In C++, the `ofstream` class is used to create and write to files, allowing you to store output data in a specified file format.
#include <fstream>
#include <iostream>
int main() {
std::ofstream outFile("example.txt");
if (outFile.is_open()) {
outFile << "Hello, World!" << std::endl;
outFile.close();
} else {
std::cerr << "Unable to open file" << std::endl;
}
return 0;
}
What is ofstream?
`ofstream` stands for output file stream. This C++ class is primarily used to write data to files. It streamlines the process of handling file outputs, making it a fundamental part of file management in C++. Understanding how to work with `ofstream` is crucial for any C++ developer, as it allows for persistent data storage, making applications more robust and effective.
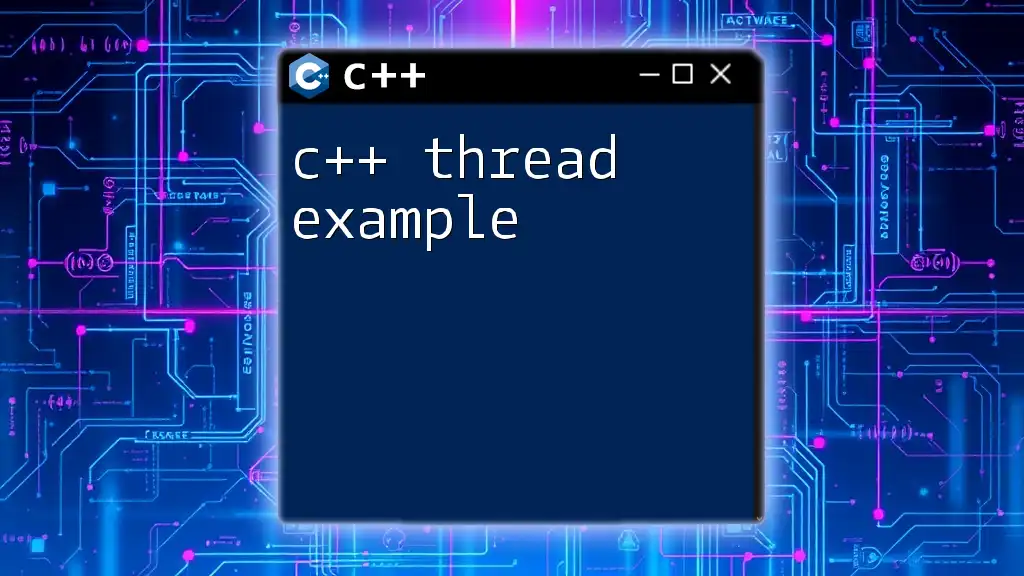
Understanding File Streams
In C++, file streams form part of the I/O (input/output) operations, which involve reading and writing data. The primary types of streams are:
- Input streams: Used for reading data from files.
- Output streams: Used for writing data to files. `ofstream` falls under this category.
By using streams in C++, you abstract the complexity of file handling, allowing developers to focus on data processing rather than file operations.
