Clang to compile your code.
- Libraries: CMake or Boost for handling dependencies and building your applications.
Installation Process
Installing a C++ web framework can vary, but most frameworks can be set up similarly. For instance, when installing CPPCMS:
- Install Dependencies: Ensure you have CMake and a compatible compiler.
- Download CPPCMS: Clone the repository from GitHub.
- CMake the Project: Navigate to the project folder:
mkdir build cd build cmake .. make
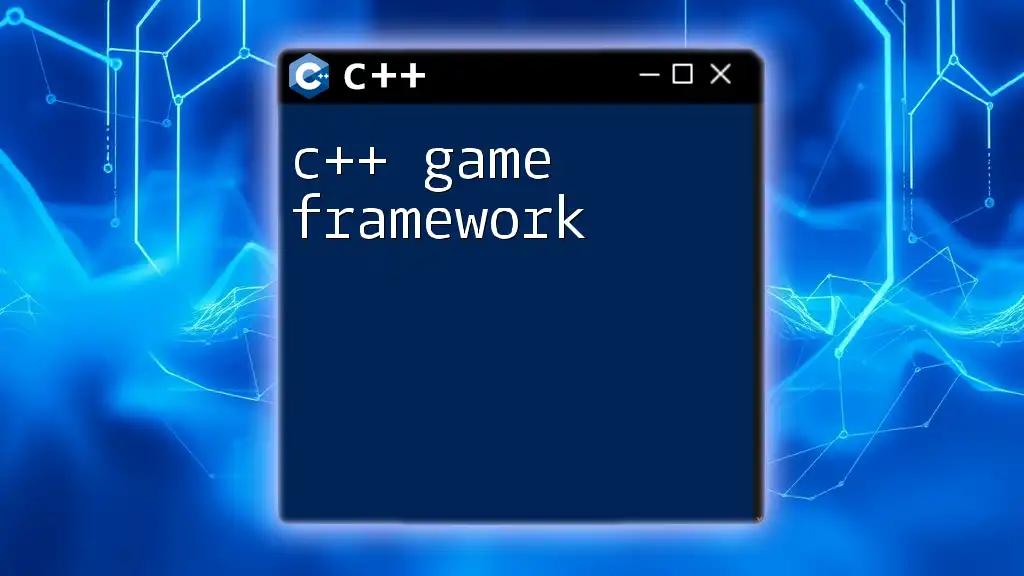
Building Your First C++ Web Application
Creating a Simple Web Application
Creating a basic web application using Crow can be accomplished through a few simple steps. Here's a basic example demonstrating how to set up a web server that responds with a welcome message.
#include "crow_all.h"
int main() {
crow::SimpleApp app;
CROW_ROUTE(app, "/")([] {
return "Welcome to my first C++ web application!";
});
app.port(18080).multithreaded().run();
}
Handling HTTP Requests
Understanding different HTTP methods is crucial in web applications. For example, handling GET and POST requests with Crow can look like this:
CROW_ROUTE(app, "/submit").methods("POST"_method)([](const crow::request& req) {
auto name = req.body; // Get POST body
return "Received: " + name;
});
This code snippet shows how to handle a webpage that processes user-submitted data through POST requests, underlining the flexibility of many C++ web frameworks.
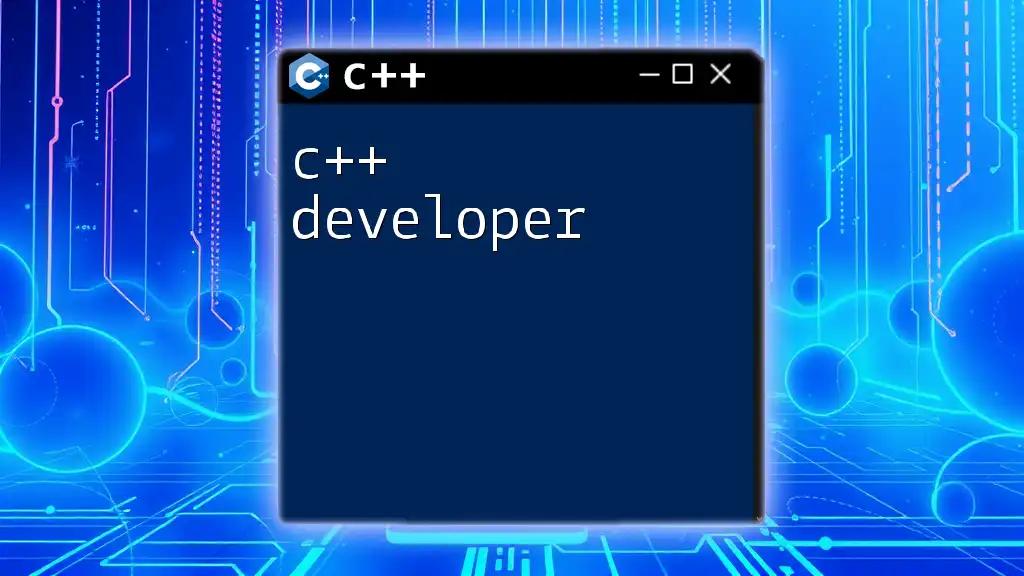
Connecting to a Database
Integrating Databases in C++ Web Applications
For many applications, working with a database is a necessity. C++ can efficiently interface with databases such as MySQL or PostgreSQL using libraries like MySQL Connector/C++.
