The Steam Deck C++ runtime provides developers with the necessary tools and libraries to create and run C++ applications on the Steam Deck platform, ensuring optimal performance and compatibility.
Here's a simple example of a "Hello, World!" program in C++ that you could run in the Steam Deck development environment:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding the Steam Deck
What is the Steam Deck?
The Steam Deck is a handheld gaming device developed by Valve Corporation, designed to deliver a comprehensive gaming experience similar to that of a desktop PC. It runs on Steam OS, a Linux-based operating system that facilitates access to a vast library of games available on the Steam platform. The device boasts notable specifications, including a custom AMD APU that provides enough power for modern gaming.
Why C++ for Game Development?
When it comes to game development, C++ stands out as a premier choice for several reasons:
- Performance: C++ offers speed and efficiency, vital for rendering graphics and handling complex calculations, which are essential in gaming.
- Control: Developers have fine control over system resources, memory management, and hardware, allowing for optimized performance.
- Industry Standard: Many game engines and frameworks, including Unreal Engine and Unity, offer extensive support for C++, making it a staple in the industry.
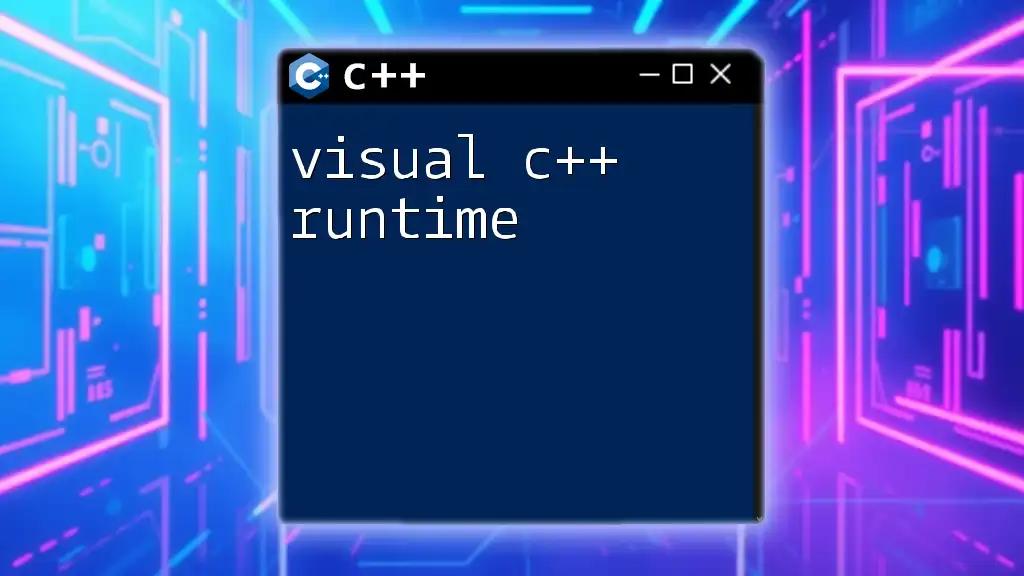
Setting Up Your Development Environment
Required Tools and Libraries
Before diving into C++ development on the Steam Deck, you need to set up your environment. Essential tools include:
- Compiler: A C++ compiler like GCC or Clang.
- Build System: Tools such as CMake or Make to organize your project efficiently.
- Libraries: Popular graphics libraries like SDL or SFML for rendering and input processing.
Installation Guidelines
Setting up your environment involves several steps. To illustrate the process:
-
Install the Compiler: For instance, using `apt` on the Steam Deck's Linux terminal:
sudo apt install build-essential
-
Set Up Your Libraries: Suppose you want to use SDL, you can install it with:
sudo apt install libsdl2-dev
These commands will prepare your system for C++ development.
