In C++, using integer division with negative numbers can yield unexpected results due to flooring behavior; for instance, `-5 / 2` results in `-3` instead of `-2`.
Here's an example code snippet:
#include <iostream>
int main() {
int a = -5;
int b = 2;
int result = a / b; // Result will be -3
std::cout << "Integer division of " << a << " by " << b << " is: " << result << std::endl;
return 0;
}
Understanding Integer Division
What is Integer Division?
Integer division refers to the division of two integers where the result is also an integer, discarding any fractional part of the answer. For instance, when you divide 5 by 2 in integer division, the result is 2, not 2.5. This is a fundamental behavior in many programming languages, including C++.
The distinction between integer and floating-point division is crucial as it affects how results are interpreted and stored. When you perform floating-point division, C++ retains the decimal portion of the result. Understanding this difference allows programmers to make informed decisions depending on the context of their calculations.
Why Integer Division Matters in C++
Integer division is significant in C++ because it can lead to unexpected results if one does not fully grasp how C++ treats negative numbers. Developers frequently encounter scenarios where they need to perform division, especially in algorithms, games, and financial calculations, where the outcome can determine the logic flow or the correctness of outputs.

C++ Division Operators
Overview of Division Operators
In C++, division is performed using two main operators:
- The division operator (`/`) is used for division.
- The modulus operator (`%`) is used to find the remainder of an integer division.
These operators work quite differently when dealing with integers compared to floating-point numbers. For instance, if you perform:
#include <iostream>
int main() {
int a = 7;
int b = 2;
std::cout << "Integer division: " << a / b << std::endl; // Outputs: 3
std::cout << "Modulus: " << a % b << std::endl; // Outputs: 1
return 0;
}
In this example, `7 / 2` results in `3`, and `7 % 2` results in `1`, clearly illustrating integer division and modulus behavior.

Behavior of Integer Division with Negative Numbers
Rules of Integer Division
When it comes to negative numbers, integer division in C++ adheres to specific rules that may differ from what one might traditionally expect. In particular, C++ truncates toward zero when dividing integers. This means that regardless of the signs of the operands, the output will always round toward zero.
Example 1: Positive Divisor, Negative Dividend
#include <iostream>
int main() {
int dividend = -5;
int divisor = 2;
int result = dividend / divisor;
std::cout << "Result of " << dividend << " / " << divisor << " is " << result << std::endl; // Outputs: -2
return 0;
}
In this example, we see `-5 / 2` yields `-2`. Here, the result is rounded towards zero, demonstrating C++'s truncation behavior, which can lead to unexpected outcomes if you previously assumed floor division.
Example 2: Negative Divisor, Positive Dividend
#include <iostream>
int main() {
int dividend = 5;
int divisor = -2;
int result = dividend / divisor;
std::cout << "Result of " << dividend << " / " << divisor << " is " << result << std::endl; // Outputs: -2
return 0;
}
Again, in this case, the output is also `-2` for `5 / -2`. Regardless of whether the dividend is positive or negative, the resulting truncation continues to produce results that may not align with expectations based solely on arithmetic rules.
Example 3: Both Dividend and Divisor Negative
#include <iostream>
int main() {
int dividend = -5;
int divisor = -2;
int result = dividend / divisor;
std::cout << "Result of " << dividend << " / " << divisor << " is " << result << std::endl; // Outputs: 2
return 0;
}
This scenario is unique since both values are negative. The result turns out to be `2` because, again, the integer division strictly adheres to truncation rules. Hence, understanding these subtleties is vital for accurate calculations in C++.

Edge Cases and Common Pitfalls
Division by Zero
One of the most critical errors to avoid in integer division is dividing by zero. In C++, attempting to divide by zero causes undefined behavior, which can lead to crashes or other unpredictable results. To manage this potential pitfall, it's advisable to implement checks on the divisor before performing division.
#include <iostream>
int main() {
int dividend = 5;
int divisor = 0; // Simulating division by zero
if (divisor == 0) {
std::cerr << "Error: Division by zero!" << std::endl;
} else {
int result = dividend / divisor;
std::cout << "Result of " << dividend << " / " << divisor << " is " << result << std::endl;
}
return 0;
}
This code snippet effectively prevents division by zero by checking the divisor, ultimately improving the robustness of the application.
Consistency Across Different Compilers
Integer division behavior can vary depending on the compiler. Thus, a vital part of software development involves testing code on multiple compilers and platforms. When portability is a concern, employing consistent practices is essential for ensuring expected results.