Example: Connecting to MySQL
#include <mysql_driver.h>
#include <mysql_connection.h>
sql::mysql::MySQL_Driver* driver;
sql::Connection* con;
driver = sql::mysql::get_mysql_driver_instance();
con = driver->connect("tcp://127.0.0.1:3306", "user", "password");
This code initializes a connection to a MySQL database, establishing the groundwork for executing queries and retrieving data.
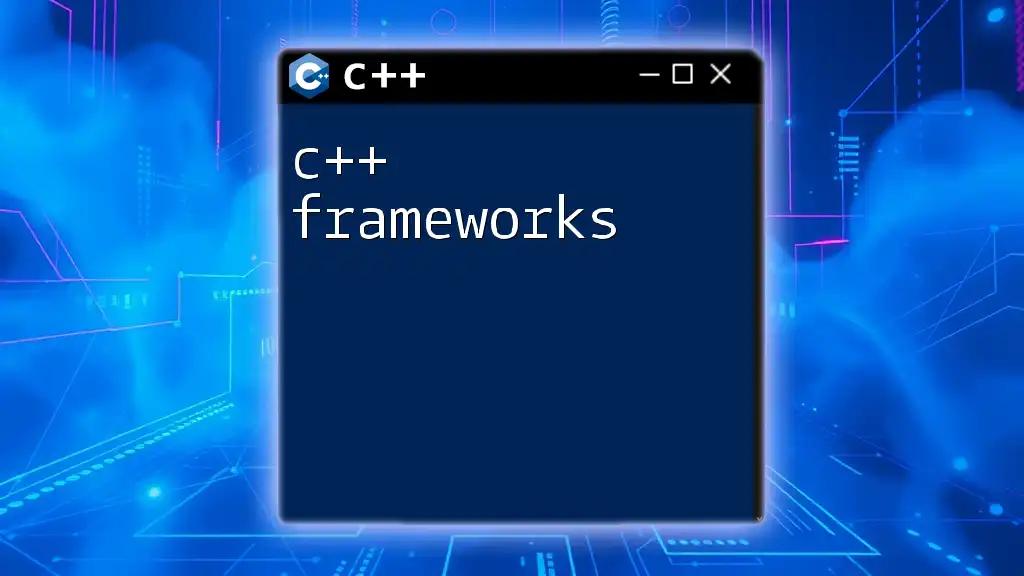
Advanced Topics in C++ Web Development
Asynchronous Programming
Asynchronous programming allows a web application to handle numerous requests concurrently, leading to improved performance. In C++, this can often be done using the standard library or frameworks that support async operations.
Security Best Practices
Web security is paramount. It's essential to protect your application from common vulnerabilities such as SQL Injection and Cross-Site Scripting (XSS). Always validate and sanitize user inputs, and use prepared statements when dealing with databases.
