Access specifiers in C++ control the visibility and accessibility of class members, with the primary specifiers being `public`, `private`, and `protected`.
Here’s a code snippet demonstrating the use of access specifiers:
class Example {
public:
int publicVar; // Accessible from anywhere
private:
int privateVar; // Accessible only within this class
protected:
int protectedVar; // Accessible within this class and derived classes
};
Understanding the Basics of Access Specifiers
What are Access Specifiers?
Access specifiers are keywords used in C++ to specify the accessibility or visibility of class members (attributes and methods). They control how and where these members can be accessed from other code. Access specifiers are fundamental to the principles of encapsulation and data protection in object-oriented programming, allowing developers to maintain control over the state and behavior of objects.
Types of Access Specifiers
In C++, there are three main types of access specifiers that dictate the visibility of class members:
- Public: Members declared as public can be accessed from any part of the program.
- Protected: Members declared as protected can be accessed within the class itself and by derived classes, but not by other parts of the program.
- Private: Members declared as private are only accessible within the class itself, protecting them from outside interference.
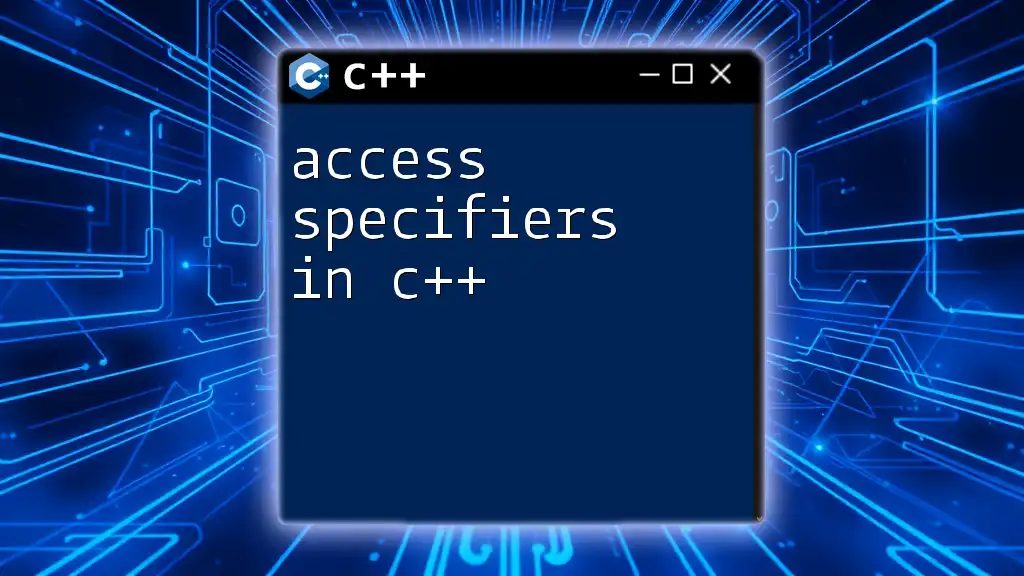
Public Access Specifier
Definition and Characteristics
The public access specifier allows members of a class to be accessed from any part of the program. This includes external functions, other classes, or derived classes. When a member is declared as public, there are no restrictions on how it can be accessed.
Here's an example demonstrating a public member:
class Example {
public:
int publicValue;
void publicMethod() {
// Public method implementation
}
};
int main() {
Example obj;
obj.publicValue = 10; // Accessing public member
obj.publicMethod(); // Calling public method
return 0;
}
Use Cases for Public Members
Public members are crucial when you want certain attributes or methods to be universally accessible. For instance, in libraries, if you create a class for a mathematical operation like addition, the method to perform the addition should be public so that users of the library can call it directly without restrictions. Ensuring your API is friendly often involves exposing public methods that users can interact with directly.
