An inner class in C++ is a class defined within the scope of another class, allowing for tighter encapsulation and organization of related functionalities.
class Outer {
public:
class Inner {
public:
void display() {
std::cout << "Hello from Inner class!" << std::endl;
}
};
};
int main() {
Outer::Inner innerObj;
innerObj.display();
return 0;
}
Understanding C++ Inner Classes
What is an Inner Class?
A C++ inner class is a class defined within the scope of another class, known as the outer class. This structure provides a way to logically group classes that are only used in one place, which enhances encapsulation and organization. The key distinguishing feature is that an inner class can access the members (including private ones) of its outer class.
Consider the following example of a simple inner class:
class Outer {
public:
class Inner {
public:
void display() {
std::cout << "Inner class method called" << std::endl;
}
};
};
void example() {
Outer::Inner innerObj;
innerObj.display(); // Output: Inner class method called
}
In this code snippet, the `Inner` class resides within the `Outer` class. The `display()` method can be called on an instance of `Inner`, clearly demonstrating the functionality of inner classes.
Types of Inner Classes
Non-static Inner Classes
A non-static inner class is associated with an instance of its enclosing class. This means that it can directly access all the members of the outer class, including private members. Here’s an example illustrating this concept:
class Outer {
private:
int outerVar = 10;
public:
class Inner {
public:
void accessOuter(Outer& outer) {
std::cout << "Accessing outerVar: " << outer.outerVar << std::endl;
}
};
};
void example() {
Outer outerObj;
Outer::Inner innerObj;
innerObj.accessOuter(outerObj); // Output: Accessing outerVar: 10
}
In this example, the `Inner` class's `accessOuter` method directly accesses the `outerVar` of the `Outer` instance.
Static Inner Classes
A static inner class is different because it does not have a reference to an instance of its enclosing class. Therefore, it cannot access the outer class’s instance variables or methods directly; it can only access static members. This is particularly useful for utility functions or constants that are closely related to the outer class. Here's an example:
class Outer {
private:
static int staticOuterVar;
public:
class StaticInner {
public:
void display() {
std::cout << "Accessing staticOuterVar: " << Outer::staticOuterVar << std::endl;
}
};
};
int Outer::staticOuterVar = 42;
void example() {
Outer::StaticInner staticInnerObj;
staticInnerObj.display(); // Output: Accessing staticOuterVar: 42
}
In this case, the `StaticInner` class can access the static member `staticOuterVar` of the `Outer` class.
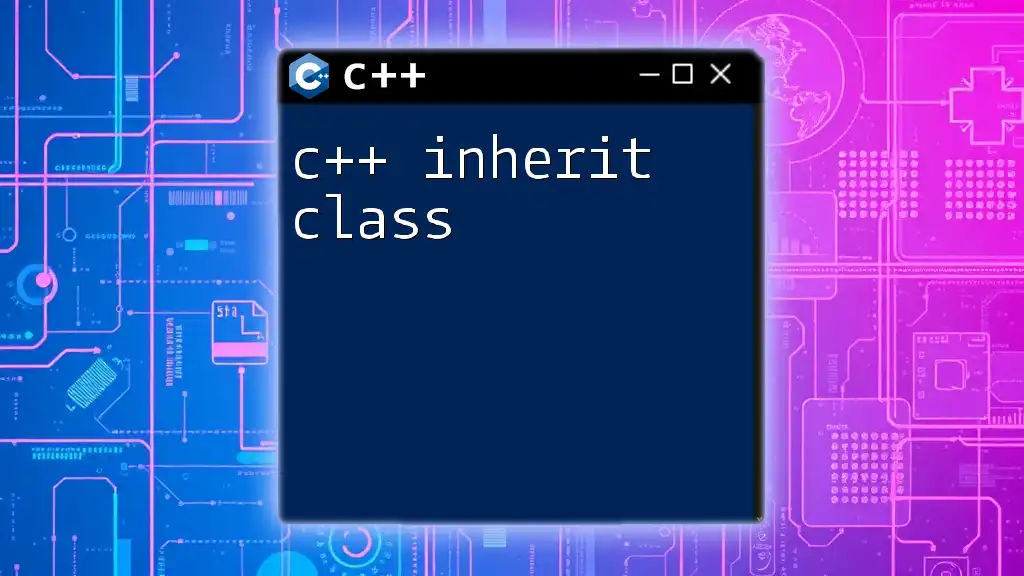
Benefits of Using Inner Classes
Encapsulation
One of the primary advantages of C++ inner classes is enhanced encapsulation. By utilizing inner classes, you can hide implementation details and expose only what is necessary. This leads to improved security and integrity of your class design. Because inner classes can access private members of the outer class, they serve as a means of structuring functionality that is closely tied to the outer class's implementation without exposing those details to the rest of the program.
