The `istream` class in C++ is used to read input from various sources, including standard input, files, and strings, and can be utilized with the `>>` operator or the `getline()` function to fetch data efficiently.
Here's a code snippet demonstrating how to read an integer and a string using `istream`:
#include <iostream>
#include <string>
int main() {
int number;
std::string name;
std::cout << "Enter an integer: ";
std::cin >> number;
std::cout << "Enter your name: ";
std::cin.ignore(); // clear the newline character from the input buffer
std::getline(std::cin, name);
std::cout << "You entered: " << number << " and your name is " << name << std::endl;
return 0;
}
Understanding `istream`
What is `istream`?
`istream` is a fundamental component of C++ input/output operations, serving as the base class for input streams. It provides a standardized way for input handling and is used extensively throughout C++ programming for reading data from various sources, including the keyboard and files.
Key Features of `istream`
`istream` boasts several essential features that enhance input handling:
- Buffering Input: This means that input is temporarily stored in a buffer before being processed, which can significantly improve performance, especially with larger datasets.
- Input Formatting and State Flags: `istream` maintains various state flags that indicate the status of the input stream, allowing developers to manage and respond to different input conditions effectively.
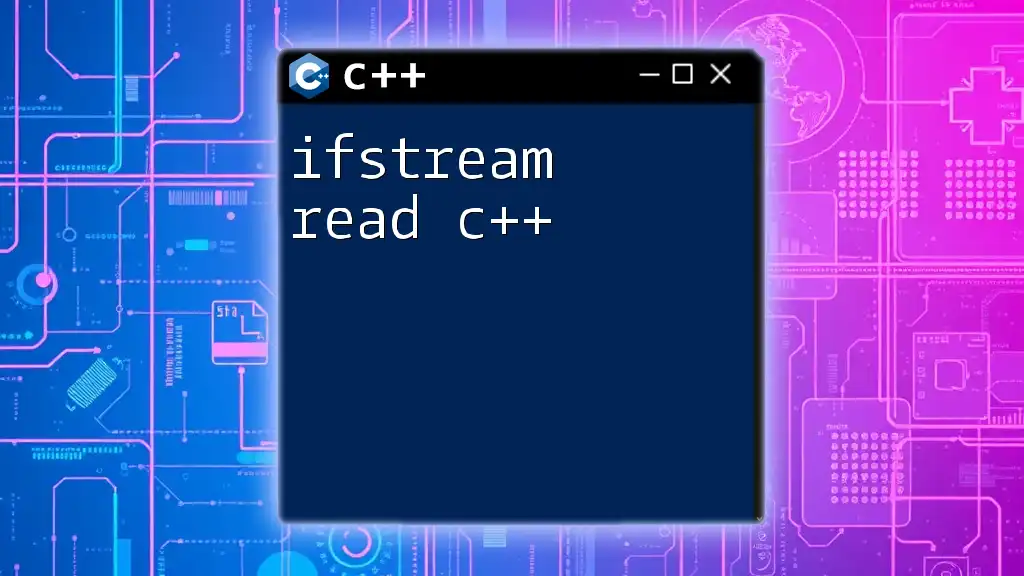
Basic Usage of `istream`
How to Include `istream` in Your Code
To use `istream` effectively, you should include the necessary headers and declare the relevant namespaces. The most common way to achieve this is by including the `<iostream>` header:
#include <iostream>
using namespace std;
Reading from Standard Input
The most common instance of `istream` is `cin`, which is used for reading input from the standard input (usually the keyboard). Here’s how to read an integer:
int number;
cin >> number;
cout << "You entered: " << number << endl;
In this code snippet, `cin` reads an integer value and stores it in the variable `number`, which is then printed out.
