C++ subclassing allows a derived class to inherit properties and behaviors (methods) from a base class, facilitating code reuse and logical organization.
Here’s a simple example:
class Base {
public:
void display() {
std::cout << "Base class display" << std::endl;
}
};
class Derived : public Base {
public:
void show() {
std::cout << "Derived class show" << std::endl;
}
};
Understanding C++ Subclassing
What is Subclassing?
Subclassing is a fundamental concept in object-oriented programming (OOP) that allows a new class, known as a subclass, to inherit properties and behaviors from an existing class, referred to as the superclass or base class. This relationship creates a hierarchy that facilitates code reuse, where common functionality can be encapsulated in the superclass, while specific features are defined in the subclass.
Benefits of Using Subclassing in C++
The use of subclassing in C++ offers several key advantages:
- Code Reusability: Rather than duplicating code, developers can create subclasses that inherit functionality, leading to more efficient and maintainable code.
- Simplified Maintenance: Changes made in the superclass propagate to all subclasses, making updates easier and reducing the risk of errors.
- Enhanced Functionality: Subclassing allows developers to extend or modify the behavior of existing classes without altering their original definitions.
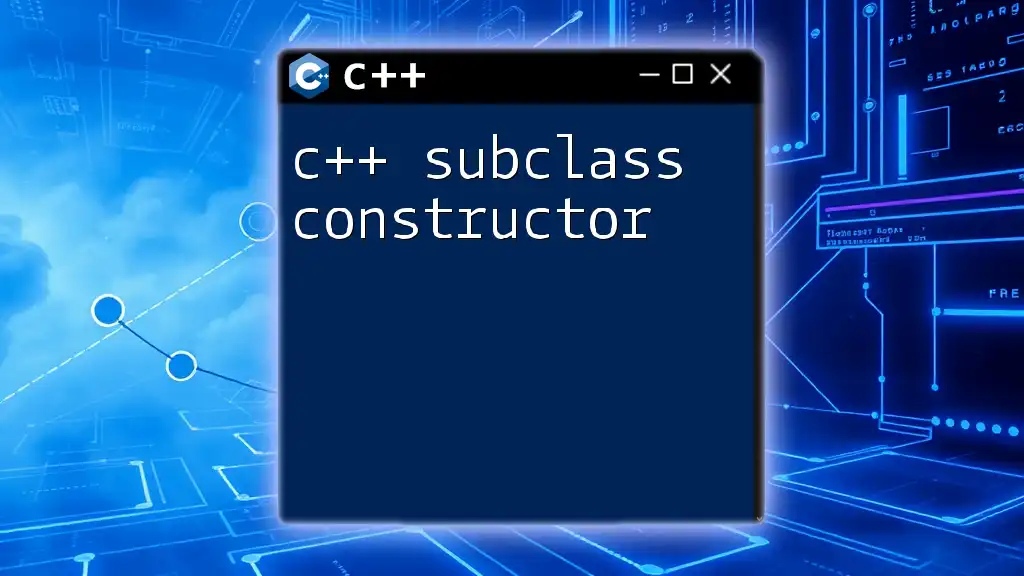
The Basics of C++ Inheritance
Definition of Inheritance
In C++, inheritance is a mechanism for establishing a new class from an existing one. The new class inherits attributes and methods of the existing class, allowing for specialization of behaviors.
Types of Inheritance
There are several types of inheritance in C++:
- Single Inheritance: One subclass extends a single superclass.
- Multiple Inheritance: A subclass inherits from multiple superclasses, allowing more complex hierarchies.
- Multilevel Inheritance: A chain of inheritance where a subclass serves as a superclass for another subclass.
- Hierarchical Inheritance: Multiple subclasses inherit from one superclass, creating a tree-like structure.
