An Integrated Development Environment (IDE) for C++ provides a comprehensive platform that combines code editing, compiling, and debugging tools to streamline the development process, making it easier for programmers to write efficient C++ code.
Here's a simple example of a C++ program in markdown format:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is an Integrated Development Environment (IDE)?
An Integrated Development Environment (IDE) is a software application that provides comprehensive facilities to computer programmers for software development. IDEs typically consist of a source code editor, a compiler, a debugger, and often build automation tools, all integrated into one cohesive environment. The convenience of having all these tools in one application greatly enhances productivity and efficiency during C++ programming.
Components of an IDE
Source Code Editor: This is where developers write their code. A good source code editor supports syntax highlighting, auto-indentation, and code folding, which helps improve readability and organization.
Compiler: The role of a compiler in C++ programming is to translate the source code written by the developer into executable machine code. This is essential for running the programs created.
Debugger: Debugging tools allow the developer to monitor the code execution, inspect variables, set breakpoints, and diagnose issues effectively. This capability is critical in identifying and resolving bugs.
Build Automation Tools: These tools help automatically compile and link code, simplifying the development process. They can manage dependencies and streamline repetitive tasks.
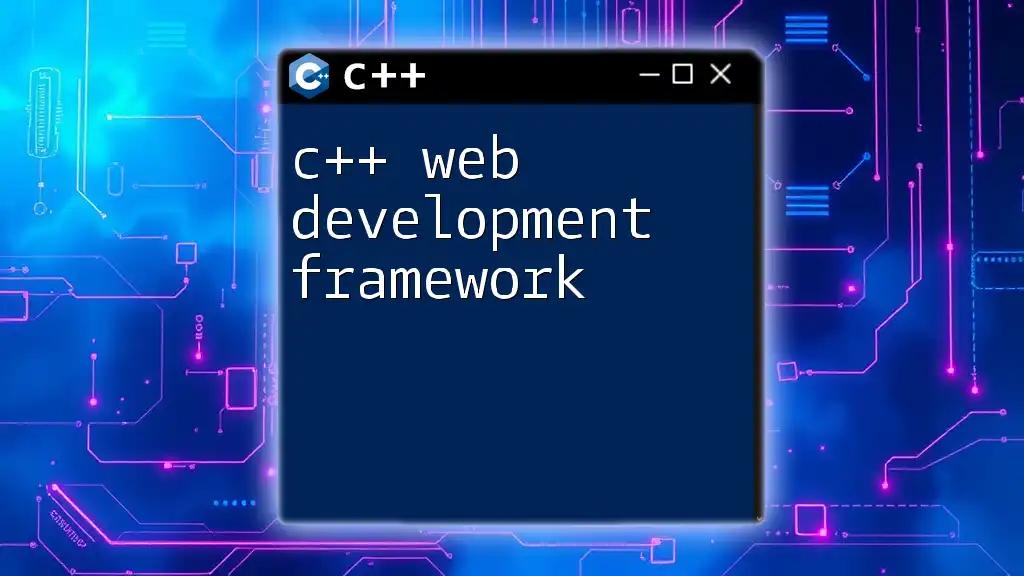
Popular IDEs for C++
Microsoft Visual Studio
Overview and Features
Microsoft Visual Studio is widely recognized for its powerful features supporting C++ development. It provides an intuitive user interface, integrated debugging, and extensive libraries.
Code Snippet Example
#include <iostream>
int main() {
std::cout << "Hello, World!";
return 0;
}
With Visual Studio, running this simple program is just a few clicks away, making it accessible for beginners.
Why Choose Visual Studio?
Visual Studio is particularly beneficial for developers aiming to build Windows applications due to its robust support for Windows APIs and detailed documentation.
Code::Blocks
Overview and Features
Code::Blocks is an open-source, cross-platform IDE designed specifically for C++ programming. It’s lightweight and boasts high configurability with various plugins.
Code Snippet Example
#include <iostream>
void greet() {
std::cout << "Welcome to Code::Blocks!" << std::endl;
}
Users can easily compile and run this greeting function within the IDE.
Benefits of Using Code::Blocks
It provides a simple setup and is less resource-intensive compared to other IDEs, making it an excellent choice for those starting with C++ or those who prefer a more straightforward interface.
Eclipse CDT (C/C++ Development Tooling)
Overview and Features
Eclipse CDT is part of the larger Eclipse IDE, known for its flexibility through the incorporation of various plugins. The CDT component specifically supports C/C++ development with features like a powerful editor and project management tools.