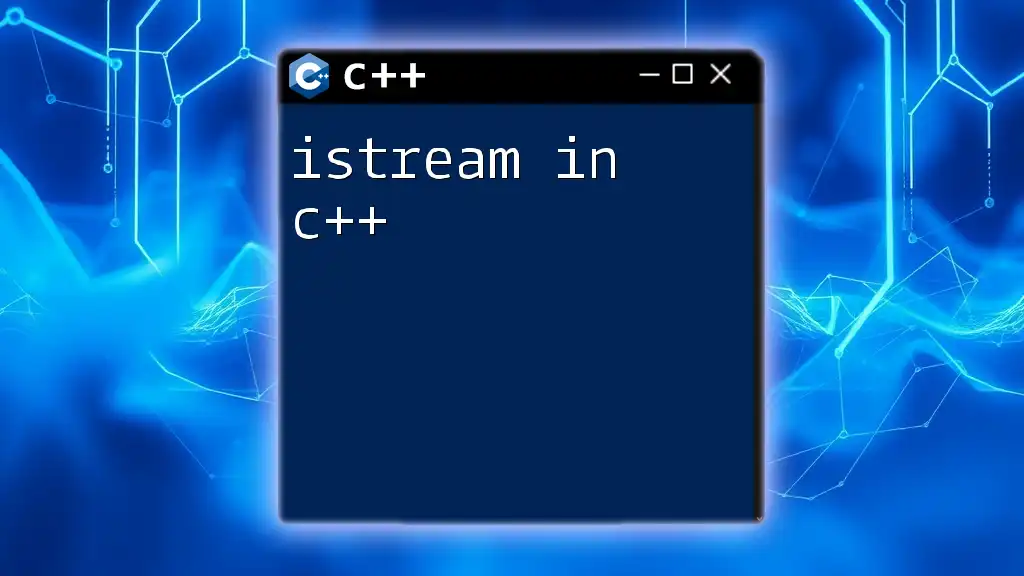
Reading Different Data Types with `istream`
Reading Integers
You can read integers directly using `cin`, as demonstrated in the following example:
int a;
cin >> a;
cout << "You entered integer: " << a << endl;
Reading Floating Point Numbers
For floating point numbers, the process is similar:
double b;
cin >> b;
cout << "You entered: " << b << endl;
Reading Strings
To read strings, you can use both `getline` and `cin`. However, each method serves different purposes. `getline` reads an entire line of text, including spaces, while `cin` reads until the first whitespace:
string name;
cout << "Enter your name: ";
getline(cin, name);
cout << "Hello, " << name << endl;
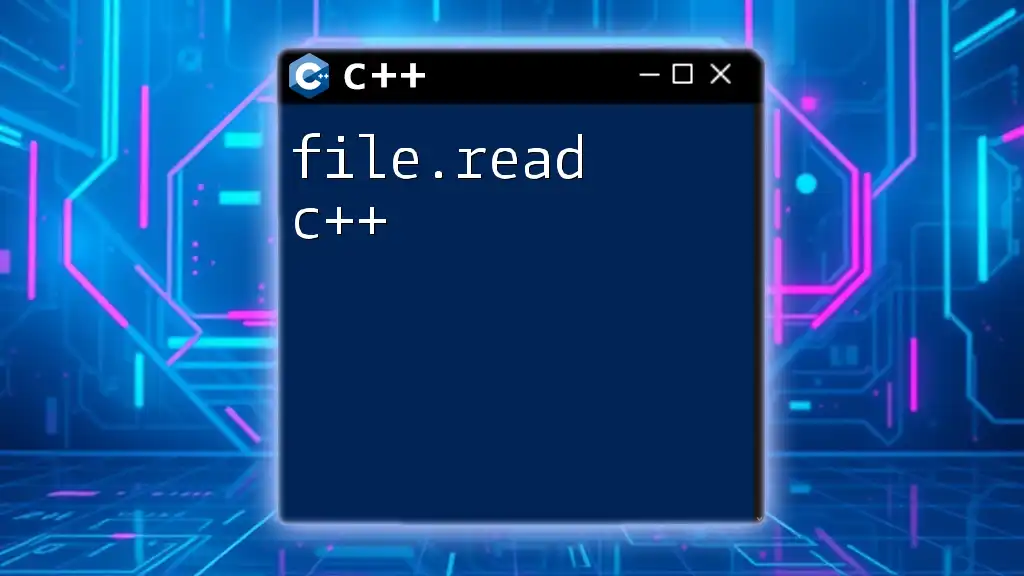
Advanced `istream` Features
Input Validation Techniques
Input validation is crucial to ensure the integrity of the data being received. One common technique is to check if the input operation failed. If it does fail, you should gracefully handle the error:
int input;
while (!(cin >> input)) {
cin.clear(); // clear the error flag
cin.ignore(numeric_limits<streamsize>::max(), '\n'); // ignore the rest of input
cout << "Invalid input, please enter a number: ";
}
This loop will continue until the user provides a valid integer, thus ensuring that the program does not enter an inconsistent state.
