C++ generics allow developers to write flexible and reusable code by creating templates that can operate with any data type.
#include <iostream>
template <typename T>
T add(T a, T b) {
return a + b;
}
int main() {
std::cout << add(5, 10) << std::endl; // Outputs: 15
std::cout << add(3.5, 2.5) << std::endl; // Outputs: 6.0
return 0;
}
Understanding Templates
What are Templates?
Templates are a powerful feature in C++ that allow developers to write generic and reusable code. Rather than creating multiple versions of functions or classes to handle different data types, templates enable you to define a blueprint that can work with any data type. There are primarily two types of templates in C++: class templates and function templates. This distinction is crucial for understanding how to leverage generics effectively in your applications.
Why Use Templates?
Using templates in C++ presents several advantages:
-
Code Reusability: Templates enable you to write a function or class that can be used with different data types without code duplication, leading to cleaner and more maintainable code.
-
Type Safety at Compile Time: Because types are checked at compile time, templates help catch errors early, reducing runtime crashes related to type mismatches.
-
Elimination of Typecasting: Using templates minimizes the need for typecasting, thus lowering the chances of introducing bugs.
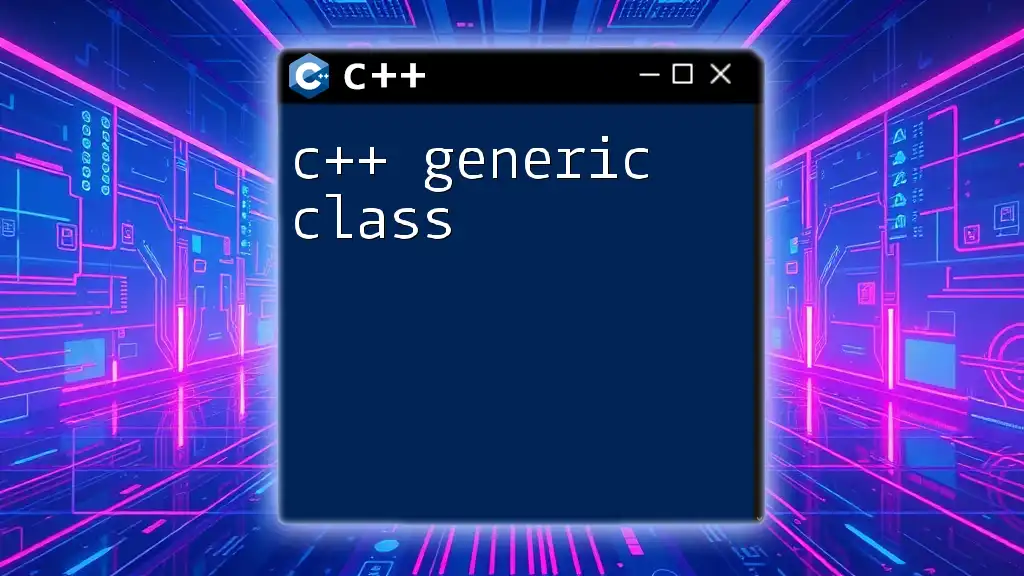
Function Templates
Defining a Function Template
A function template allows you to create a function that can accept any data type as parameters. The basic syntax involves the `template` keyword followed by a template parameter enclosed in angle brackets.
Here’s an example of a simple function template to add two values:
template <typename T>
T add(T a, T b) {
return a + b;
}
In this example, `T` is a placeholder for any data type. You can use the `add` function with integers, floats, or even user-defined types as long as the `+` operator is defined for those types.
Specialization of Function Templates
Sometimes, you may want to customize the behavior of a function template for a specific data type. This is where template specialization comes into play.
Here’s how you can specialize the `add` function for `std::string`:
template <>
std::string add<std::string>(std::string a, std::string b) {
return a + " " + b;
}
This specialization clearly shows how the behavior changes for strings, adding a space between them.
