In C++, a class with a constructor is defined to initialize its objects with specific values upon creation, as demonstrated in the following code snippet:
class MyClass {
public:
int value;
// Constructor
MyClass(int val) {
value = val;
}
};
int main() {
MyClass obj(10); // Creates an object of MyClass and initializes value to 10
return 0;
}
Understanding the Syntax of a Class
In C++, a class is a blueprint for creating objects. It groups data (attributes) and methods (functions) that operate on that data. The basic structure of a class includes members which can be variables and functions. In C++, we also have visibility specifiers that help define access control for class members:
- Private: Members are accessible only within the class.
- Protected: Members are accessible within the class and its derived classes.
- Public: Members are accessible from anywhere within the program.
Here’s a simple example of a class definition:
class Car {
public:
std::string brand;
int year;
};
In this example, `Car` is a class with two public members: `brand` and `year`. This structure allows other parts of the program to access and modify these properties.
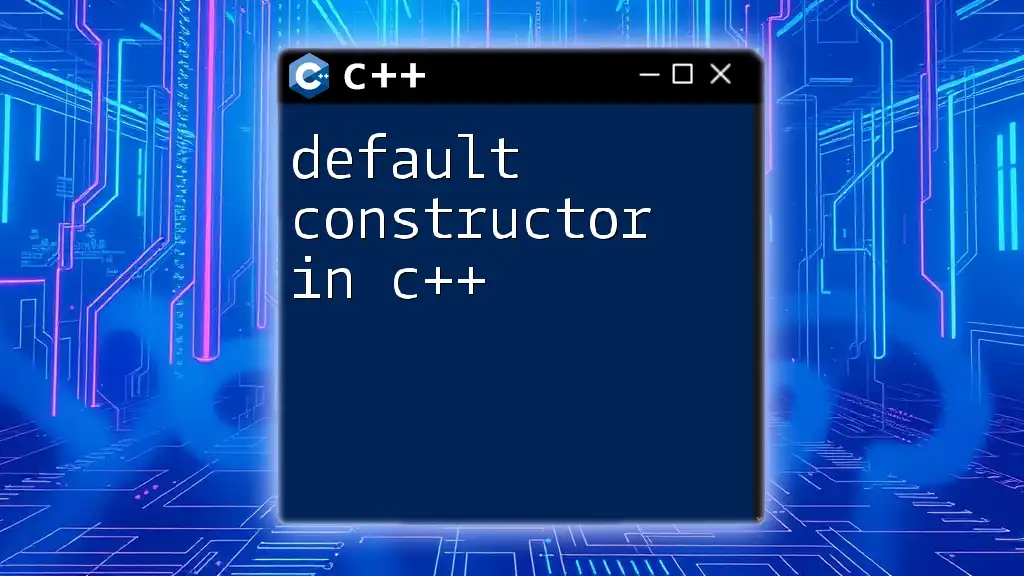
What is a Constructor?
A constructor is a special type of member function that is automatically called when an object of the class is created. Its primary role is to initialize the object’s attributes. Constructors have a few important characteristics:
- They have the same name as the class.
- They do not have a return type (not even `void`).
- A class can have multiple constructors, a feature known as constructor overloading.
Here’s an example of a default constructor:
class Car {
public:
std::string brand;
int year;
// Default constructor
Car() {
brand = "Unknown";
year = 0;
}
};
In this example, when a `Car` object is instantiated without parameters, the default constructor initializes `brand` to "Unknown" and `year` to `0`.