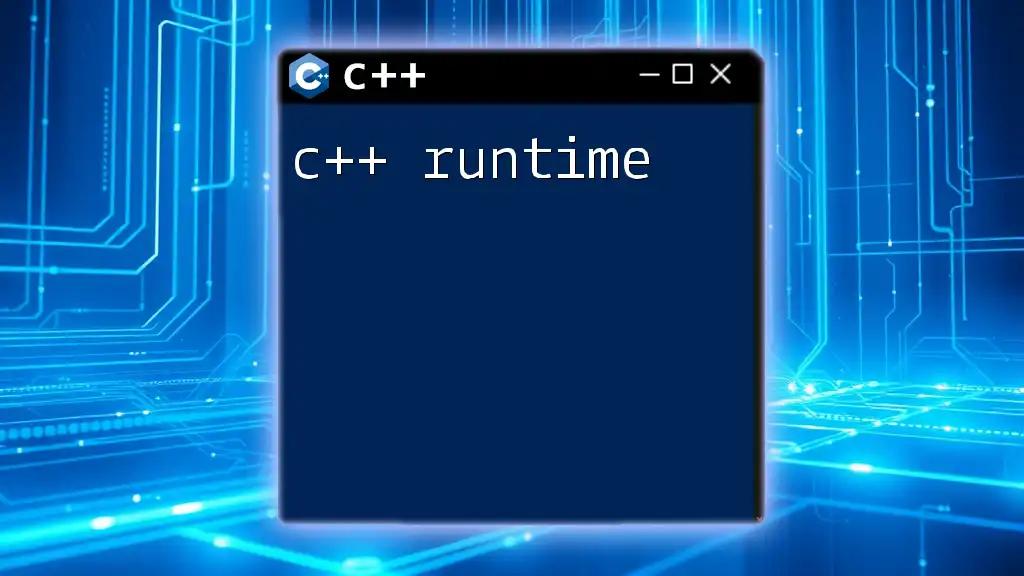
The C++ Runtime Environment on Steam Deck
Overview of C++ Runtime
The C++ runtime is a crucial component that enables your applications to execute smoothly. On the Steam Deck, it consists of the necessary libraries and tools required to run C++ applications effectively within the operating system. Understanding how this environment operates is vital for successful development.
Managing Dependencies and Libraries
Managing C++ libraries and dependencies effectively is paramount. Utilizing package managers, like vcpkg or Conan, simplifies this process significantly. For example, you can install libraries using vcpkg with simple commands:
./vcpkg install sdl2
This approach allows you to easily integrate and manage dependencies throughout the development process.
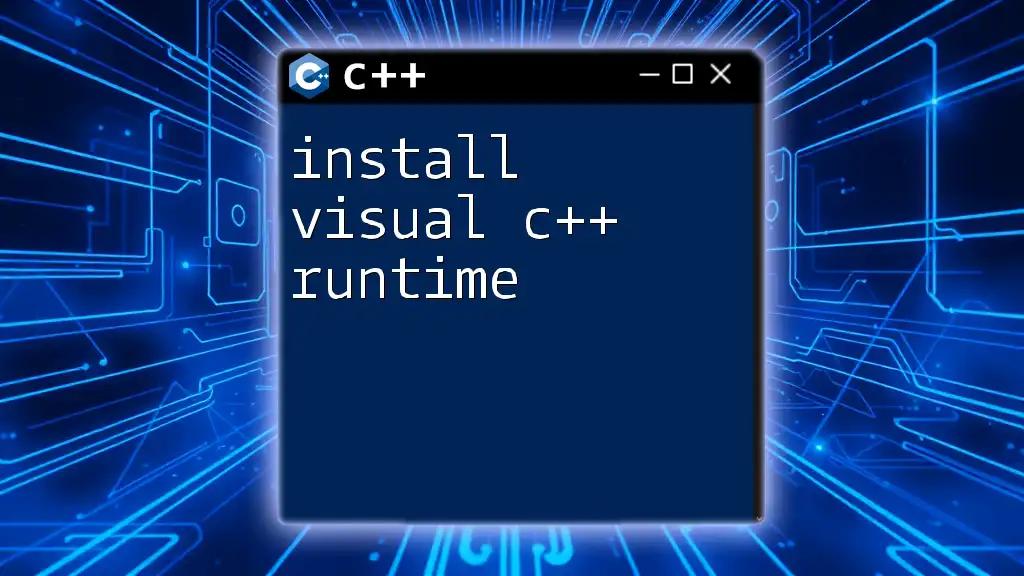
Writing Your First C++ Application for Steam Deck
Creating a Simple Project
Let's get started with a basic C++ application. Create a new folder for your project, and within it, create a file named `main.cpp`. Here’s a simple example to begin:
#include <iostream>
int main() {
std::cout << "Welcome to Steam Deck Development!" << std::endl;
return 0;
}
This example includes the basic structure of a C++ program, which consists of the `main()` function—the entry point of the application.