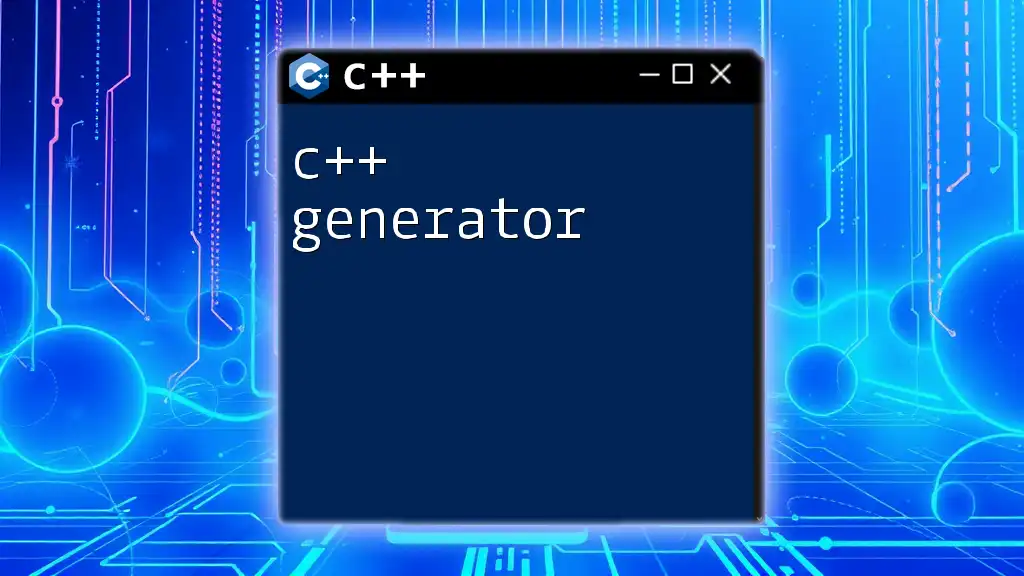
Class Templates
Creating a Class Template
Class templates are similar in concept to function templates but are designed for classes. Through class templates, you can create an entire class that can handle various data types.
Here’s an example of a class template:
template <typename T>
class Box {
public:
Box(T item) : item(item) {}
T getItem() { return item; }
private:
T item;
};
In this `Box` class, the template parameter `T` allows you to create boxes containing any type of item, whether it’s an integer, string, or a custom object.
Advantages of Class Templates
Using class templates provides significant flexibility and reusability in your code. Instead of writing separate classes for different data types, you can create one template class that works for all. This paradigm not only streamlines the codebase but also simplifies the maintenance process as any change needs to be made only in one place.
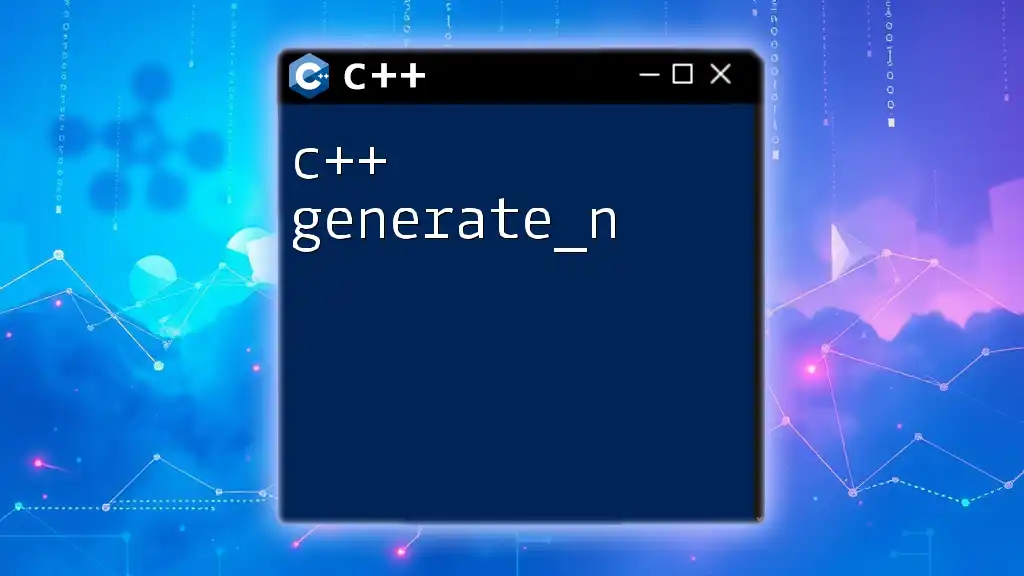
Template Arguments
Non-Type Template Parameters
In addition to data type parameters, templates can accept non-type template parameters. These are parameters that are not types, such as integers or pointers.