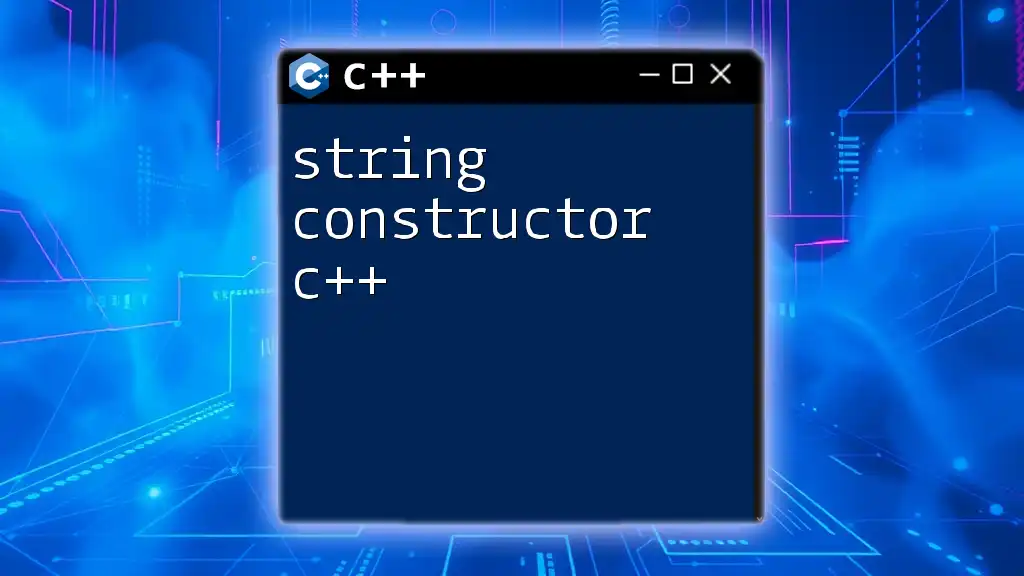
Types of Constructors
Default Constructor
A default constructor is one that does not take any arguments. It is used to create objects with default values.
Example:
Car myCar; // Calls the default constructor
Parameterized Constructor
A parameterized constructor allows you to provide initial values for the member variables when creating an object. This enables more flexibility during object creation.
Example:
class Car {
public:
std::string brand;
int year;
// Parameterized constructor
Car(std::string b, int y) {
brand = b;
year = y;
}
};
Car myCar("Toyota", 2022); // Calls the parameterized constructor
In this case, `myCar` will be initialized with `brand` set to "Toyota" and `year` set to `2022`.
Copy Constructor
A copy constructor is used to create a new object as a copy of an existing object. This is particularly useful when dealing with dynamic memory allocation and complex objects.
Example:
class Car {
public:
std::string brand;
int year;
// Copy constructor
Car(const Car &c) {
brand = c.brand;
year = c.year;
}
};
Car originalCar("Honda", 2020);
Car copiedCar = originalCar; // Calls the copy constructor
Here, `copiedCar` is created as a copy of `originalCar`, with its attributes duplicated.
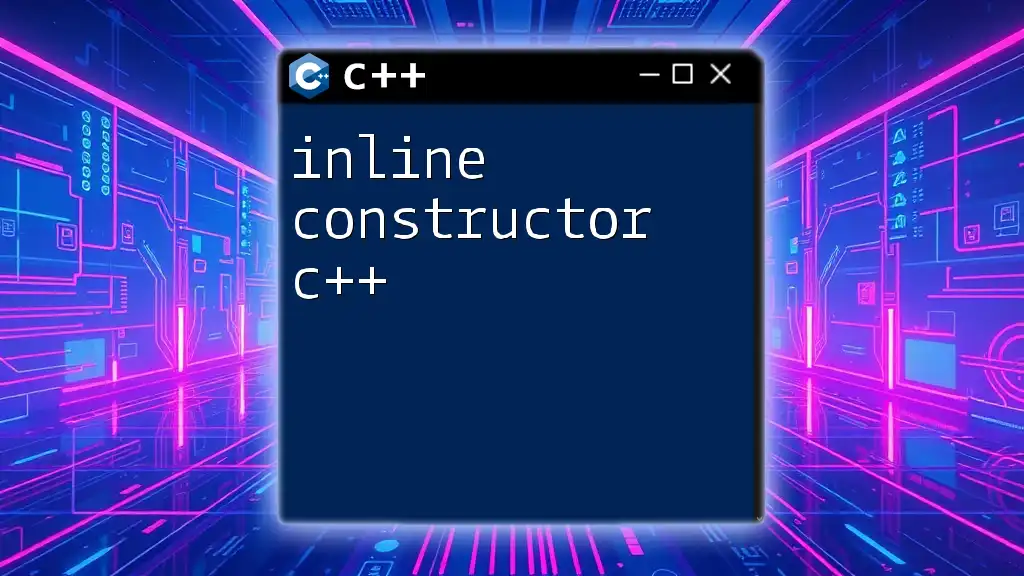
Constructor Initialization Lists
Initialization lists provide a more efficient way to initialize member variables in a constructor. They are particularly useful when dealing with constants, references, or when you want to ensure the members are initialized before the constructor body is executed.