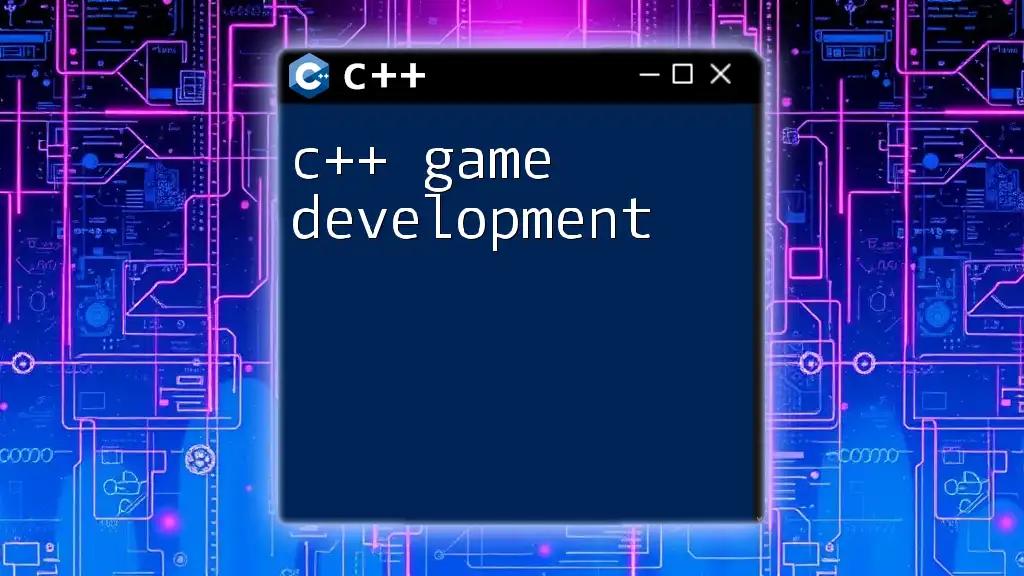
Testing and Debugging Your C++ Web Application
Tools for Testing C++ Web Applications
Testing frameworks like Google Test allow developers to write unit tests for their applications. This practice ensures that components function as expected and fosters robust development.
Debugging Techniques
Debugging can be approached using various methods. Using an interactive debugger like GDB allows developers to step through their code, inspect variable states, and trace errors:
gdb ./my_application
With GDB, you can set breakpoints and run the application up to a specific point, giving you the ability to diagnose issues in real-time.

Conclusion
As a powerful tool in the arsenal of web developers, the C++ web development framework offers unique advantages, particularly in high-performance scenarios. By delving into various frameworks and understanding their capabilities, you can effectively leverage C++ for rich and efficient web applications.
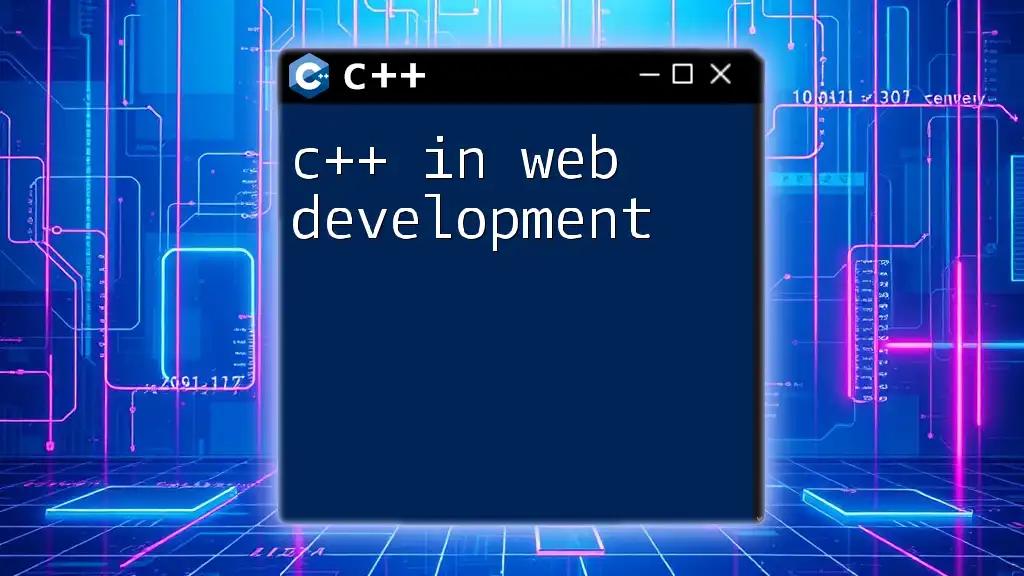
Additional Resources
To deepen your expertise in C++ web development frameworks, consider exploring books like "C++ Concurrency in Action" and tutorials from the community. Engaging with forums and online platforms can also provide invaluable support as you navigate this sophisticated domain.