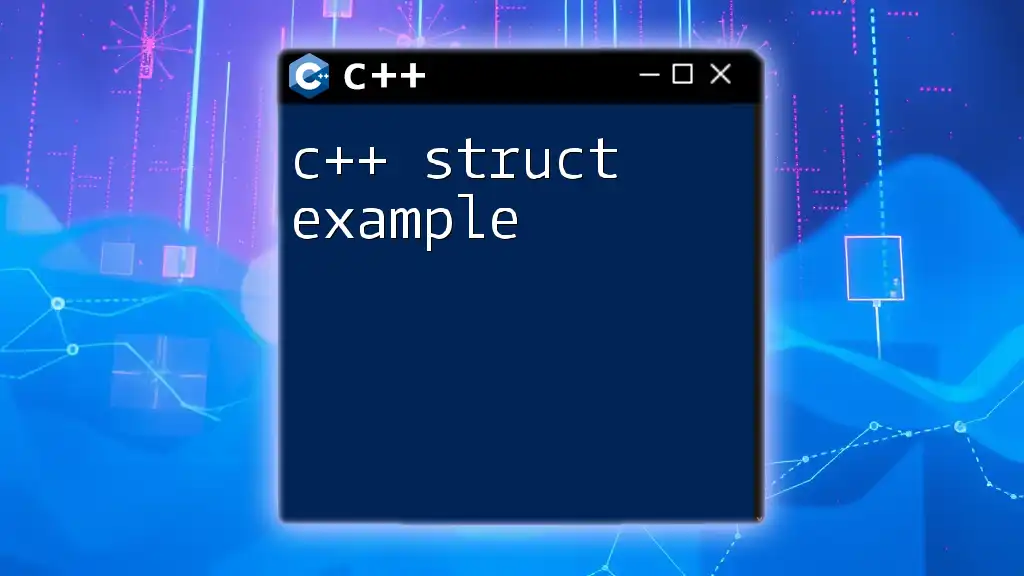
Setting Up Your Environment
Tools and Compilers Needed
To start programming in C++, you’ll need a reliable environment. Popular Integrated Development Environments (IDEs) include:
- Visual Studio
- Code::Blocks
- Eclipse CDT
- CLion
These tools come with built-in support for compiling and debugging C++ code, ensuring a smooth development process.
Creating Your First C++ Program
Once you've chosen your IDE, create a new C++ project. As a first step, consider writing a simple "Hello, World!" program to verify that everything is set up correctly:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Executing this code successfully confirms your environment is ready for C++ development.
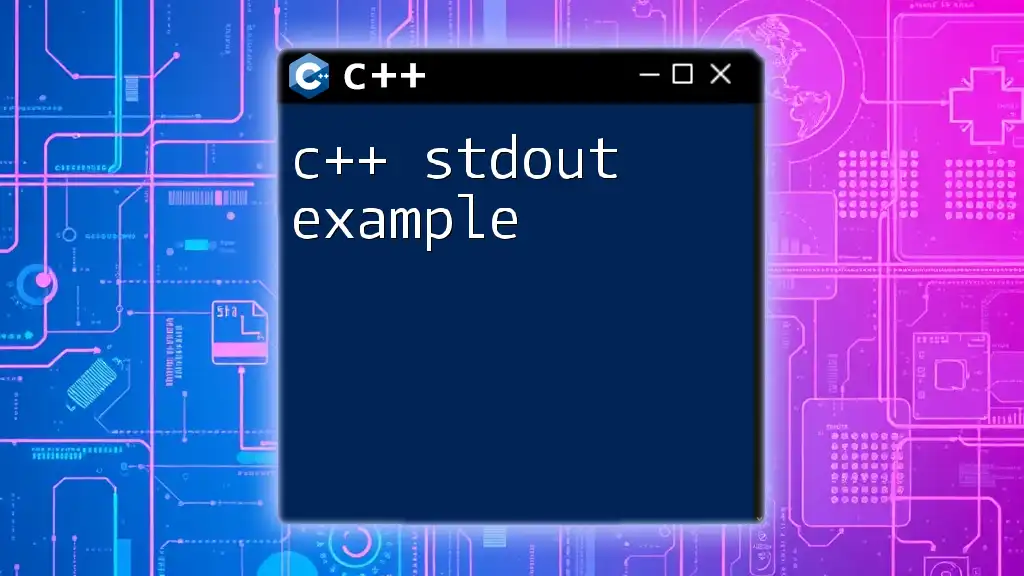
Using ofstream: Basic Concepts
Initializing ofstream
To utilize `ofstream`, you need to create an instance of the class, associating it with a file you wish to write to. The creation can be done in multiple ways:
- Using the constructor directly:
std::ofstream outputFile("example.txt");
- Using the `open` method:
std::ofstream outputFile;
outputFile.open("example.txt");
In both cases, if the file "example.txt" does not exist, it will be created. However, if the file exists, it will be overwritten by default.