Here’s an example:
class Car {
public:
std::string brand;
int year;
// Constructor with initialization list
Car(std::string b, int y) : brand(b), year(y) {}
};
In this case, `brand` and `year` are initialized directly in the list before the constructor body begins executing, which can improve performance, especially with complex data types.
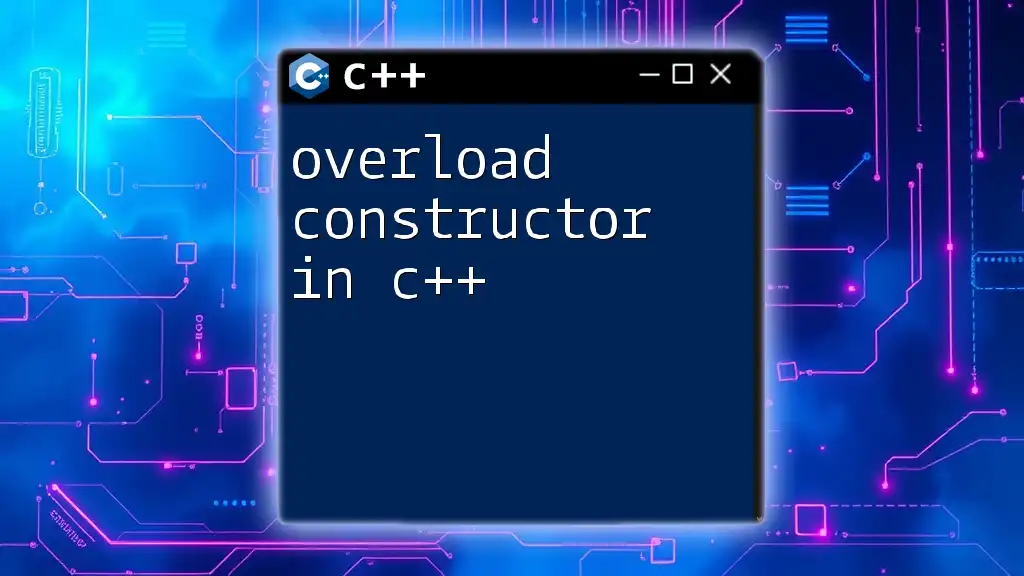
Destructor: The Counterpart of Constructor
A destructor is a special member function that is called when an object goes out of scope or is explicitly deleted. Its primary purpose is to clean up any resources that the object may have allocated during its lifetime. A destructor has the same name as the class but is prefixed with a tilde (`~`) and does not take parameters nor does it return anything.
Example:
class Car {
public:
std::string brand;
int year;
// Destructor
~Car() {
// Clean-up code can go here
}
};
Although this example doesn’t implement specific cleanup code, destructors are often used for releasing resources, such as memory or file handles, which helps in preventing memory leaks.
