In C++, a subclass (or derived class) constructor is a special function that initializes objects of that subclass, often invoking the constructor of its parent (or base) class to ensure proper construction.
Here's an example:
#include <iostream>
class Base {
public:
Base() { std::cout << "Base class constructor called." << std::endl; }
};
class Derived : public Base {
public:
Derived() : Base() { std::cout << "Derived class constructor called." << std::endl; }
};
int main() {
Derived obj; // Creates a Derived object, calls both base and derived constructors
return 0;
}
What is a Constructor?
A constructor is a special member function in C++ that is invoked when an object of a class is created. This function is primarily responsible for initializing the object's data members.
Types of Constructors
- Default Constructor: A constructor with no parameters. It initializes an object without any initial values.
- Parameterized Constructor: A constructor that requires arguments to initialize an object with specific values.
- Copy Constructor: A constructor that creates a new object as a copy of an existing object.
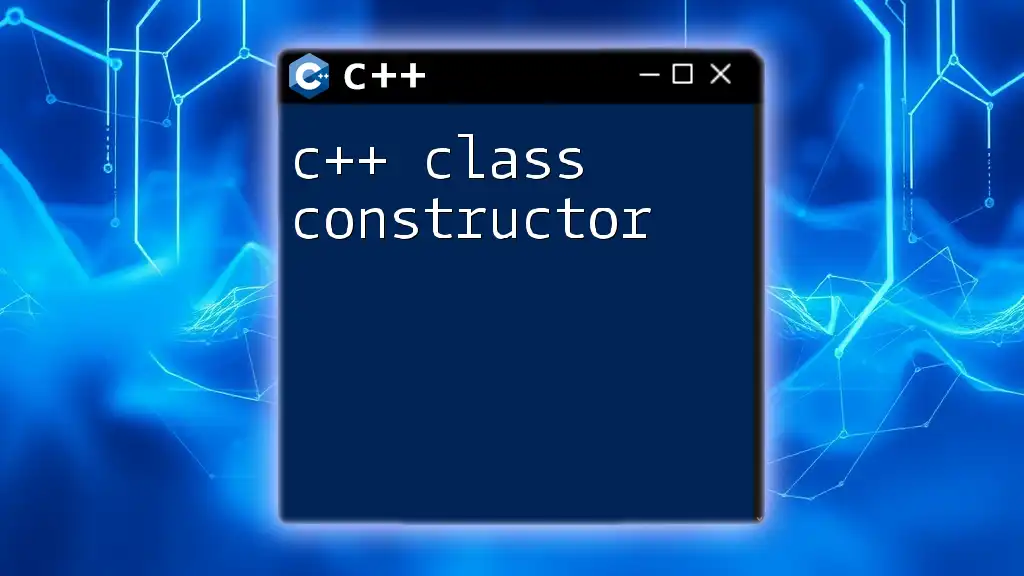
Understanding Inheritance in C++
Inheritance is a fundamental concept in C++ that allows a class (known as a subclass or derived class) to inherit properties and behaviors (methods) from another class (known as a base class). This mechanism promotes code reusability and enhances the organizational structure of your code.
Benefits of Using Inheritance
- Reusability: You can reuse code from the base class in the subclass, reducing redundancy.
- Organization: Code becomes easier to understand when inherited hierarchies are well-defined.
- Maintainability: Changes made in the base class propagate to the subclasses, simplifying upkeep.
