In C++, a class structure defines a blueprint for creating objects, encapsulating data and functions that operate on that data within a single entity.
class MyClass {
public:
int myNumber; // Data member
void myFunction() { // Member function
// Function implementation
}
};
Understanding Classes
What is a Class?
A class in C++ serves as a blueprint for creating objects. It encapsulates both data and functions that operate on the data, thereby promoting modularity and code reuse. Classes are essential for defining custom types in C++, allowing programmers to model real-world entities and behaviors.
Key Characteristics of classes include:
- Data Encapsulation: Classes bundle data and methods into a single unit, promoting better organization.
- Abstraction: Classes hide complex implementation details, exposing only what is necessary.
- Inheritance: Classes can inherit properties and behaviors from other classes, facilitating code reuse.
- Polymorphism: Classes can be designed to operate in multiple forms, enhancing flexibility in programming.
Components of a Class
A class comprises two primary components:
-
Attributes: These are variables that define the state of an object. For instance, in a class representing a person, attributes could include `name` and `age`.
class Person { public: string name; int age; };
-
Methods: These are functions that define the behavior of the class. For example, a method to display a person's details.
class Person { public: void displayDetails() { cout << "Name: " << name << ", Age: " << age << endl; } };

Class Structure Syntax
Basic Syntax of a Class
Understanding the syntax of a class is crucial for defining your types:
class ClassName {
public:
// Public attributes and methods
private:
// Private attributes and methods
};
In this structure:
- public: Members declared under this specifier are accessible from outside the class.
- private: Members declared here are accessible only within the class itself.
Example: Defining a Simple Class
Let's implement a simple class to exemplify the concepts discussed:
class Dog {
public:
string name;
int age;
void bark() {
cout << "Woof! My name is " << name << " and I am " << age << " years old." << endl;
}
};
In this `Dog` class, the attributes `name` and `age` define the state of each dog object, while the method `bark()` describes its behavior.
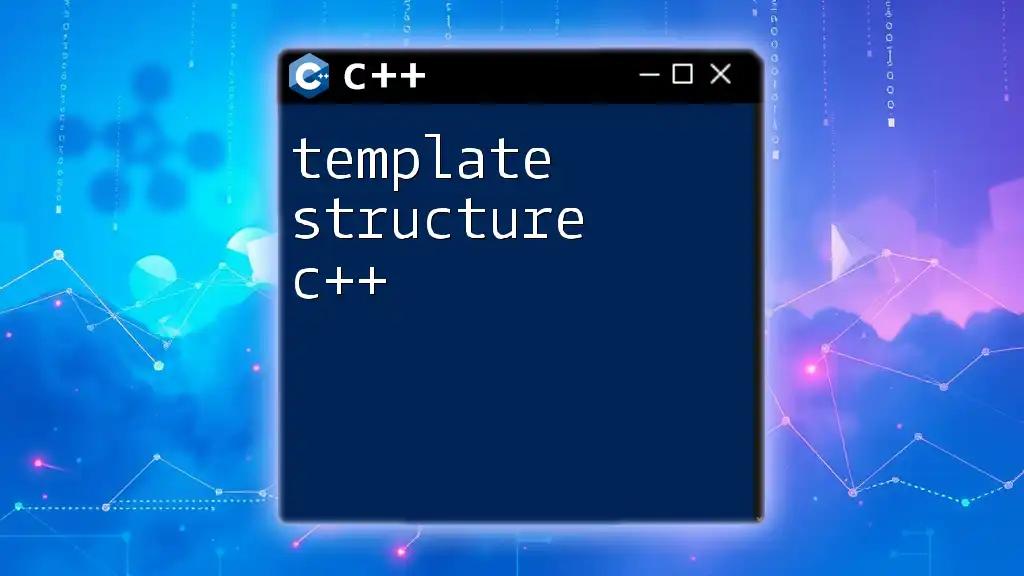
Access Specifiers in Classes
Public Access Specifier
The public access specifier allows class members to be accessed from anywhere in the program. This is vital for methods that need to be called outside the class.
class Person {
public:
string name;
};
Here, the attribute `name` can be accessed and modified from objects of the class `Person`.
