To create a simple game in C++, you can utilize basic input/output, control structures, and a game loop to manage the game's flow while rendering a simple output to the console.
Here's a basic code snippet for a simple number guessing game:
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
srand(static_cast<unsigned int>(time(0))); // Seed random number generator
int number = rand() % 100 + 1; // Generate random number between 1 and 100
int guess;
std::cout << "Guess the number (between 1 and 100): ";
do {
std::cin >> guess;
if (guess > number) {
std::cout << "Too high! Try again: ";
} else if (guess < number) {
std::cout << "Too low! Try again: ";
}
} while (guess != number);
std::cout << "Congratulations! You've guessed the number." << std::endl;
return 0;
}
Setting Up Your Development Environment
Choosing an IDE
To start with how to make a game in C++, choosing the right Integrated Development Environment (IDE) is crucial. Several popular IDEs cater specifically to C++ game development:
- Visual Studio: Known for its powerful debugging tools and user-friendly interface, making it a preferred choice among many developers.
- Code::Blocks: An open-source IDE that provides a simple interface and is suitable for beginners looking to get started with C++.
- CLion: A powerful cross-platform IDE by JetBrains that offers advanced code assistance and seamless integration with CMake.
Installing Necessary Libraries
To create a game, you'll often need additional libraries to help with graphics, sound, and other essential components. Here are some renowned game development frameworks:
- SFML (Simple and Fast Multimedia Library): A popular choice for 2D games, it simplifies the graphics and sound components significantly.
- SDL (Simple DirectMedia Layer): Widely used for game development, particularly for creating cross-platform applications.
- Allegro: An open-source library that is versatile for 2D games.
To install these libraries, follow each library's installation guide provided on their official websites. Most installations for SFML and SDL can be done via package managers or by compiling from source.
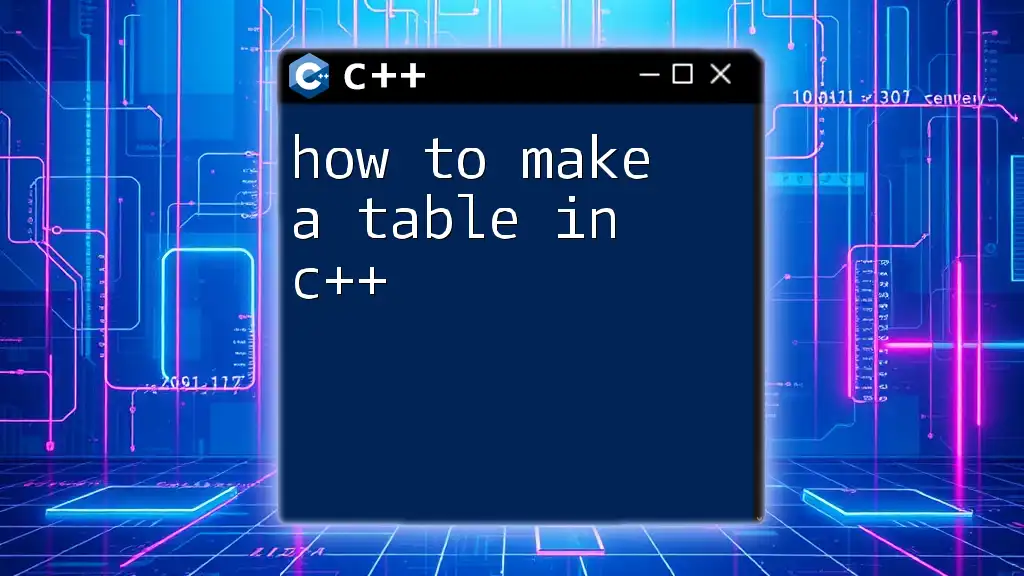
Understanding Basic Game Mechanics
The Game Loop
A core concept in game development is the game loop. This loop continuously runs to create the active gameplay experience. It's mainly responsible for handling user input, updating the game state, and rendering graphics.
Here's an essential structure of a game loop:
while (gameRunning) {
// Handle player input
// Update game state
// Render the game
}
Rendering Graphics
Utilizing SFML simplifies the process of rendering graphics. First, you must initialize a window for your game. Below is a basic example demonstrating how to create a window using SFML:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "My Game");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.display();
}
return 0;
}
This code sets up a simple window that handles closing events, requiring just a few lines of code to get started.