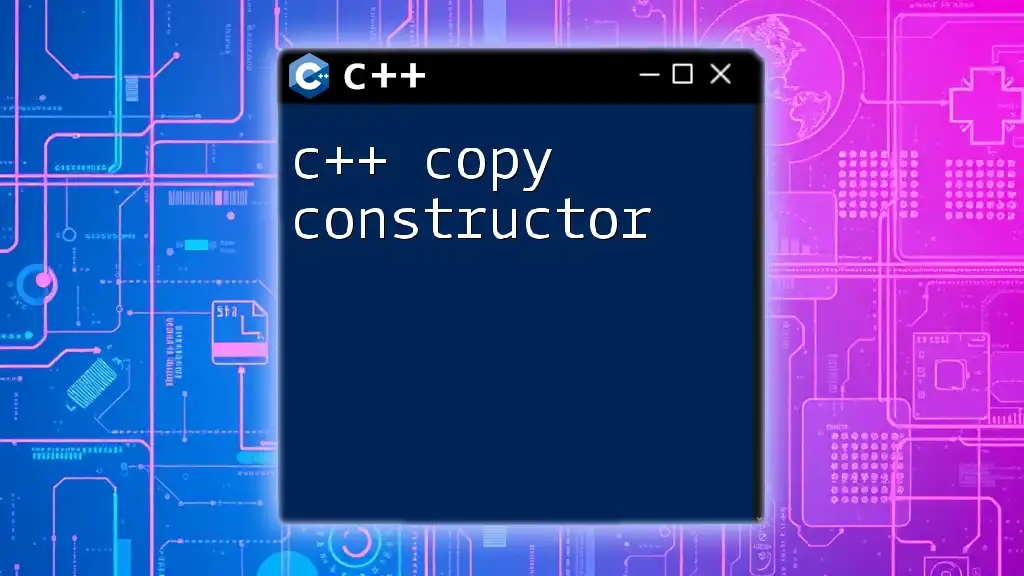
Anatomy of a Subclass Constructor
What is a Subclass Constructor?
A subclass constructor is a constructor that initializes an instance of a subclass. It sets up the new object while also ensuring that the base class is appropriately initialized.
Syntax of a Subclass Constructor
The syntax for a subclass constructor requires that you explicitly call the base class constructor in the initialization list. Here’s an example:
class Base {
public:
Base(int x) { /*...*/ }
};
class Subclass : public Base {
public:
Subclass(int x, int y) : Base(x) { /*...*/ }
};
In this example, the `Subclass` constructor takes two parameters and initializes the base class `Base` using the initialization list. The base class constructor (`Base(x)`) is invoked before the subclass constructor's body executes.
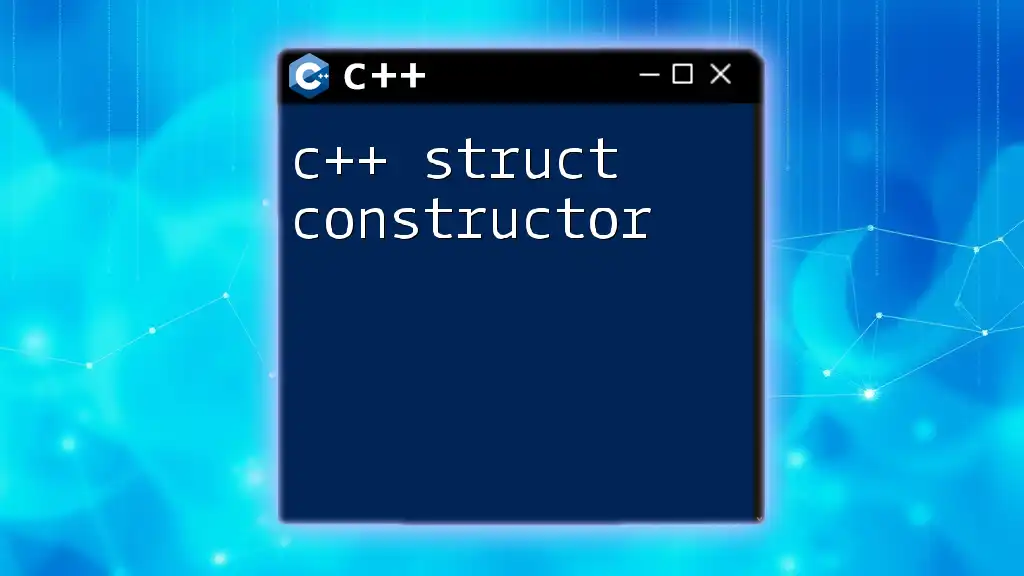
How to Initialize a Base Class in a Subclass Constructor
Using an Initialization List
An initialization list is used in the constructor definition to initialize base class members before the body of the constructor runs. This approach is generally preferred over assignment within the constructor body because it can lead to better performance and correctness.
Example of Using Initialization List
Consider the following classes:
class Animal {
public:
Animal(const std::string& name) { /*...*/ }
};
class Dog : public Animal {
public:
Dog(const std::string& name, int age) : Animal(name) { /*...*/ }
};
In this snippet, the `Dog` constructor initializes the base class `Animal` first, ensuring that the `Animal` part of the `Dog` object is completely constructed with the specified `name` before executing the body of the `Dog` constructor.
