The `cin.getline` function in C++ is used to read a line of text from the standard input (cin), including spaces, until a specified delimiter is encountered or a maximum number of characters is reached.
Here’s a simple example:
#include <iostream>
using namespace std;
int main() {
char buffer[100];
cout << "Enter a line of text: ";
cin.getline(buffer, 100); // Reads up to 99 characters or until newline
cout << "You entered: " << buffer << endl;
return 0;
}
Understanding `cin` in C++
In C++, `cin` is an instance of the `istream` class that is used to handle standard input. This allows programmers to read input from the user via the keyboard. The basic usage of `cin` involves simple data types like integers, floats, and strings. For example:
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
cin >> age;
cout << "You are " << age << " years old." << endl;
return 0;
}
In this example, the user is prompted to enter their age, which is stored as an integer. However, while `cin` works well for basic input scenarios, it has limitations, especially when handling strings that contain spaces.
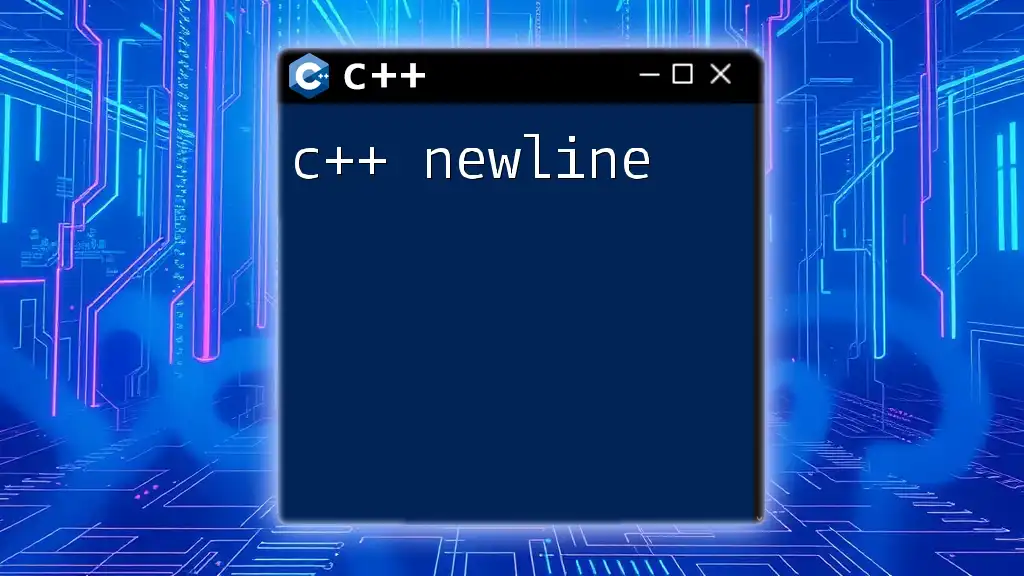
What is `cin.getline`?
`cin.getline` is a specialized function designed to read an entire line of text, including spaces, until a newline character is encountered or the specified limit is reached. Its signature looks like this:
cin.getline(char* buffer, streamsize size);
Here, buffer is the character array where the input is stored, and size is the maximum number of characters to read, including the null terminator. This function is particularly useful when dealing with strings that may have spaces, such as full names or addresses.