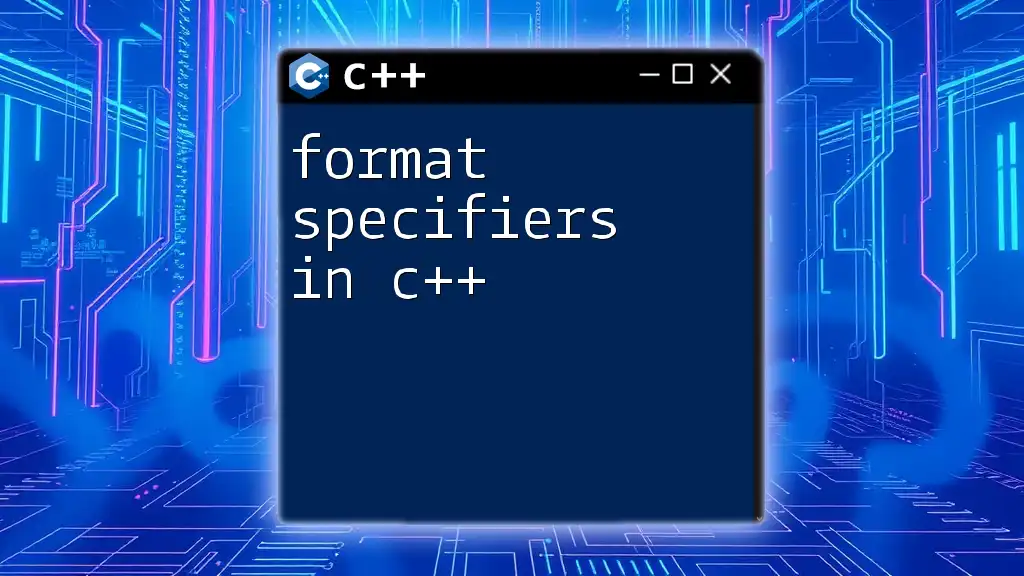
Protected Access Specifier
Definition and Characteristics
The protected access specifier allows members to be accessed within the class itself and by derived classes. However, it does not permit access from non-derived classes. This is useful in scenarios where you want to allow subclasses to utilize certain data members while keeping them hidden from the rest of the program.
Here's an example demonstrating a protected member:
class Base {
protected:
int protectedValue;
};
class Derived : public Base {
public:
void derivedMethod() {
protectedValue = 5; // Accessible in derived class
}
};
Use Cases for Protected Members
The protected access specifier is helpful in inheritance scenarios. For instance, if you have a base class that defines common attributes which should be shared with subclasses but hidden from the rest of the program, using protected members can provide that functionality. It is often seen in frameworks, where you'd want to expose certain behaviors in subclasses while maintaining a level of security and encapsulation in the base class.
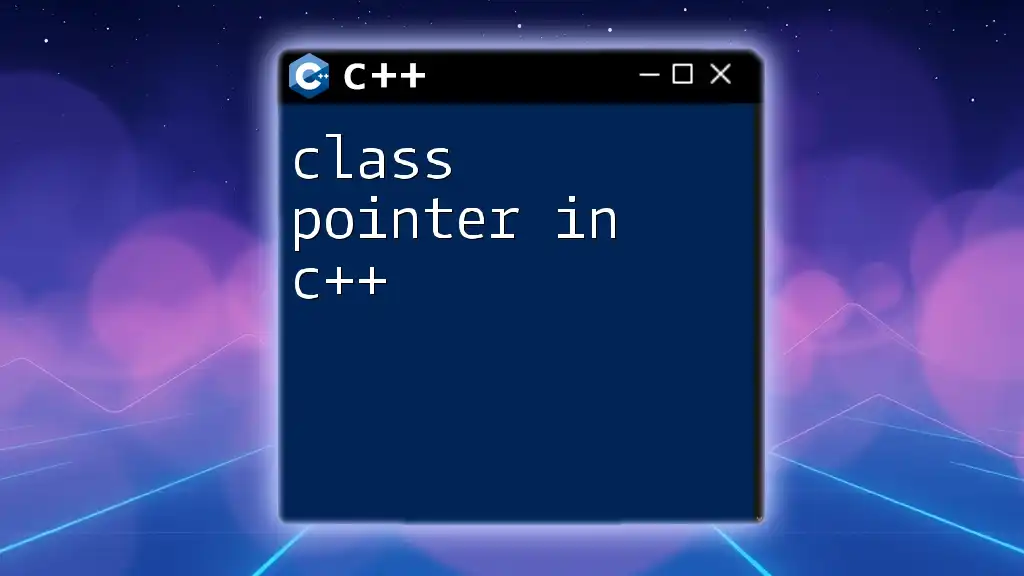
Private Access Specifier
Definition and Characteristics
Private access specifiers restrict access to members of a class, meaning they are only accessible within the class itself. This is one of the primary tools used for encapsulation, allowing developers to hide internal representation and expose only necessary information through public methods.
Here's a code snippet demonstrating a private member:
class Example {
private:
int privateValue;
public:
void setPrivateValue(int value) {
privateValue = value; // Setting value through public method
}
int getPrivateValue() {
return privateValue; // Accessing private member through public method
}
};
int main() {
Example obj;
obj.setPrivateValue(10); // Accessible through public method
int value = obj.getPrivateValue(); // Fetching the private value
return 0;
}
Use Cases for Private Members
Using private members is essential for protecting an object's internal state. For instance, if you have a class that represents a bank account, you would likely keep balance management private and provide public methods to deposit or withdraw funds. This ensures the balance cannot be modified directly from outside the class, helping to maintain data integrity and consistency.
