`reinterpret_cast` in C++ is used to convert one pointer type to another pointer type, allowing for low-level reinterpreting of the bit pattern of a variable.
Here's a simple example of how to use `reinterpret_cast`:
#include <iostream>
int main() {
int value = 5;
void* ptr = reinterpret_cast<void*>(&value); // Reinterpret int* as void*
int* intPtr = reinterpret_cast<int*>(ptr); // Reinterpret void* back to int*
std::cout << *intPtr << std::endl; // Outputs: 5
return 0;
}
Understanding C++ Type Casting
What is Type Casting in C++?
Type casting in C++ refers to the method of converting one data type into another. This is crucial for various programming scenarios, allowing developers to manipulate data in a way that suits their application's needs. In C++, there are multiple casting methods, each serving specific use cases.
Types of Type Casting in C++
C++ provides several types of casting mechanisms:
- Static Casting: Primarily used for safe conversion of types.
- Dynamic Casting: Focuses on safe downcasting in polymorphic class hierarchies.
- Const Casting: Used to add or remove the `const` qualifier.
- reinterpret_cast: Allows for low-level manipulation of data types.
The focus of this article will be the `reinterpret_cast`, a powerful but potentially risky tool.
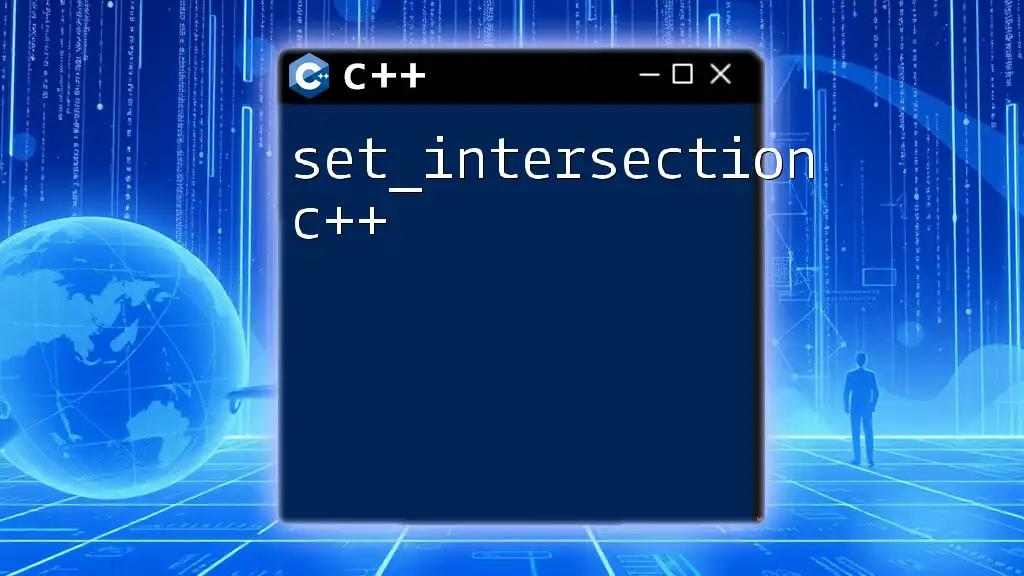
What is reinterpret_cast in C++?
Definition and Purpose of reinterpret_cast
`reinterpret_cast` is one of the four main casting operators in C++. Its primary function is to convert any pointer type to any other pointer type, regardless of their types. This casting is particularly useful in low-level programming, such as systems programming or applications requiring direct memory manipulation.
Syntax of reinterpret_cast
The syntax of `reinterpret_cast` is straightforward. Here’s how you can use it:
TypeName* newPointer = reinterpret_cast<TypeName*>(existingPointer);
This snippet shows the casting of an existing pointer to a new pointer type, potentially leading to different behavior depending on the context of usage.