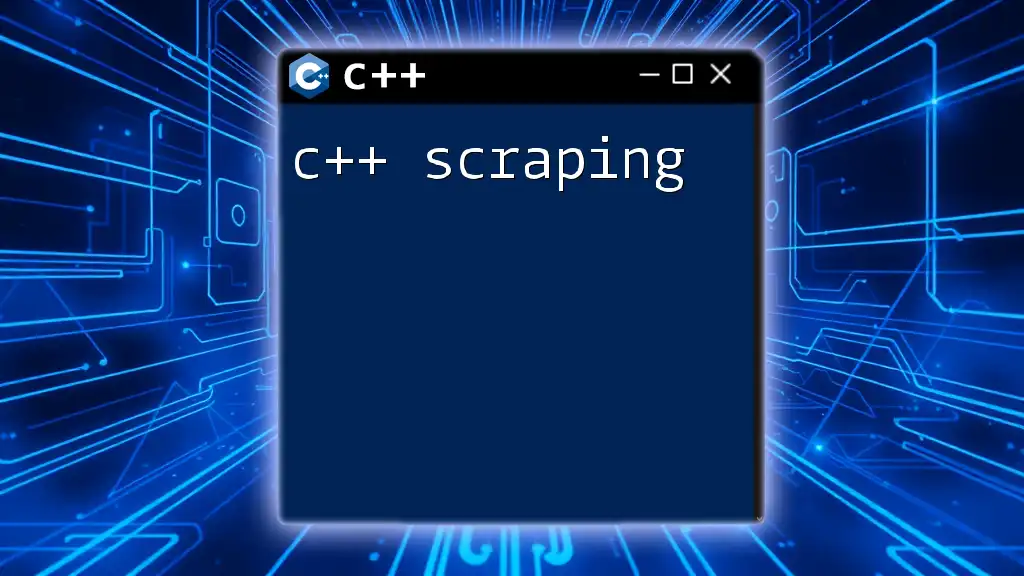
Creating a Subclass in C++
Syntax for Defining a Subclass
Creating a subclass in C++ is straightforward. The syntax involves using a colon followed by the keyword indicating the access level (e.g., public, private, protected).
class ParentClass {
// member variables and functions
};
class SubClass : public ParentClass {
// subclass-specific members
};
Example of a Simple Subclass
Consider a basic example involving a `Vehicle` superclass and a `Car` subclass:
class Vehicle {
public:
void start() {
std::cout << "Vehicle started." << std::endl;
}
};
class Car : public Vehicle {
public:
void honk() {
std::cout << "Car honking." << std::endl;
}
};
int main() {
Car myCar;
myCar.start(); // Output: Vehicle started.
myCar.honk(); // Output: Car honking.
return 0;
}
In this example, the `Car` subclass inherits the `start` method from `Vehicle`, showcasing how a subclass can leverage functionality from its parent.
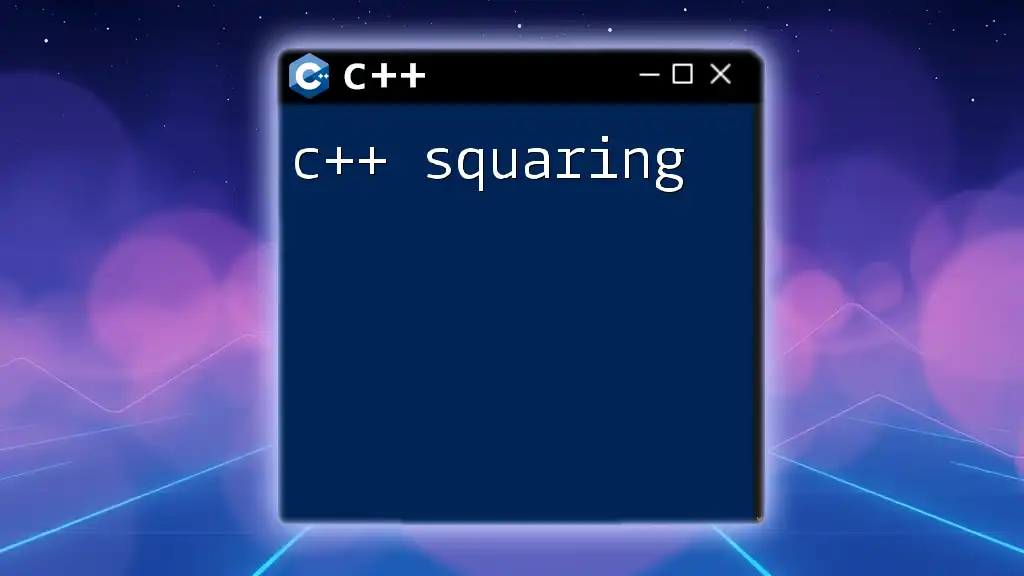
Overriding and Overloading in Subclassing
What is Method Overriding?
Method overriding occurs when a subclass provides a specific implementation of a method that is already defined in its superclass. This allows subclasses to modify or enhance inherited behaviors.