Code Snippet Example
#include <iostream>
class Sample {
public:
void display() {
std::cout << "Using Eclipse CDT!" << std::endl;
}
};
With this example, developers can easily implement and test class functionality within Eclipse.
Why Use Eclipse CDT?
Eclipse offers a robust community and extensive resources for troubleshooting and improvement, making it suitable for both novice and experienced developers.
CLion
Overview and Features
Developed by JetBrains, CLion is a modern C++ IDE known for its smart code assistance, refactoring capabilities, and integrated terminal. It provides a sophisticated development experience for professionals.
Code Snippet Example
#include <iostream>
int main() {
std::cout << "Hello from CLion!" << std::endl;
}
Executing this snippet in CLion demonstrates its intelligent features, such as live code analysis and performance suggestions.
Pros and Cons of CLion
While CLion offers state-of-the-art features and a user-friendly experience, its subscription-based pricing model may deter some potential users, particularly those just beginning their C++ journey.
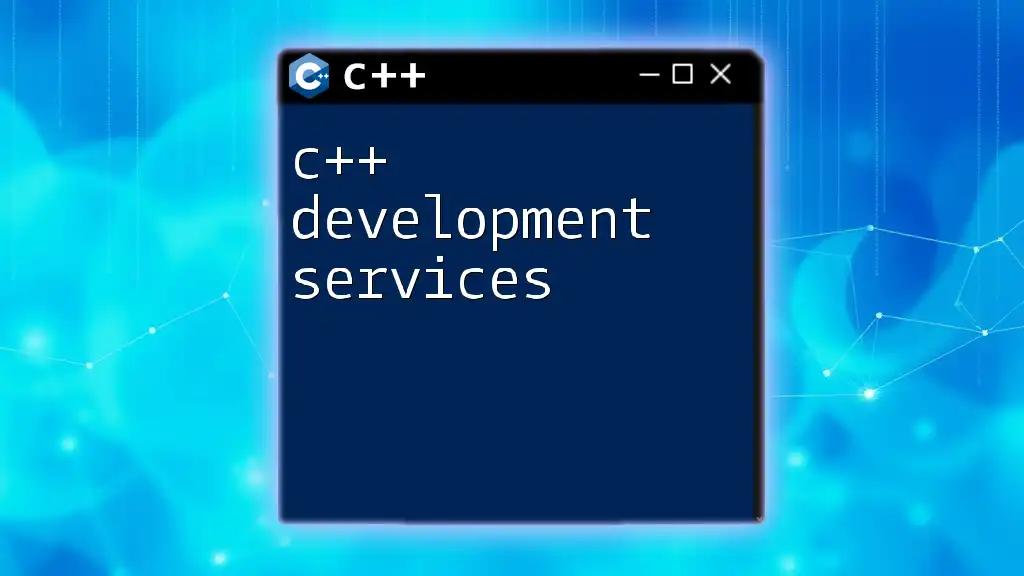
Key Features to Look for in a C++ IDE
When searching for the best integrated development environment for C++, consider the following features:
User-Friendly Interface: A well-designed interface is crucial for efficient coding. IDEs should offer intuitive navigation to benefit both novices and seasoned developers.
Code Completion and IntelliSense: Features like auto-completion save time and reduce errors by suggesting relevant code snippets, function names, and properties as the developer types.
Debugging Tools: Comprehensive debugging support—like real-time error highlighting, breakpoints, and watch variables—are essential for efficiently locating and fixing issues.
Project Management: Good IDEs streamline project management, allowing the organization of files and dependencies with ease, enabling users to focus more on coding.
Version Control Integration: Built-in support for version control systems like Git helps developers maintain code reliability and manage changes effortlessly.
