The Fibonacci series in C++ generates a sequence where each number is the sum of the two preceding ones, starting from 0 and 1, which can be implemented using a simple loop.
#include <iostream>
using namespace std;
int main() {
int n = 10, t1 = 0, t2 = 1, nextTerm;
cout << "Fibonacci Series: " << t1 << ", " << t2 << ", ";
for (int i = 3; i <= n; ++i) {
nextTerm = t1 + t2;
cout << nextTerm << ", ";
t1 = t2;
t2 = nextTerm;
}
return 0;
}
Understanding Fibonacci Numbers in C++
The Fibonacci series consists of numbers where each number is the sum of the two preceding ones, usually starting with 0 and 1. In mathematical terms, this series can be defined by the recurrence relation:
- F(0) = 0,
- F(1) = 1,
- F(n) = F(n - 1) + F(n - 2) for n > 1.
The first several Fibonacci numbers are: 0, 1, 1, 2, 3, 5, 8, 13, 21, ....
Fibonacci numbers have a variety of applications, from algorithm design to modeling phenomena in biology and art.
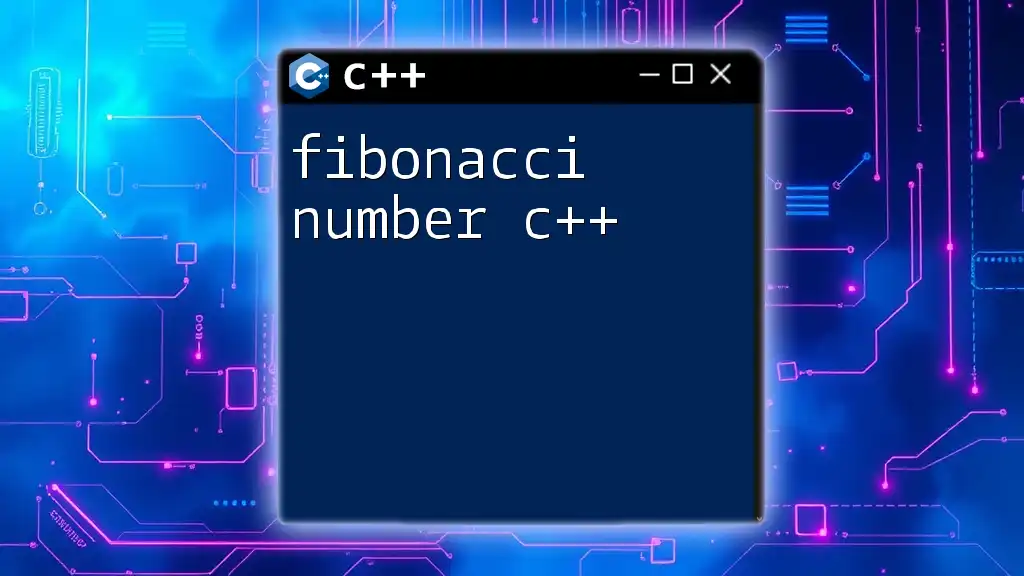
Overview of Fibonacci Series in C++
Learning to generate the fibonacci series in C++ equips you with essential programming skills as it demonstrates fundamental concepts such as loops, recursion, and optimization techniques. Understanding different methods for generating Fibonacci numbers can help develop problem-solving strategies for a variety of programming challenges.
