Here's a clear explanation and a code snippet using `fstream` in C++:
The `fstream` library allows you to create, read, and write to files in C++, as demonstrated in the following example that writes "Hello, World!" to a text file.
#include <fstream>
int main() {
std::ofstream outfile("example.txt");
outfile << "Hello, World!" << std::endl;
outfile.close();
return 0;
}
Understanding fstream in C++
fstream, short for file stream, is a powerful feature in C++ that facilitates file handling by providing a way to perform input and output operations on files. With fstream, programmers can read from and write to files, which is essential for data persistence.
Types of File Streams
C++ provides three main types of file streams:
-
ifstream (Input File Stream): This stream is specifically used for reading data from files. It’s useful when you only need to access data and not modify it.
std::ifstream inFile("input.txt");
-
ofstream (Output File Stream): This stream is meant for writing data to files. If you need to create or modify a file, ofstream is the go-to option.
std::ofstream outFile("output.txt");
-
fstream (Bidirectional File Stream): This versatile stream allows both reading and writing operations on the same file. This is particularly useful when you need to manipulate data within a file dynamically.
std::fstream myFile("testfile.txt", std::ios::in | std::ios::out);

Setting Up Your Development Environment
Before diving into coding, ensure that you have the right tools installed for developing C++ applications.
- Install a C++ Compiler: Popular options include GCC and Clang, which can be installed on various platforms.
- Choose an IDE: While you can write C++ code in any text editor, using an IDE like Visual Studio, Code::Blocks, or CLion can significantly enhance your productivity by providing features like debugging tools and code completion.
- Create Your Project: Once your environment is set up, create a new project to experiment with file handling examples.
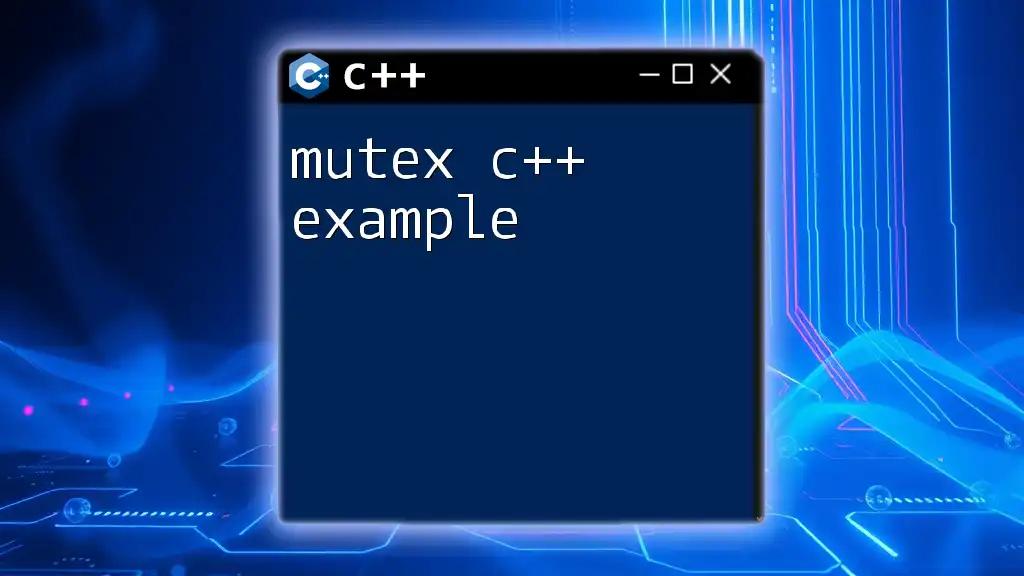
Basic Syntax of fstream
When working with fstream, understanding the syntax for opening and closing files is vital. The following are key methods and operations:
-
Opening a File: Use the `open()` function to open a file for reading or writing.
myFile.open("example.txt", std::ios::out | std::ios::in | std::ios::trunc);
-
Closing a File: Always remember to close the file using the `close()` method to release resources.
-
Checking File Status: Before performing any operations, ensure the file opened successfully using `is_open()`.