Using `istream` with Files
You can also use `istream` with file input. The `ifstream` class allows you to read from files easily:
ifstream file("data.txt");
if (!file) {
cerr << "Error opening file!" << endl;
return 1;
}
string line;
while (getline(file, line)) {
cout << line << endl;
}
In this example, the program attempts to open "data.txt" and reads its content line by line, printing each line to the console. Always check if the file opened successfully to avoid runtime errors.
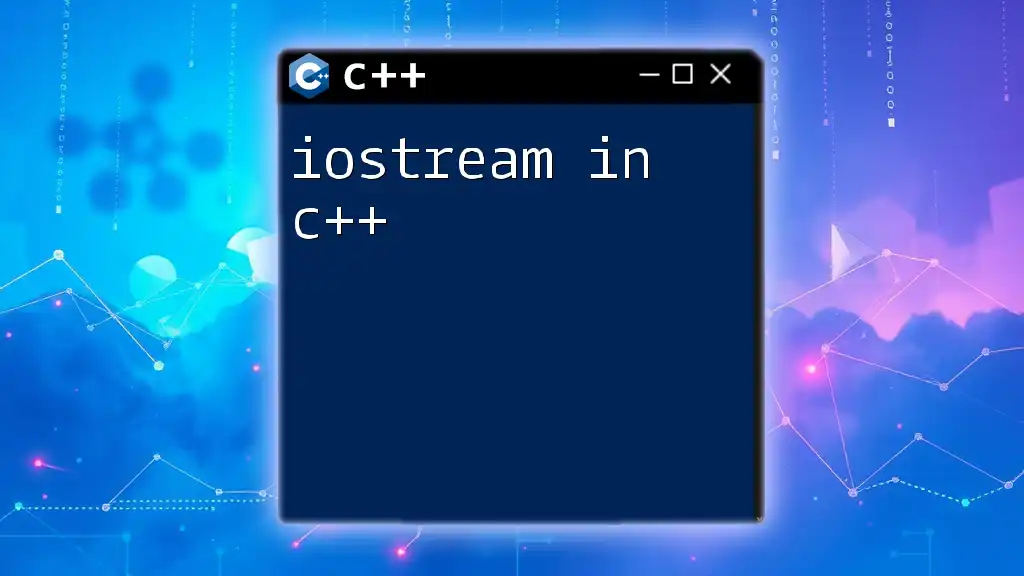
Best Practices for Using `istream`
Efficient Input Handling
To maximize input performance, consider the following tips:
- Use `cin.tie(0)` in performance-critical code to untie `cin` from `cout`, minimizing unnecessary flushes.
- Avoid excessive input/output operations in tight loops, as this can lead to significant slowdowns.
Common Pitfalls to Avoid
When using `istream`, there are common mistakes that developers often make:
- Misunderstanding Buffer Behavior: Always remember that input operations can leave characters in the input buffer, especially when switching between `cin` and `getline`.
- Forgetting to Check Input Stream State: Always verify the state of the input stream after reading. If the state is fail, it could indicate a problem that needs to be addressed before proceeding.