Private Access Specifier
The private access specifier restricts access to the class's internal data, providing a mechanism for data hiding. This is crucial for protecting sensitive data.
class BankAccount {
private:
double balance;
public:
void deposit(double amount) {
balance += amount;
}
};
In this example, `balance` cannot be accessed directly from outside the class, ensuring that it can only be altered through controlled methods like `deposit()`.
Protected Access Specifier
The protected access specifier allows access only within the defined class and its derived classes. This is particularly useful in inheritance situations.
class Base {
protected:
int protectedValue;
};
Members of `Base` can be accessed in classes that derive from it while remaining hidden from the global scope.
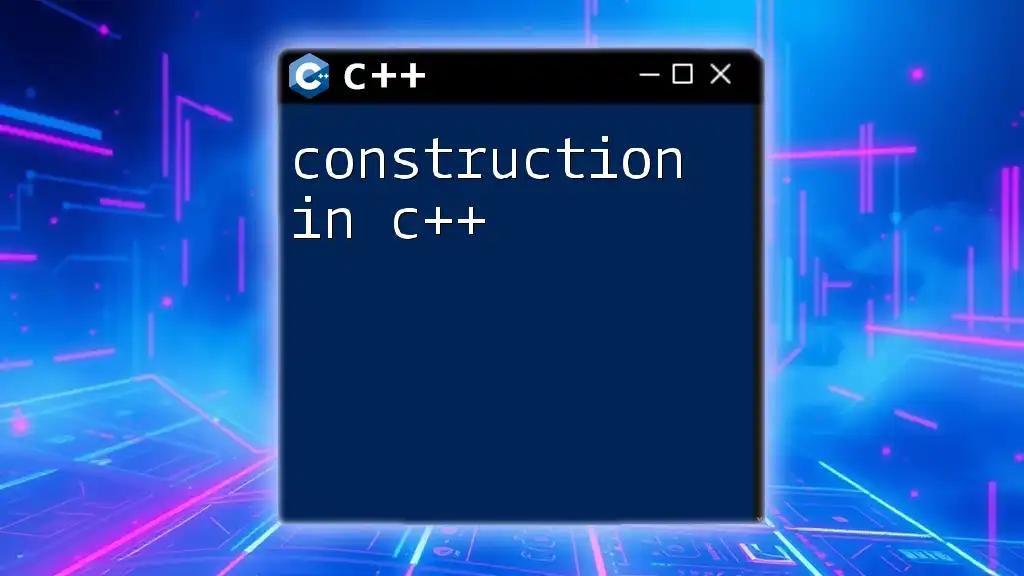
Member Functions
Defining Member Functions
Member functions are essential for defining class behaviors. They can be declared inside and outside the class.
class Car {
public:
void start() {
cout << "Car started." << endl;
}
};
In this `Car` class, `start()` is a member function that can be called on `Car` objects to execute the defined behavior.
Accessing Member Variables
Member functions can also access internal attributes to affect the state of an object.
class Employee {
private:
string name;
public:
void setName(string empName) {
name = empName;
}
void showName() {
cout << "Employee Name: " << name << endl;
}
};
In the `Employee` class, `setName` allows external code to modify the private variable `name`, while `showName` provides a way to access it.