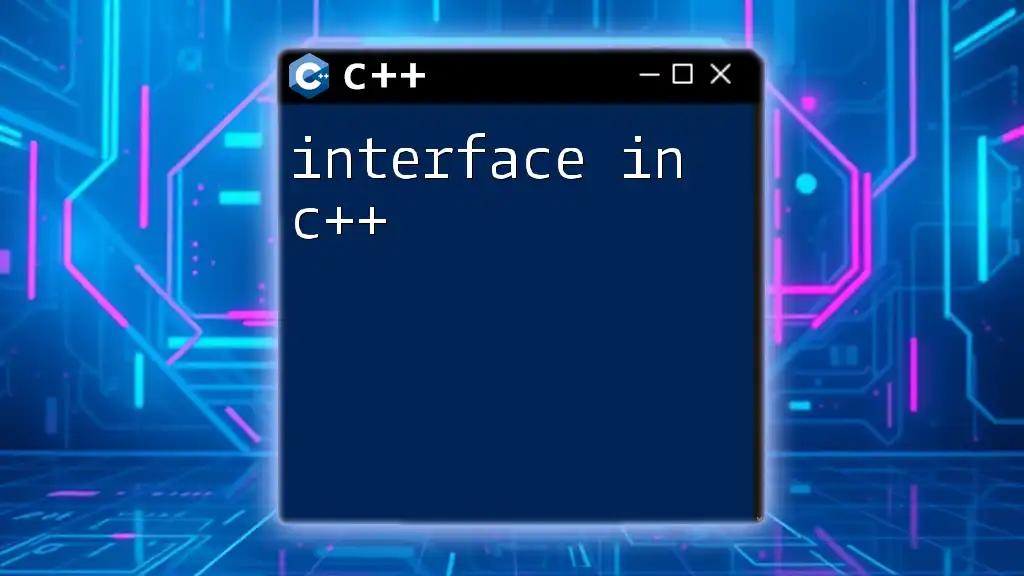
How Does reinterpret_cast Work?
Memory Representation and Type Casting
`reinterpret_cast` operates at a binary level. When you use it, you essentially tell the compiler to treat the memory occupied by a variable as if it were of a different type. This can lead to optimizations, but programmers must be cautious since it bypasses the type safety provided by C++.
Types That Can Be Used with reinterpret_cast
You can perform several types of conversions with `reinterpret_cast`, including:
- Converting between pointer types (e.g., `int*` to `void*`)
- Casting between incompatible class types
- Transforming function pointers
However, using `reinterpret_cast` improperly can result in undefined behavior, so it’s crucial to ensure you're aware of what you're doing.

Safe vs Unsafe Usage of reinterpret_cast
Risks Associated with reinterpret_cast
The flexibility of `reinterpret_cast` comes with risks. It can easily lead to issues such as dereferencing pointers of incorrect types, resulting in memory corruption or crashes. Here are some potential dangers:
- Undefined Behavior: If the type size or layout are misinterpreted.
- Loss of Information: Casting between incompatible types may discard critical data.
When to Use reinterpret_cast
`reinterpret_cast` is appropriate in scenarios that require strict control over memory and performance. Examples include:
- Interfacing with hardware APIs that require specific memory layouts.
- Implementing polymorphic behavior through base class pointers.
However, always consider using safer casting methods before opting for `reinterpret_cast`.

Examples of reinterpret_cast in C++
Basic Examples
Here’s a simple example of using `reinterpret_cast`:
int main() {
int num = 10;
void* ptr = reinterpret_cast<void*>(&num);
// Here we treat the int as a void pointer.
}
In this code, the integer `num` is cast to a void pointer, enabling us to work with it at a lower abstraction level.
Advanced Examples
Let’s explore an example involving structs:
struct A { int x; };
struct B { double y; };
A a;
B* b = reinterpret_cast<B*>(&a);
// Now we have casted a pointer of type A to type B.
In this scenario, the `reinterpret_cast` allows us to access memory allocated for `A` as if it were of type `B`. It’s vital to remember the layout and size of the data structures to avoid potential issues.
Using reinterpret_cast with Function Pointers
Function pointers also lend themselves to `reinterpret_cast`:
typedef void (*FuncPtr)();
void myFunction() {}
FuncPtr func = reinterpret_cast<FuncPtr>(&myFunction);
// Now func can be called as if it were a function pointer to myFunction.
This example demonstrates how `reinterpret_cast` is used for converting between function pointers. Calling `func()` will execute `myFunction`, but care must be taken to ensure the function signature matches.