Constructor Chaining
Constructor chaining refers to the practice of calling a constructor of a base class from the constructor of a subclass, potentially chaining through multiple levels of inheritance.
Example of Constructor Chaining
Here’s how constructor chaining operates in a more complex example:
class Vehicle {
public:
Vehicle(int wheels) { /*...*/ }
};
class Car : public Vehicle {
public:
Car(int wheels, const std::string& model) : Vehicle(wheels) { /*...*/ }
};
class ElectricCar : public Car {
public:
ElectricCar(int wheels, const std::string& model, int batteryLife)
: Car(wheels, model) { /*...*/ }
};
In this example, when you create an instance of `ElectricCar`, the `Car` constructor is called first, followed by the `Vehicle` constructor, illustrating how each level of the hierarchy is set up in order.
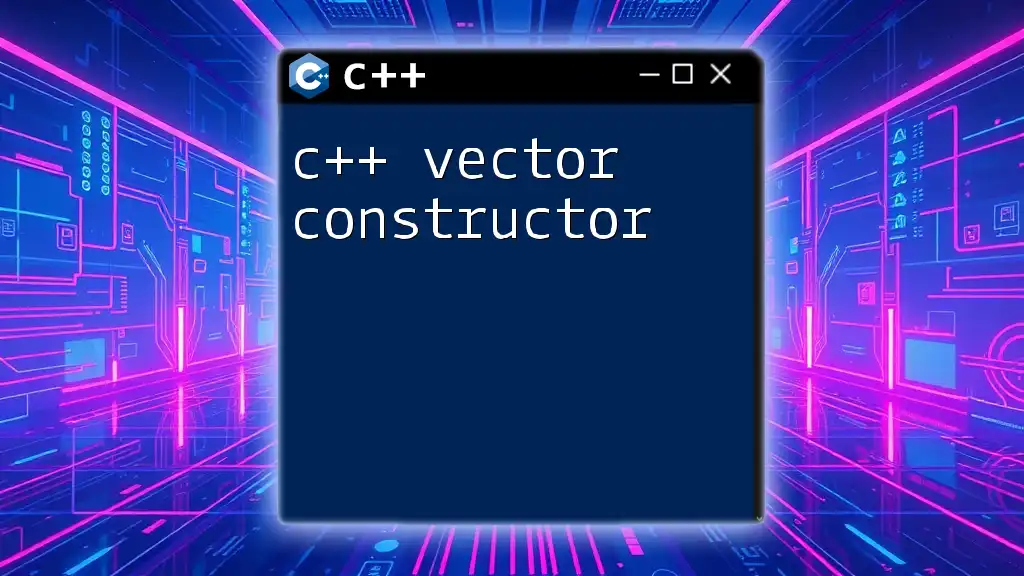
Best Practices for Subclass Constructors
Keep It Concise
Constructors should be kept clear and concise. A complex constructor can be difficult for others (or yourself in the future) to understand. If a constructor requires many parameters, consider using default arguments, encapsulating complex initialization logic in helper functions, or redesigning your class.
Use Default Arguments
A default constructor argument can simplify the creation of objects, allowing certain parameters to be omitted when instantiating an object.
Example
class Shape {
public:
Shape(int sides = 0) { /*...*/ }
};
class Triangle : public Shape {
public:
Triangle(int sides = 3) : Shape(sides) { /*...*/ }
};
In this case, `Triangle` objects default to a specific state while still allowing for custom values to be passed.
Consider Using Access Specifiers
Access specifiers (public, protected, private) affect the visibility of base class members. Utilizing them correctly can ensure encapsulation and control over your data, preventing unauthorized access.
