To reverse a number in C++, you can repeatedly extract the last digit and build the reversed number by multiplying the existing reversed number by 10 and adding the extracted digit.
Here's a code snippet to demonstrate this:
#include <iostream>
using namespace std;
int main() {
int num, reversed = 0;
cout << "Enter an integer: ";
cin >> num;
while (num != 0) {
reversed = reversed * 10 + num % 10;
num /= 10;
}
cout << "Reversed Number: " << reversed << endl;
return 0;
}
Understanding the Concept of Number Reversal
What is Number Reversal?
Number reversal is the process of transforming a given integer into its reverse form. For example, the number `12345` becomes `54321`. This operation is not just an academic exercise; it's foundational in programming. Understanding how to manipulate numbers effectively is crucial for algorithm development, data processing, and even user input validation.
Real-Life Examples
Number reversal has various practical applications. It can be used in:
- Palindrome checks: Verifying if a number reads the same forwards and backwards, like `121` or `12321`.
- Data formatting: Adjusting numerical values to fit specific output formats or criteria within applications.
- Games and puzzles: Implementing logic for challenges that require number manipulation.
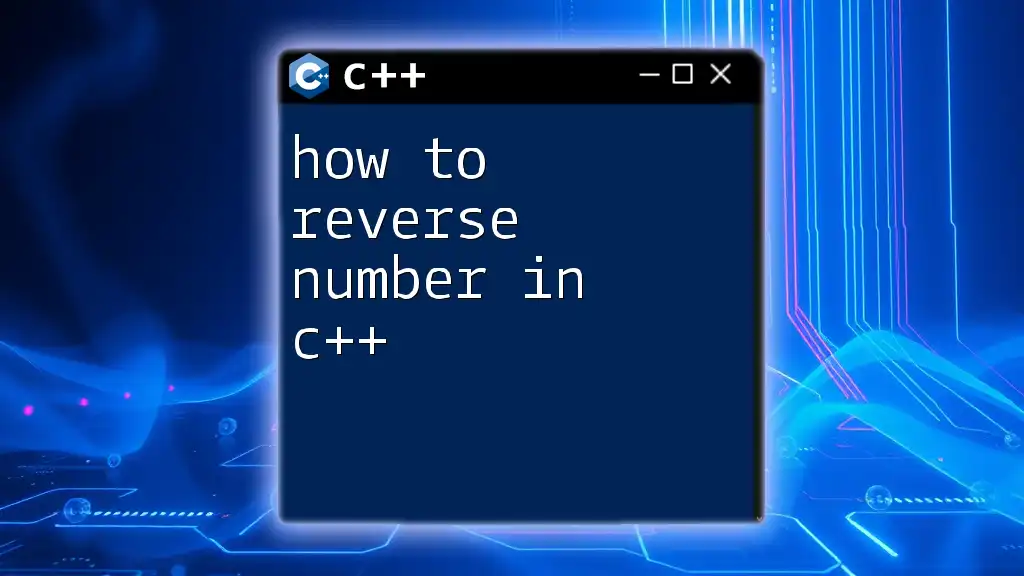
Basic Methods to Reverse Numbers
Using Arithmetic Operations
Explanation of the Approach
One of the simplest and most effective ways to reverse a number is by using basic arithmetic operations. The logic is based on the idea of repeatedly extracting the last digit of the number and building the reversed number by appending it.
Code Example
#include <iostream>
using namespace std;
int reverseNumber(int num) {
int reversed = 0;
while (num > 0) {
reversed = reversed * 10 + num % 10;
num /= 10;
}
return reversed;
}
int main() {
int number = 12345;
cout << "Reversed Number: " << reverseNumber(number) << endl;
return 0;
}
Code Explanation
- The `reverseNumber` function initializes a variable `reversed` to `0`.
- A `while` loop continues to execute as long as `num` is greater than `0`.
- Within the loop, we use `num % 10` to get the last digit and update `reversed` by multiplying it by `10` and adding this last digit.
- The number is then updated using integer division by `10`, effectively removing the last digit.