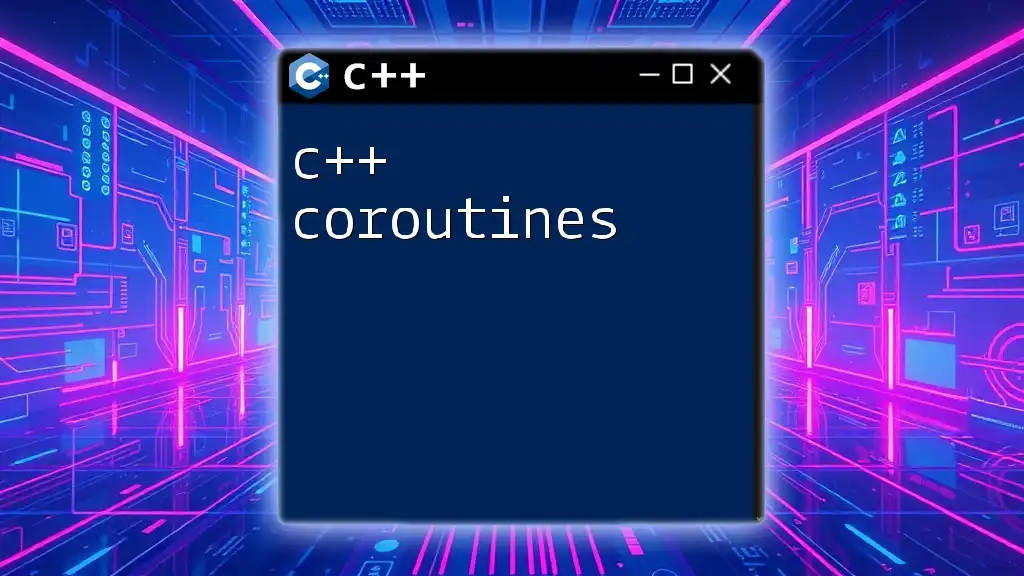
Using `cin.getline` in C++
Basic Syntax and Example
The basic syntax of `cin.getline` is straightforward. Here’s an example that demonstrates how to use it effectively:
#include <iostream>
using namespace std;
int main() {
char name[100];
cout << "Enter your full name: ";
cin.getline(name, 100);
cout << "Hello, " << name << "!" << endl;
return 0;
}
In this example, `cin.getline` reads the entire line that the user inputs into the `name` buffer, allowing for first names, middle names, and last names.
Key Parameters of `cin.getline`
-
Character Array (Buffer): The character array is crucial because it serves as storage for the string that will be read. Always ensure proper sizing; too small a buffer can lead to input truncation, while too large a buffer can waste memory.
-
Streamsize: This parameter controls how many characters to read. If the input exceeds this limit, `cin.getline` will stop reading and leave the excess characters in the input buffer, which could lead to unexpected behavior in subsequent input operations.
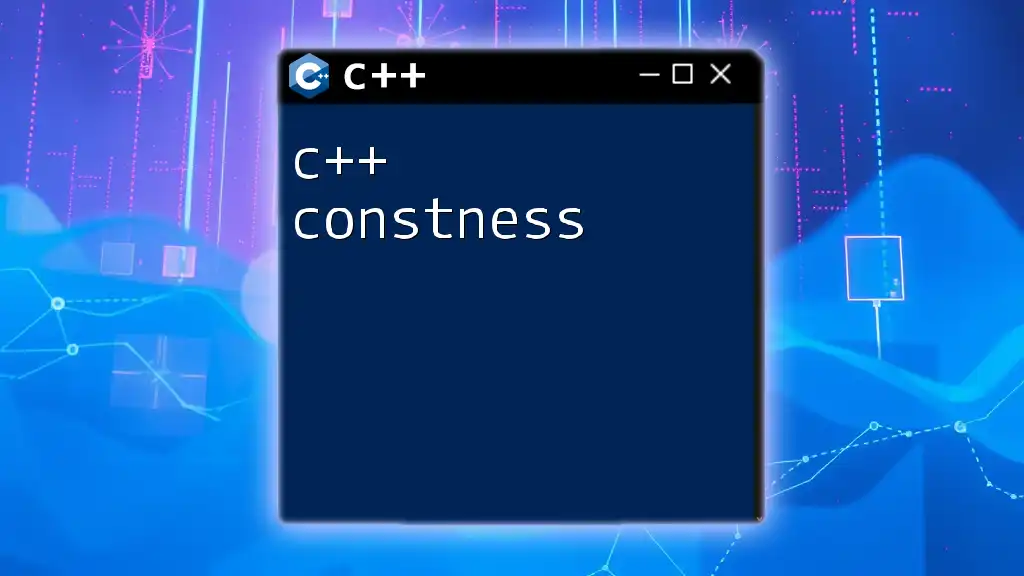
Differences Between `cin` and `cin.getline`
Input Handling
`cin` treats whitespace as a delimiter, meaning it will stop reading as soon as it encounters a space, tab, or newline. In contrast, `cin.getline` reads everything up until the newline characters, capturing spaces within the input, making it ideal for full names or multi-word addresses.