For example, here’s how you can define an array with a non-type template parameter representing its size:
template <typename T, int size>
class Array {
public:
T& operator[](int index) { return data[index]; }
private:
T data[size];
};
In this `Array` class template, `size` becomes a compile-time constant that dictates the array's length.
Default Template Arguments
You can also specify default values for template parameters, which is particularly helpful for simplifying function calls. For instance:
template <typename T = int>
class DefaultBox {
public:
DefaultBox(T item) : item(item) {}
T getItem() { return item; }
private:
T item;
};
In this example, if a template argument isn't provided, `DefaultBox` will default to using `int`.
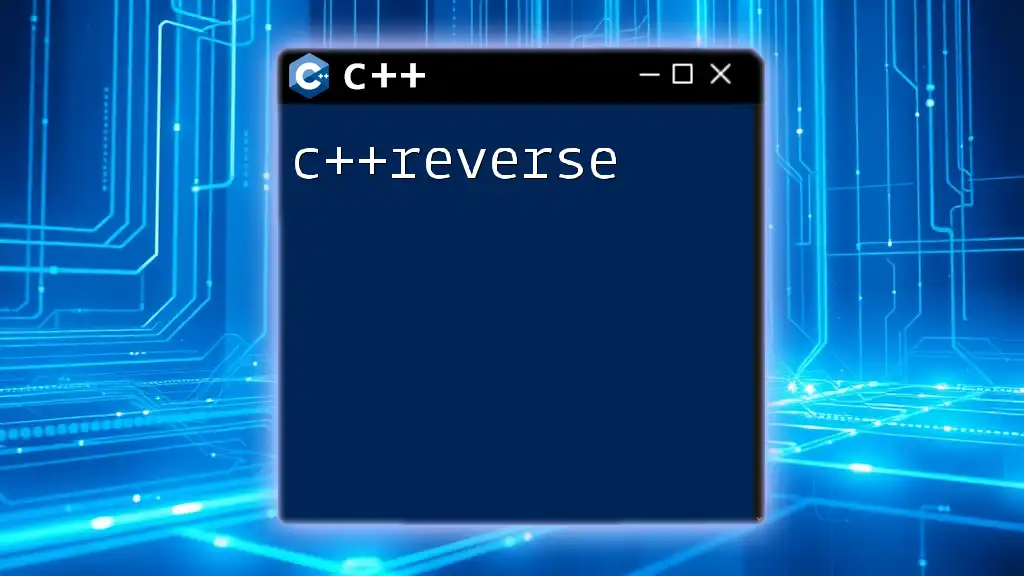
Variadic Templates
Introduction to Variadic Templates
Variadic templates allow you to define templates that can accept an arbitrary number of parameters. This feature enhances the functionality of your templates, providing great flexibility when you need to handle various data types or quantities.
Example of a Variadic Template Function
Here is a simple implementation of a variadic template function, which allows you to print multiple values:
template <typename T>
void print(T value) {
std::cout << value << std::endl;
}
template <typename T, typename... Args>
void print(T value, Args... args) {
std::cout << value << ", ";
print(args...);
}
With this setup, you can call `print(1, "Hello", 3.14)` and it will output all values in a single call.
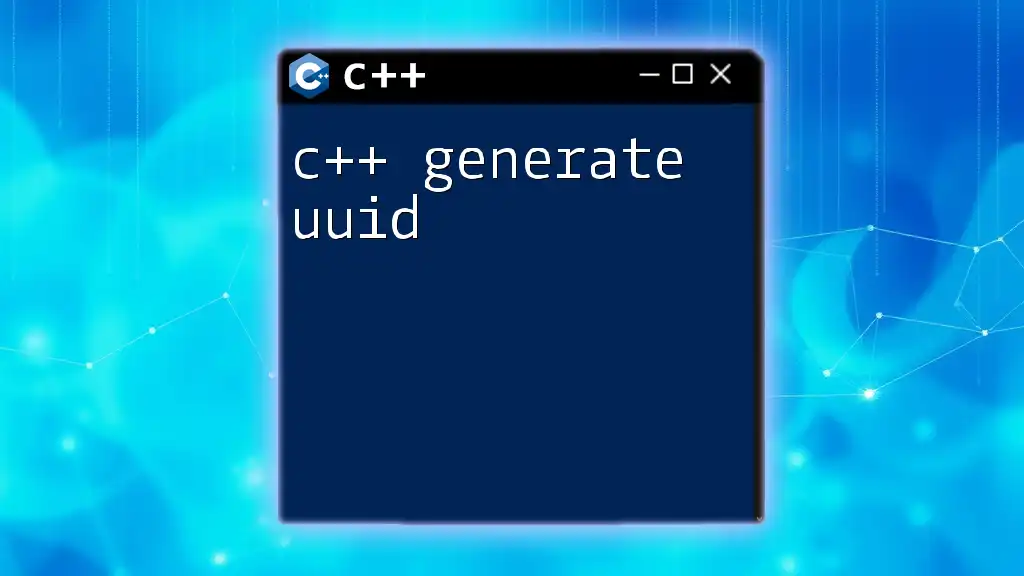
Template Metaprogramming
What is Template Metaprogramming?