- This continues until all digits are processed, resulting in the reversed number.
Using String Manipulation
Explanation of the Approach
An alternative method to reverse a number is by treating it as a string. This can be particularly useful when your operations don't depend strictly on numerical properties but instead on the manipulation of the character sequence.
Code Example
#include <iostream>
#include <string>
using namespace std;
string reverseNumberAsString(int num) {
string strNum = to_string(num);
reverse(strNum.begin(), strNum.end());
return strNum;
}
int main() {
int number = 12345;
cout << "Reversed Number: " << reverseNumberAsString(number) << endl;
return 0;
}
Code Explanation
- In `reverseNumberAsString`, we first convert the integer to a string using `to_string`.
- The `reverse` function from the `<algorithm>` library is then employed to reverse the string.
- The result is returned as a string, allowing for easy manipulation or display.
- This method highlights a key advantage of C++: versatility in data types and operations.
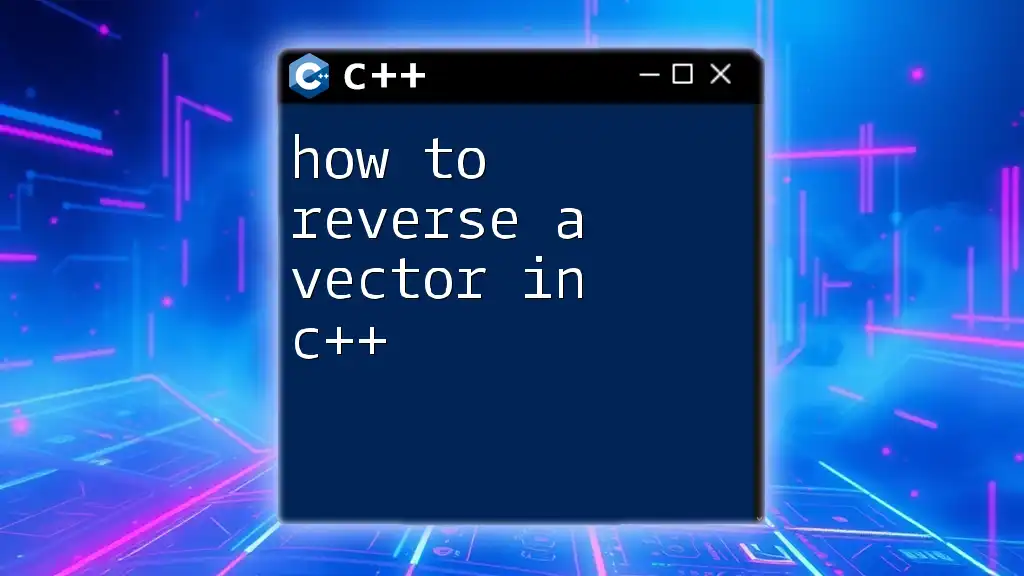
Advanced Methods for Reversal
Recursion Method
Explanation of Recursion
Recursion is a powerful programming technique where a function calls itself to solve a problem. In the case of reversing a number, recursion can provide a more elegant solution, breaking the problem down into simpler subproblems.
Code Example
#include <iostream>
using namespace std;
void reverseRec(int num, int &reversed) {
if (num == 0) return;
reversed = reversed * 10 + num % 10;
reverseRec(num / 10, reversed);
}
int main() {
int number = 12345;
int reversed = 0;
reverseRec(number, reversed);
cout << "Reversed Number: " << reversed << endl;
return 0;
}
Code Explanation
- The `reverseRec` function takes two arguments: the number to reverse and a reference to `reversed` that collects the digits.
- If the number is `0`, we hit our base case and return, ending the recursion.
- The last digit is processed similarly, and the function recursively calls itself with the number reduced by one digit.
- This leads to building the reversed number as each function call completes, demonstrating recursion’s power in simplifying solutions.
Using STL (Standard Template Library)
Explanation of Using STL Functions
C++'s Standard Template Library (STL) offers a wealth of functions and classes designed to streamline various programming tasks. For number reversal, STL allows for efficient manipulation of data structures.
