An assignment constructor in C++ is a special type of constructor that initializes an object using the assignment operator, allowing for the copying of values from one object to another.
Here's a simple code snippet demonstrating an assignment constructor:
class MyClass {
public:
int value;
// Default constructor
MyClass(int val) : value(val) {}
// Assignment constructor
MyClass(const MyClass &obj) {
value = obj.value; // Copying value from obj
}
};
int main() {
MyClass obj1(10); // Creating an object with value 10
MyClass obj2 = obj1; // Using assignment constructor to copy obj1 to obj2
return 0;
}
Understanding Assignment in C++
What is an Assignment Operation?
In C++, an assignment operation happens when you assign a value to an already initialized variable. Unlike initialization, which often occurs when the object is created, assignment takes an existing object and modifies its state. Understanding this distinction is crucial because it impacts how objects behave in your programs.
The Role of the Assignment Operator
The assignment operator (`=`) is a fundamental part of C++. It allows you to transfer the values of one object to another. However, it's essential to understand that C++ performs a shallow copy by default when you use the assignment operator on objects. This means that if your object contains pointers or dynamically allocated resources, both objects will point to the same memory location, potentially leading to double deletions or memory leaks.
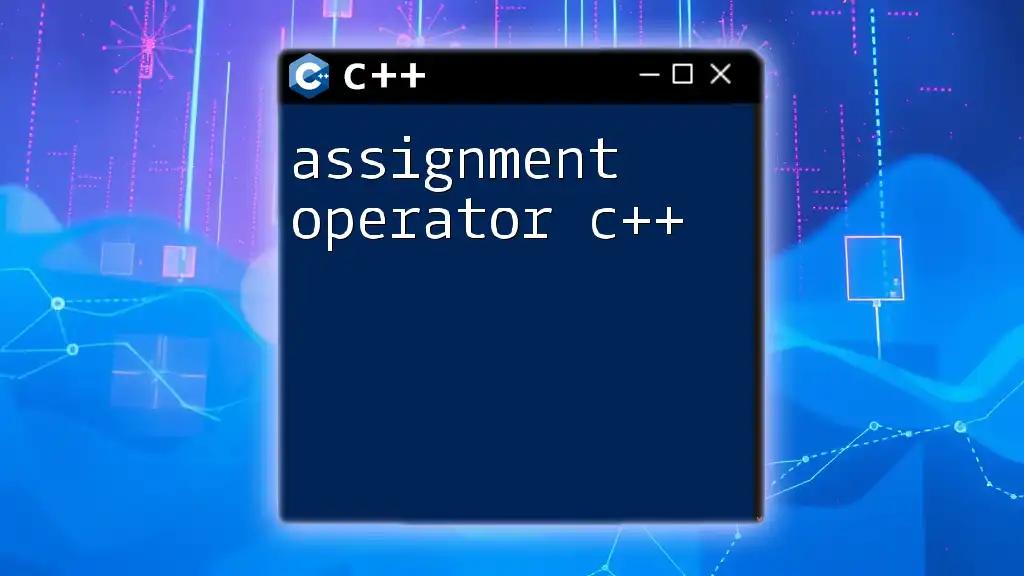
Assignment Constructor: Definition and Purpose
What is an Assignment Constructor?
An assignment constructor isn't a technically formal term in C++, but it generally refers to the way you define the assignment operator within your class. The assignment operator function is critical for defining how one object can be assigned the values of another object of the same type.
When you do not provide a custom implementation of the assignment operator, C++ provides a default assignment operator, which does a shallow copy. This can create problems, especially if your class allocates resources dynamically.
Purpose of a Custom Assignment Constructor
A custom assignment operator allows you to define how to properly copy resources when one object is assigned to another. The absence of a well-defined assignment operator can lead to subtle bugs in larger applications, especially when dealing with dynamic resource allocation.