Code Snippet: Basic ofstream Initialization
#include <iostream>
#include <fstream>
int main() {
std::ofstream outputFile("example.txt");
if(outputFile.is_open()) {
outputFile << "Hello, World!";
outputFile.close();
}
return 0;
}
Explanation of the Code Snippet
- The first line includes the necessary libraries (`<iostream>` for input and output and `<fstream>` for file operations).
- We create an `ofstream` object named `outputFile` and associate it with "example.txt".
- The `is_open()` method checks if the file was successfully opened. If true, we write "Hello, World!" to the file and close the file afterward.
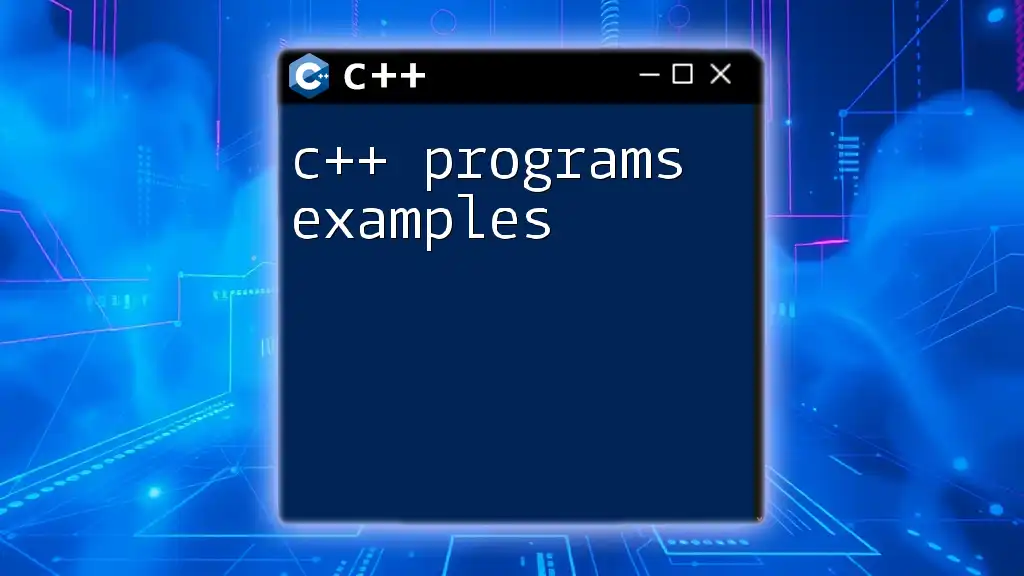
Writing Data to Files
Appending versus Overwriting
By default, `ofstream` will overwrite any existing content in the file. If you want to add new content without losing the current data, you should open the file in append mode using `std::ios::app`. This adds new data at the end of the file rather than at the beginning.
Code Snippet: Writing and Appending Data
#include <fstream>
int main() {
std::ofstream outputFile("example.txt", std::ios::app);
if (outputFile.is_open()) {
outputFile << "\nAppending this line.";
outputFile.close();
}
return 0;
}
Detailed Explanation of Modes
In this example, the addition of `std::ios::app` opens "example.txt" for appending. Therefore, any new output is added below the existing content, preserving what's already written. Understanding the mode in which a file is opened is crucial to avoid data loss.