Compiling Your Application
To compile your code for the Steam Deck, use the `g++` compiler from the terminal. Navigate to your project folder and execute:
g++ main.cpp -o my_first_app
This command compiles the `main.cpp` file and outputs an executable named `my_first_app`. Run your program with:
./my_first_app
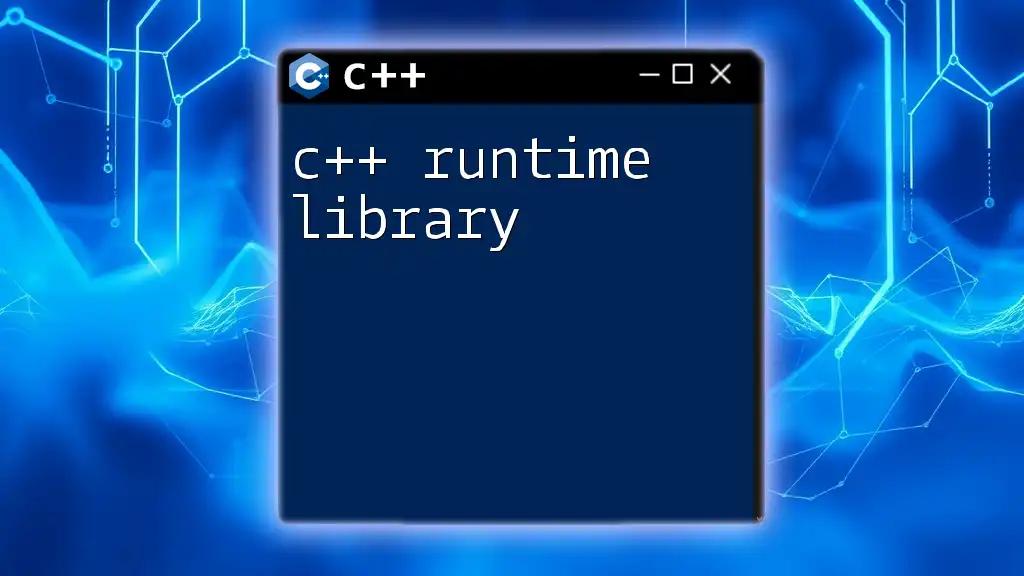
Debugging and Testing Your C++ Application
Debugging Tools Available
Debugging is crucial in the development process. On the Steam Deck, you can utilize tools like GDB (GNU Debugger) or IDE features in Visual Studio Code. GDB allows you to set breakpoints, inspect variables, and step through your code to identify issues.
Testing on the Steam Deck
Testing is an integral part of ensuring your application runs smoothly. Best practices include:
- Testing on actual hardware to mimic real user experiences.
- Using profiling tools to assess performance and resource utilization. Performance testing can help you identify how efficiently your application runs on the Steam Deck, which is critical for a gaming device.

Utilizing the Steam API with C++
Introduction to Steamworks SDK
The Steamworks SDK is a robust platform that gives developers access to features like Achievements, Multiplayer, and Cloud Saves. Understanding this SDK opens up tremendous opportunities for enhancing your game.
Integrating Steam API in Your C++ Project
To utilize the Steam API, follow the integration steps carefully. First, download the SDK from the [Steamworks website](https://partner.steamgames.com/home). Next, configure your project settings to link the Steamworks libraries.
Here is a simple code snippet demonstrating how to initialize the Steam API:
#include <steam/steam_api.h>
void InitializeSteam() {
if (SteamAPI_Init()) {
std::cout << "Steam API Initialized!" << std::endl;
} else {
std::cerr << "Failed to initialize Steam API!" << std::endl;
}
}
This code checks if the Steam API initializes correctly and provides feedback.

Performance Optimization Techniques
Key Optimization Strategies
Optimizing your C++ code can significantly enhance the performance of your application. Key strategies include:
- Memory Management: Make efficient use of memory through proper allocations and deallocations. Avoid memory leaks by utilizing smart pointers.
- Algorithm Efficiency: Opt for algorithms and data structures that reduce computational complexity to keep the game running smoothly.
Profiling Your Application
Profiling is essential for identifying performance bottlenecks in your application. Tools like Valgrind or gprof can provide insightful data on where your program spends the most time and resources. After running a profiler, you may receive output indicating which functions are consuming the most CPU time, aiding in further optimization.
