Creating threads in C++ can be accomplished using the `<thread>` library, allowing you to run multiple threads concurrently for more efficient processing.
Here's a simple example:
#include <iostream>
#include <thread>
void hello() {
std::cout << "Hello from thread!" << std::endl;
}
int main() {
std::thread t(hello); // Create a new thread that runs the hello function
t.join(); // Wait for the thread to finish
return 0;
}
Understanding C++ Threading Models
C++ supports multithreading, allowing multiple threads to execute concurrently. This is essential for maximizing performance and responsiveness in applications, especially those that require parallel processing.
Key Components of the Standard C++ Thread Library
The Standard C++ Thread Library provides several key components that developers need to understand to work effectively with threads:
- `std::thread`: This is the main class used to represent a thread in C++. It facilitates the execution of a function or callable object in a separate thread.
- `std::mutex`: A mutex (mutual exclusion) is crucial for preventing data races by ensuring that only one thread can access shared resources at a time.
- `std::condition_variable`: This allows threads to wait and be notified of changes in shared data state, enabling better synchronization between threads.
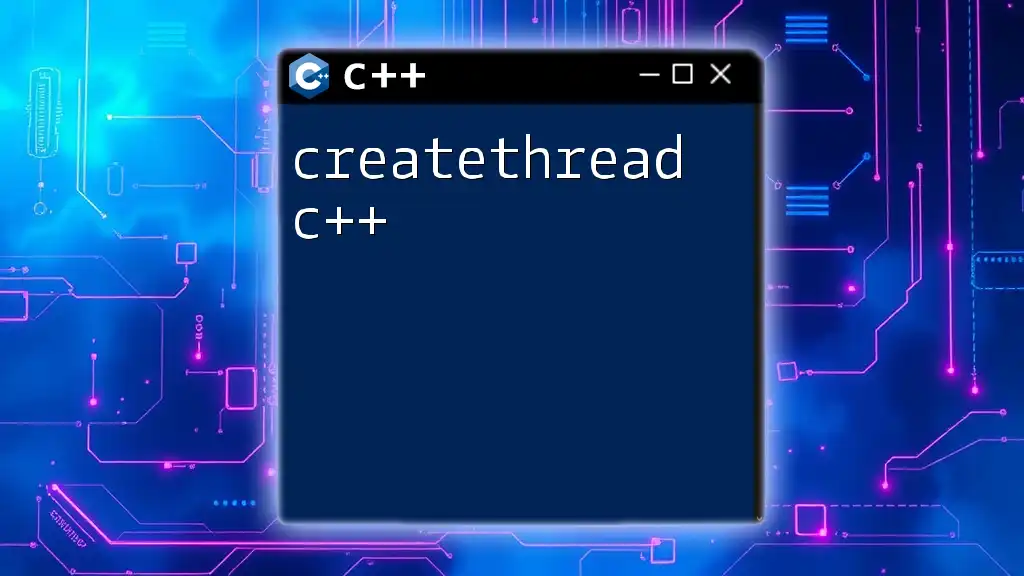
How to Create a Thread in C++
Creating a thread in C++ is straightforward, thanks to the `std::thread` class. To create a thread, you need a callable object (function, lambda, etc.) that represents the task you want to execute concurrently.
Basic Syntax of Thread Creation
The syntax to create a thread is as follows:
std::thread myThread(callableObject);
Example: Creating a Simple Thread
Here’s a basic example of creating a simple thread that executes a function:
#include <iostream>
#include <thread>
void threadFunction() {
std::cout << "Hello from the thread!" << std::endl;
}
int main() {
std::thread myThread(threadFunction); // Create a new thread
myThread.join(); // Wait for the thread to finish
return 0;
}
In this example, `threadFunction()` prints a message. The `std::thread` object `myThread` is constructed with `threadFunction` as the callable object. The `join()` method is called to ensure that the main thread waits until `myThread` completes its execution. This is crucial because it helps prevent program termination before the thread has finished running.