Example of Method Overriding
Let’s explore method overriding through a practical example with a `Vehicle` superclass:
class Vehicle {
public:
virtual void start() {
std::cout << "Vehicle started." << std::endl;
}
};
class Car : public Vehicle {
public:
void start() override {
std::cout << "Car started with a roar!" << std::endl;
}
};
In this case, the `Car` subclass overrides the `start` method to provide a specialization. The `virtual` keyword in the superclass enables this behavior, while the `override` keyword ensures that the method is indeed overriding a base class function.
What is Method Overloading?
Method overloading allows multiple functions with the same name to coexist in the same scope, differentiated by their parameter types or counts. While this is a different concept from overriding, both enhance flexibility and functionality in class design.
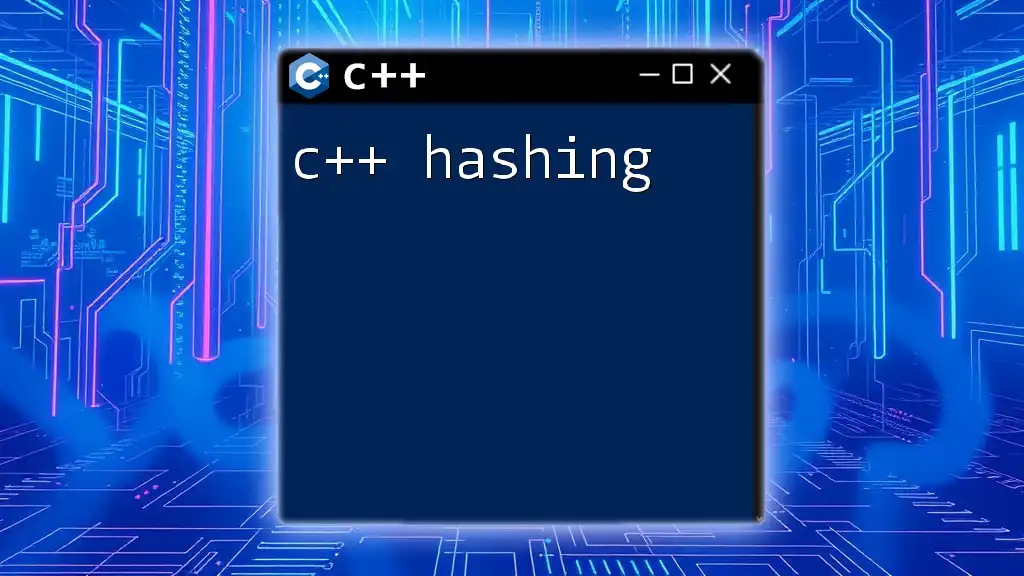
Access Specifiers and Their Role in Subclassing
Understanding Access Specifiers
C++ employs three access specifiers: public, protected, and private. Each handles the visibility of class members and affects how subclasses interact with these members.
Impact of Access Specifiers on Subclassing
- Public Members: Accessible from any part of the program, including outside the class.
- Protected Members: Accessible within the class and by derived classes, but not from other parts of the program.
- Private Members: Accessible only from within the class itself, not by subclasses or outside classes.
This hierarchy of access controls how subclasses can interact with the data and behavior of their superclasses, and thus influences design choices in subclassing.
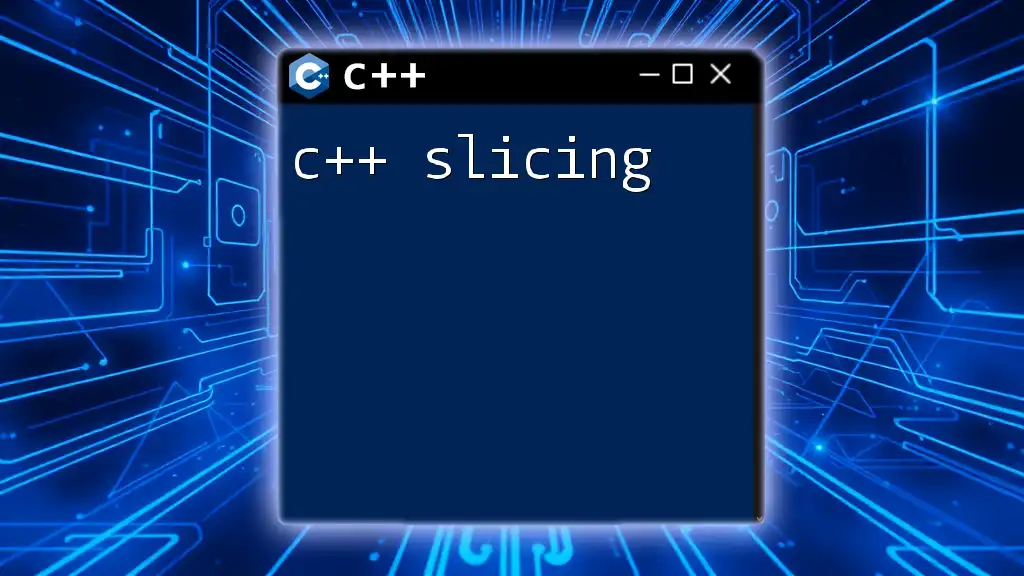
Abstract Classes and Interfaces in C++
What is an Abstract Class?