Improved Organization
When designing software, organization is crucial. Inner classes help to keep your codebase organized by grouping related classes together. This can lead to easier maintenance and readability. For example, if an inner class is only relevant to the outer class and does not have any utility elsewhere, keeping it nested keeps the namespace clean and focuses the reader's attention where it matters.
Access to the Outer Class
Inner classes have direct access to the outer class's members. This capability simplifies the design by reducing boilerplate code needed to pass references to the outer class. Here’s an example that illustrates the ease of access:
class Outer {
private:
int outerData = 20;
public:
class Inner {
public:
void showData(Outer& outer) {
std::cout << "Outer data: " << outer.outerData << std::endl;
}
};
};
void example() {
Outer outerObj;
Outer::Inner innerObj;
innerObj.showData(outerObj); // Output: Outer data: 20
}
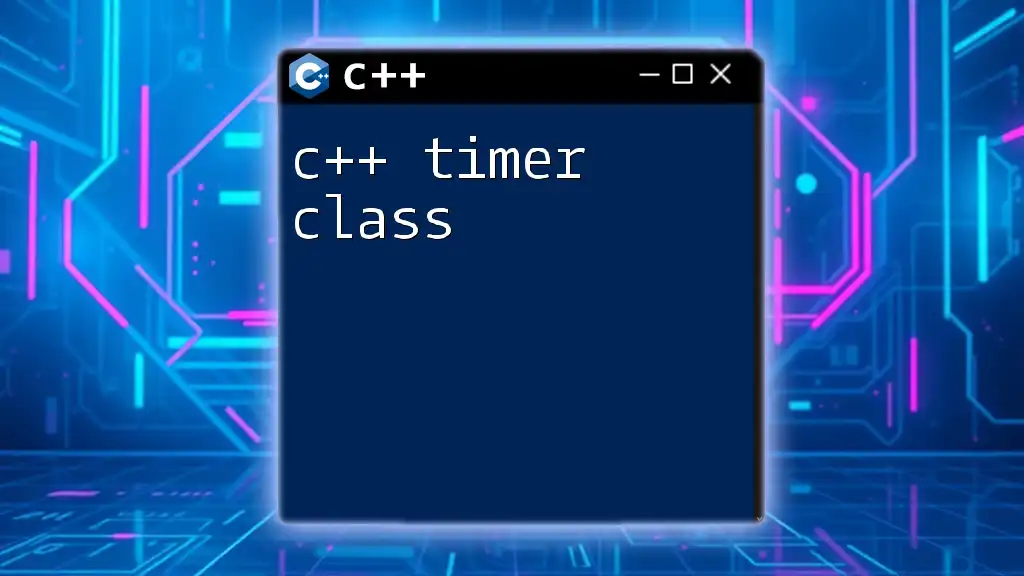
Practical Applications of Inner Classes
Real-World Example: GUI Framework
Consider a graphical user interface (GUI) framework where you might have various elements such as buttons, panels, and windows. Using inner classes, you can keep all related classes that pertain to a particular window encapsulated and organized together.
class Window {
public:
class Button {
public:
void click() {
std::cout << "Button Clicked!" << std::endl;
}
};
};
void createButton() {
Window::Button btn;
btn.click(); // Output: Button Clicked!
}
In this scenario, the `Button` class is tightly coupled with the `Window` class. By nesting it as an inner class, you streamline the structure of your GUI components.
Data Structures and Algorithms
Inner classes can also simplify the implementation of complex data structures. For example, a tree or a graph might use inner classes to define nodes or edges. This allows you to encapsulate functionality specific to nodes or edges without cluttering the main structure.
class Tree {
public:
class Node {
public:
int value;
Node* left;
Node* right;
Node(int val) : value(val), left(nullptr), right(nullptr) {}
};
// Implementation of tree functions...
};
Here, the `Node` class, which serves as a building block for the `Tree`, is defined as an inner class, maintaining logical cohesion.