Best Practices for Handling Integer Division
Using Wrappers or Helper Functions
Creating wrapper functions can be an effective method to handle integer division safely. This approach centralizes your division logic, allowing you to abstract error handling and keep your core application logic clean.
#include <iostream>
int safeDivide(int dividend, int divisor) {
if (divisor == 0) {
std::cerr << "Error: Division by zero!" << std::endl;
return 0; // or throw exception
}
return dividend / divisor;
}
This function checks for division by zero before proceeding. By utilizing such helper functions, you can reduce repetitive error checks throughout your code.
Using Floating Point for Precision
In some cases, using floating-point numbers is advantageous for achieving precision, especially when dealing with potentially fractional results. It’s a straightforward way to avoid the pitfalls of integer division.
#include <iostream>
int main() {
int dividend = -5;
int divisor = 2;
double result = static_cast<double>(dividend) / divisor;
std::cout << "Result of " << dividend << " / " << divisor << " is " << result << std::endl; // Outputs: -2.5
return 0;
}
In this example, casting the integer to a double provides a more accurate representation of the division, resulting in `-2.5`, thereby preserving the decimal portion.
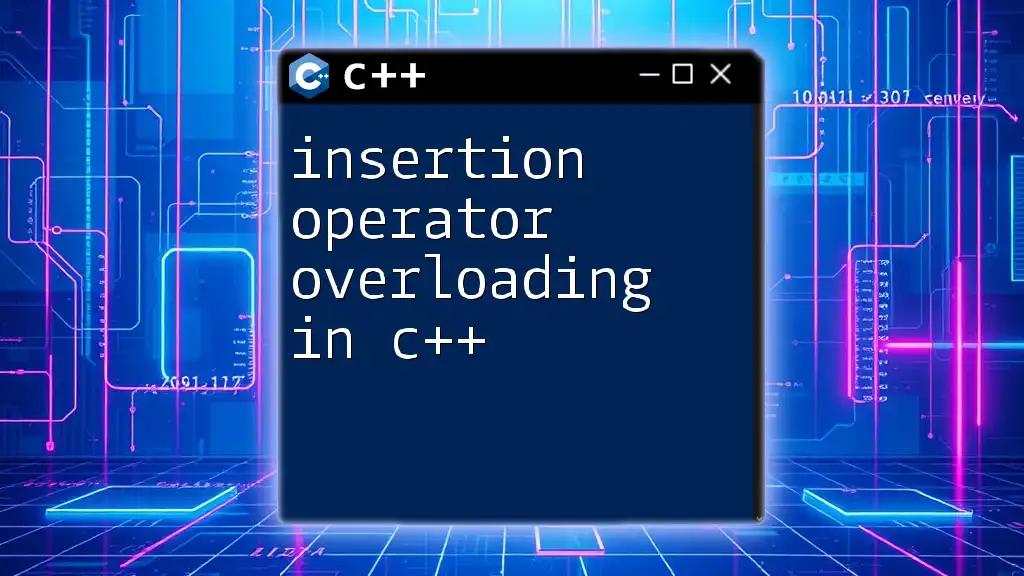
Conclusion
Understanding how C++ handles integer division with negative numbers is paramount for developing robust applications. The rule of truncating towards zero, particularly with negative operands, can lead to unexpected behavior if not properly understood. By practicing with different scenarios and implementing best practices—such as using helper functions and considering floating-point arithmetic—developers can write more reliable code and mitigate common pitfalls associated with integer division.
Now that you have a deeper understanding of this topic, experiment with the examples, and apply the insights to your C++ programming endeavors!