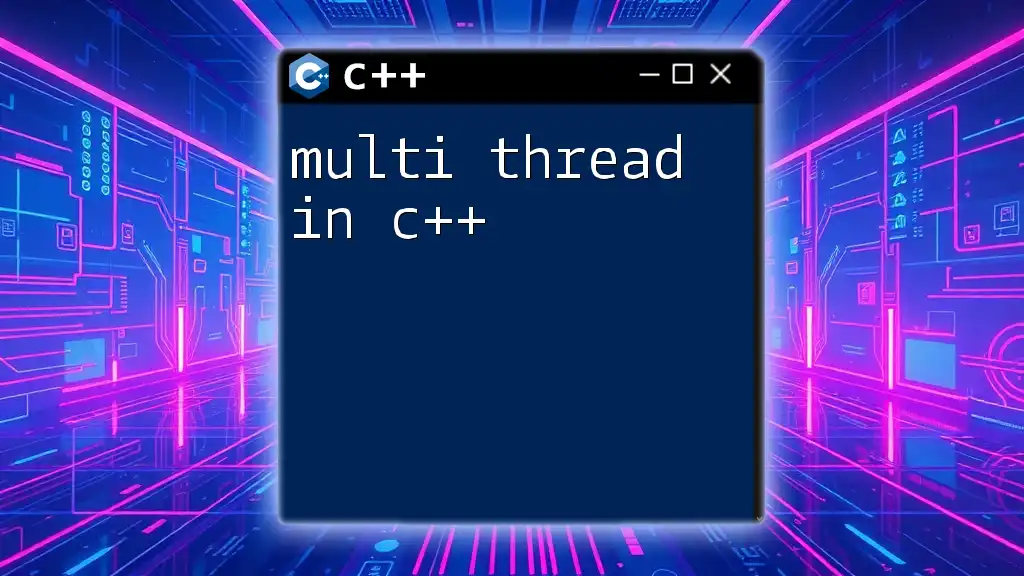
Managing Threads Effectively
Detaching Threads
Sometimes, you may not need to wait for a thread to finish. In such cases, you can detach a thread. Detaching allows the thread to run independently, without waiting for it to complete.
When and Why to Detach a Thread
Detaching is useful for fire-and-forget tasks where you do not need to coordinate with the thread's execution. However, ensure that any resources the thread accesses remain valid during its execution.
Example of Detaching a Thread
Here’s how you can detach a thread:
#include <iostream>
#include <thread>
void threadFunction() {
std::cout << "Thread is running independently!" << std::endl;
}
int main() {
std::thread myThread(threadFunction);
myThread.detach(); // Continue without waiting for myThread
// Main thread continues executing...
return 0;
}
In this example, the main thread continues its execution without waiting for `myThread`, which runs in the background.
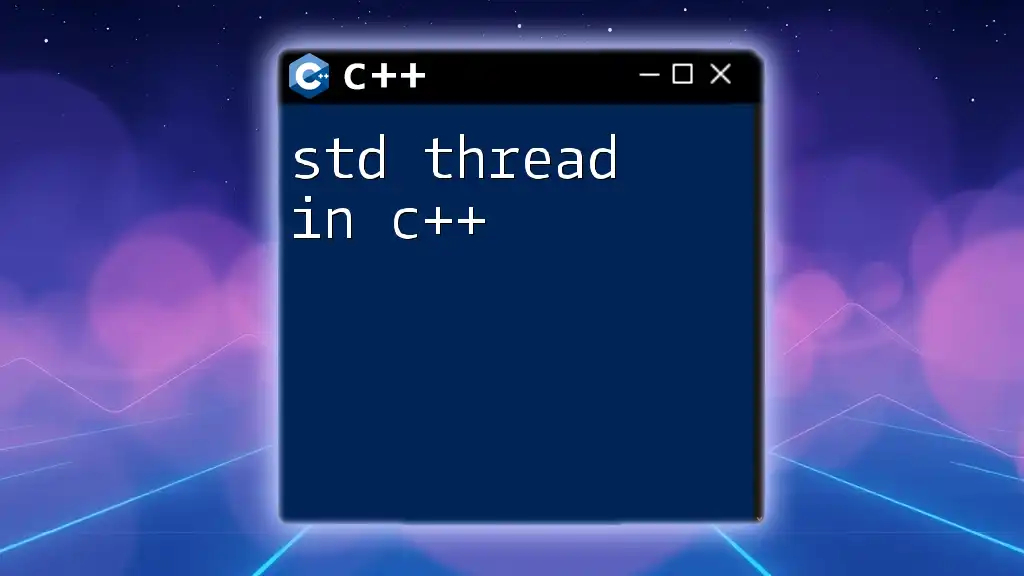
Passing Arguments to Threads
When creating threads, you often need to execute functions that require parameters. C++ provides ways to pass arguments to thread functions.