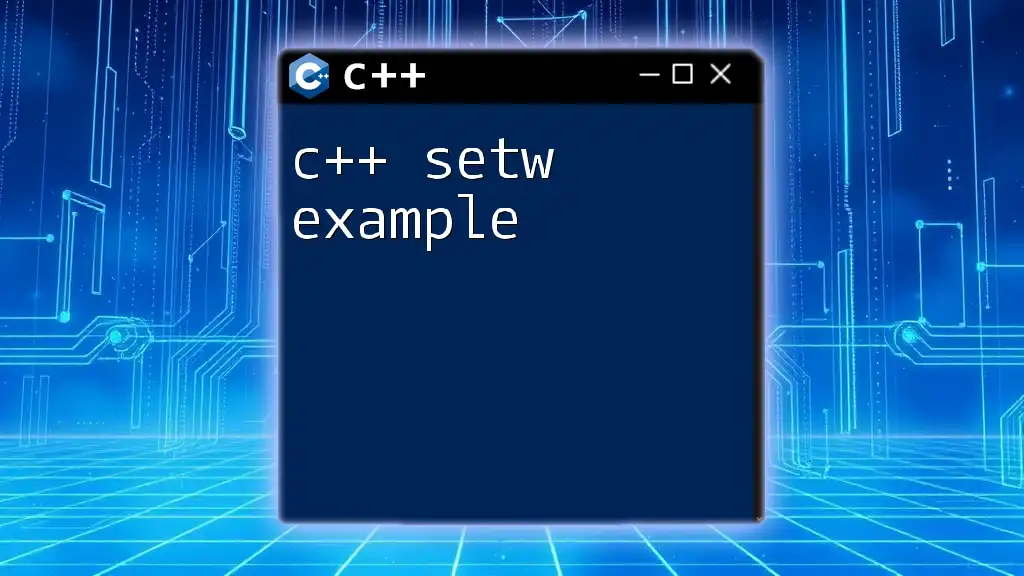
Error Handling with ofstream
Checking if the File Opened Successfully
When working with files, it's essential to verify that the file opened correctly. This can be done using the `is_open()` method:
if (outputFile.is_open()) {
// Write operations here
}
If you attempt to write to a file that failed to open (for instance, due to permissions issues), your program might crash or produce unexpected results.
Handling Errors Gracefully
It's good practice to handle errors adequately. For example, if you try to open a file that doesn’t exist or cannot be created, logging the error provides valuable feedback.
Code Snippet: Error Handling Example
#include <iostream>
#include <fstream>
int main() {
std::ofstream outputFile("non_existent_directory/example.txt");
if (!outputFile) {
std::cerr << "Error opening file!" << std::endl;
}
return 0;
}
Analysis of the Error Handling Approach
In this snippet, if the file cannot be opened, an error message is printed. This simple check can save you significant troubleshooting time, as you'll have a clear indication of what went wrong instead of a silent failure.
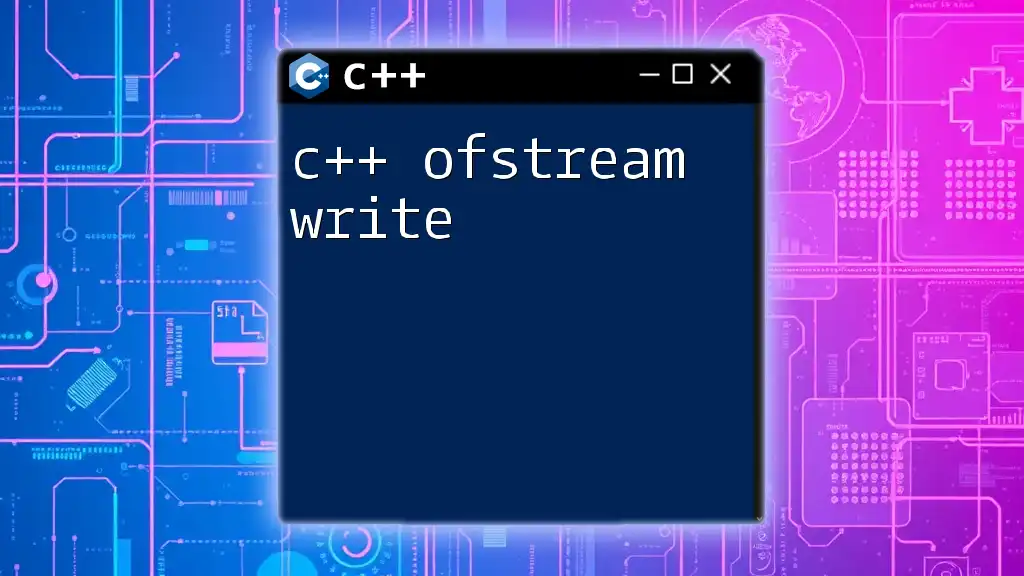
Closing ofstream Objects
Why is Closing Important?
Properly closing file streams is vital. It ensures that all data is flushed from memory to disk, and also releases system resources. Neglecting to close a file can lead to data corruption or memory leaks.