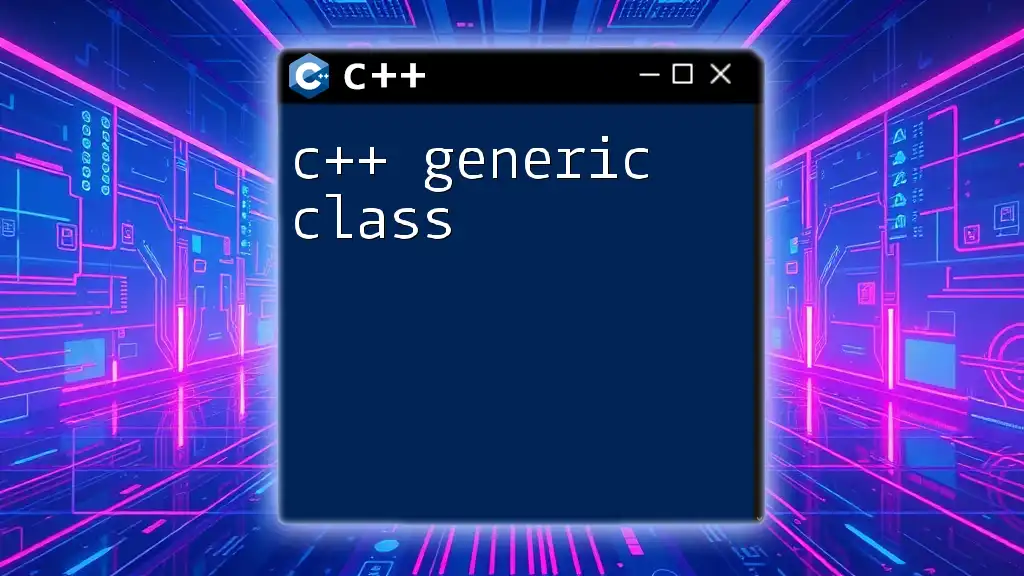
Best Practices for Using Inner Classes
Keep it Simple
When implementing C++ inner classes, it's essential to maintain clarity. Inner classes should remain straightforward and tied closely to the functionality of the outer class. Overcomplicating inner classes can lead to confusion and decreased readability. Use inner classes only when they serve a clear purpose related to the outer class’s functionality.
Avoid Overusing Inner Classes
While inner classes can provide organization, excessive nesting can lead to unwieldy code structures. Make sure to strike a balance between depth of nesting and the maintainability of your code. In scenarios where inner classes proliferate, consider whether refactoring the design to use free-standing classes might make it more understandable.
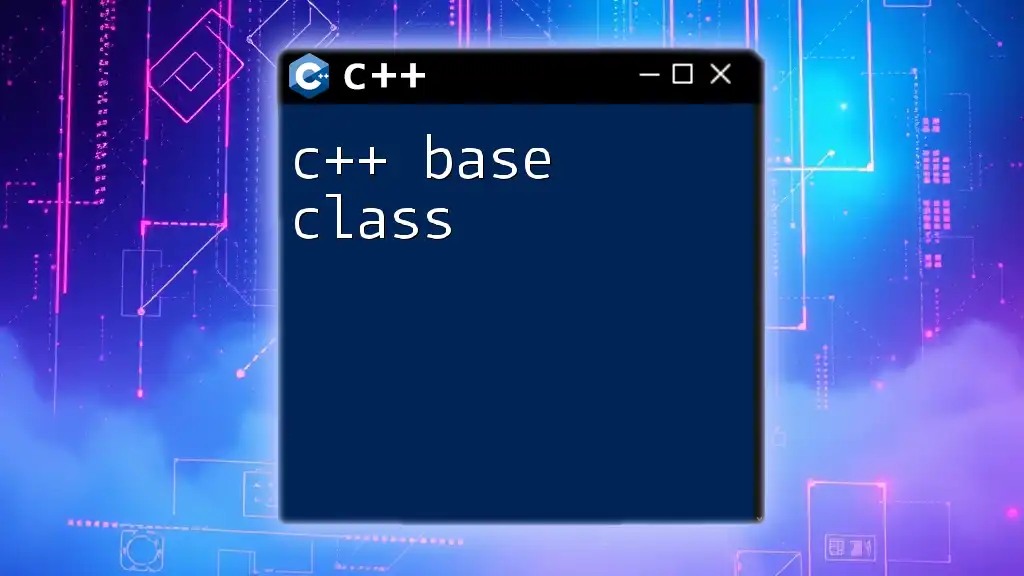
Common Pitfalls and Misconceptions
Misunderstanding Scope and Access
One common misconception about inner classes is related to scope and access rules. Developers may find themselves confused about what members are accessible from within an inner class. It's imperative to understand that non-static inner classes can access all members (both static and non-static) of the outer class, but static inner classes can only access static members.
Performance Considerations
While the performance impact of using C++ inner classes is generally minimal, it’s worth being aware that overly complex arrangements could lead to challenges in optimization. Ensure code readability and performance do not suffer from excessive nesting.