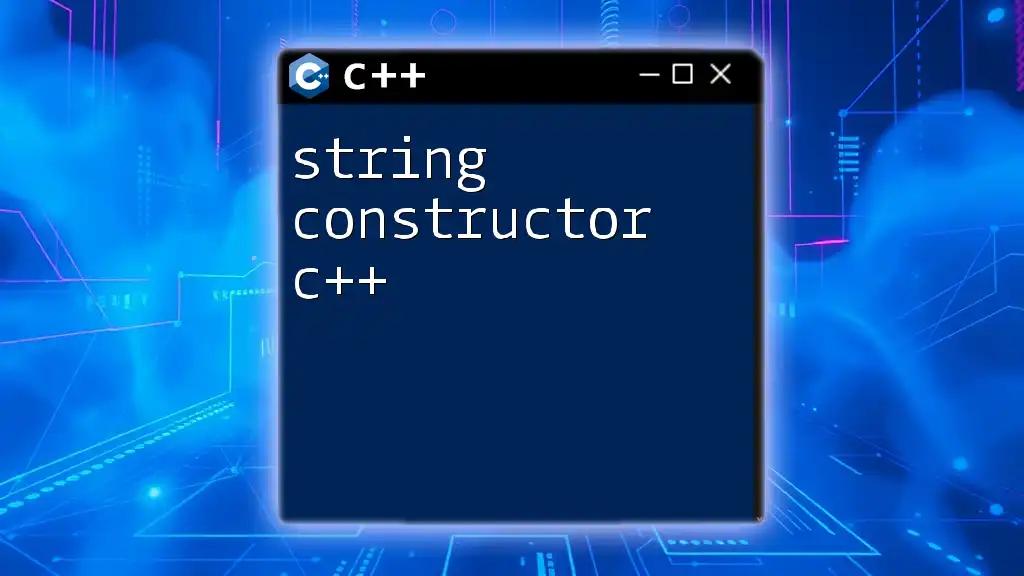
Syntax of Assignment Constructors
Basic Syntax
The syntax for declaring an assignment operator in C++ is as follows:
ClassName& operator=(const ClassName& other) {
// implementation
}
Note: Using `ClassName&` allows the function to return a reference to the current object, supporting chaining of assignments (e.g., `a = b = c`).
Returning `*this`
Returning `*this` from the assignment operator is crucial. It ensures that you return the current object rather than a copy of it. This enables the ability to chain assignments, thus making your code more readable and efficient.
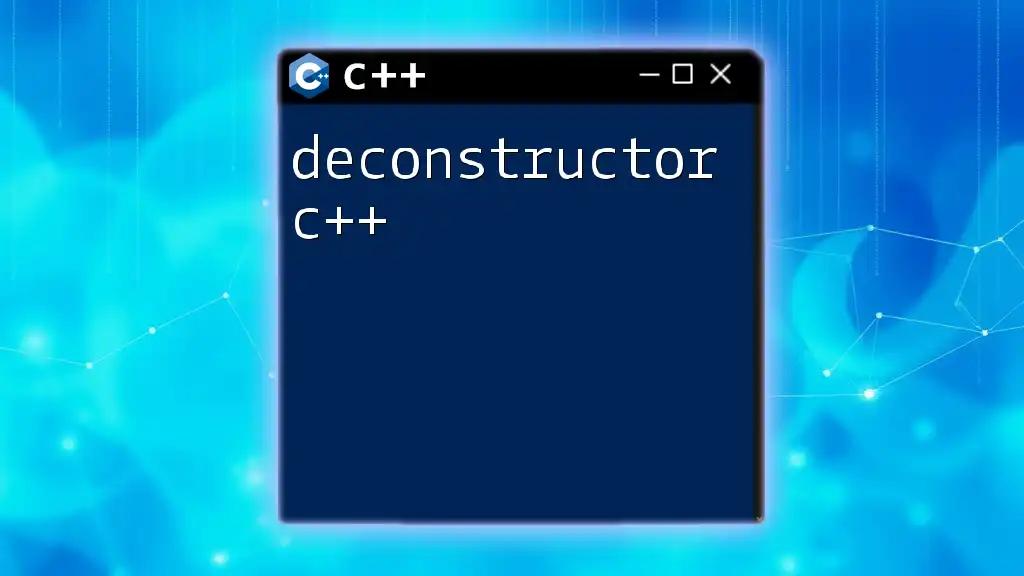
Implementing an Assignment Constructor
Step-by-Step Guide
To implement an assignment constructor, follow these steps:
- Check for self-assignment: Always check if you are attempting to assign the object to itself.