Best Practices for Closing Files
Always close your files after you are done writing to them. This can be done explicitly using the `close()` method or implicitly when the `ofstream` object goes out of scope.
Code Snippet: Properly Closing ofstream
#include <fstream>
int main() {
std::ofstream outputFile("example.txt");
// write operations
outputFile.close(); // Closing explicitly
return 0;
}
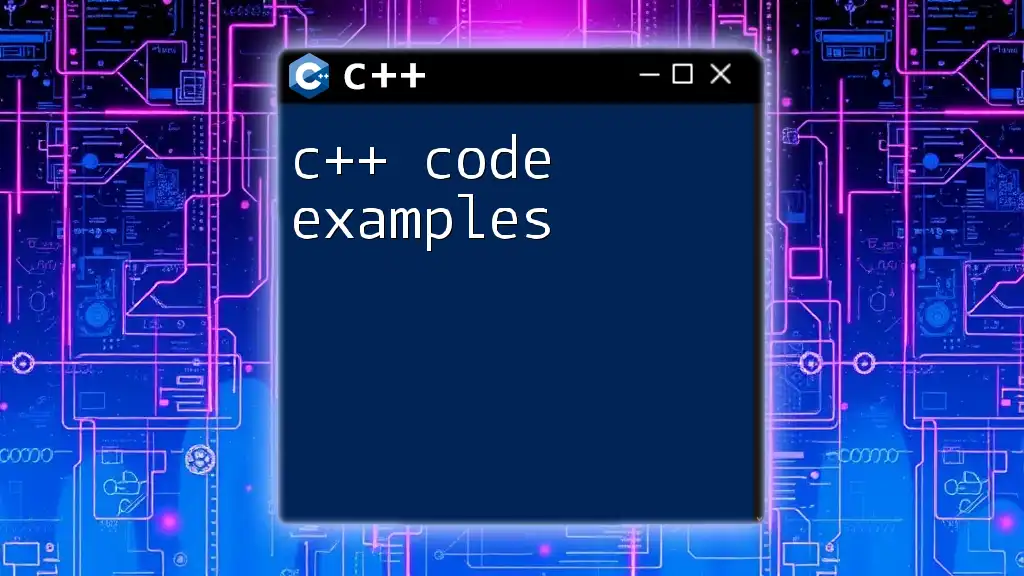
Advanced ofstream Techniques
Writing Different Data Types
One of the advantages of `ofstream` is its versatility when it comes to data types. You can write integers, floats, strings, and other types without extra modifications.
Code Snippet: Writing Various Data Types
#include <fstream>
int main() {
std::ofstream outputFile("data.txt");
int age = 30;
float height = 5.9;
std::string name = "John Doe";
outputFile << "Name: " << name << "\nAge: " << age << "\nHeight: " << height;
outputFile.close();
return 0;
}
Understanding Formatting and Manipulation
In this example, different data types are written to the "data.txt" file seamlessly. C++ allows you to manipulate formatting using manipulators like `std::fixed` and `std::setprecision`, which are particularly useful when dealing with floating-point numbers to achieve specific formatting in the output.