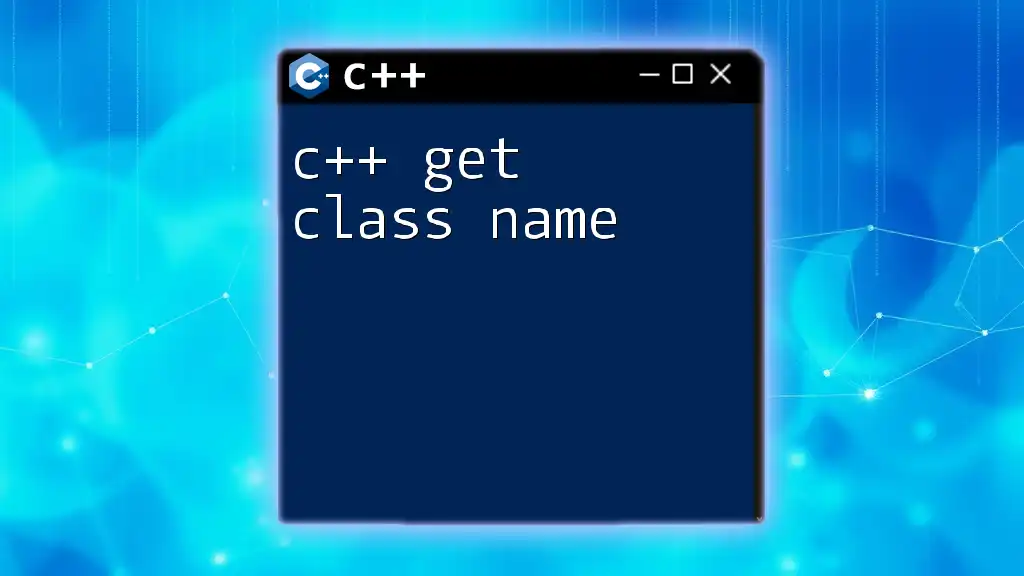
Conclusion
In conclusion, understanding C++ inner classes is crucial for modern C++ programming. They provide meaningful encapsulation, improve organization, and facilitate direct access to outer class members. As you practice and integrate inner classes into your projects, you’ll find they can simplify complex problem-solving and help structure your applications effectively. Embrace the benefits, but be cautious of overcomplicating designs with unnecessary nesting. Happy coding!
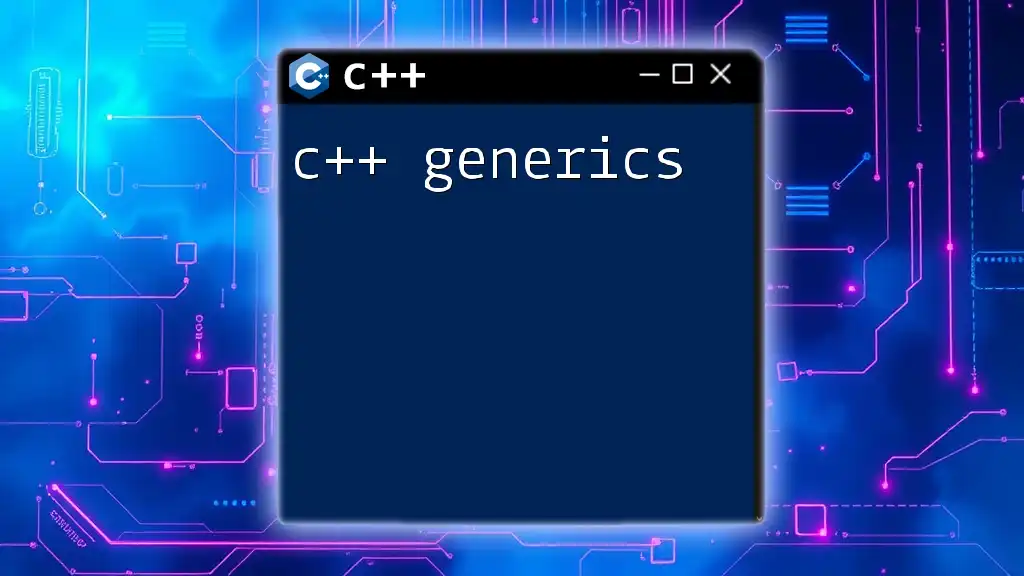
Further Reading and Resources
To expand your knowledge further, consider checking out resources on C++ design patterns, advanced object-oriented programming techniques, and recommended books on C++.