C++ game development involves using the C++ programming language to create interactive games, leveraging its performance and control over system resources.
Here's a simple code snippet that demonstrates how to create a basic game loop in C++:
#include <iostream>
int main() {
bool isRunning = true;
while (isRunning) {
// Game logic goes here
std::cout << "Game is running!" << std::endl;
// For demonstration, we'll stop the loop after one iteration
isRunning = false;
}
return 0;
}
Understanding the Basics of C++
C++ is a powerful, high-performance programming language that has features such as object-oriented programming, data abstraction, and low-level memory manipulation, making it an excellent choice for game development. C++ is known for its speed and efficiency, which is why many successful game engines use it as their core language.
Setting Up Your Development Environment
To kickstart your journey into C++ game development, you should set up a suitable development environment. Popular Integrated Development Environments (IDEs) include Visual Studio, Code::Blocks, and CLion. Here’s how to set up your environment:
- Choose an IDE that suits your style. Visual Studio is widely used for Windows development, while Code::Blocks is lightweight and highly configurable.
- Install a C++ compiler. Get GCC (GNU Compiler Collection) for Linux, Clang for macOS, or Microsoft Visual C++ Compiler for Windows. Follow the installation prompts specific to your OS.
Fundamental Concepts in C++
Now that your environment is set, it's crucial to understand some basic C++ concepts that will be foundational in game development.
Data Types and Variables
C++ offers a variety of data types including `int`, `float`, `char`, and `bool`, to name a few. Understanding data types is pivotal, as they form the backbone of variable declaration:
int playerScore = 0;
float playerHealth = 100.0f;
char playerSymbol = 'A';
bool gameIsRunning = true;
Control Structures
Control structures allow you to dictate the flow of your game. Conditional statements and loops are essential tools at your disposal.
- Conditional statements: Use `if`, `else`, and `switch` to control which code runs based on certain conditions. For example:
if (playerHealth < 20) {
std::cout << "Warning: Low Health!" << std::endl;
}
- Loops: Efficiently handle repetitive tasks using `for`, `while`, and `do-while` loops. A simple `for` loop to move a player character could look like this:
for (int i = 0; i < 10; ++i) {
movePlayerForward();
}
Functions and Object-Oriented Programming
Functions are blocks of code designed to perform specific tasks. C++ empowers you to call these functions throughout your game, enhancing code reusability:
void movePlayer(int direction) {
// Implementation for moving player
}
Object-Oriented Programming (OOP) is another integral concept, allowing you to create classes and objects. For instance, creating a simple class for your game entities could be structured like this:
class Player {
public:
int health;
int score;
void takeDamage(int damage) {
health -= damage;
}
};
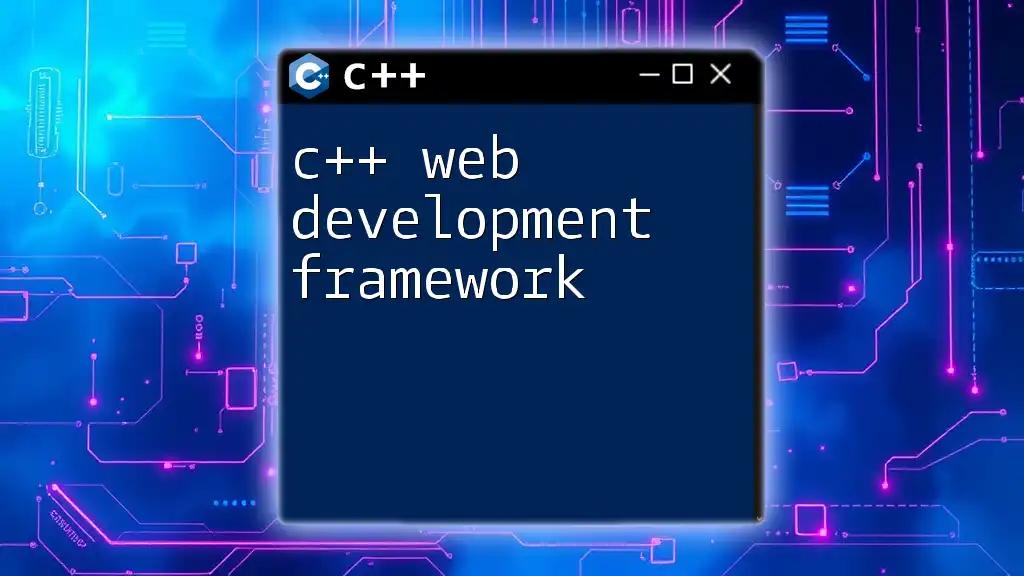
Game Development Frameworks and Libraries
Choosing the right framework or library forms the basis of your game. They provide tools to handle graphics, audio, and input.