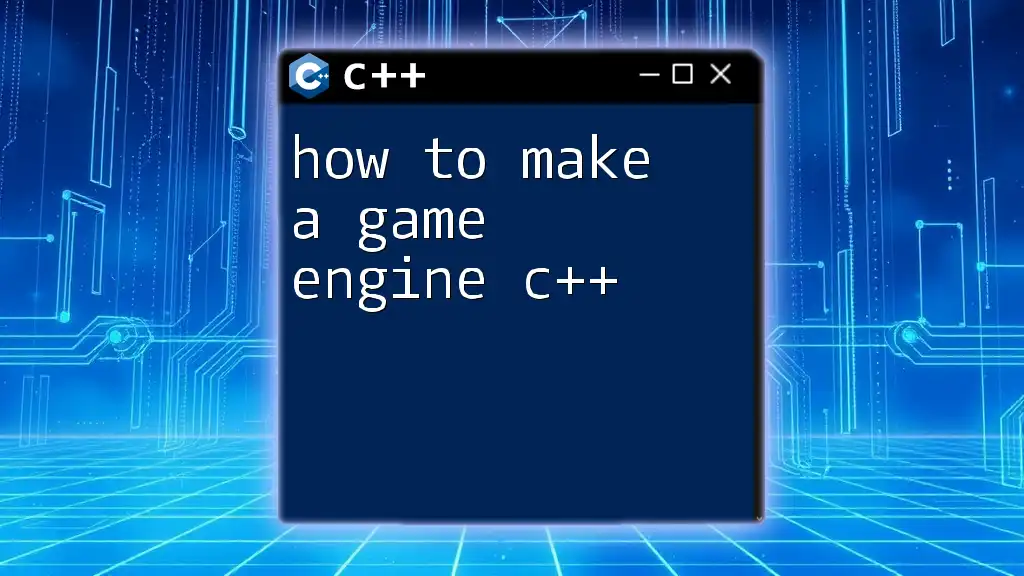
Developing Game Features
Implementing Player Controls
Once you have a basic window, the next step in how to make a game in C++ is implementing player controls. Handling input allows players to interact with your game effectively.
You can use keyboard inputs to move the player character. Here’s an example:
if (sf::Keyboard::isKeyPressed(sf::Keyboard::Right)) {
player.move(speed, 0);
}
This code snippet checks if the right arrow key is pressed and moves the player to the right accordingly.
Adding Game Objects
Creating game objects often involves defining classes. For instance, you can create a `Player` class to encapsulate the player characteristics and behaviors.
Here’s a simple example of a `Player` class:
class Player {
public:
void move(float x, float y) { /* Movement logic */ }
void draw(sf::RenderWindow& window) { /* Rendering logic */ }
};
Organizing your code into classes helps maintain clarity and structure as your game expands.
Collision Detection
A critical component of most games is collision detection. This ensures that game objects interact precisely. A straightforward way to achieve this is through bounding box detection.
Here’s a basic example of checking for collisions:
if (player.getGlobalBounds().intersects(enemy.getGlobalBounds())) {
// Handle collision
}
By checking if the bounds of two objects intersect, you can determine if a collision has occurred and respond accordingly.
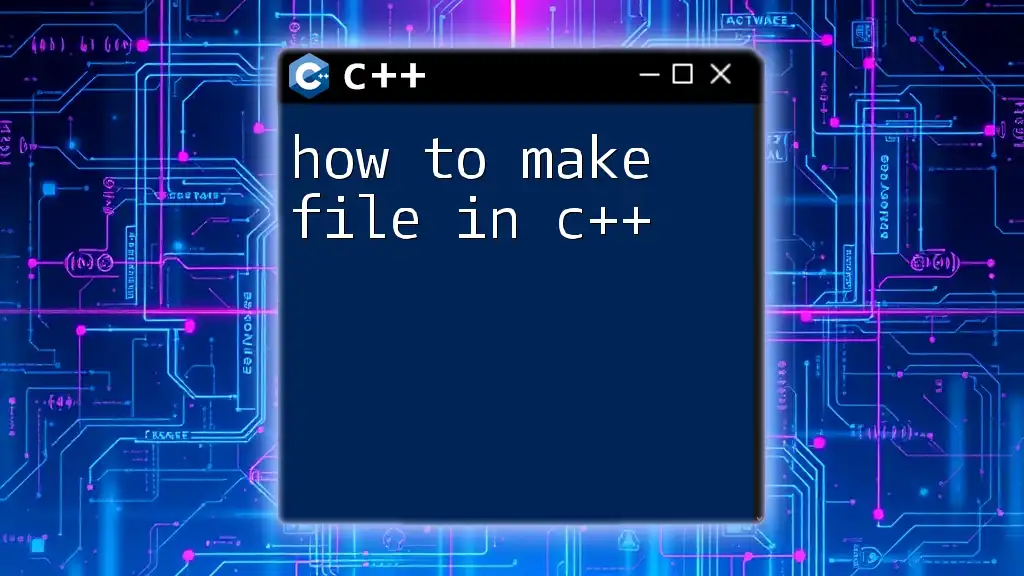
Enhancing the Gameplay
Adding Sound Effects and Music
Incorporating sound can significantly enhance player immersion. SFML provides a user-friendly interface for handling sound and music.
Here’s how you can play a sound effect using SFML:
sf::SoundBuffer buffer;
buffer.loadFromFile("sound.wav");
sf::Sound sound;
sound.setBuffer(buffer);
sound.play();
This script loads a sound file and plays it once the game event that triggers the sound occurs, such as a collision or button press.
Scoring System and Levels
Game mechanics often involve scoring systems that track player performance. Designing an effective scoring system allows players to gauge their progress.
For example, you can maintain a simple score variable and increment it based on certain actions:
int score = 0;
score += 10; // Increment score
This approach not only encourages players to aim for higher scores but also provides a sense of achievement as they advance through levels.