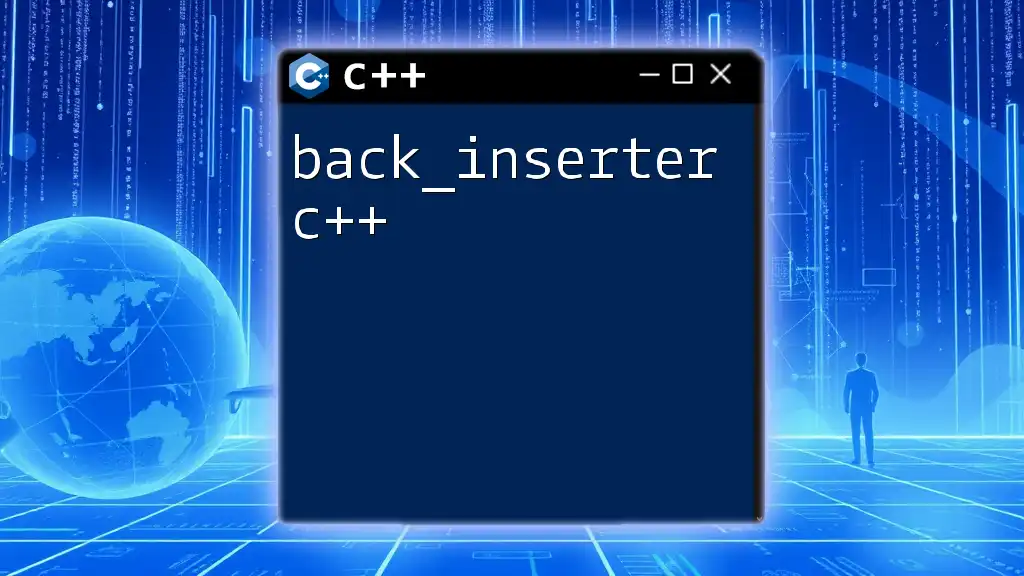
Methods to Generate Fibonacci Series in C++
Iterative Approach
The iterative method is a straightforward way to generate Fibonacci numbers. This approach efficiently calculates each Fibonacci number in a loop, making it easy to understand and implement.
Here's a simple example of generating Fibonacci numbers iteratively:
#include <iostream>
void fibonacci_iterative(int n) {
int a = 0, b = 1, c;
if (n <= 0)
return;
std::cout << a << " ";
for (int i = 1; i < n; ++i) {
std::cout << b << " ";
c = a + b;
a = b;
b = c;
}
}
int main() {
int n = 10; // Change this value for more numbers
fibonacci_iterative(n);
return 0;
}
In this code, we define a function `fibonacci_iterative(int n)` that takes an integer n and prints the first n Fibonacci numbers. The variables `a` and `b` store the last two Fibonacci numbers, and the loop continues until we reach the desired number of terms.
Pros:
- Simple and easy to understand
- Efficient in time complexity (O(n))
Cons:
- Requires linear space for maintaining state variables.
Recursive Approach
The recursive method relies on the mathematical definition of Fibonacci numbers, making it conceptually elegant. However, it can be inefficient due to repeated calculations.
Here's an example:
#include <iostream>
int fibonacci_recursive(int n) {
if (n <= 1)
return n;
return fibonacci_recursive(n - 1) + fibonacci_recursive(n - 2);
}
int main() {
int n = 10; // Change this value for nth Fibonacci number
for (int i = 0; i < n; ++i) {
std::cout << fibonacci_recursive(i) << " ";
}
return 0;
}
In this example, the function `fibonacci_recursive(int n)` computes the Fibonacci number for a given n using recursion. As each call breaks the problem into smaller subproblems, it returns the sum of the two preceding Fibonacci numbers.
Pros:
- Conceptually straightforward and matches mathematical definition.
Cons:
- Exponential time complexity (O(2^n)), which can lead to a significant number of repeated calculations, making it impractical for larger n.
Using Dynamic Programming
To optimize the recursive approach, we can use dynamic programming, which stores previously computed Fibonacci numbers to avoid redundant work, achieving linear time complexity.
Here’s how it can be implemented:
#include <iostream>
#include <vector>
void fibonacci_dynamic(int n) {
std::vector<int> fib(n);
fib[0] = 0;
fib[1] = 1;
for (int i = 2; i < n; ++i) {
fib[i] = fib[i - 1] + fib[i - 2];
}
for (int i = 0; i < n; ++i) {
std::cout << fib[i] << " ";
}
}
int main() {
int n = 10; // Change this value for more numbers
fibonacci_dynamic(n);
return 0;
}
In this code, we use a `std::vector` to store Fibonacci numbers as they are computed. This enables efficient access to previously calculated values, dramatically improving performance.
Pros:
- Efficient with O(n) time complexity
- Avoids redundancy in calculations
Cons:
- Requires additional space for storage.
Using Matrix Exponentiation
A powerful and less common method for calculating the Fibonacci numbers is matrix exponentiation. This technique uses the property of matrices to compute Fibonacci numbers in logarithmic time complexity.