Data Types and Conversion
While `cin` handles primitive types and stops at whitespace, `cin.getline` reads characters, meaning you get the entire line as a string representation without any need for conversion.
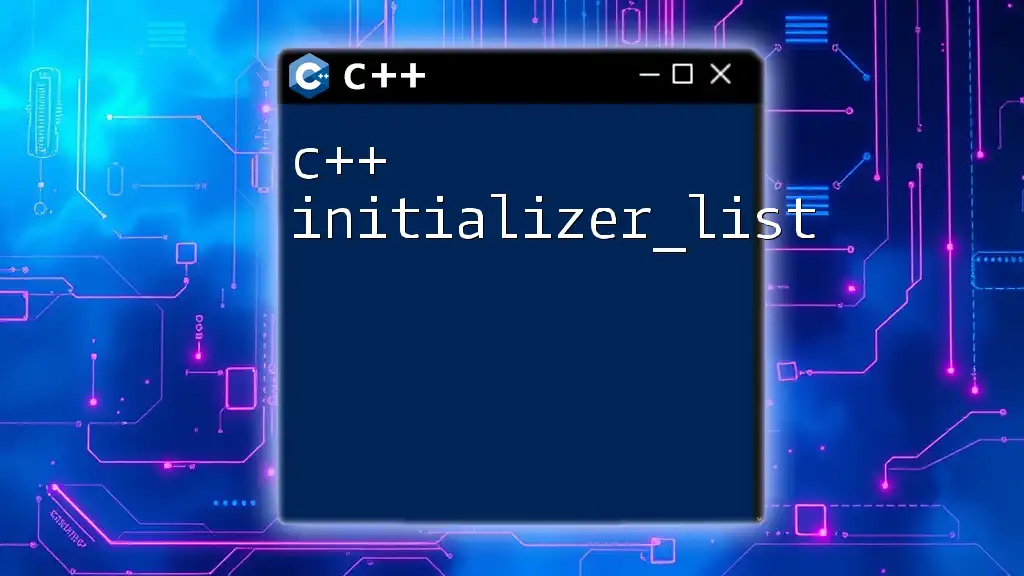
Error Handling with `cin.getline`
Checking for Input Errors
To ensure robustness in your programs, you should always check for input errors. After calling `cin.getline`, you can use `cin.fail()` to determine if the input was successful. Here's how to implement this:
#include <iostream>
using namespace std;
int main() {
char input[10];
cout << "Enter text: ";
cin.getline(input, 10);
if (cin.fail()) {
cout << "Input error occurred." << endl;
cin.clear(); // clear the error state
cin.ignore(numeric_limits<streamsize>::max(), '\n'); // ignore the remaining input
} else {
cout << "You entered: " << input << endl;
}
return 0;
}
Handling Buffer Overflows
To prevent buffer overflows, it’s essential to have a way to alert the user if their input was truncated. You can check the `cin.gcount()` function, which returns the number of characters extracted. If it equals the buffer size minus one, you know the input was too large:
#include <iostream>
#include <limits>
using namespace std;
int main() {
char input[10];
cout << "Enter text: ";
cin.getline(input, 10);
if (cin.gcount() == sizeof(input) - 1) {
cout << "Warning: Input truncated to prevent overflow." << endl;
