To create a stack in C++, you can use the Standard Template Library (STL) `stack` class, which allows you to add and remove elements in a Last In First Out (LIFO) manner.
#include <iostream>
#include <stack>
int main() {
std::stack<int> myStack;
myStack.push(10);
myStack.push(20);
myStack.push(30);
std::cout << "Top element: " << myStack.top() << std::endl; // Outputs 30
myStack.pop();
std::cout << "New top element after pop: " << myStack.top() << std::endl; // Outputs 20
return 0;
}
Understanding the Stack Data Structure
What is a Stack?
A stack is a fundamental data structure that operates on a Last In, First Out (LIFO) principle. This means the last element added to the stack is the first one to be removed. Think of it as a stack of plates where you can only take the top plate off or add a new one to the top.
Common applications of stacks include:
- Managing function calls in programming languages.
- Implementing undo mechanisms in applications such as text editors.
- Evaluating mathematical expressions and performing backtracking algorithms.
Real-World Analogy
Consider a stack of plates in a cafeteria. You can only add or remove plates from the top, mirroring how a stack data structure functions. This LIFO nature simplifies certain problems in programming and optimizes memory management.

Stack Implementation in C++
Using Standard Library
The Standard Template Library (STL) in C++ provides a robust and efficient way to implement stacks. By utilizing STL, developers can avoid common pitfalls associated with manual implementation and instead focus on higher-level code design.
To use a stack, you first need to include the stack library in your C++ program:
#include <stack>
Creating a Stack in C++
Declaring a Stack
To declare a stack, use the following syntax:
std::stack<int> myStack;
This statement initializes a stack named `myStack` that holds integers.
Basic Operations on Stack
Push Operation
The push operation adds an element to the top of the stack.
For example:
myStack.push(10);
myStack.push(20);
In this example, the numbers 10 and 20 are added to the stack, with 20 being the top element.
Pop Operation
The pop operation removes the top element from the stack.
Here’s how you can use it:
myStack.pop(); // Removes the top element (20 in this case)
After this operation, the new top element would be 10.
Top Operation (Peek)
The top method retrieves the top element without removing it, allowing you to see what's currently at the top of the stack.
Example:
int topElement = myStack.top(); // topElement is 20
This allows you to interact with the top element as needed without modifying the stack.
Empty Operation
You can also check if the stack is empty. This is useful for preventing errors that might occur when trying to access elements in an empty stack.
Here's how to use it:
bool isEmpty = myStack.empty(); // Returns true if the stack is empty
Size Operation
To find out how many elements are currently in the stack, you can use the size method:
int stackSize = myStack.size(); // Returns the number of elements in the stack
Example of Stack in C++
Here’s a complete example demonstrating the various stack operations:
#include <iostream>
#include <stack>
int main() {
std::stack<int> myStack;
// Pushing elements onto the stack
myStack.push(10);
myStack.push(20);
myStack.push(30);
// Displaying the top element
std::cout << "Top element: " << myStack.top() << std::endl; // Outputs 30
// Popping an element
myStack.pop(); // 30 is removed
std::cout << "Top element after pop: " << myStack.top() << std::endl; // Outputs 20
// Displaying stack size
std::cout << "Stack size: " << myStack.size() << std::endl; // Outputs 2
// Checking if the stack is empty
std::cout << "Is the stack empty? " << (myStack.empty() ? "Yes" : "No") << std::endl; // Outputs No
return 0;
}
In this code, we demonstrate how to push, pop, and analyze the properties of the stack. Each step includes necessary output that helps to visualize the current state of the stack.