Here’s an implementation:
#include <iostream>
#include <vector>
void multiply(std::vector<std::vector<int>> &F, std::vector<std::vector<int>> &M) {
int x = F[0][0] * M[0][0] + F[0][1] * M[1][0];
int y = F[0][0] * M[0][1] + F[0][1] * M[1][1];
int z = F[1][0] * M[0][0] + F[1][1] * M[1][0];
int w = F[1][0] * M[0][1] + F[1][1] * M[1][1];
F[0][0] = x;
F[0][1] = y;
F[1][0] = z;
F[1][1] = w;
}
void power(std::vector<std::vector<int>> &F, int n) {
if (n == 0 || n == 1)
return;
std::vector<std::vector<int>> M = { {1, 1}, {1, 0} };
power(F, n / 2);
multiply(F, F);
if (n % 2 != 0)
multiply(F, M);
}
int fibonacci_matrix(int n) {
std::vector<std::vector<int>> F = { {1, 1}, {1, 0} };
if (n == 0)
return 0;
power(F, n - 1);
return F[0][0];
}
int main() {
int n = 10; // Change this value for nth Fibonacci number
std::cout << fibonacci_matrix(n) << std::endl;
return 0;
}
In this example, we use a two-dimensional vector to represent the transformation matrix. The `power` function raises the matrix to the n - 1 power, and then `F[0][0]` will hold the nth Fibonacci number.
Pros:
- Efficient with O(log n) time complexity
- Reduces space usage significantly
Cons:
- More complicated to implement and understand.
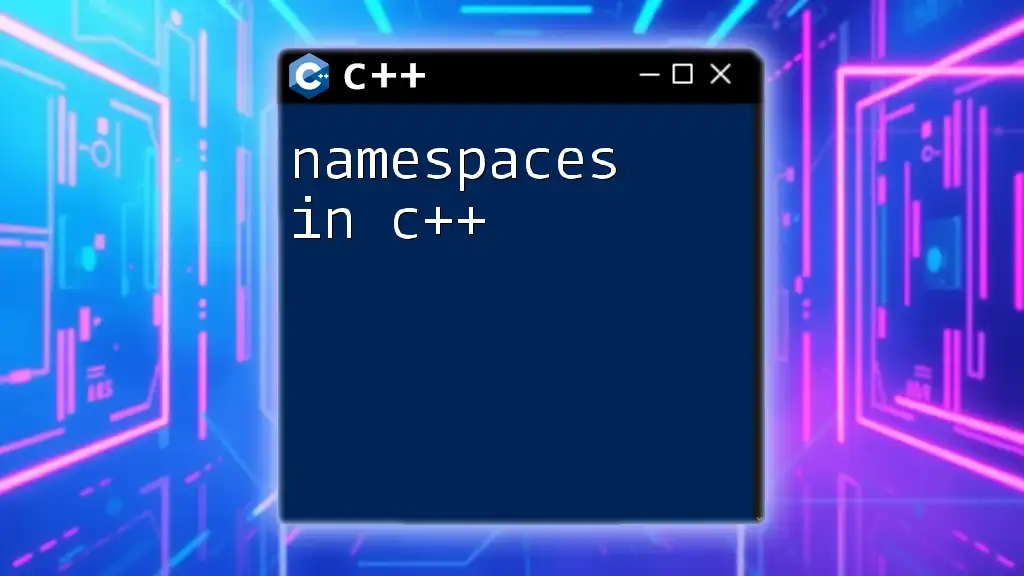
Comparing Approaches
When comparing these methods, the iterative approach stands out for its simplicity and efficiency in terms of both time and space. The recursive solution can be elegant but is largely impractical for larger Fibonacci numbers due to its exponential time complexity. Dynamic programming balances efficiency and simplicity, making it ideal for most use cases. Finally, matrix exponentiation offers a powerful alternative with logarithmic time complexity, though it requires a deeper understanding of mathematical concepts.