Overview of Popular Frameworks
- SDL (Simple DirectMedia Layer): A cross-platform library to handle graphics and sound.
- SFML (Simple and Fast Multimedia Library): Great for 2D graphics and faster learning curve compared to others.
- Unreal Engine: A comprehensive game engine utilizing C++, suitable for both beginners and advanced developers.
Installing and Setting Up SDL
SDL is a great starting point for beginners. To set it up:
-
Step-by-step guide for installation: Download the SDL development libraries from the SDL website and follow the provided instructions for your OS.
-
Example: Creating a window with SDL: Here’s a simple code snippet to get started:
#include <SDL2/SDL.h>
int main(int argc, char* argv[]) {
SDL_Init(SDL_INIT_VIDEO);
SDL_Window* window = SDL_CreateWindow("Hello, SDL!",
SDL_WINDOWPOS_UNDEFINED, SDL_WINDOWPOS_UNDEFINED,
640, 480, 0);
SDL_Event event;
bool running = true;
while (running) {
while (SDL_PollEvent(&event)) {
if (event.type == SDL_QUIT) {
running = false;
}
}
}
SDL_DestroyWindow(window);
SDL_Quit();
return 0;
}
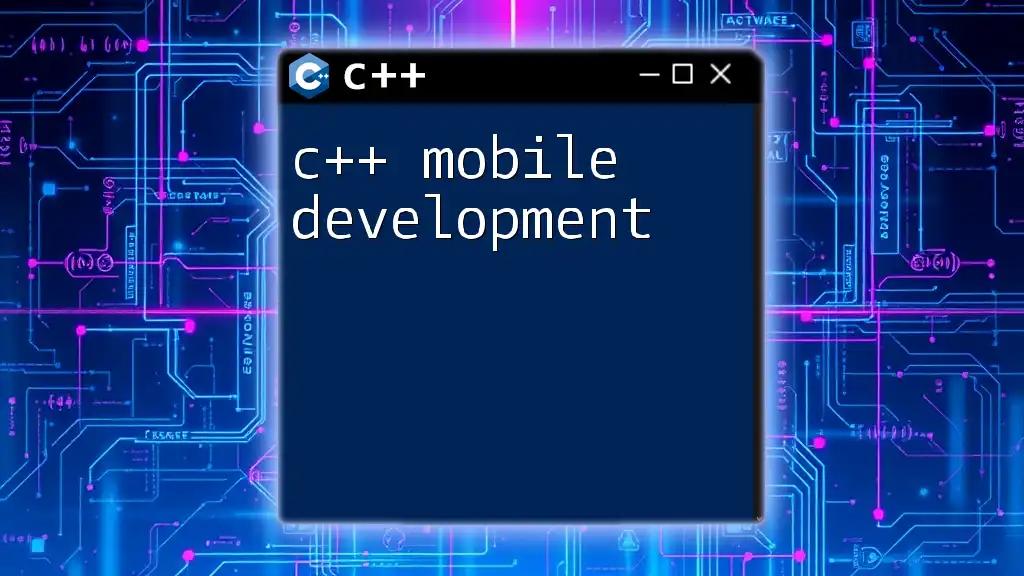
Core Concepts in Game Development
There are several vital concepts every game developer should understand, including the game loop, game states, and rendering techniques.
Game Loop
The game loop is the core of any game, enabling continuous updates and rendering. It generally includes three major steps: input handling, game logic updates, and rendering.
void gameLoop() {
while (running) {
handleInput();
updateGame();
renderGraphics();
}
}
A well-structured game loop ensures smooth gameplay and responsive controls.