Custom Implementation of Stack
Building a Stack Class
While the STL provides a ready-made solution for stacks, implementing your own can deepen your understanding of data structures.
Defining the Stack Structure
The first step in custom implementation is defining a node structure to represent each element in the stack:
struct Node {
int data;
Node* next;
};
In this structure, `data` holds the value of the node, and `next` points to the following node.
Class Definition
Next, define the Stack class to encapsulate stack operations:
class Stack {
private:
Node* top; // Pointer to the top of the stack
public:
Stack(); // Constructor
void push(int value);
void pop();
int top();
bool empty();
int size();
};
Implementing Stack Methods
Each method is essential for the stack's functionality:
- Constructor: Initializes the top pointer.
- Push method: Creates a new node and adds it to the top.
- Pop method: Removes the top node from the stack.
- Top method: Returns the value of the top node.
- Empty method: Checks if the stack is empty.
- Size method: Counts the nodes in the stack.
Here’s how you might implement these methods:
class Stack {
private:
Node* top;
public:
Stack() : top(nullptr) {}
void push(int value) {
Node* newNode = new Node();
newNode->data = value;
newNode->next = top;
top = newNode;
}
void pop() {
if (!empty()) {
Node* temp = top;
top = top->next;
delete temp; // Free the memory
}
}
int top() {
if (!empty())
return top->data;
throw std::runtime_error("Stack is empty");
}
bool empty() {
return top == nullptr;
}
int size() {
int count = 0;
Node* current = top;
while (current) {
count++;
current = current->next;
}
return count;
}
};
This implementation provides a simple yet effective way to create a stack using custom nodes.

Conclusion
Creating a stack in C++ can be effectively accomplished using the Standard Template Library or through custom implementations. Each method has its advantages; leveraging STL allows for faster, error-free coding, while building a custom stack deepens understanding of data structures.
Hands-on practice is essential to mastering stack operations. Modify the provided examples or explore stacks in different contexts to solidify your knowledge.

Call to Action
As you continue your journey in C++, consider branching out to explore related data structures such as queues and linked lists. Engaging with the programming community can enhance your skills and provide support. Join discussions, share your experiences, and don’t hesitate to ask questions!
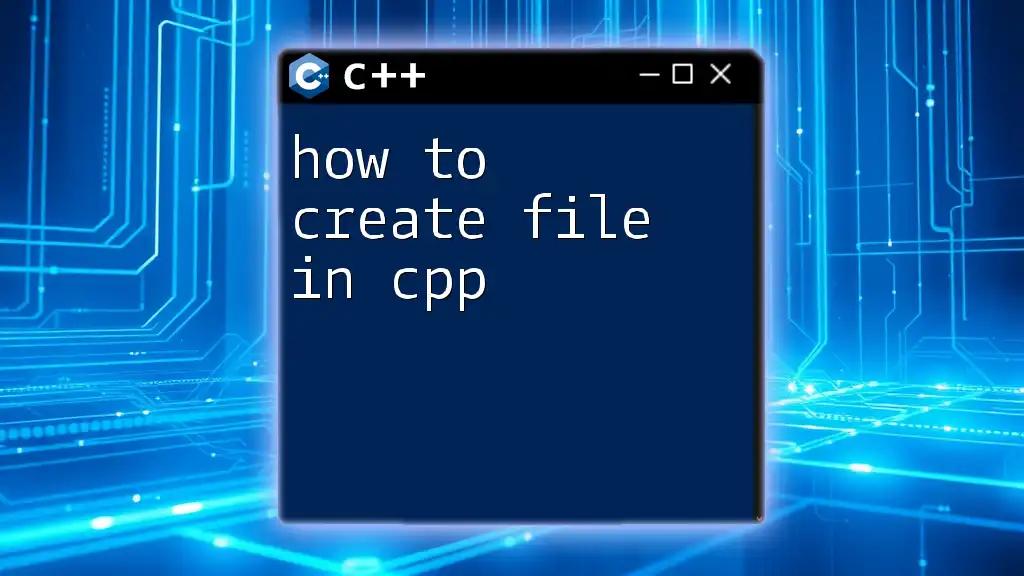
Additional Resources
For further learning, refer to the official C++ documentation and explore recommended books on data structures that can provide deeper insights into the stack and other essential data structures.