The `ifstream getline` function in C++ is used to read a line of text from a file into a string variable, allowing for efficient file input handling.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("example.txt");
std::string line;
if (file.is_open()) {
while (getline(file, line)) {
std::cout << line << std::endl;
}
file.close();
}
return 0;
}
Understanding ifstream in C++
What is ifstream?
The `ifstream` class in C++ stands for input file stream. It is a part of the C++ Standard Library and is used to read data from files. ifstream allows users to handle input operations effectively, enabling them to open a file, read its contents, and manage various types of data seamlessly.
The primary purpose of using `ifstream` is to facilitate reading data from external files. It provides various member functions to interact with the file system, including the ability to check if the file is opened successfully, read data, and handle the end-of-file (EOF) condition.
How to Use ifstream
To use `ifstream`, you need to include the `<fstream>` header file. The basic syntax for creating an `ifstream` object is as follows:
std::ifstream ifs("filename.txt");
In this statement, `"filename.txt"` should be replaced with the actual file name you wish to open. If your file is located in a different directory, ensure you specify the relative or absolute path correctly.
Here's a simple example of opening a file with `ifstream` and checking if it opened correctly:
#include <iostream>
#include <fstream>
int main() {
std::ifstream file("example.txt");
if (!file.is_open()) {
std::cerr << "Error: Could not open the file!" << std::endl;
return 1; // Return an error code if the file cannot be opened
}
// You can proceed with reading from the file here
file.close();
return 0;
}
This code provides a basic understanding of `ifstream` and how to ensure that your file operations can proceed only when the file is successfully opened.
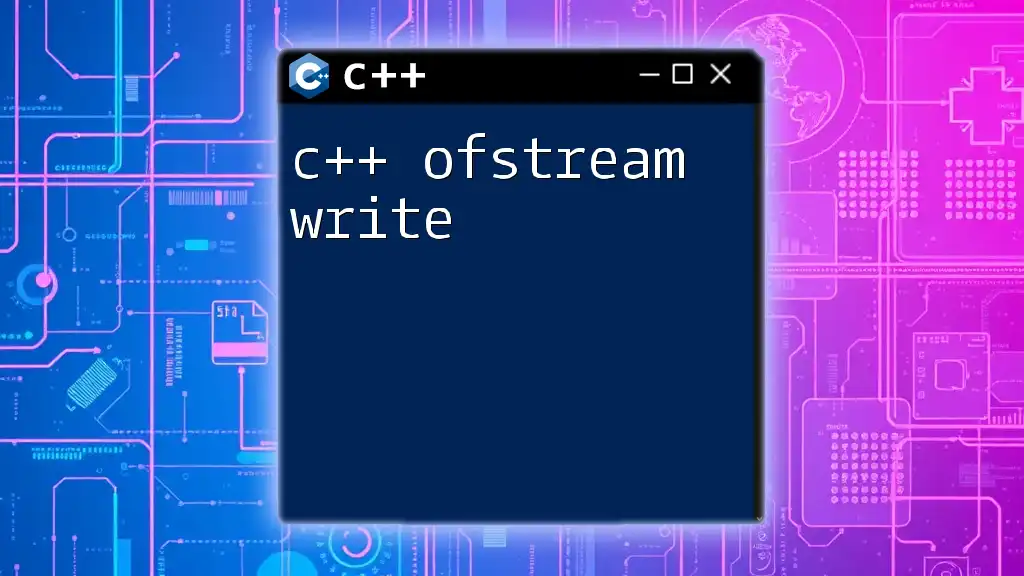
The getline Function in C++
What is getline?
The `getline` function is used in conjunction with streams — including `ifstream` — to read an entire line from a file. It is particularly helpful as it allows for capturing input that contains spaces, which is often not possible with standard input methods.
Syntax of getline
The syntax for the `getline` function is quite straightforward:
std::getline(input_stream, string_variable, delimiter);
- input_stream: This is the `ifstream` object from which we want to read data.
- string_variable: This variable will store the line read from the file.
- delimiter: This is an optional parameter, which defines the character that marks the end of the line. If not specified, the default is a newline character (`\n`).
How to Use getline with ifstream
Basic Example
Using `getline` with `ifstream` allows you to read lines from a file efficiently. Below is an example that reads lines from a file and prints them:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("example.txt");
std::string line;
if (file.is_open()) {
while (std::getline(file, line)) {
std::cout << line << std::endl;
}
file.close();
} else {
std::cerr << "Error: Could not open file." << std::endl;
}
return 0;
}
In this example, we open "example.txt", read it line by line using `getline`, and print each line to the console. The loop continues until `getline` returns `false`, indicating that the end of the file has been reached.