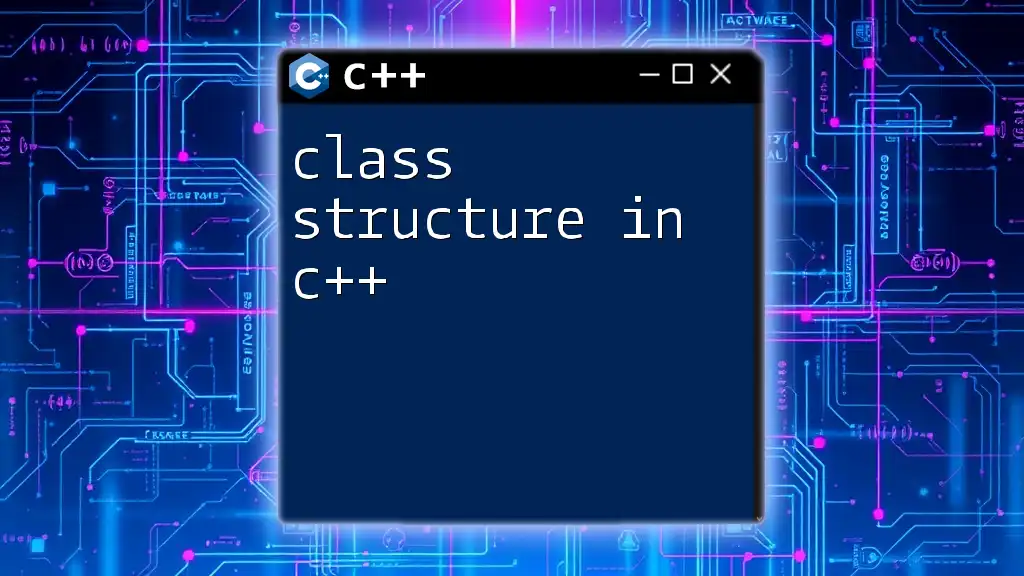
Best Practices for Using Constructors
Use of Default Constructor: While default constructors are convenient, it’s important to consider your class design. For example, avoid having a default constructor that creates invalid objects. It often makes more sense to use parameterized constructors to ensure objects are initialized correctly from the start.
Constructor Overloading: Constructor overloading is a powerful feature in C++. Having multiple constructors allows you to create objects in various states based on the requirements at the time of object creation.
Encapsulation in Constructor: Encapsulating your class members is a critical best practice. Always keep members private and expose them through public getters and setters. This not only maintains data integrity but also provides flexibility for future changes to the underlying data representation.
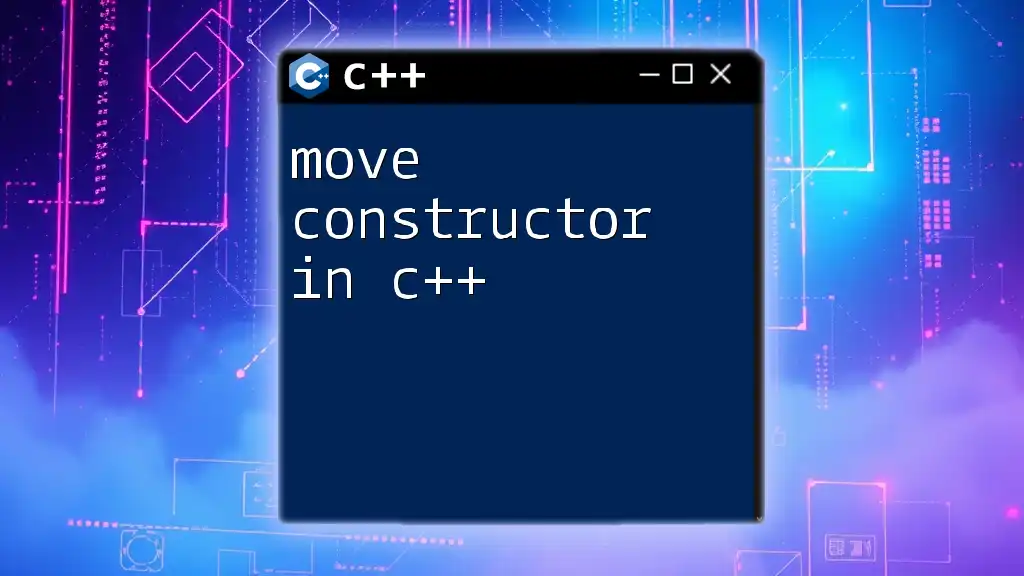
Conclusion
Constructors are an essential feature of the class with constructor in C++ paradigm, playing a crucial role in initializing class instances. By mastering constructors, you not only enhance your understanding of C++ but also improve your ability to design robust, maintainable classes. Use this knowledge to practice and experiment with various constructors in your C++ projects. Whether through default, parameterized, or copy constructors, understanding these concepts will greatly elevate your coding proficiency.