}
return 0;
}
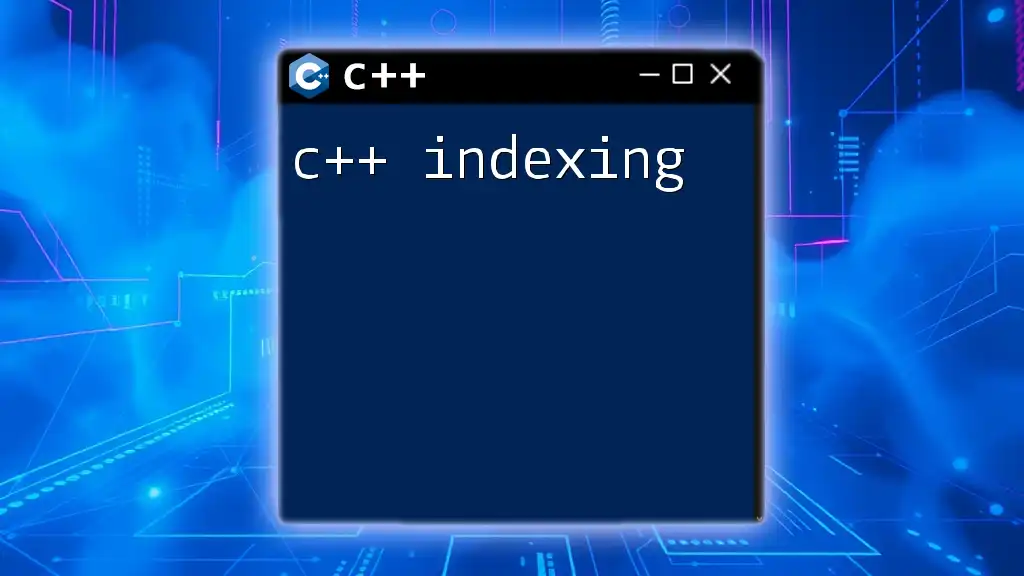
Advanced Usage of `cin.getline`
Using `cin.getline` with `std::string`
For more modern C++ programming, using the `std::string` class is advisable. You can use the `getline` function from the `<string>` library to easily handle strings:
#include <iostream>
#include <string>
using namespace std;
int main() {
string input;
cout << "Enter your response: ";
getline(cin, input);
cout << "You entered: " << input << endl;
return 0;
}
This approach avoids many pitfalls associated with character arrays, such as buffer overflows and manual memory management.
Application in Real-World Scenarios
`cin.getline` is widely utilized in applications where multi-word input is common, such as forms, configuration files, or user prompts for natural language input. Understanding how to effectively capture such input can significantly enhance the user experience in your applications.
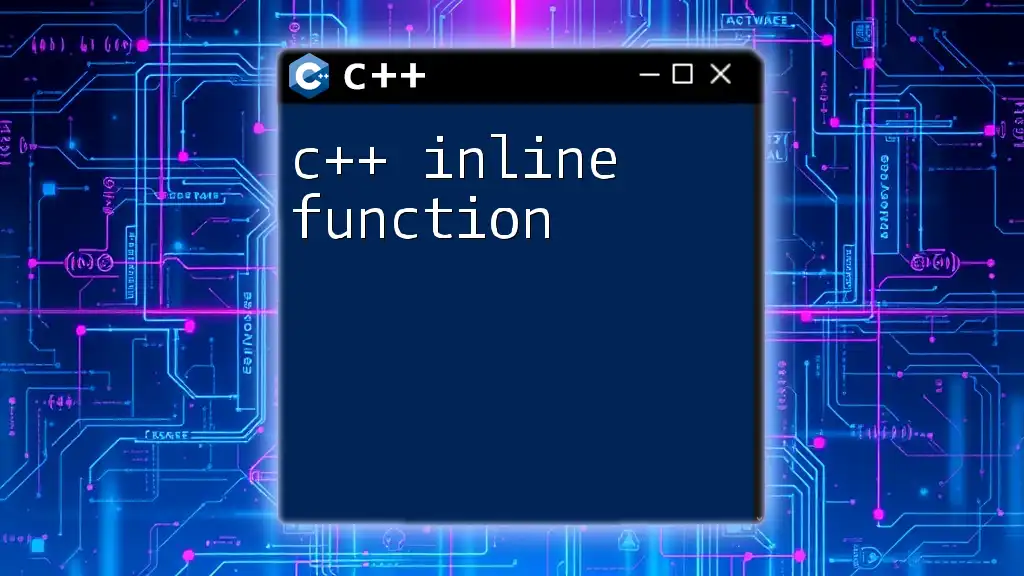
Best Practices for Using `cin.getline`
- Choose the Right Buffer Size: Always allocate sufficient buffer space according to your expected input length.
- Validate Input: Always check for errors post-input to avoid unpredictable behavior in your application.
- Prefer `std::string`: When possible, opt for `std::string` to handle dynamic string lengths and reduce the risks associated with fixed-size buffers.