Handling Different Data Types
When reading data from a file, you may encounter cases where you need to convert the input to different data types. After using `getline`, you can convert the string to other types with the help of `std::stoi` or `std::stof` functions:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("numbers.txt");
std::string line;
if (file.is_open()) {
while (std::getline(file, line)) {
int number = std::stoi(line); // Convert string to integer
std::cout << "Number: " << number << std::endl;
}
file.close();
} else {
std::cerr << "Error: Could not open file." << std::endl;
}
return 0;
}
In this example, lines representing integer values are read from "numbers.txt" and converted to integers using `std::stoi`, enabling further processing as needed.
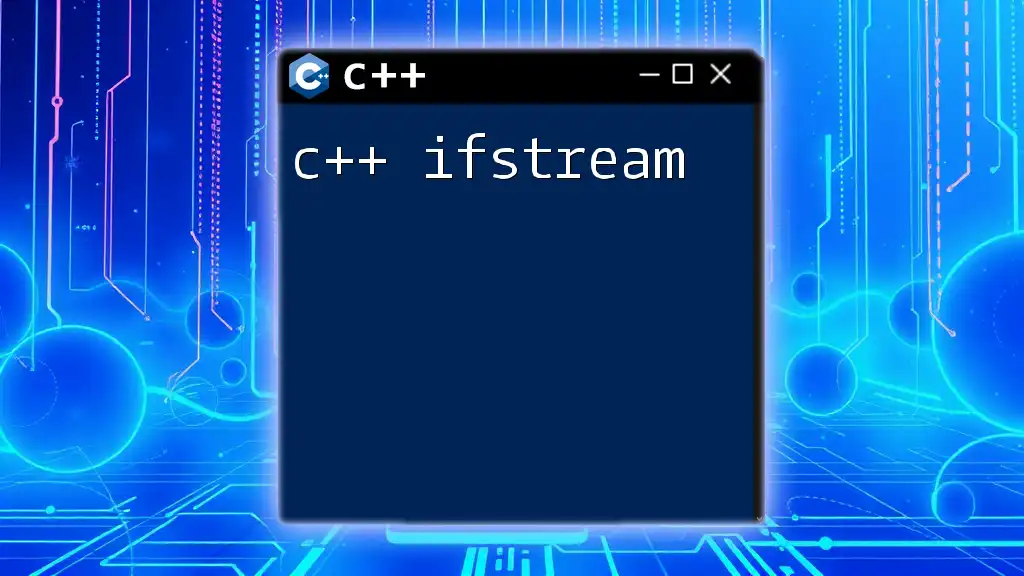
Advantages of Using getline in ifstream
Handling Large Data
One of the primary advantages of using `getline` is its ability to handle large data files efficiently. Instead of loading the entire file into memory, `getline` reads it line by line. This not only improves memory efficiency but also enhances performance, especially when dealing with very large files.
Reading Special Characters and Spaces
The `getline` function excels at reading lines with spaces and special characters. Unlike `operator>>`, which stops at whitespace, `getline` captures everything up to the specified delimiter:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("example_with_spaces.txt");
std::string line;
if (file.is_open()) {
while (std::getline(file, line)) {
std::cout << "Read: " << line << std::endl;
}
file.close();
} else {
std::cerr << "Error: Could not open file." << std::endl;
}
return 0;
}
In this code, even if lines contain spaces, `getline` captures them fully, allowing you to retain the original formatting of the data.