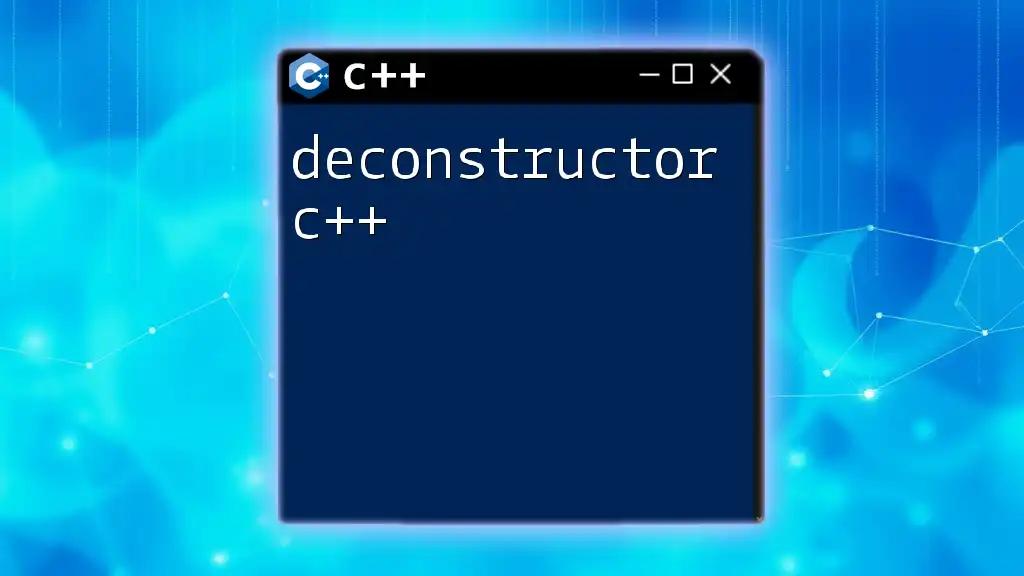
Constructors and Destructors
What is a Constructor?
Constructors are special member functions that are automatically called when an object is created. They initialize the object's attributes.
class Book {
public:
string title;
Book(string bookTitle) {
title = bookTitle;
}
};
In this `Book` class, the constructor accepts a parameter `bookTitle` and initializes the `title` attribute.
What is a Destructor?
Destructors are executed when an object goes out of scope or is explicitly deleted. They help to free resources.
class Resource {
public:
Resource() {
cout << "Resource allocated." << endl;
}
~Resource() {
cout << "Resource deallocated." << endl;
}
};
Here, the `Resource` class allocates resources upon creation and cleans them up when the object is destroyed, ensuring efficient resource management.
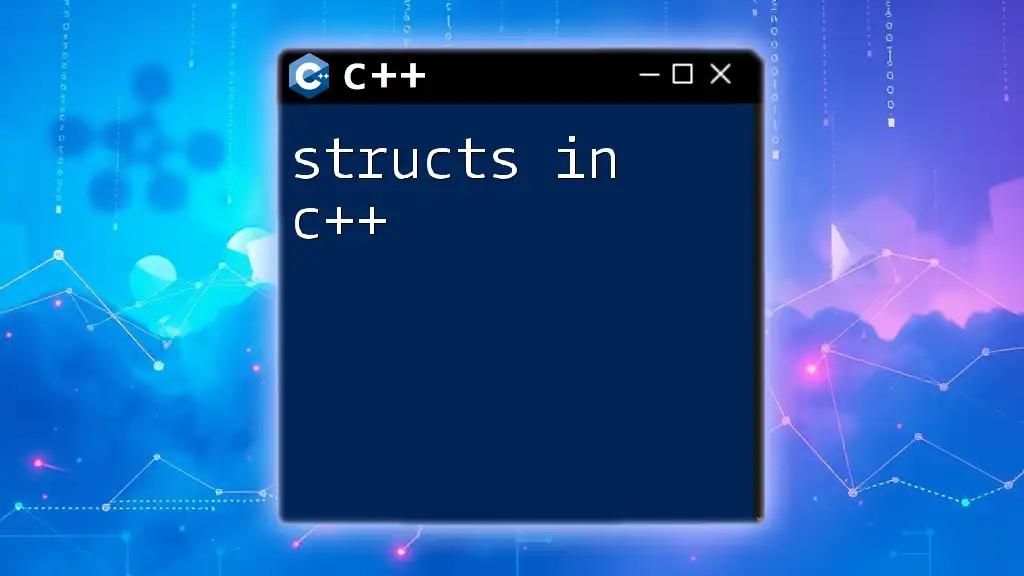
Inheritance and Class Hierarchies
Introduction to Inheritance
Inheritance allows classes to derive properties and methods from other classes, creating a hierarchy. This is a powerful feature of C++ that encourages code reuse and logical class organization.