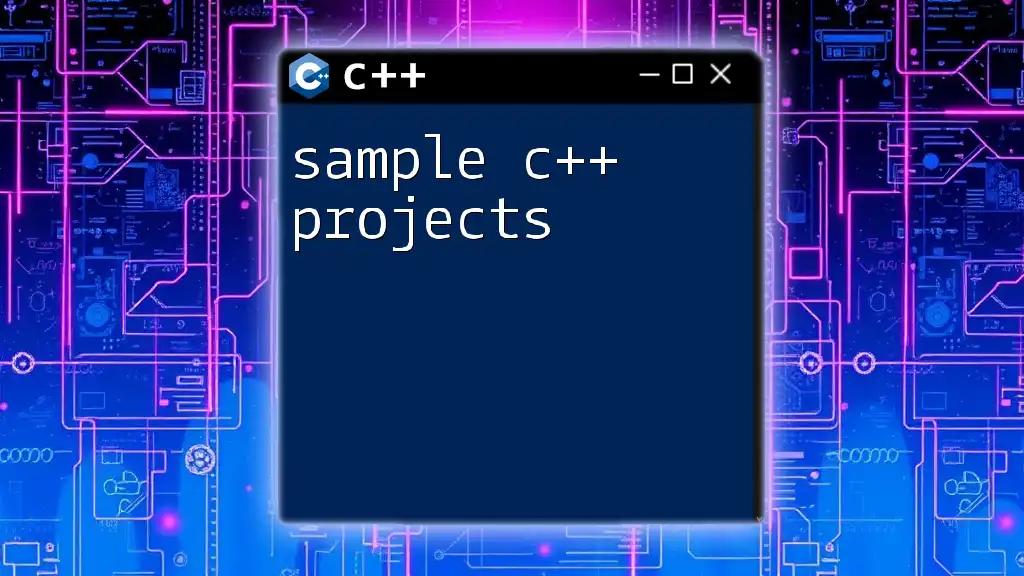
Common Challenges and Solutions
Troubleshooting C++ Runtime Issues
Developers often encounter a variety of runtime issues when developing for the Steam Deck. Common problems include library version mismatches and linker errors. Solutions typically involve ensuring that the correct versions of libraries are installed and linked properly in your project configuration.
Community Resources and Support
Engaging with the community can significantly boost your learning curve. Numerous forums and platforms are dedicated to Steam Deck development. Take advantage of Stack Overflow, GitHub discussions, and dedicated Steamworks forums to seek guidance and share your experiences.
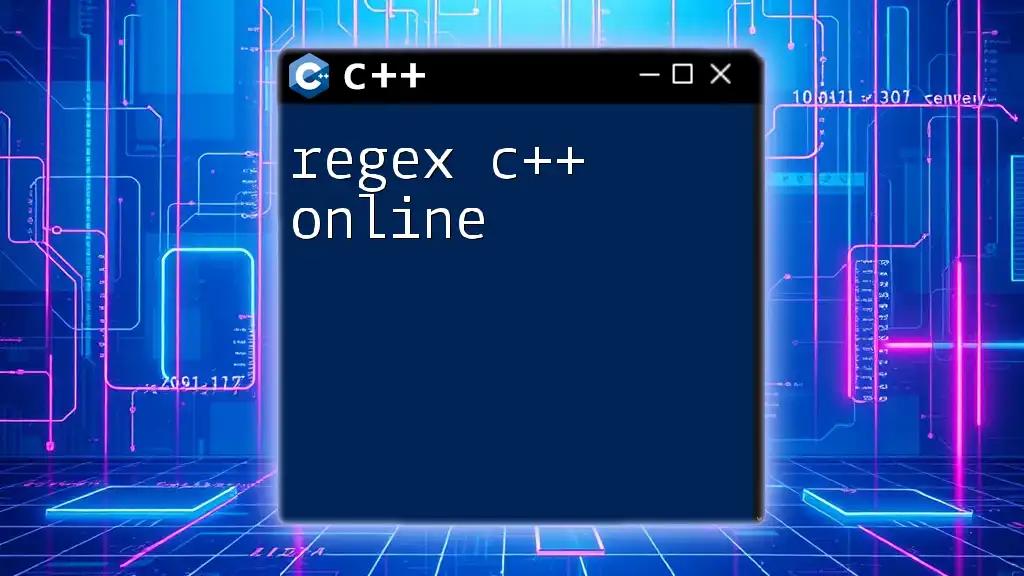
Conclusion
In conclusion, mastering the Steam Deck C++ runtime empowers developers to create optimized gaming experiences on this innovative platform. With a solid understanding of setup, coding, debugging, and API integration, you can explore the exciting possibilities that await. Don't hesitate to dive into development and join the vibrant community surrounding the Steam Deck. This is just the beginning—continue learning, experimenting, and crafting amazing games for players around the world!

Additional Resources
For further exploration, refer to the official [Steamworks documentation](https://partner.steamgames.com/doc/home), and explore C++ community resources for tutorials, libraries, and best practices in game development.