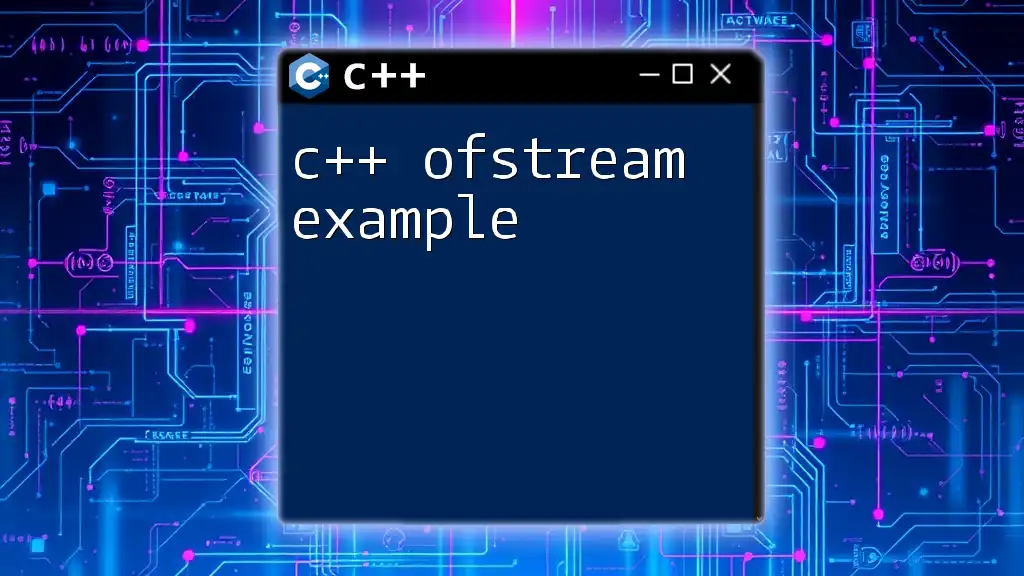
Error Handling with ifstream and getline
Common Errors and Solutions
Using `ifstream` and `getline` may lead to several common errors. For example, attempting to read from a file that does not exist will result in an error. Always check if the file is opened successfully before reading:
if (!file.is_open()) {
std::cerr << "Error: Could not open the file!" << std::endl;
}
Incorporating error checks ensures that your program does not proceed with invalid file operations, which could lead to crashes or undefined behavior.
Best Practices
To make the best use of `ifstream` and `getline`, consider the following best practices:
- Ensure file paths are correct: Always check your file paths for accuracy to avoid file-not-found errors.
- Check for end-of-file: Utilize appropriate checks after reading to handle cases where the end of the file is reached.
- Close your files: Always close files when done with them to free up resources, as shown in previous examples.
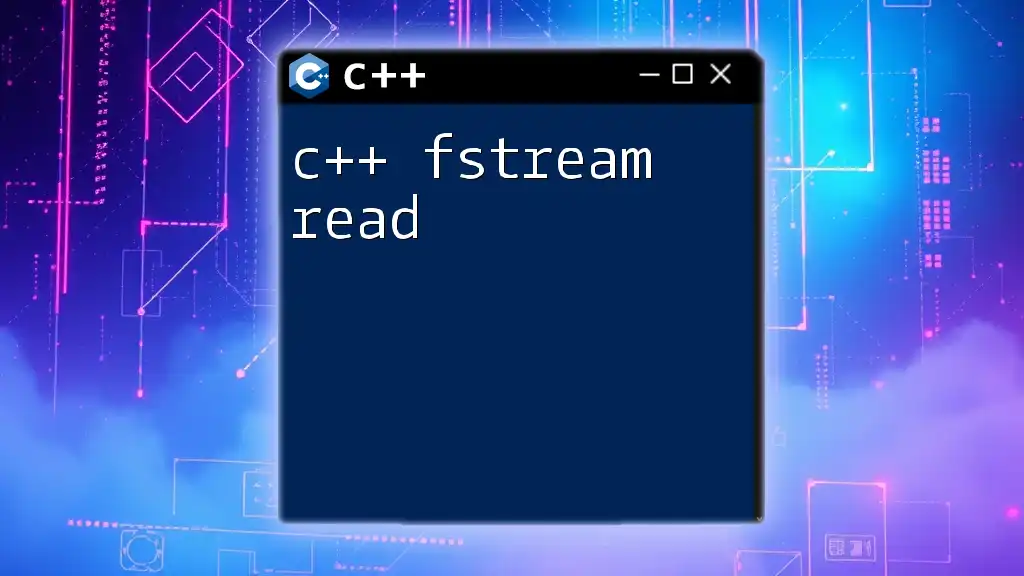
Conclusion
In conclusion, combining `ifstream` with `getline` provides a powerful mechanism for file handling in C++. Whether you are reading text files, processing large datasets, or extracting data types from text, mastering these tools will enhance your programming skills significantly. By understanding the syntax, error handling, and best practices, you empower yourself to handle files effectively in your C++ applications.
As you continue to explore the depths of file handling in C++, don’t hesitate to practice regularly and consult additional resources for a more robust understanding of these concepts.