Template metaprogramming is an advanced C++ feature that leverages templates to perform computations at compile time. This powerful technique can optimize code and create highly efficient libraries.
Example of Template Metaprogramming
A common use of template metaprogramming is to create type traits. Here’s an example:
template <typename T>
struct is_pointer {
static const bool value = false;
};
template <typename T>
struct is_pointer<T*> {
static const bool value = true;
};
In this code, `is_pointer` determines if a type is a pointer or not, showcasing the ability to manipulate types through templates.
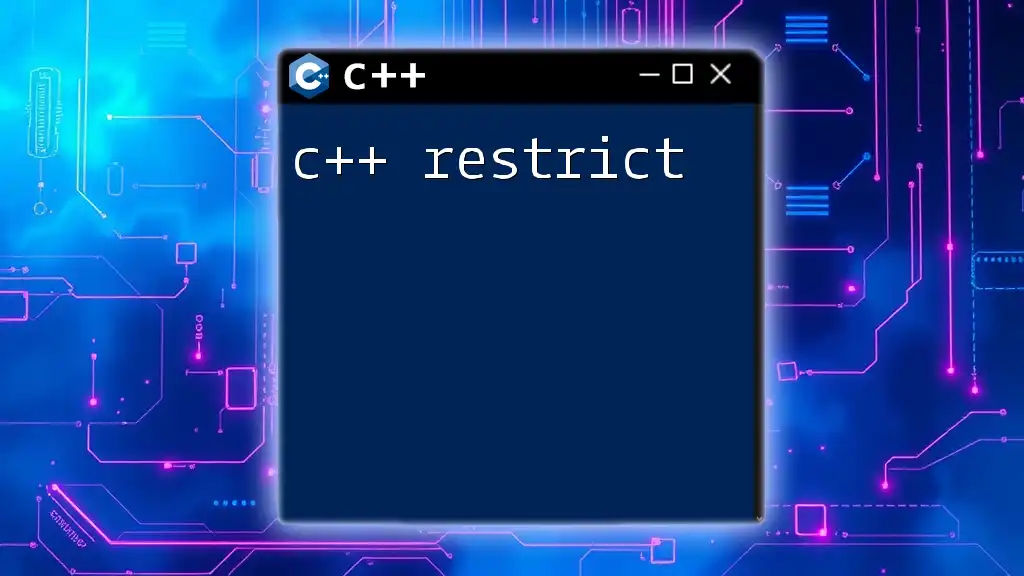
Best Practices for Using Templates in C++
Keeping Templates Simple
When using templates, it's essential to keep definitions concise and to avoid overly complex type relationships. This simplicity not only helps maintain readability but also makes it easier for other developers to understand and use your code effectively.
Avoiding Template Bloat
Template instantiation can lead to increased code sizes, commonly referred to as template bloat. To manage this, prefer using generic programming techniques judiciously and limit instances where templates are instantiated multiple times. Emphasizing good design patterns can also mitigate bloat effectively.
Code Readability and Documentation
Due to the complexity that can arise from templated code, ensuring that your code remains clear and well-documented is crucial. State the purpose and usage of your templates clearly to ensure anyone interacting with your code can do so efficiently.