Game States
Managing various game states is crucial, as games typically have multiple phases such as the start menu, gameplay, and game over screens. Implementing a state machine can help efficiently transition between these states, making your code organized and easier to maintain.
Graphics and Rendering Techniques
C++ can handle both 2D and 3D graphics, although your choice will depend on the game type you are developing. Basic rendering techniques, such as drawing shapes and textures, are essential building blocks in creating visual elements:
// Pseudo-code for rendering logic
void renderGraphics() {
SDL_RenderClear(renderer);
// Draw all game objects
SDL_RenderPresent(renderer);
}
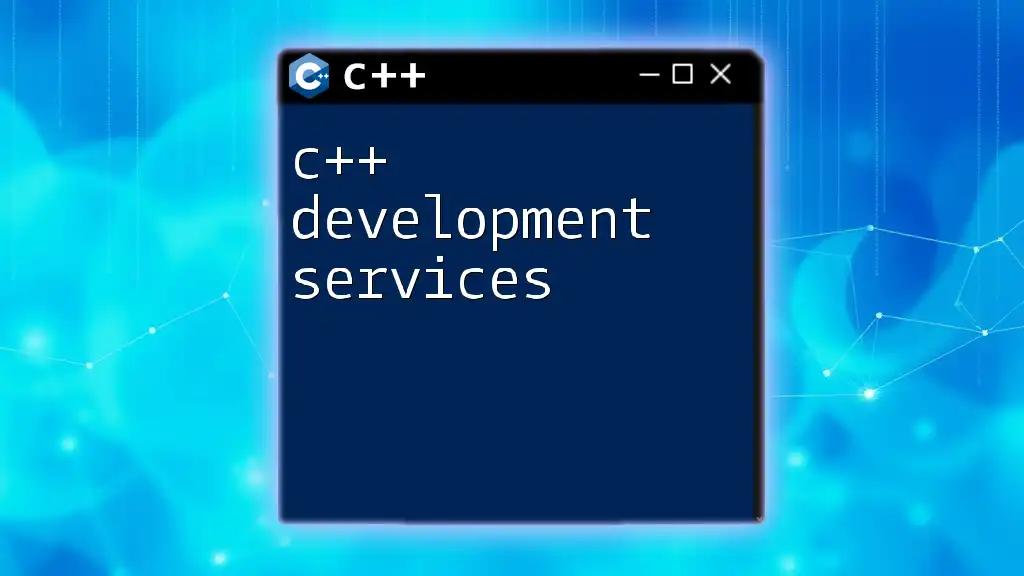
Game Design Principles
To create engaging gameplay, understanding game design principles is essential.
Understanding Game Mechanics
A game mechanic is a rule or system that defines player interactions within your game. Examples include health systems, scoring mechanics, and player movement. Balancing these mechanics is vital for a rewarding gaming experience.
Level Design Fundamentals
Effective level design involves planning and creating environments that challenge players. Tools such as Tiled or Unity can assist in designing complex level layouts but understanding the base mechanics in C++ will allow you to implement these levels more effectively in your game.
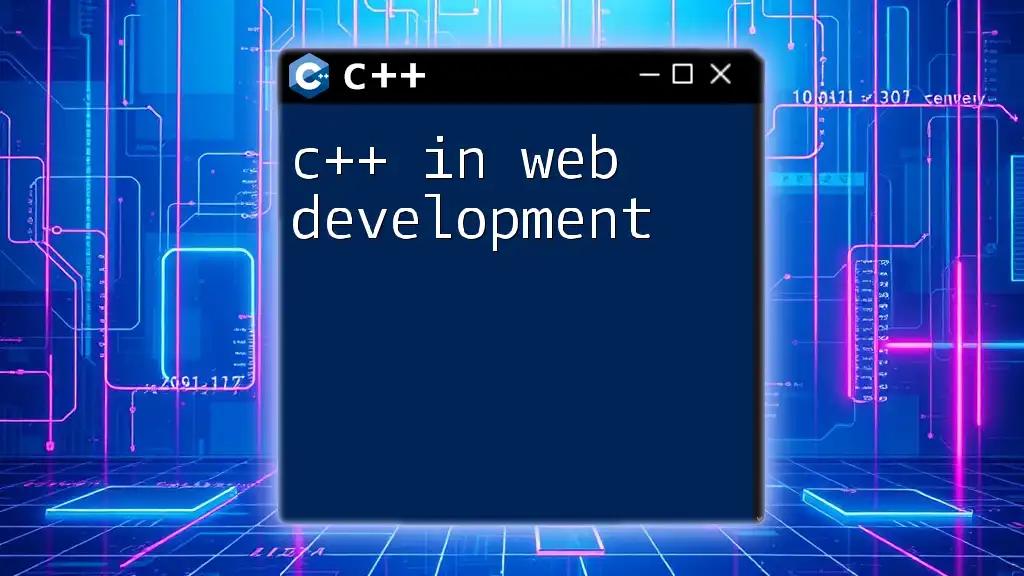
Animation and Audio
Incorporating animation and audio adds a dynamic element to your game.