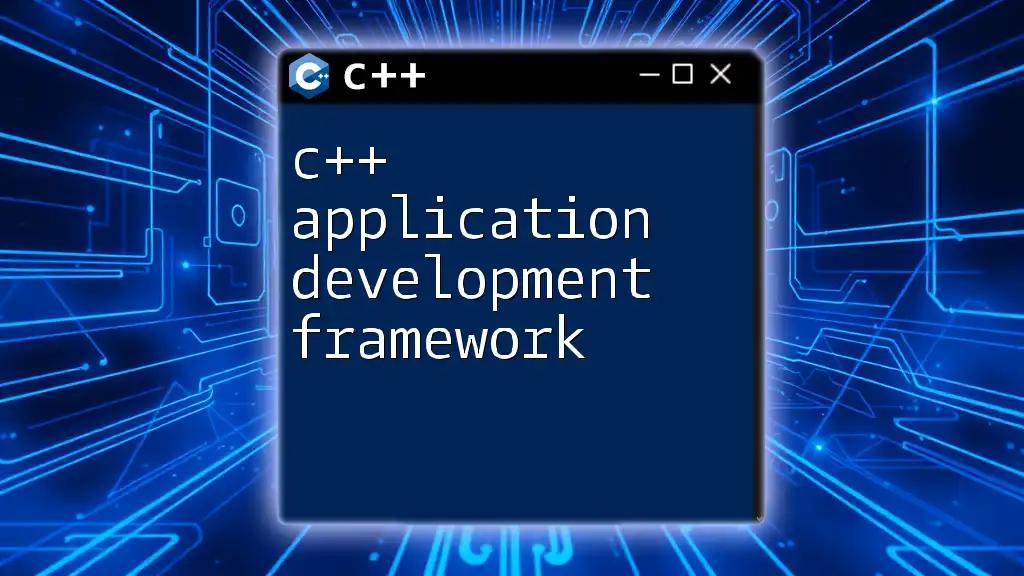
How to Set Up Your IDE for C++ Development
Installation Steps
Each IDE typically has its installation process. For the most part, it includes downloading the installer from the official website, running it, and following the prompts to set up required components.
Configuring Your Environment
Upon installation, configuring your environment involves setting up relevant paths for the compiler and libraries. This can usually be achieved in the IDE's settings or preferences menu.
Writing Your First C++ Program
To write your first C++ program, create a new project in your chosen IDE, create a new source file, and input your code. For example:
#include <iostream>
int main() {
std::cout << "Hello, C++ Developer!" << std::endl;
return 0;
}
After writing your program, use the build option to compile and run it. The output should display in the console.

Conclusion
The choice of an integrated development environment for C++ can significantly influence your programming experience. Whether you're using Visual Studio for its comprehensive tools, Code::Blocks for its simplicity, Eclipse CDT's flexibility, or CLion's advanced features, an appropriate IDE will streamline your work and improve your productivity as a C++ developer.
Not only does each IDE offer a unique set of strengths, but exploring multiple environments can enhance your knowledge and proficiency in C++. You’re encouraged to experiment with the options available to discover which IDE aligns best with your coding style and project requirements.
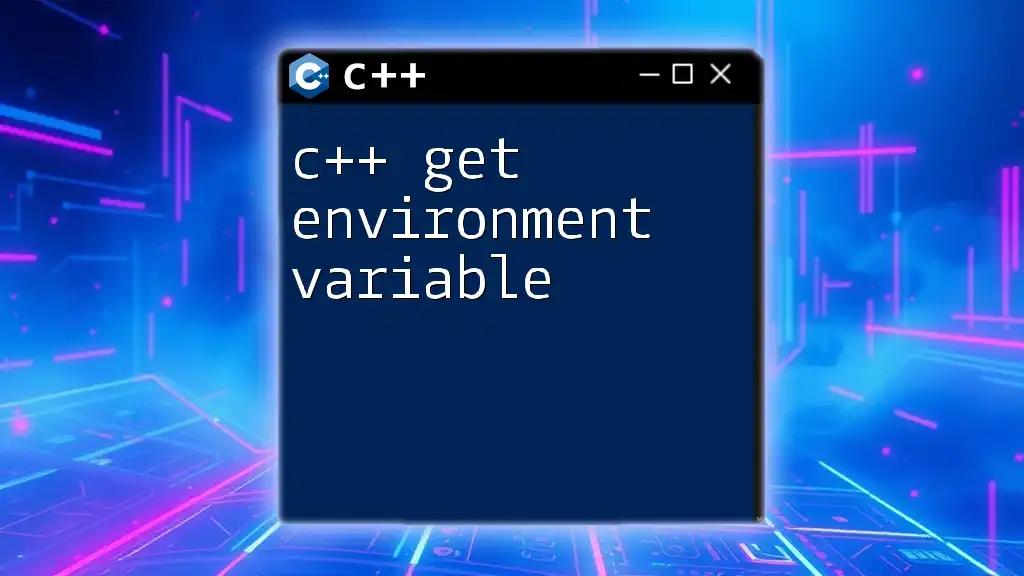
Further Resources
To deepen your understanding, engage with the following resources:
- Documentation for each IDE, including setup guides and feature explanations.
- Online Communities such as forums or social media groups where developers share tips, discuss challenges, and provide peer support.

Call to Action
Stay engaged and elevate your C++ skills further by subscribing for tips and insights into effectively utilizing integrated development environments and mastering C++.