Code Example
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
int reverseUsingSTL(int num) {
vector<int> digits;
while (num > 0) {
digits.push_back(num % 10);
num /= 10;
}
int reversed = 0;
for (int digit : digits) {
reversed = reversed * 10 + digit;
}
return reversed;
}
int main() {
int number = 12345;
cout << "Reversed Number: " << reverseUsingSTL(number) << endl;
return 0;
}
Code Explanation
- The function `reverseUsingSTL` employs a `vector` to store each digit of the number.
- By repeatedly extracting digits, we populate the `digits` vector.
- Once all digits are collected, a simple loop constructs the reversed number.
- This method leverages STL to manage dynamic arrays, enhancing code clarity and maintainability.
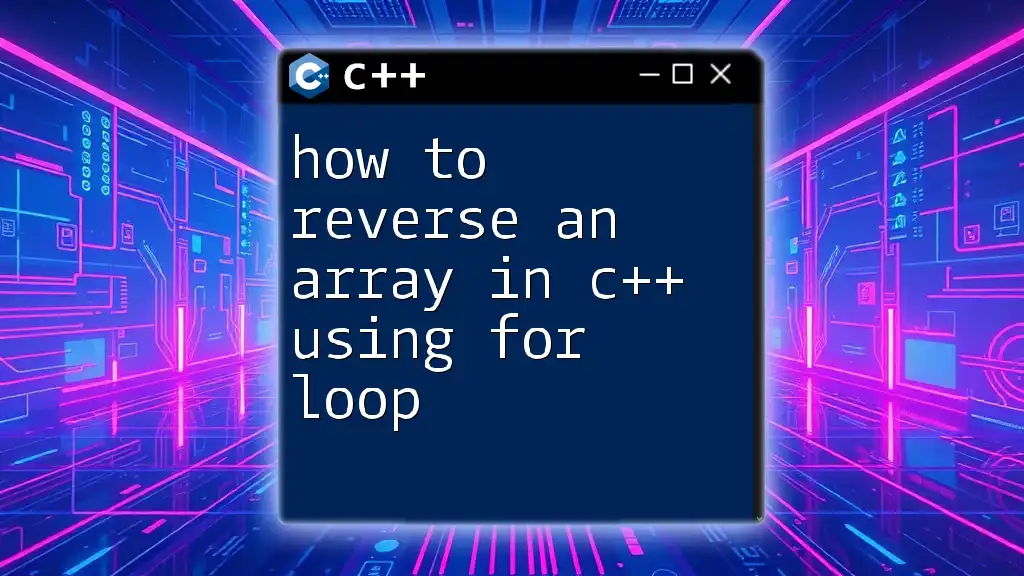
Common Errors and Troubleshooting
Logical Errors
When implementing number reversal, you may encounter logical errors such as:
- Off-by-one errors: Ensure that indexing and boundary conditions are correctly handled, particularly in recursive and loop-based methods.
- Mismanagement of the sign: Negative numbers need special handling to maintain their sign post-reversal.
Performance Issues
Each method has its complexities:
- The arithmetic method runs in O(n) time complexity, where n is the number of digits.
- The string manipulation approach may have additional overhead due to string operations and conversions.
To optimize performance, consider using arithmetic operations wherever possible, as they tend to be more efficient.