- Iterative: O(n) time complexity, O(1) space complexity
- Recursive: O(2^n) time complexity, O(n) space complexity
- Dynamic Programming: O(n) time complexity, O(n) space complexity
- Matrix Exponentiation: O(log n) time complexity, O(1) space complexity
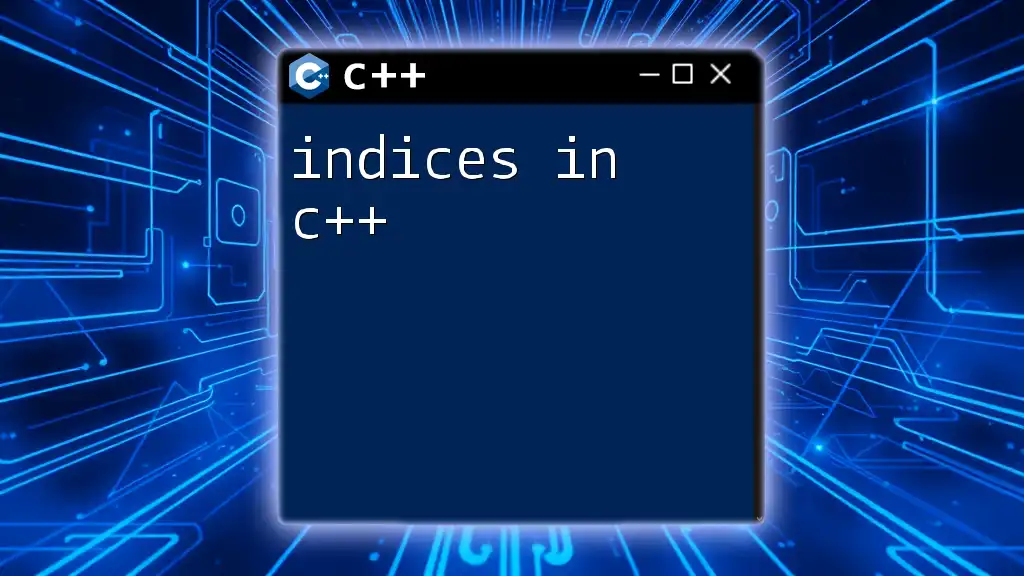
Conclusion
The fibonacci series in C++ is an excellent example to explore various programming techniques, from basic loops to advanced algorithms like matrix exponentiation. Each method has its unique merits and drawbacks, emphasizing the importance of context and efficiency when choosing a solution. By experimenting with these approaches, you can deepen your understanding of C++ and its capabilities. Feel free to explore and share your experiences with Fibonacci numbers in the world of programming!
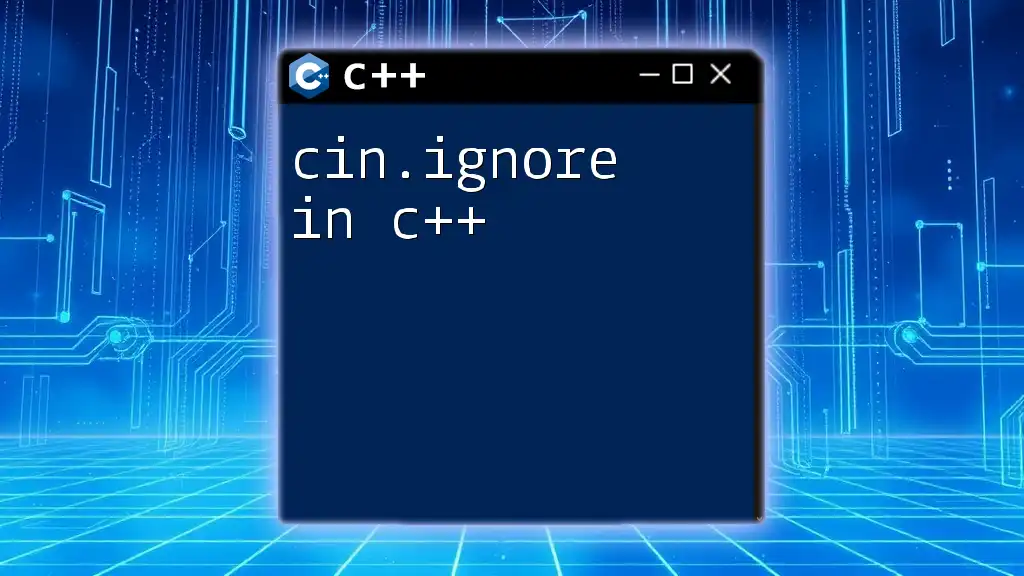
Additional Resources
For further reading and practice, consider looking for C++ references online, use online compilers to experiment with the code, or jump into more advanced topics on algorithms and data structures.