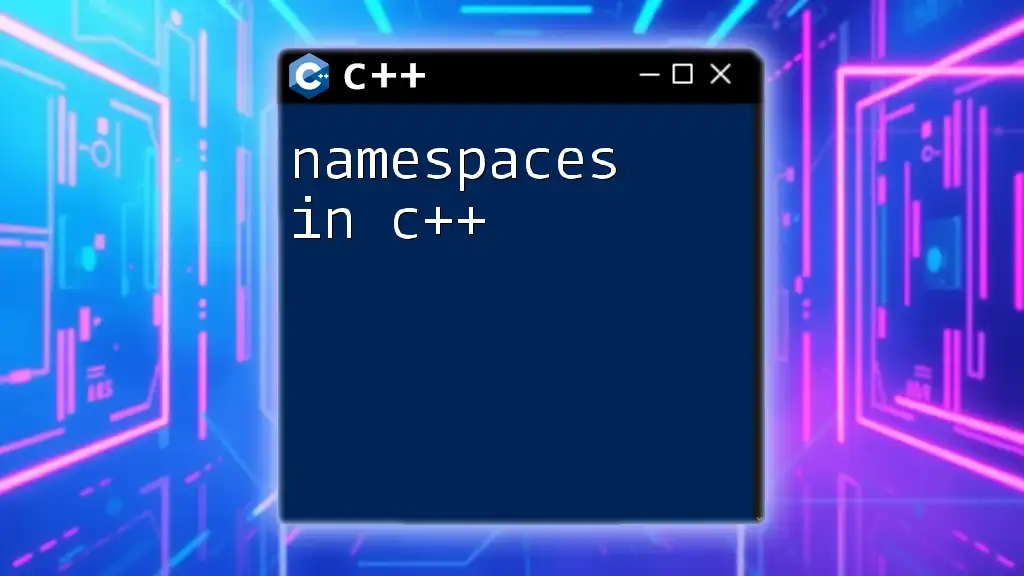
Access Specifier Summary
Comparison of Access Specifiers
To encapsulate the key differences between the access specifiers:
- Public: Accessible from anywhere.
- Protected: Accessible only within the class and derived classes.
- Private: Accessible only within the class itself.
Each access specifier serves a unique purpose depending on the design requirements of your program and enhances the overall security and robustness.
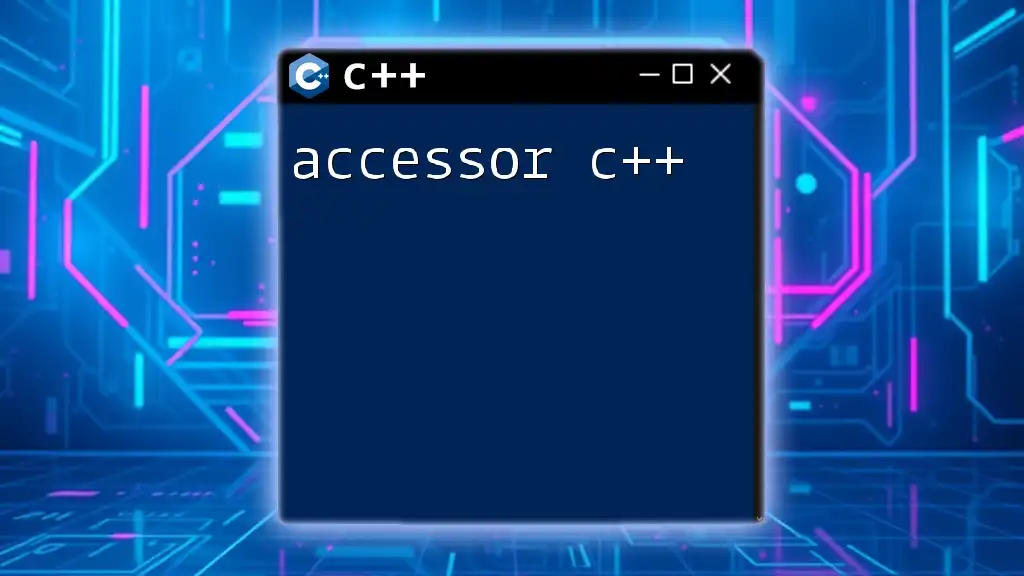
Best Practices in Using Access Specifiers
General Guidelines
When deciding which access specifier to use, consider the role of the member in your class. Use public for members that need to be exposed to users, protected for shared attributes among subclasses, and private for internal workings of the class. This thoughtful consideration helps maintain a clean and manageable codebase.