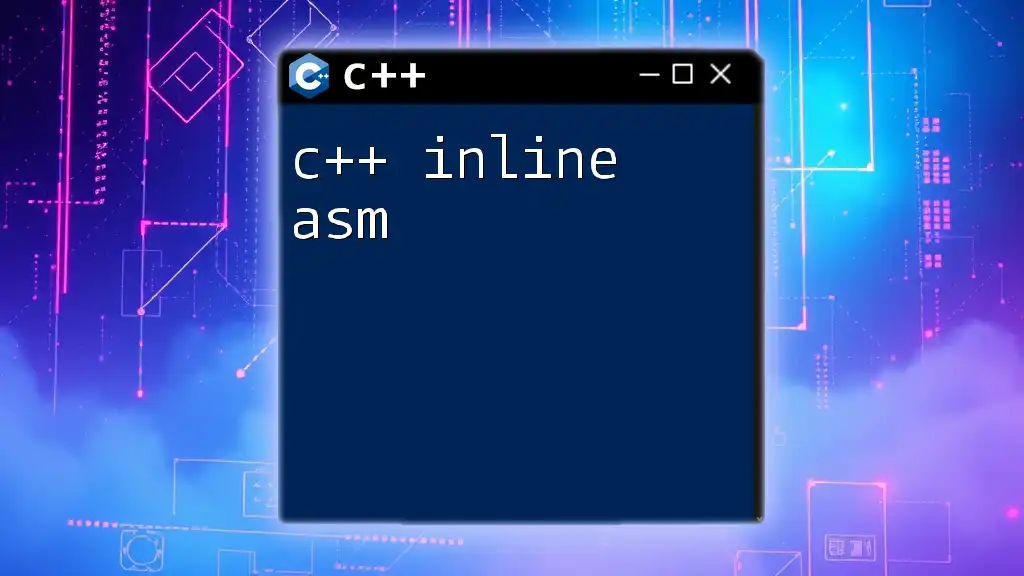
Conclusion
In summary, `c++ cin.getline` is a powerful tool for reading input from users, uniquely accommodating multi-word strings effectively. Its correct use can prevent data input errors and enhance the overall input experience in your C++ programs. I encourage you to practice by implementing it in your projects, experimenting with its features, and integrating it alongside other input methods to gain a comprehensive understanding of input handling in C++.
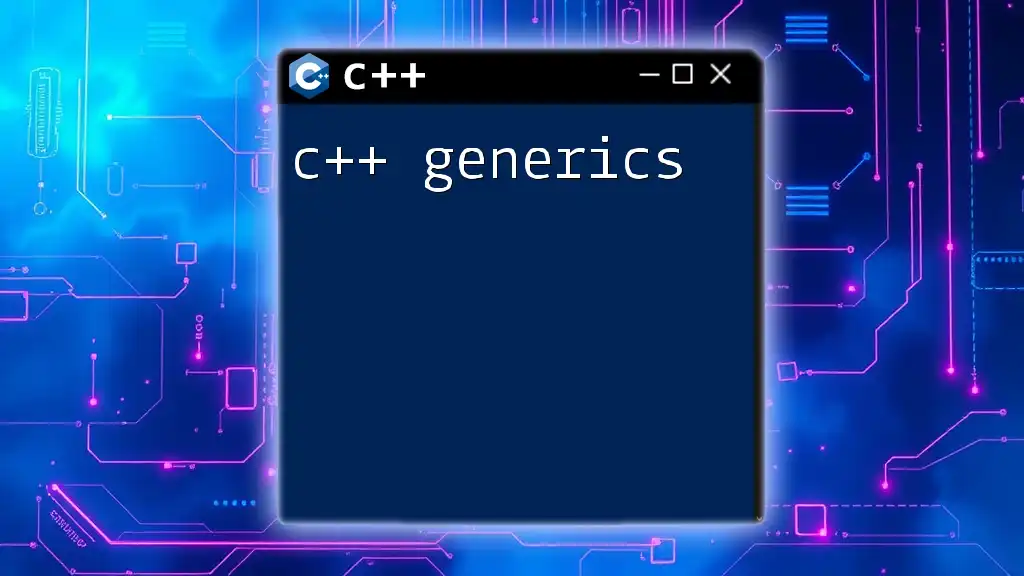
Additional Resources
For those interested in diving deeper into C++ input methods, several tutorials and books provide extensive exploration of C++ concepts helpful for mastering both basic and advanced input handling techniques.