Here's an example demonstrating these concepts:
#include <fstream>
#include <iostream>
int main() {
std::fstream myFile;
myFile.open("example.txt", std::ios::out | std::ios::in | std::ios::trunc);
if (myFile.is_open()) {
std::cout << "File successfully opened." << std::endl;
}
myFile.close();
return 0;
}
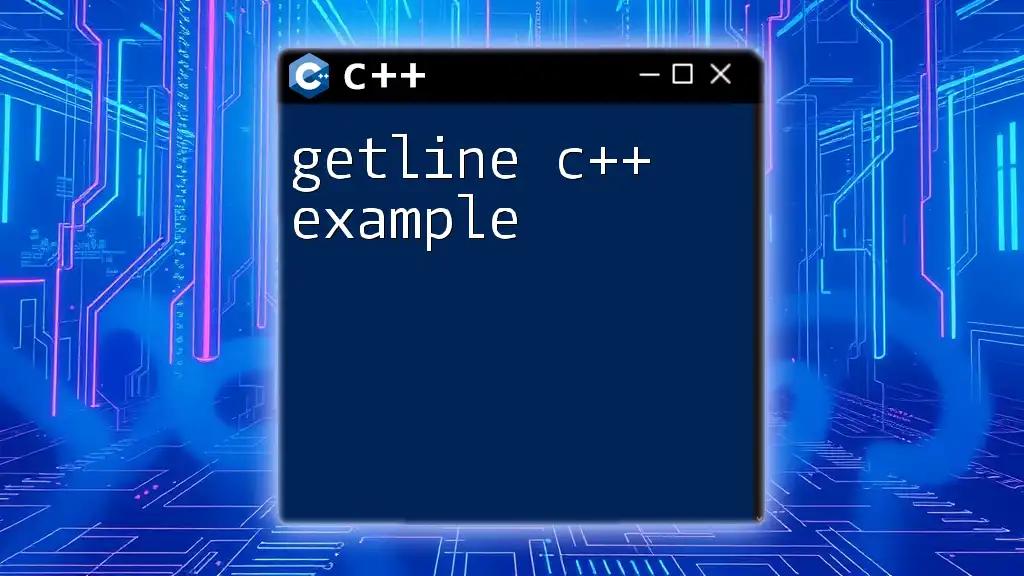
Reading from a File using ifstream
To read data from a file in C++, the ifstream class is utilized. Inside a program, it's essential to handle the data efficiently.
Syntax for Reading Data
You can read data using the extraction operator (`>>`) or by using the `getline()` function for complete lines.
Here's an example that demonstrates reading data from a file:
#include <fstream>
#include <iostream>
#include <string>
int main() {
std::ifstream inFile("input.txt");
std::string line;
if (inFile.is_open()) {
while (getline(inFile, line)) {
std::cout << line << std::endl;
}
inFile.close();
} else {
std::cout << "Unable to open file." << std::endl;
}
return 0;
}
This snippet reads each line from `input.txt` and prints it to the console. Be sure to implement error checks to manage any potential issues when opening the file.
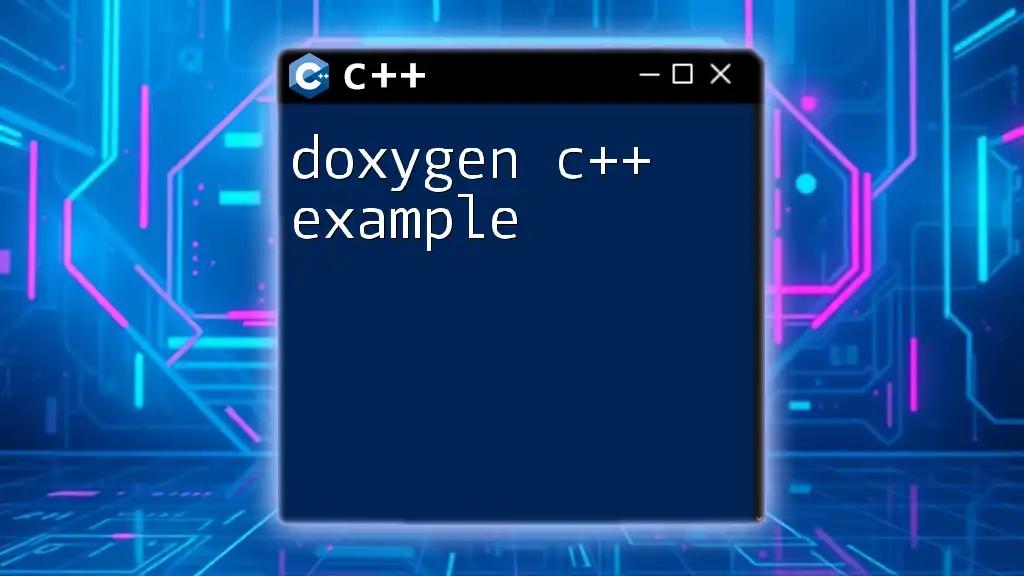
Writing to a File using ofstream
When you need to create or modify a file, ofstream comes into play. It's designed to write output to files effectively.