An abstract class serves as a blueprint for other classes and contains at least one pure virtual function. This enforces a contract for subclasses, ensuring that they implement specific methods.
Implementing Abstract Classes in Subclassing
In the following example, we define an abstract class `Shape`:
class Shape {
public:
virtual void draw() = 0; // Pure virtual function
};
Creating Concrete Subclasses from Abstract Classes
Subclasses implementing this abstract class must define the pure virtual function:
class Circle : public Shape {
public:
void draw() override {
std::cout << "Drawing a circle." << std::endl;
}
};
This structure promotes a consistent interface across different subclasses, ensuring that all shapes can be "drawn."
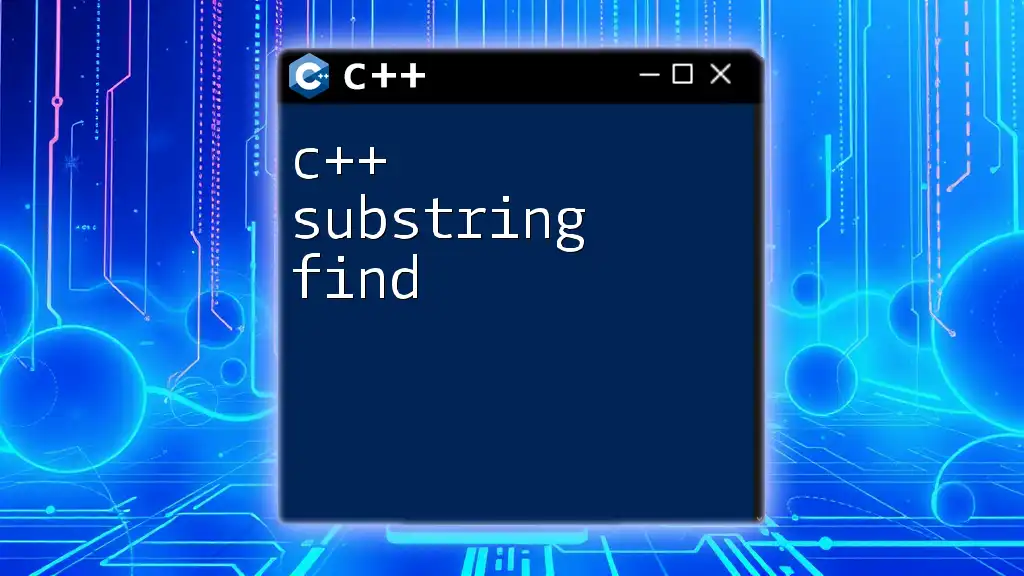
Polymorphism and Subclassing
Understanding Polymorphism in C++
Polymorphism allows objects of different subclasses to be treated as objects of a common superclass. This is key in creating flexible and extendable code designs.
Example of Polymorphism
Utilizing base class pointers, polymorphism allows for invoking overridden methods seamlessly:
void renderShape(Shape* shape) {
shape->draw();
}
Circle circle;
renderShape(&circle); // Output: Drawing a circle.
This showcases how polymorphism fosters a layer of abstraction, enabling code that operates on superclass references to engage with subclass implementations efficiently.
