In C++, indices are used to access elements in arrays or vectors, with the first element starting at index 0.
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {10, 20, 30, 40, 50};
std::cout << "The first element is: " << numbers[0] << std::endl; // Accessing the element at index 0
return 0;
}
Understanding Indices in C++
What Are Indices?
Indices are integral to programming, allowing developers to access and manipulate data structures like arrays, vectors, and strings. They serve as pointers to individual elements within these structures. Understanding how to use indices effectively in C++ is crucial for operating on data collections efficiently and correctly.
Types of Indices
When dealing with indices, it's essential to recognize the two predominant styles of indexing:
Zero-Based Indexing: This is the default system used in C++. In zero-based indexing, the first element of a data structure begins at index 0. For instance, if you have an array `arr`, the first element is accessed as `arr[0]`.
One-Based Indexing: Although less common in C++, it's important to mention this as some programming languages (like MATLAB) utilize this system. In one-based indexing, the first element is accessed as `arr[1]`.

Using Indices with Arrays
Introduction to Arrays
An array is a collection of elements of the same type stored in contiguous memory locations. In C++, arrays provide a way to store and manage data efficiently.
Accessing Array Elements
Accessing array elements in C++ is straightforward. The syntax involves the array name followed by the index in square brackets.
Example of Accessing Array Elements:
int arr[] = {10, 20, 30, 40, 50};
cout << arr[2]; // Output: 30
In this example, the value at index 2 is accessed, which corresponds to the third element of the array (since indexing starts at 0).
Modifying Array Elements
Updating array elements using indices is just as simple as accessing them. You can assign a new value to a specific index.
Example of Modifying Array Elements:
arr[2] = 35; // Updating the third element
In this example, we change the value of the third element from 30 to 35.
Multi-Dimensional Arrays
Understanding Multi-Dimensional Arrays
Multi-dimensional arrays are essentially arrays of arrays, allowing you to store data in more than one dimension. This is particularly useful for representing matrices or grids.
Accessing Multi-Dimensional Arrays
Accessing elements in a multi-dimensional array requires multiple indices.
Example of Accessing a 2D Array:
int matrix[3][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
cout << matrix[1][2]; // Output: 6
In this case, `matrix[1][2]` accesses the element located in the second row and third column of the matrix.
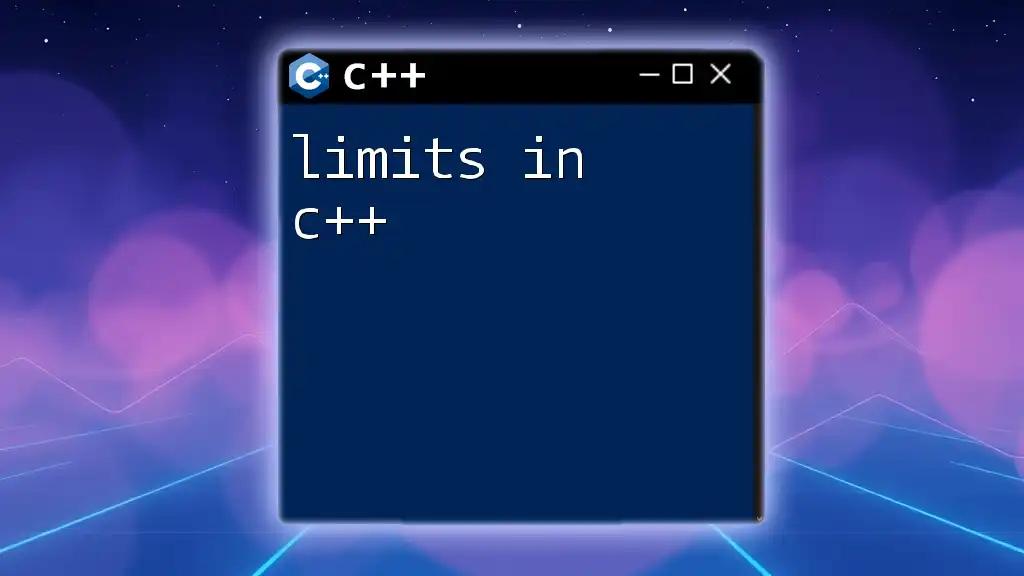
Using Indices with Vectors
Introduction to Vectors
Vectors are a dynamic array type provided by the C++ Standard Library. They can adjust their size automatically, making them more flexible than static arrays.
Accessing Vector Elements
Accessing vector elements is similar to arrays but with additional advantages such as dynamic size management.
Example of Accessing Vector Elements:
vector<int> vec = {1, 2, 3, 4, 5};
cout << vec[0]; // Output: 1
Here, we access the first element of the vector.
Modifying Vector Elements
Updating elements in a vector works the same way as in arrays.
Example of Modifying Vector Elements:
vec[1] = 10; // Update the second element
This line changes the second element from 2 to 10.
Using the `at()` Method
While the `[]` operator is common for accessing elements, C++ also provides the `at()` method. This method performs bounds checking and can throw exceptions if the index is out of range, making it a safer choice.