Syntax for Writing Data
Data can be written using the insertion operator (`<<`), making it straightforward to output text:
Here's a concise example of how to use ofstream:
#include <fstream>
#include <iostream>
int main() {
std::ofstream outFile("output.txt");
if (outFile.is_open()) {
outFile << "Hello, World!" << std::endl;
outFile << "This is a test file." << std::endl;
outFile.close();
} else {
std::cout << "Unable to open file." << std::endl;
}
return 0;
}
This code snippet opens (or creates) `output.txt` and writes two lines of text. Always check if the file is open before attempting to write to avoid errors.
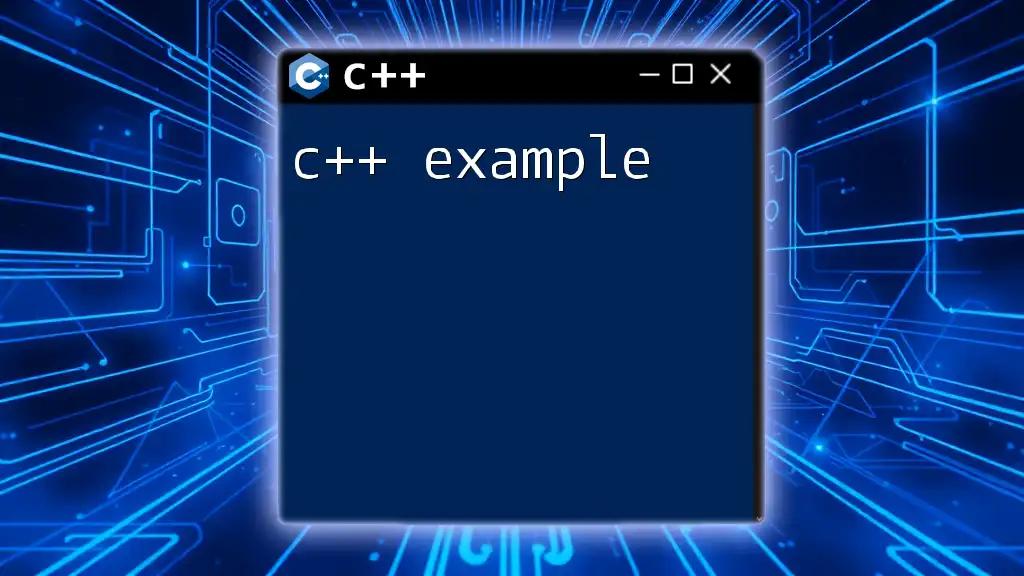
Using fstream for Bidirectional File Operations
One of the significant advantages of using fstream is its capability to handle both reading and writing in a single file. This flexibility enhances the usability of file streams in applications that require frequent updates.
Advantages of Using fstream
Utilizing fstream simplifies workflows where reading and writing occur repeatedly. For instance, when creating logs or storing states within applications, combining both operations can save time and resources.
Here's an example of using fstream for both reading and writing:
#include <fstream>
#include <iostream>
#include <string>
int main() {
std::fstream file("testfile.txt", std::ios::in | std::ios::out | std::ios::app);
if (file.is_open()) {
file << "Adding a new line to the file." << std::endl;
file.seekg(0); // Move to the beginning for reading
std::string line;
while (getline(file, line)) {
std::cout << line << std::endl;
}
file.close();
} else {
std::cout << "Unable to open file." << std::endl;
}
return 0;
}
In this code, we append a line to `testfile.txt` and then read all its contents. Note the use of `seekg()` to reset the position to the beginning of the file before reading.