Basics of Animation in C++
Animation can be achieved using sprites and keyframes. A simple way to implement animations is by cycling through frame images in a sprite sheet based on time.
Incorporating Audio
Adding sound effects and music enhances immersion in any game. Libraries such as SDL_mixer for SDL or OpenAL can be used for audio handling. For example, loading and playing a sound effect would look like:
Mix_Chunk *soundEffect = Mix_LoadWAV("sound.wav");
Mix_PlayChannel(-1, soundEffect, 0);
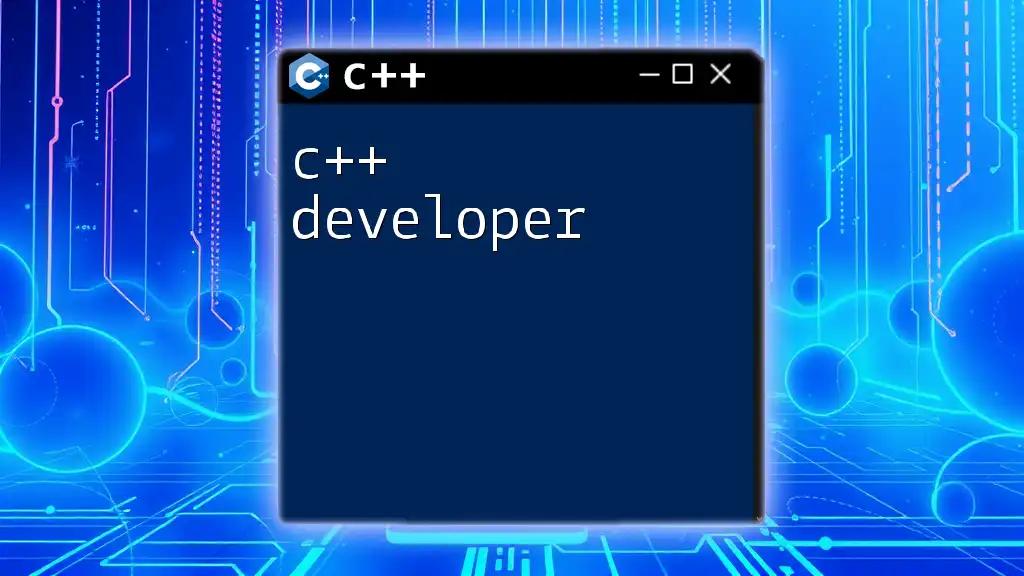
Debugging and Optimization
Debugging is an inevitable part of game development. Tools like gdb (GNU Debugger) or Valgrind can help you identify and resolve issues in your code effectively.
Importance of Debugging in Game Development
Identifying bugs early leads to a better codebase. Common debugging techniques include using print statements, breakpoints, and log files to track down issues.
Performance Optimization Techniques
Once your game mechanics are in place, optimization techniques will make sure your game runs smoothly. Profiling tools allow you to analyze where bottlenecks occur and advise on how to reduce CPU or GPU load.