- Release existing resources if any are allocated within the object.
- Copy the contents from the `other` object appropriately.
- Return *this for chaining.
Code Example
Here's a complete example of a class with a defined assignment operator:
class MyClass {
public:
int* data;
MyClass(int value) {
data = new int(value);
}
MyClass(const MyClass& other) {
data = new int(*other.data); // Deep copy in copy constructor
}
MyClass& operator=(const MyClass& other) {
if (this != &other) { // Prevent self-assignment
delete data; // Free existing resource
data = new int(*other.data); // Deep copy
}
return *this; // Return *this to allow chaining
}
~MyClass() {
delete data; // Destructor to free dynamically allocated memory
}
};
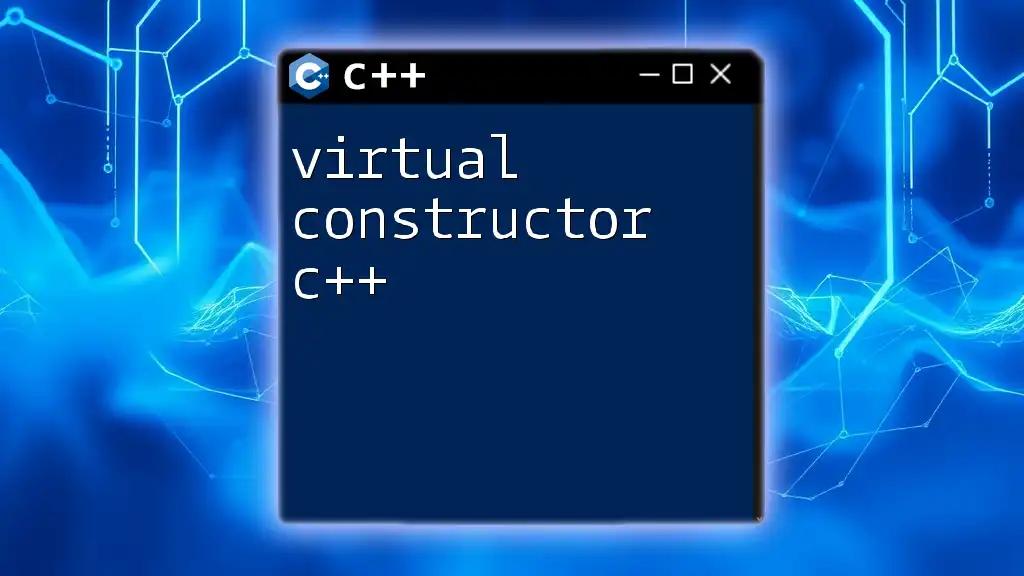
Deep Copy vs. Shallow Copy
The Concept of Shallow Copy
A shallow copy means that two objects share the same memory address for pointers. Here's a simplistic illustration:
MyClass obj1(10);
MyClass obj2 = obj1; // This uses shallow copy by default
In this example, `obj2` points to the same memory as `obj1.data`. If either object is destructed, you end up with dangling pointers.
The Concept of Deep Copy
In contrast, a deep copy duplicates the pointed-to data. This is what you implement in your assignment constructor:
MyClass& MyClass::operator=(const MyClass& other) {
if (this != &other) {
delete data;
data = new int(*other.data);
}
return *this;
}
This ensures that `obj1` and `obj2` own their separate copies of data, thus avoiding the risks of double deletion or memory corruption.