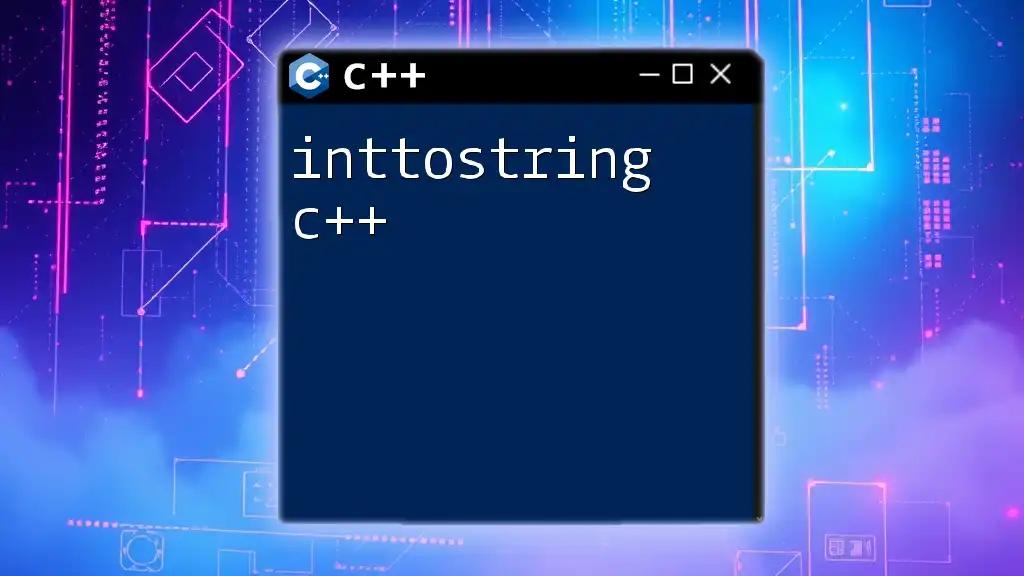
Common Mistakes with reinterpret_cast
Incorrect Usage Patterns
A common mistake is assuming the data sizes and alignments are interchangeable. For instance, casting an `int*` to a `char*` and then attempting to read it as an `int*` later can lead to data misinterpretation.
Debugging reinterpret_cast Issues
To diagnose problems arising from `reinterpret_cast`, use tools like memory analyzers. Ensure to validate pointer types before dereferencing, as this can prevent costly runtime errors.
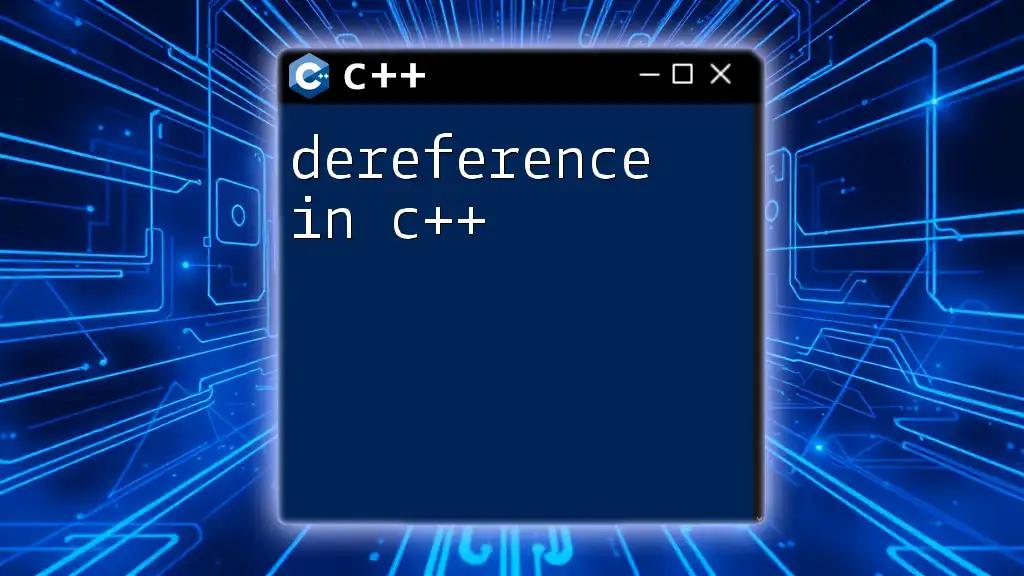
Best Practices with reinterpret_cast
Guidelines for Safe Usage
To safely utilize `reinterpret_cast`, follow these guidelines:
- Use as a Last Resort: Consider alternatives like `static_cast` or `dynamic_cast` first.
- Understand Data Layouts: Fully grasp the memory layouts of the types you are working with.
- Encapsulate in Functions: Keeping `reinterpret_cast` usage confined to specific functions can aid clarity and maintainability.
Alternatives to reinterpret_cast
When working with polymorphic classes, prefer `dynamic_cast` for safe downcasting. Use `static_cast` for more straightforward conversions when type safety is guaranteed.

Conclusion
Summary of reinterpret_cast
In summary, `reinterpret_cast in C++` is a potent casting tool that provides flexible data type handling. However, with this power comes responsibility; understanding its implications and exercising caution is essential.
Final Thoughts
C++'s casting mechanisms serve various programming needs, and mastering them enhances your development capability. Learning to wield `reinterpret_cast` wisely can tremendously benefit systems programming and type-safe object modeling.
Call to Action
Join our C++ command sessions to explore more about type casting and reinforce your understanding of `reinterpret_cast` and other casting methods. Enhance your coding repertoire with precise and effective C++ techniques!