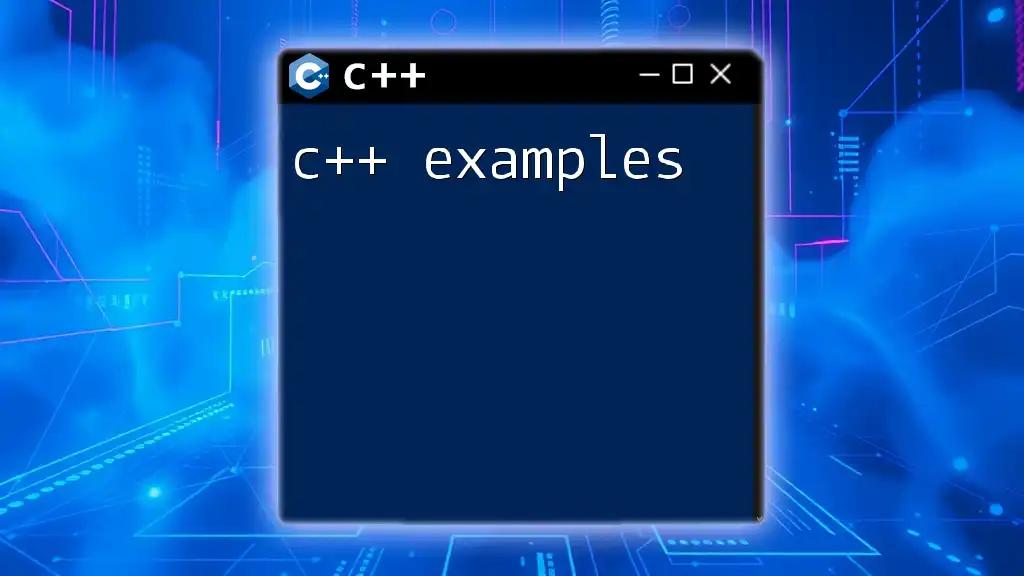
Error Handling in File Operations
Error handling in file operations is crucial for building robust C++ applications. Managing file state can prevent crashes and unexpected behavior.
Check the status of file streams using the following function calls:
- fail(): Indicates whether an input/output operation failed.
- eof(): Checks if the end of the file has been reached.
Here is an example showing how to enhance error-handling mechanisms:
if (!inFile) {
std::cerr << "Error opening file!" << std::endl;
}
Adding such checks ensures that the program gracefully handles issues rather than crashing unexpectedly.

Conclusion
In conclusion, understanding fstream and how to work with file I/O in C++ is essential for any programmer dealing with data storage and retrieval. Through this guide, key concepts such as reading from files, writing to files, utilizing bidirectional operations, and implementing error handling have been covered, equipping you with the knowledge to handle file operations effectively.
As you grow more comfortable with fstream, consider experimenting with more complex file operations, like binary file handling or utilizing file buffers for enhanced performance. Always remember, practice is key to mastering C++ file handling techniques, so dive in and explore this powerful functionality!
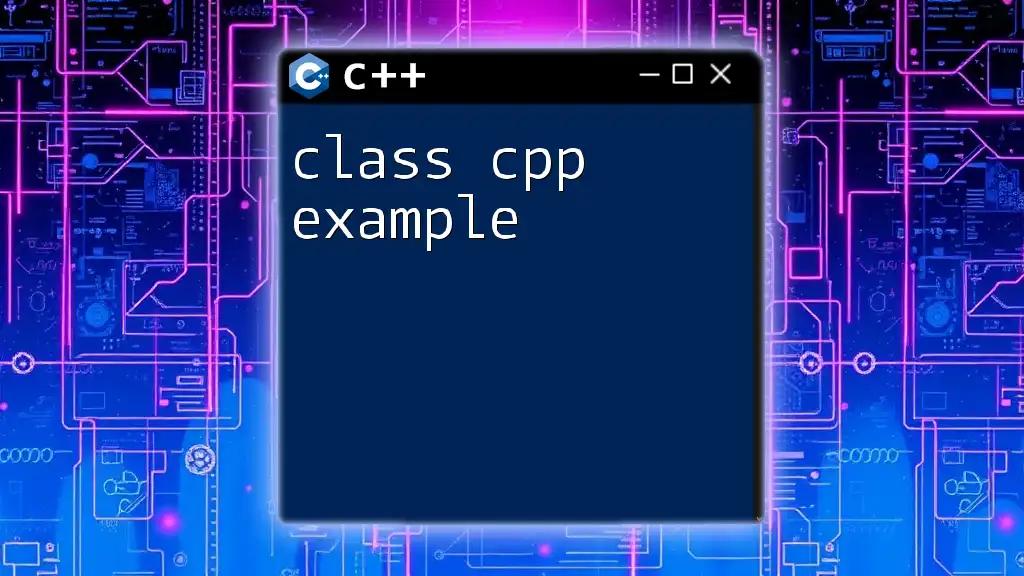
Additional Resources
To further cement your understanding, consider exploring the official C++ documentation, engaging with online courses, and reading specialized books on C++. Each of these resources can enhance your proficiency in working with fstream and other C++ features to develop sophisticated applications.