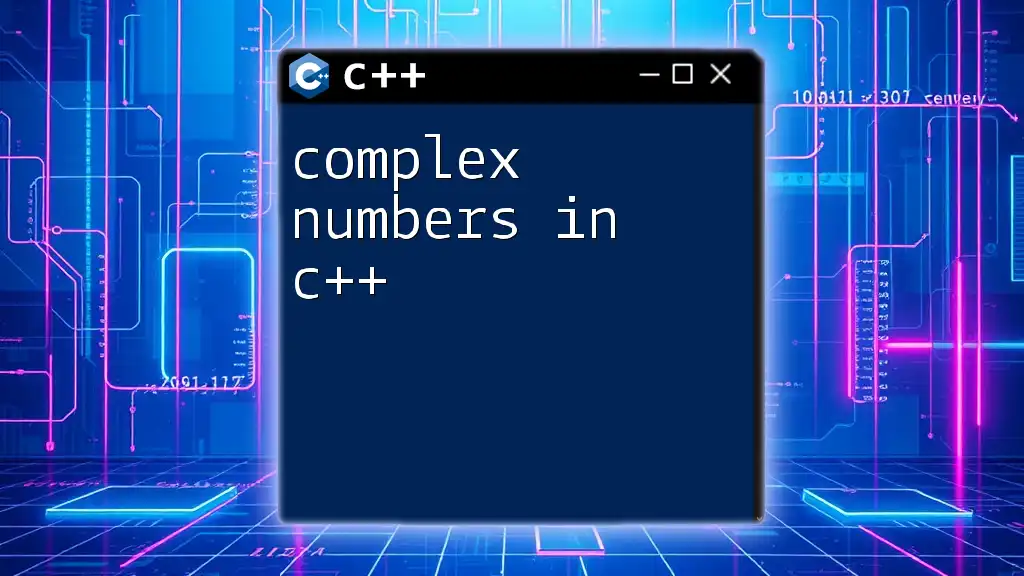
Conclusion
Reversing numbers in C++ can be achieved through various methods, each with its own merits and use cases. Whether you choose arithmetic manipulation, string handling, recursion, or STL, understanding the underlying principles shapes your programming skills. Experimenting with these methods not only enhances your coding arsenal but also deepens your appreciation for the versatility of C++. Mastering number manipulation is vital for any aspiring programmer and opens doors to more complex algorithmic challenges.
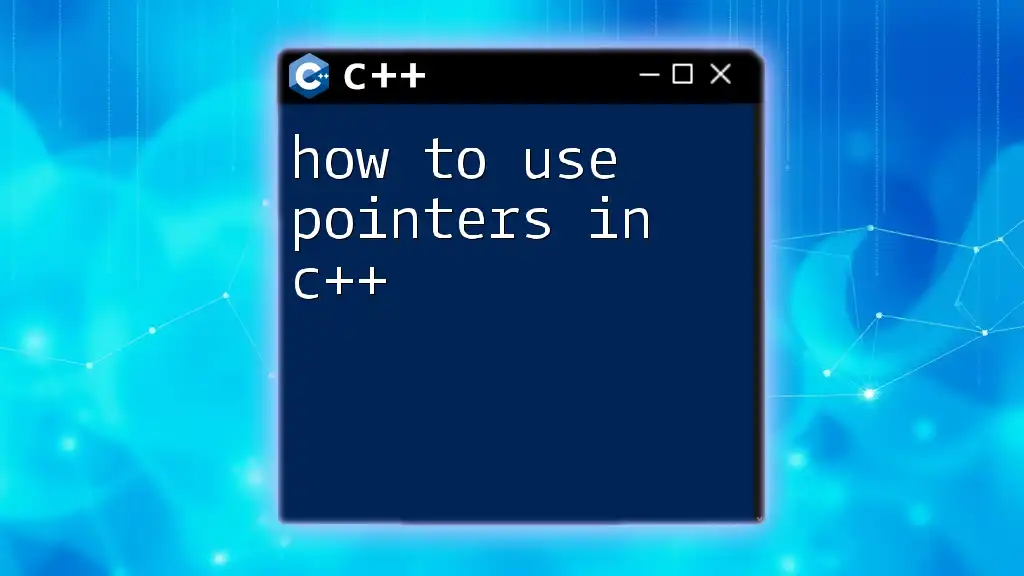
Additional Resources
For continued learning, consider exploring:
- Recommended books on C++ and algorithm design.
- Online courses focused on C++ programming and problem-solving techniques.
- Websites and forums devoted to C++ coding challenges and discussions.
By diving deeper into these resources, you will further solidify your understanding of how to reverse numbers in C++ and broaden your programming capabilities.