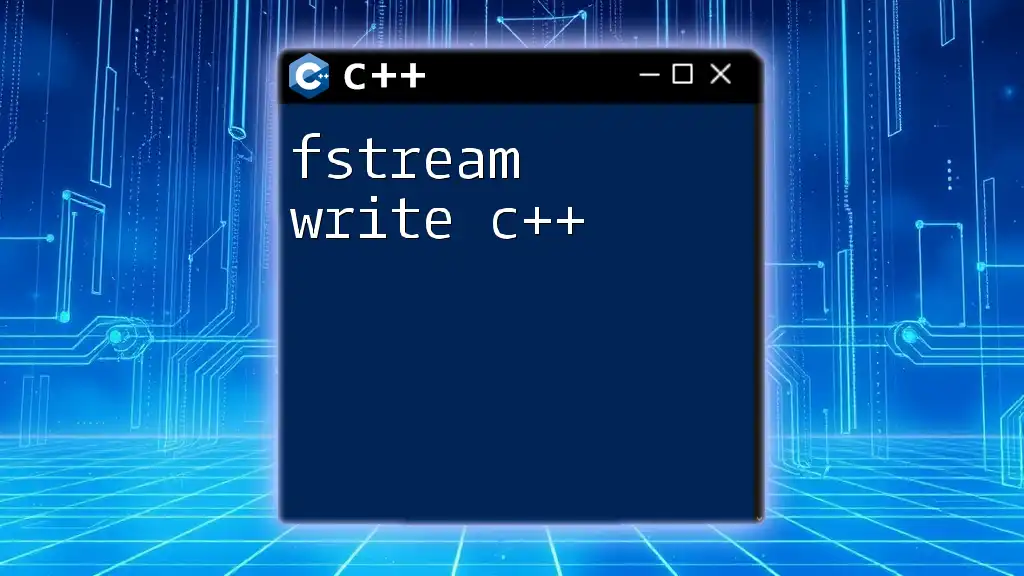
Comparing `istream` with Other Input Methods
`scanf` and `cin`: Similarities and Differences
Both `scanf` and `cin` facilitate reading user input, but `cin` is C++'s preferred approach due to its type safety and flexibility. While `scanf` allows formatted reading, it is less type-safe compared to the stream-based approach of `cin`.
When to Use `istream` vs. `stdin`
In modern C++ applications, favoring `istream` provides the added benefits of C++ features such as object-oriented programming, making your code both cleaner and more maintainable.
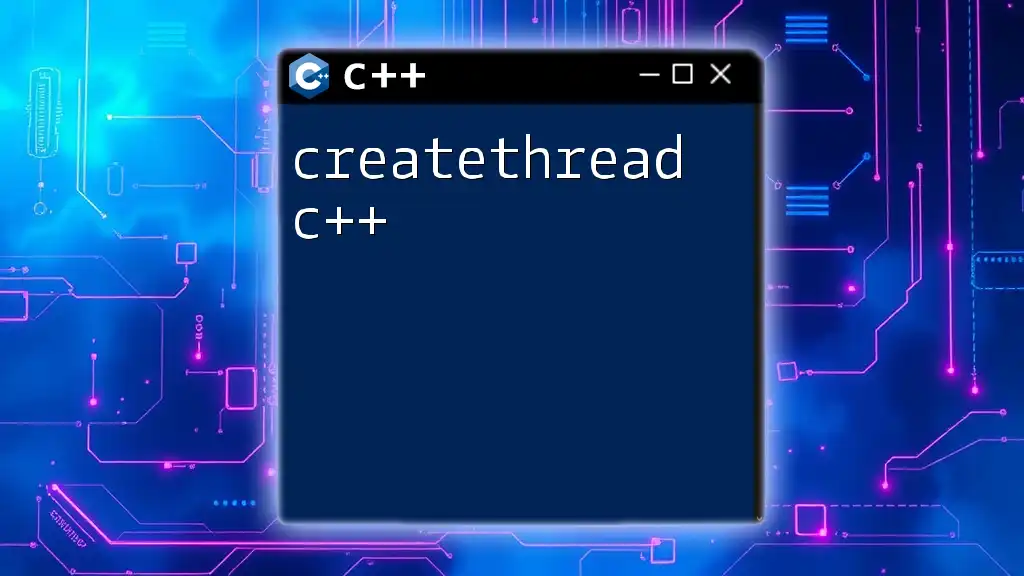
Troubleshooting Common Issues
Debugging Input Problems
If you experience issues with input, one effective way to debug is to inspect the state of the `istream`:
if (cin.fail()) {
cout << "Input failed!" << endl;
}
This simple check helps you determine if input operations are succeeding.
Real-world Scenarios and Solutions
Practicing with various input scenarios can help solidify your understanding. For example, consider how to handle cases where expected input types differ or when dealing with multiple inputs in a single line.