By Value vs. By Reference
You can pass parameters by value or by reference. Passing by value copies the argument, while passing by reference shares the original argument.
Example: Passing Arguments to a Thread Function
Here’s an example demonstrating both techniques:
#include <iostream>
#include <thread>
void threadFunction(int value) {
std::cout << "Value: " << value << std::endl;
}
int main() {
int value = 10;
std::thread myThread(threadFunction, value);
myThread.join(); // Wait for the thread to finish
return 0;
}
This example shows how to pass an integer to `threadFunction`. Since `value` is passed by value, it is copied into the thread function.
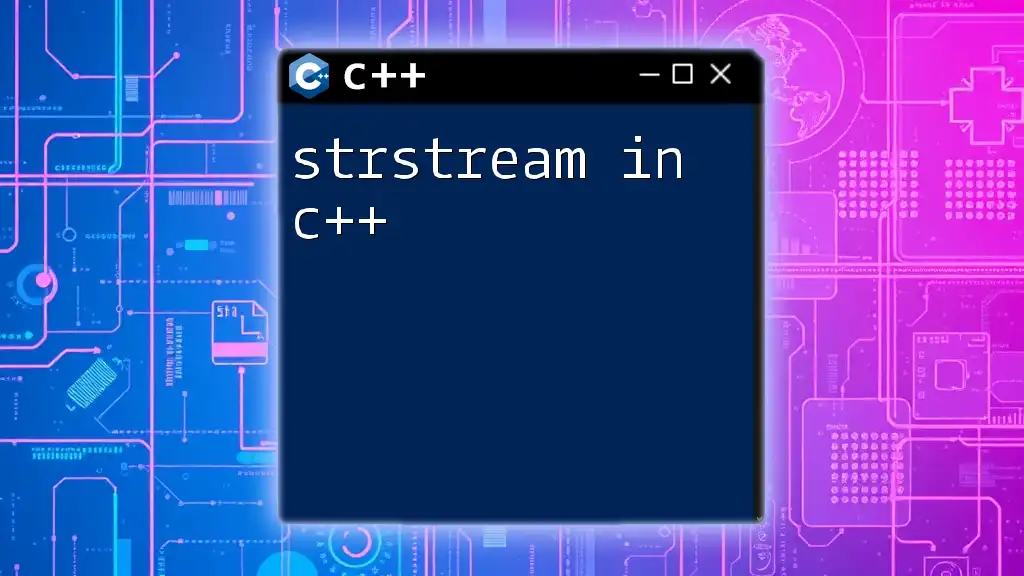
Synchronization of Threads
Introduction to Synchronization
In a multithreaded environment, synchronization is crucial to prevent data corruption and race conditions. When multiple threads access shared data simultaneously, it can lead to unpredictable outcomes.
Common Synchronization Primitives
- Mutexes: Use mutexes to control access to shared resources, ensuring that only one thread can access the critical section at a time.
- Condition Variables: These are used to block a thread until a certain condition is met, allowing for efficient signaling between threads.