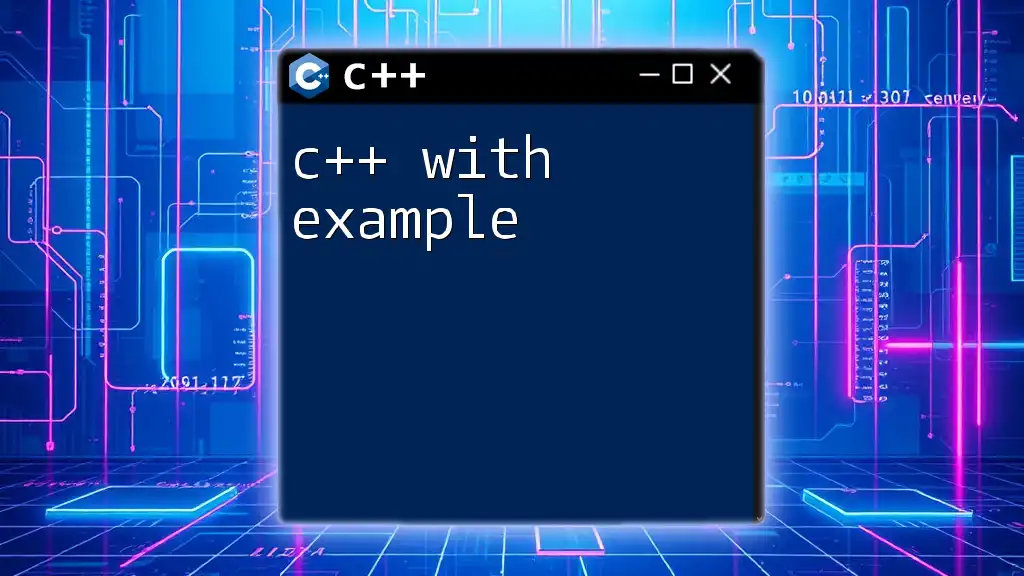
Conclusion
Recapping the key points, `ofstream` is an essential component of file handling in C++. We covered its initialization, writing, error handling, and advanced techniques. It's critical to ensure you understand how to open, write to, and close your files properly to prevent data loss and manage resources effectively.
As you practice, let your creativity flow and explore more complex structures, such as writing arrays or objects to files. Continuous experimentation will sharpen your understanding of file I/O in C++.
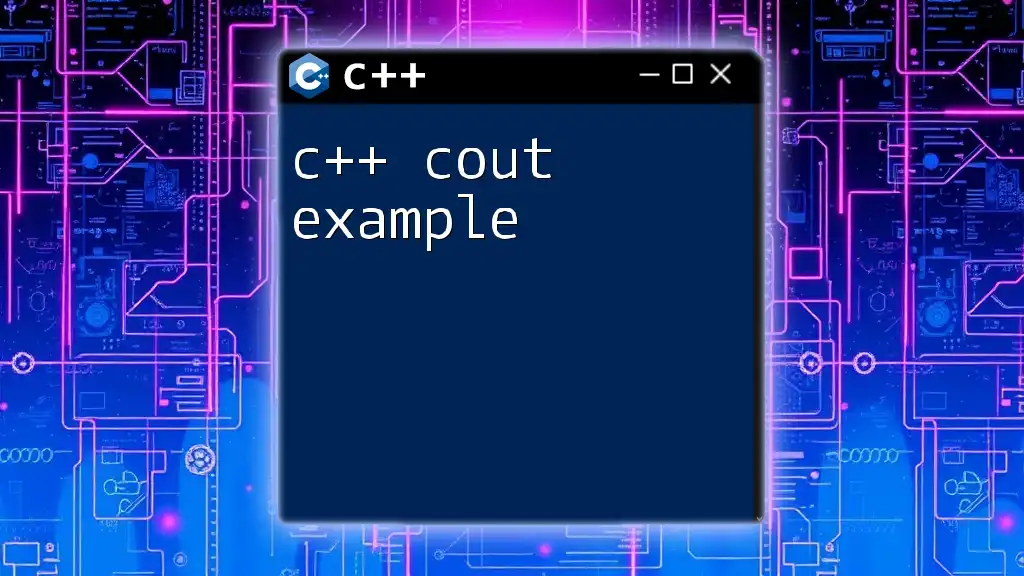
FAQs about C++ ofstream
-
What happens if I forget to close the ofstream?
Failing to close an `ofstream` can lead to memory leaks and data corruption, as not all data is guaranteed to be written to the file. -
Can I use ofstream with binary files?
Yes, you can use `ofstream` to handle binary files by opening the file with `std::ios::binary`. -
What are the differences between ofstream and fstream?
`ofstream` is designed for output operations only, while `fstream` can handle both input and output. Use `fstream` when you need to read from and write to the same file.
This comprehensive guide on `c++ ofstream example` equips you with the basic tools and understanding needed for effective file handling in your C++ projects.