Example of Inheritance
class Animal {
public:
void eat() {
cout << "Eating..." << endl;
}
};
class Dog : public Animal {
public:
void bark() {
cout << "Barking!" << endl;
}
};
In this example, `Dog` inherits from `Animal`, allowing it to access the `eat` method while also providing its own behavior through the `bark` method.

Class Composition
What is Class Composition?
Class composition involves building complex classes from simpler ones. Instead of inheriting, a class can contain instances of other classes as members. This promotes better organization by combining distinct functionalities.
Example of Class Composition
class Engine {
public:
void start() {
cout << "Engine started." << endl;
}
};
class Car {
private:
Engine engine;
public:
void startCar() {
engine.start();
cout << "Car is running." << endl;
}
};
In this `Car` class, an instance of `Engine` is included, allowing the `Car` to utilize its features without the complexity of inheritance.
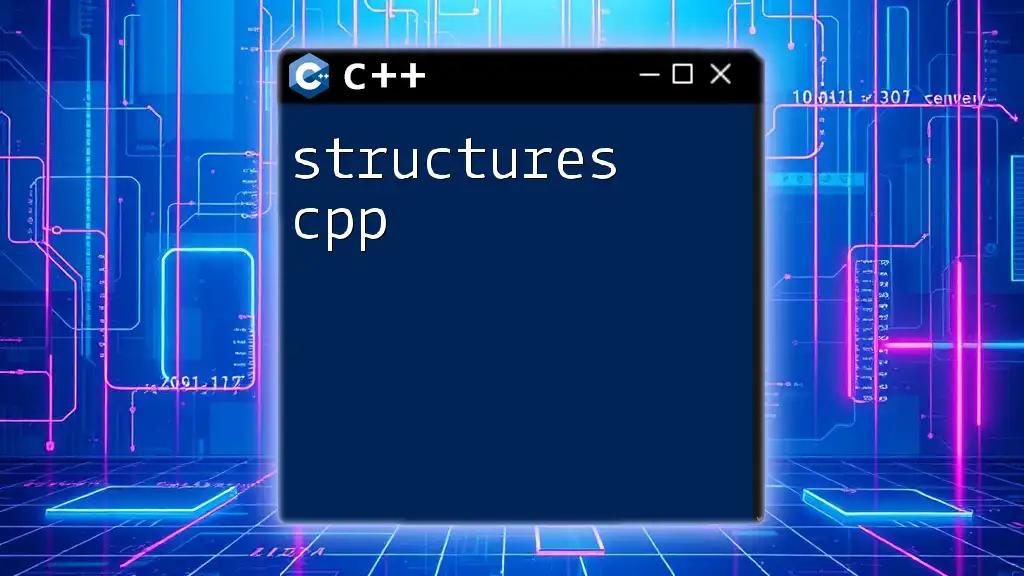
Conclusion
Mastering the class structure in C++ is fundamental for effective programming in this powerful language. By understanding components like member functions, constructors, destructors, and the use of inheritance and composition, you can create well-organized, efficient, and reusable code. These concepts not only improve your programming skills but also promote best practices in software development. Continue to explore and experiment with class structures to unlock the full potential of C++.

Additional Resources
To enhance your understanding of class structures in C++, consider exploring recommended books, online courses, and practice platforms where you can sharpen your skills further.