Example: Using `std::mutex` to Synchronize Threads
Here’s a simple example of using a mutex to protect a shared resource:
#include <iostream>
#include <thread>
#include <mutex>
std::mutex myMutex;
void threadFunction() {
myMutex.lock(); // Lock the mutex
std::cout << "Critical Section Accessed" << std::endl;
myMutex.unlock(); // Unlock the mutex
}
int main() {
std::thread myThread(threadFunction);
myThread.join();
return 0;
}
In this snippet, the mutex `myMutex` ensures that the "Critical Section Accessed" message prints safely without interruption from other threads accessing the same section.
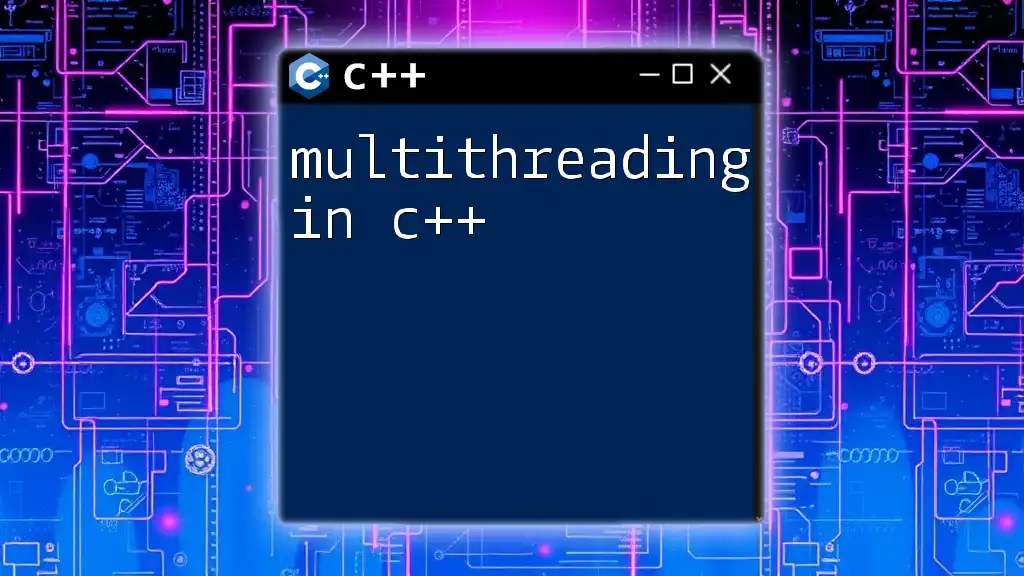
Thread Safety and Best Practices
Understanding thread safety is paramount in multithreading. Here are some best practices:
- Always use mutexes to protect shared resources.
- Avoid global variables in multithreaded applications.
- Use atomic operations when appropriate to ensure lock-free programming.
- Be cautious of deadlocks—always follow a consistent lock ordering.
![Understanding Literals in C++ [A Quick Guide]](/images/posts/l/literals-in-cpp.webp)
Advanced Thread Management Techniques
Using Thread Pools
Thread pools can efficiently manage a group of threads that can be reused for executing tasks. This avoids the overhead of creating and destroying threads for each task.
What is a Thread Pool?
A thread pool maintains a pool of worker threads that can execute tasks concurrently. Tasks are queued and assigned to available threads in the pool.
Benefits of Using Thread Pools
- Reduced Overhead: Saves the cost of thread creation and destruction.
- Controlled Concurrency: Limits the number of concurrent threads for resource management.
Example: Implementing a Simple Thread Pool
Implementing a complete thread pool is beyond the scope of this article, but you can look into libraries such as `ThreadPool` or create your own following patterns like worker-queue.