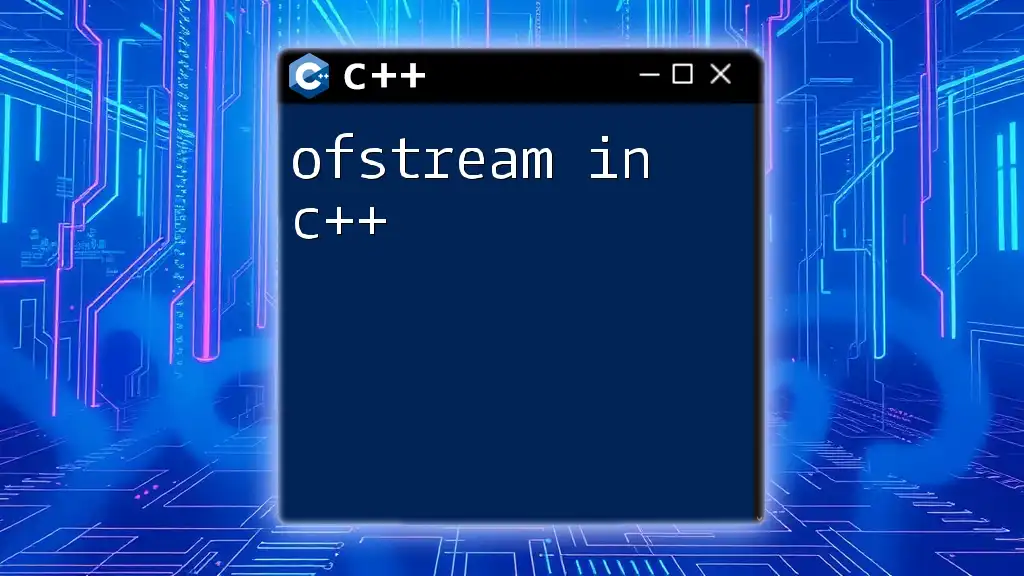
Conclusion
Understanding how to effectively use `istream` for reading data in C++ is fundamental to creating robust applications. With attention to input validation, error handling, and familiarity with advanced features like file I/O, you will be well-prepared to handle various input scenarios. Engage in hands-on practice to develop your skills further and confidently utilize `istream` in your C++ programming journey.
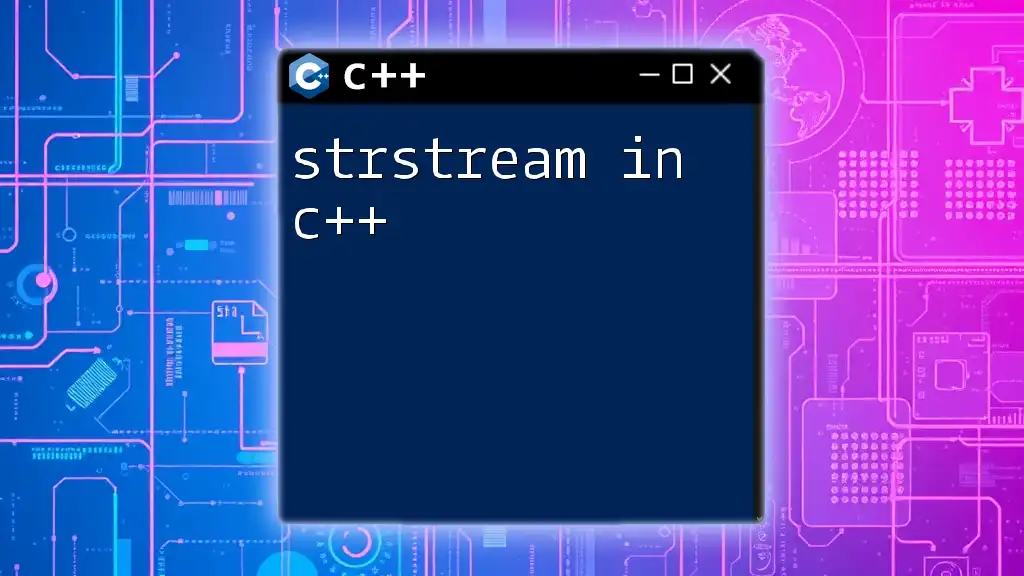
Additional Resources
To deepen your understanding of `istream` and C++ in general, explore further reading materials, such as well-reviewed books and reliable online tutorials. Additionally, consider joining C++ forums or online communities where you can seek support and share knowledge with fellow learners.