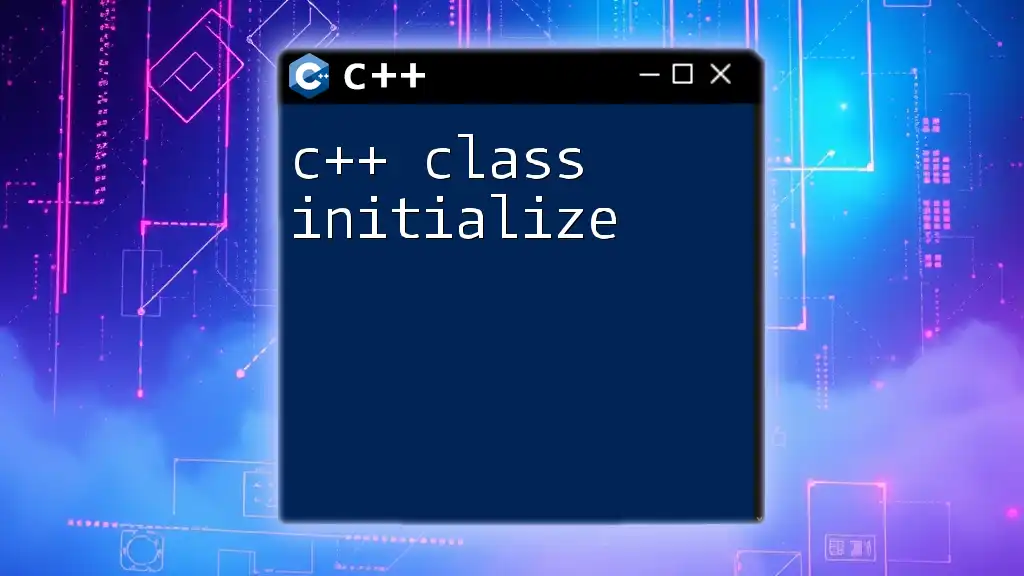
Best Practices for Subclassing in C++
Avoiding Common Pitfalls
When designing classes, it’s essential to avoid overly complex inheritance hierarchies. Such complexity can lead to code that is difficult to understand and maintain. Aim for simplicity.
Tips for Effective Subclass Design
- Composition over Inheritance: Prefer composition when appropriate, as it often leads to more flexible and clear designs.
- Favoring Interfaces and Abstract Classes: Encourage the use of abstract classes to define common behavior while allowing subclasses the liberty to implement specific functionalities.
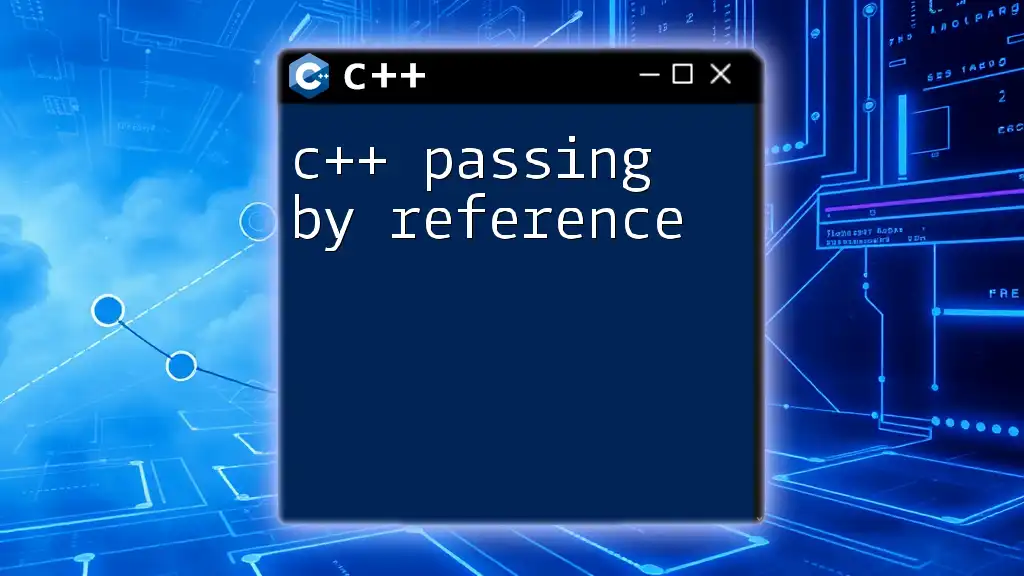
Conclusion
Understanding C++ subclassing is fundamental for mastering object-oriented programming principles. By leveraging inheritance, method overriding, and polymorphism, developers can create efficient, reusable, and maintainable code.
To truly grasp these concepts, it’s essential to practice by implementing subclassing in personal projects. The more you apply these principles, the more intuitive they will become.