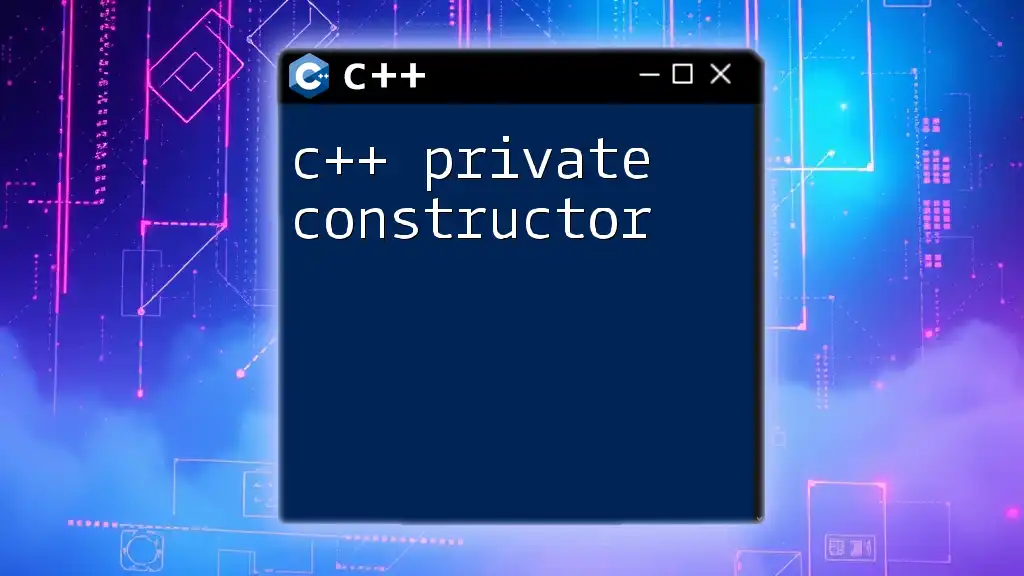
Common Pitfalls When Using Subclass Constructors
Forgetting Base Class Constructors
A common issue arises when developers neglect to call the appropriate base class constructor, which can lead to undefined behavior or uninitialized object data. Always ensure that the base class constructor is invoked to uphold object integrity.
Improper Use of Virtual Functions
Whenever you use virtual functions in a class hierarchy, it's crucial to implement a virtual destructor in the base class. This ensures that when an object is deleted through a base class pointer, the proper subclass destructor gets called, preventing memory leaks.
class Base {
public:
virtual ~Base() { /*...*/ }
};
Implementing a virtual destructor safeguards against potential runtime errors that can arise from improper cleanup.
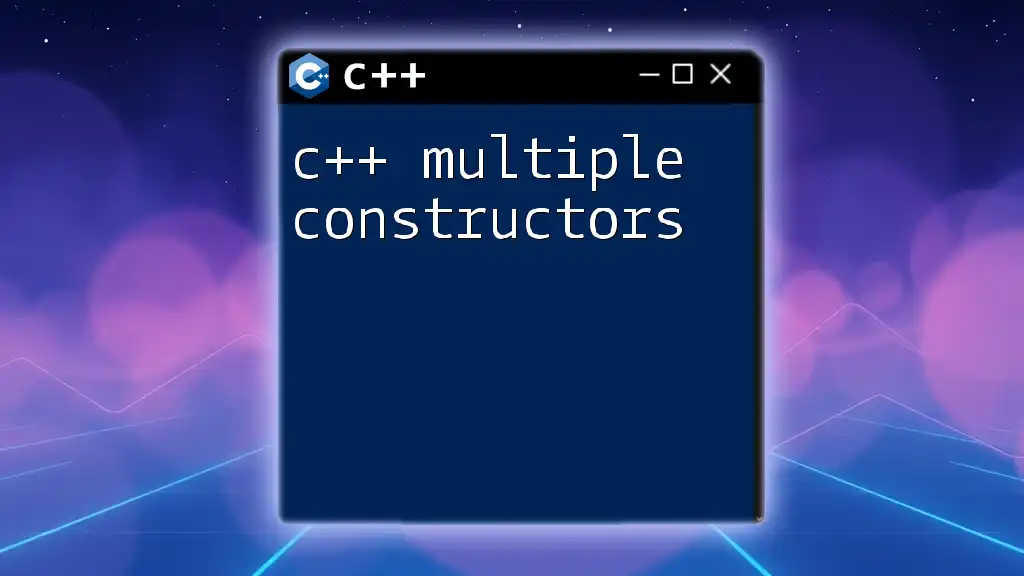
Conclusion
Understanding C++ subclass constructors is vital for effective object-oriented programming. They allow for efficient, reusable designs while ensuring proper initialization of objects in inheritance hierarchies. As you practice implementing these concepts, focus on clear design, the use of initialization lists, and the importance of constructor chaining to enhance the maintainability and readability of your code.
Explore additional resources such as books and online tutorials to deepen your understanding and keep refining your skills in using subclass constructors in C++. Practice through coding exercises will further solidify your grasp on this essential concept in C++.