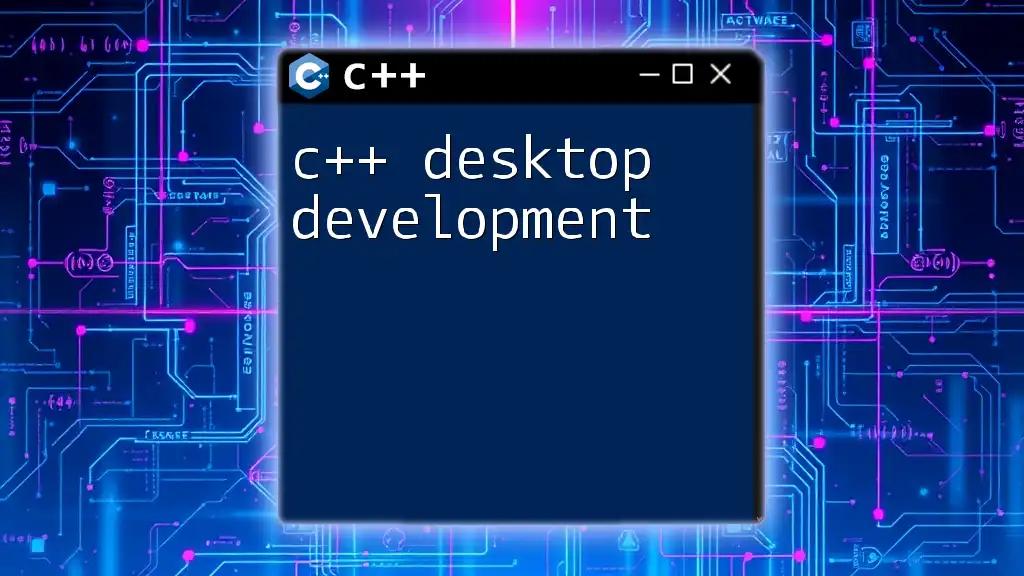
Conclusion
In this guide, we covered essential topics in C++ game development, from setting up your development environment, understanding fundamental concepts, exploring frameworks, and delving into animation and audio.
Resources for Further Learning
To continue your journey in C++ game development, consider diving deeper into authoritative books, engaging online courses, and participating in communities like StackOverflow or game dev forums.
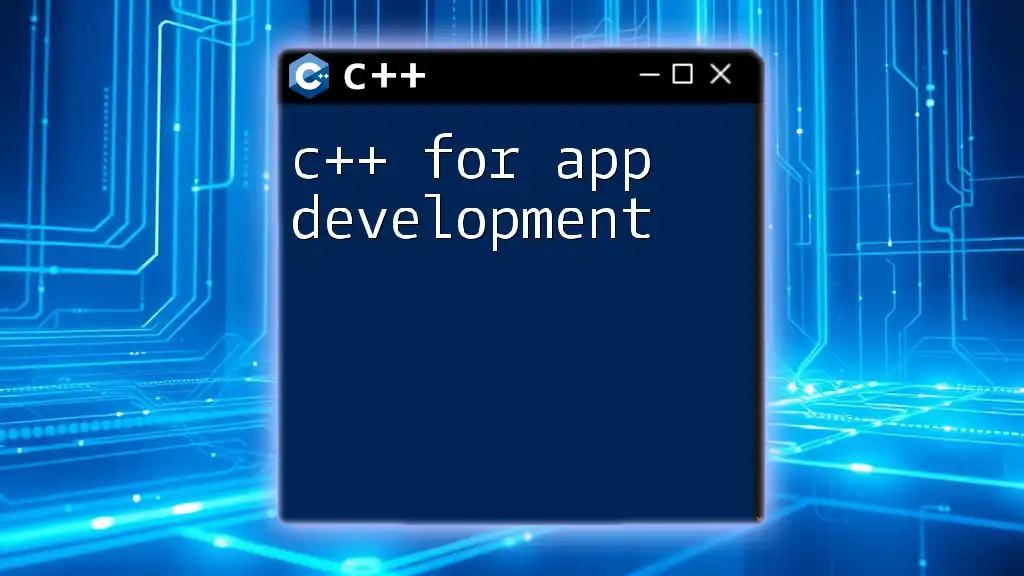
Call to Action
Share Your Experiences: We encourage you to share any projects you work on or challenges you face during your C++ game development journey.
Check Out Our Courses: Explore our comprehensive courses focused on mastering C++ commands for game development to further accelerate your learning path.