Best Practices for Assignment Constructors
Prevention of Self-Assignment
Implementing a self-assignment check is essential since assigning an object to itself can lead to undefined behavior. You can prevent this by simply adding a check at the start of your assignment operator:
if (this != &other) {
// Proceed with assignment
}
Exception Safety
Your assignment operator should be exception-safe. If an exception occurs during resource allocation (such as memory allocation failing), your program can terminate or leave your object in an inconsistent state. Employ the copy-and-swap idiom for safer assignment:
MyClass& MyClass::operator=(MyClass other) { // Pass by value
swap(*this, other); // Swap the internals
return *this;
}
Avoiding Memory Leaks
Always ensure that dynamically allocated memory is deleted before reassigning. This prevents memory leaks that can accumulate if your program runs long-term or processes large arrays.

Common Errors and Debugging Tips
Identifying and Fixing Common Mistakes
Common mistakes when implementing assignment constructors include:
- Forgetting to check for self-assignment, which leads to corrupted data.
- Not freeing previously allocated resources, causing memory leaks.
Debugging Tips
Use debugging tools like Valgrind to catch memory errors or centralize resource management within smart pointers (like `std::unique_ptr` or `std::shared_ptr`). These tools can greatly simplify managing allocations and prevent standard mistakes.
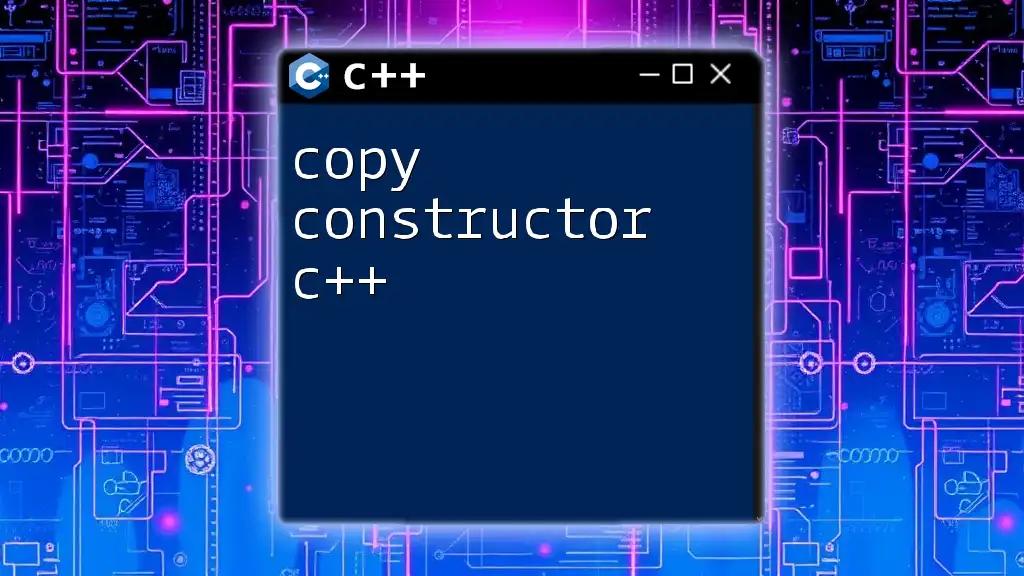
Conclusion
In this article, we explored the assignment constructor in C++, delving into its importance, implementation, and best practices. Understanding how to properly use assignment constructors is vital for writing robust and error-free C++ applications. As you continue to practice implementing assignment constructors, you'll build a firmer foundation in modern C++ programming, paving the way for more complex projects.

Additional Resources
For further reading on the intricacies of C++ and assignment constructor techniques, consider exploring foundational C++ programming books and participating in online forums where you can ask questions and engage with the community.