Importance of Encapsulation
Access specifiers play a vital role in encapsulation, ensuring that a class can control how its data is accessed and modified. This control helps prevent unintended interference, providing a solid foundation for reliable software. The use of strict access rules simplifies debugging, enhances security, and promotes better software design principles.
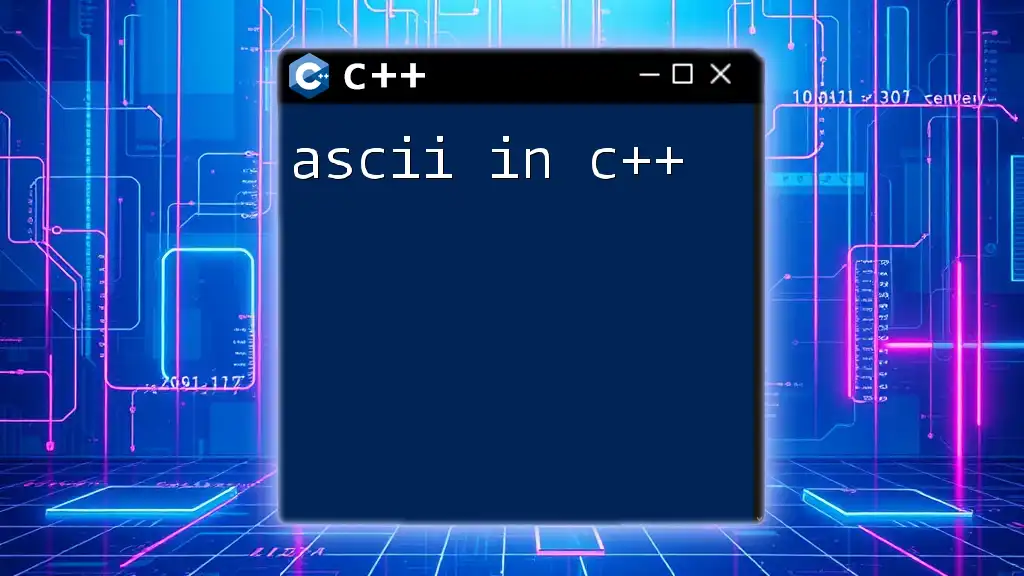
Conclusion
Access specifiers are fundamental in C++ programming, allowing developers to control visibility and access to class members effectively. Understanding the distinctions and appropriate use of public, protected, and private members will strengthen your programming skills and contribute to better software design. As you continue your journey in learning C++, embrace the concepts of encapsulation and carefully apply access specifiers to create robust and secure applications.
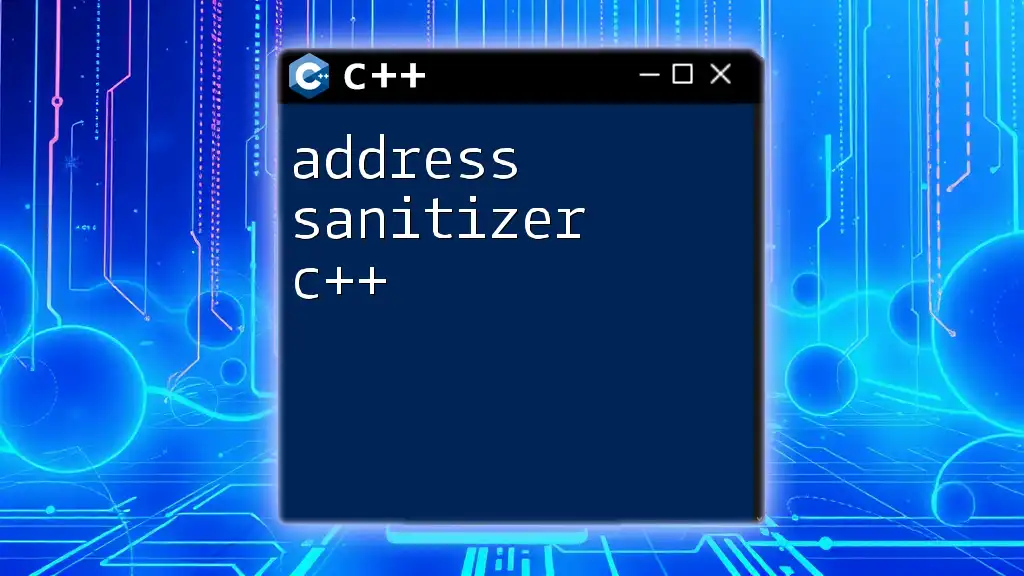
Additional Resources
Explore further readings and tutorials on C++ to deepen your understanding of access specifiers and object-oriented programming principles. Join online communities and forums to connect with fellow learners and gain diverse insights into mastering C++. Consider enrolling in courses designed for practical application in C++ to solidify your knowledge and improve your programming capabilities.