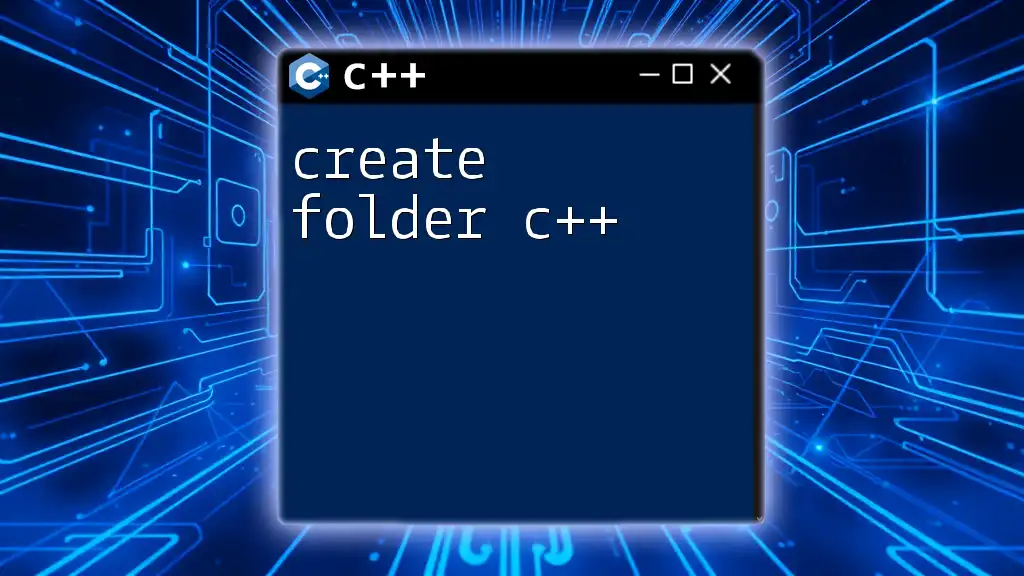
Debugging Multithreaded Applications
Debugging multithreaded applications can be challenging due to race conditions and unpredictable thread execution order. Some common techniques include:
- Use dedicated debugging tools that support multithreading (e.g., Valgrind, Visual Studio Debugger).
- Add log statements to track thread execution.
- Identify deadlock situations by analyzing thread states and locks held.
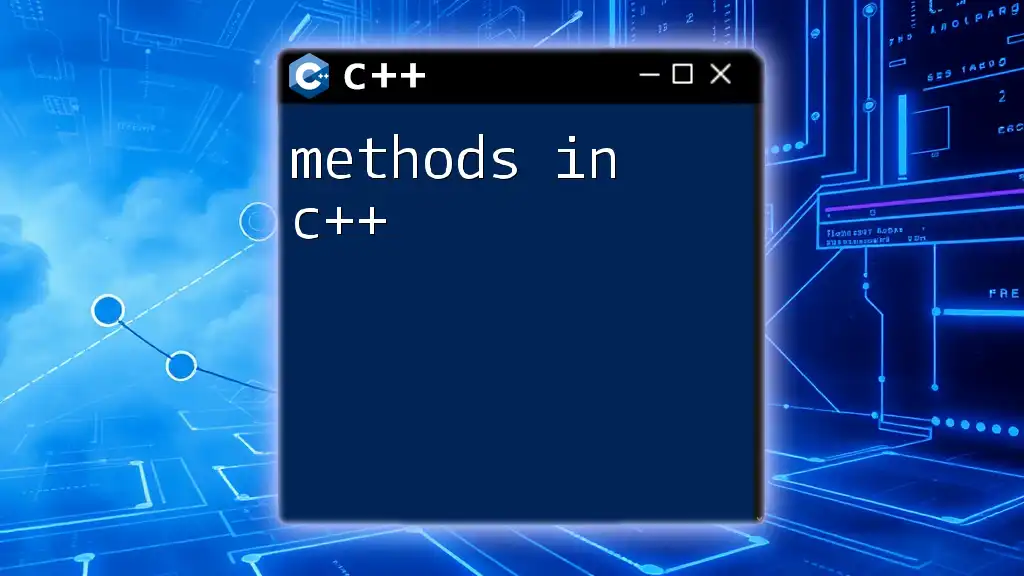
Conclusion
By understanding how to create threads in C++, manage them effectively, and synchronize their actions, you can leverage the full power of multithreading in your applications. Always keep best practices in mind to ensure safe and efficient threading. Practice regularly to build your proficiency, and utilize available resources to enhance your learning journey.