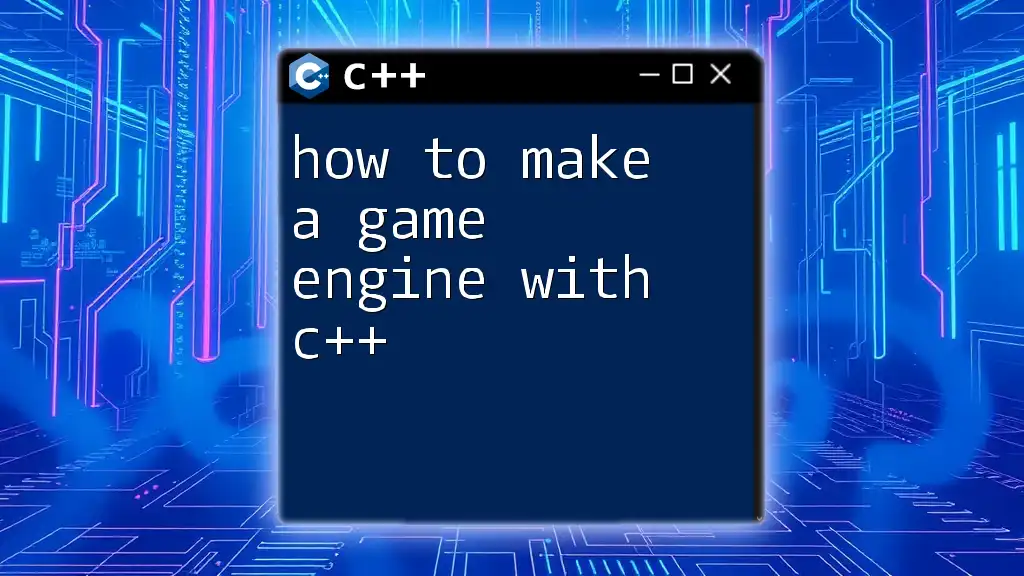
Finalizing Your Game
Testing and Debugging
Testing should be a priority throughout the development process. Key aspects to focus on include gameplay mechanics, performance, and user experience. Utilize debugging tools available in your IDE to resolve any issues efficiently.
Common debugging techniques involve using breakpoints and checking for runtime errors, ensuring that every part of your game functions as intended.
Compiling and Distributing Your Game
Once you've developed your game, the next step is compiling it into an executable format. Each IDE provides a straightforward method to create an executable file, which can then be tested or shared with others.
For distribution, consider platforms like Steam, Itch.io, or even your website, making your game accessible to a broader audience.
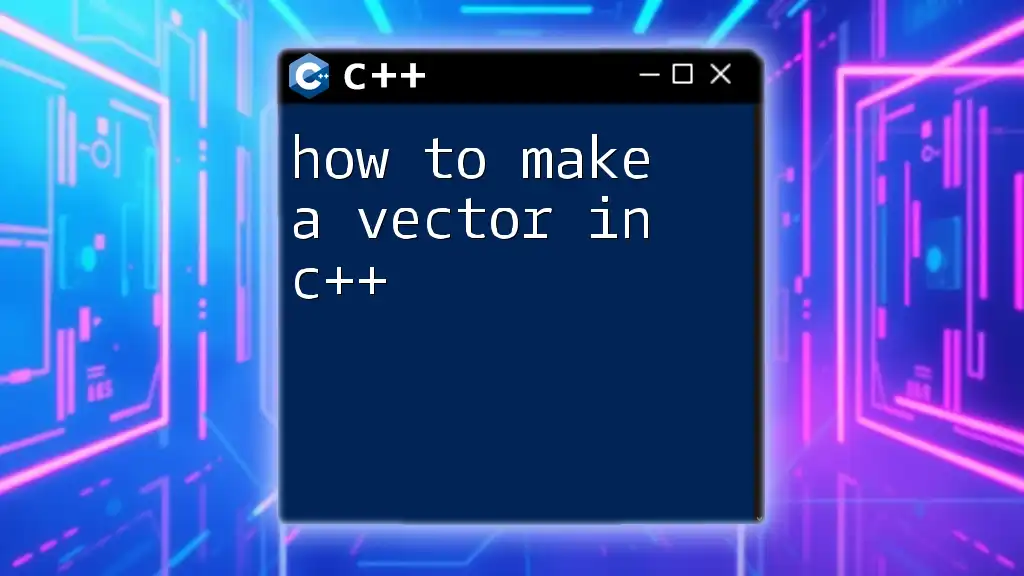
Conclusion
In this guide on how to make a game in C++, we've traversed through setting up your environment, understanding game loops, rendering graphics, and implementing gameplay mechanics.
Additional Resources
As you continue your journey into game development, explore resources such as books, online courses, and forums to further enhance your skills. Engaging with communities can provide support and additional knowledge crucial for your growth as a game developer.
Call to Action
Now it’s your turn! Start implementing these concepts and experiment with your own game projects. Embrace the learning process and share your experiences with fellow developers!