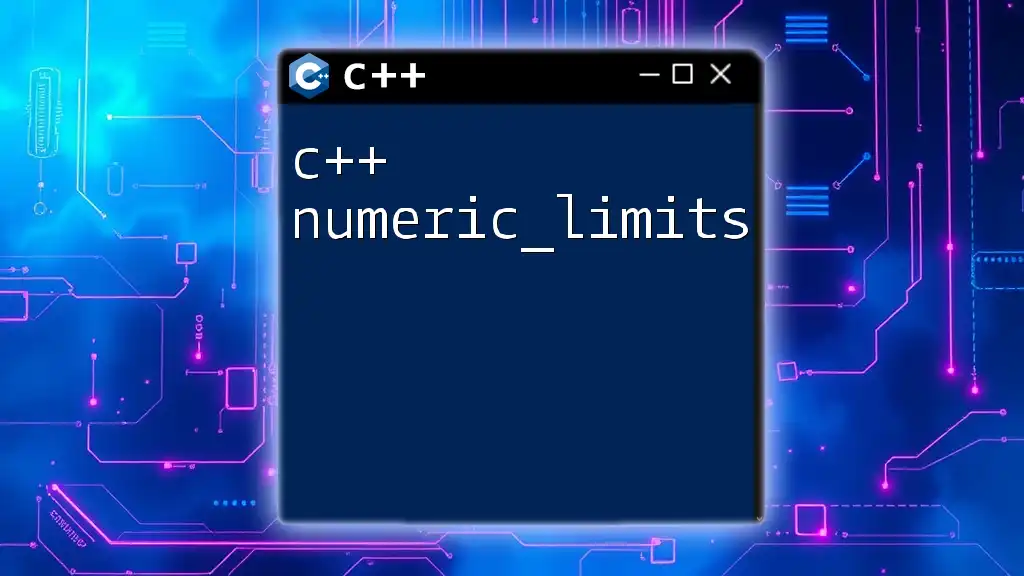
Conclusion
C++ generics through templates provide a robust framework for writing efficient, reusable, and type-safe code. Understanding the various aspects of templates, including function and class templates, specialization, and variadic templates, enables developers to utilize C++'s full potential efficiently. By following best practices regarding simplicity, bloat management, and documentation, you can create templates that enhance your codebase significantly. Engage with the community, share your experiences, and continue exploring the advanced dimensions of C++ generics for ongoing learning and application.
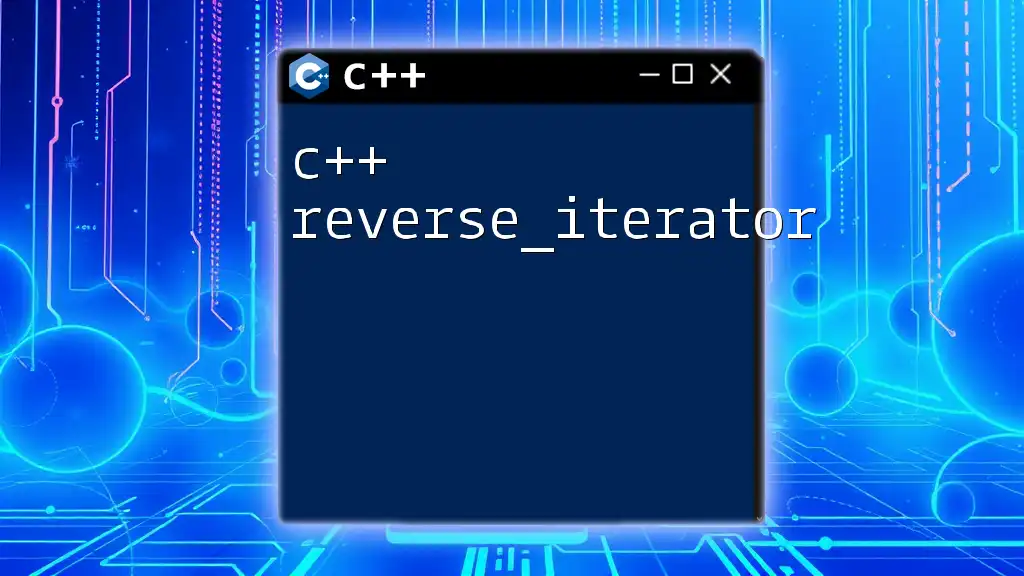
Additional Resources
For those looking to delve deeper, consider exploring recommended books on C++ templates and participating in online courses and tutorials. Engaging with C++ community forums will also provide you with valuable insights and further opportunities to refine your expertise in working with generics.