Example of Using `at()`:
cout << vec.at(2); // Output: 3
In this instance, `at(2)` access the third element just like `vec[2]`, but with safety against out-of-bounds errors.
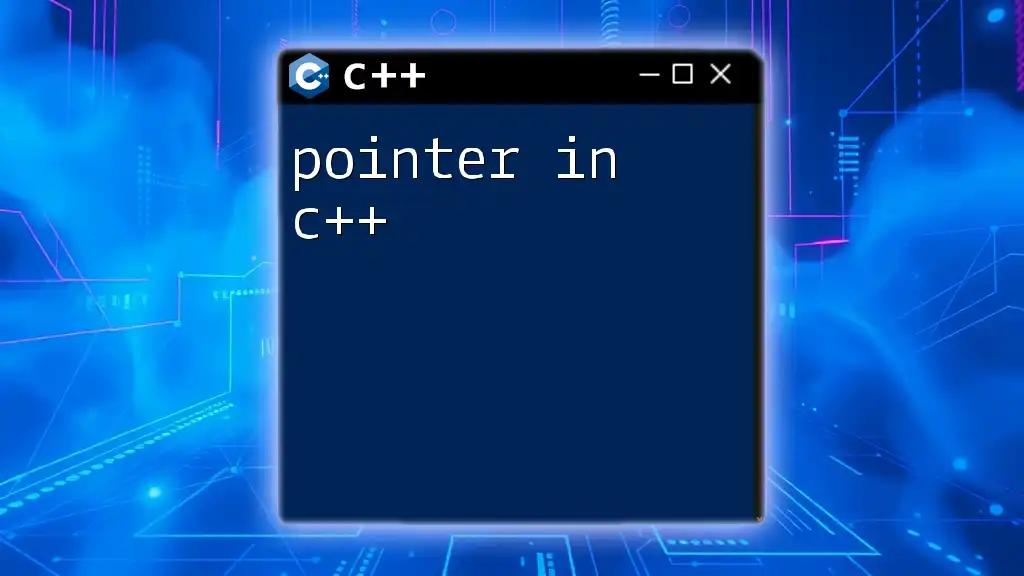
Indices in Strings
Understanding Strings in C++
In C++, strings are objects of the `std::string` class, which encapsulates character arrays. Unlike character arrays, strings provide more functionality and easier manipulation options.
Accessing String Characters
You can access individual characters in a string just like you do with arrays.
Example of Accessing Characters:
string str = "Hello, World!";
cout << str[7]; // Output: W
In this example, we retrieve the character 'W' at index 7 of the string.
Modifying String Characters
Modifying string characters works similarly to accessing them. You can change a character at any valid index.
Example of Modifying Characters:
str[0] = 'h'; // Change 'H' to 'h'
Here, we replace the first character 'H' with 'h'.
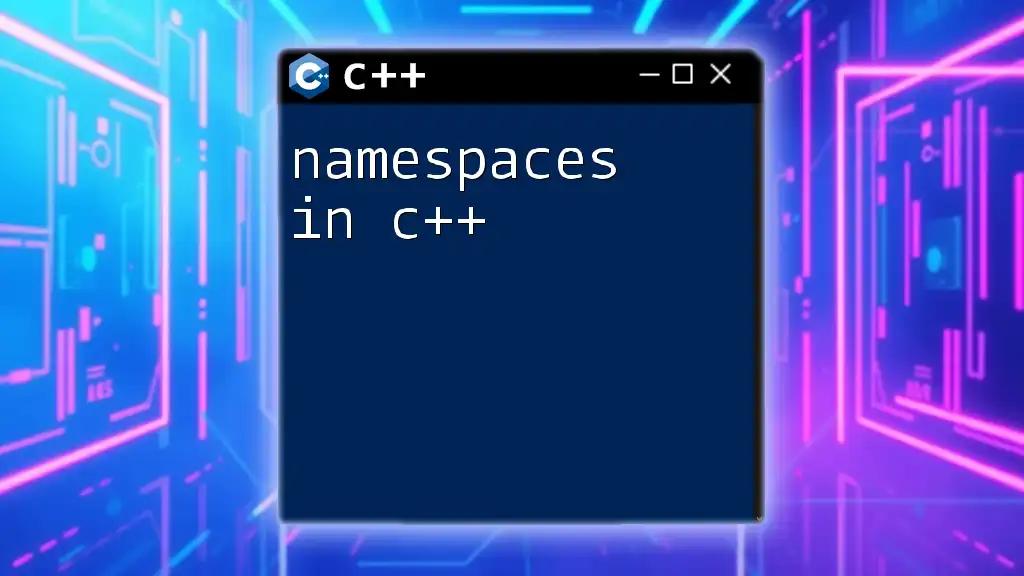
Best Practices for Using Indices
Avoiding Out-of-Bounds Errors
Out-of-bounds errors occur when you try to access an index that is not valid for the data structure, resulting in undefined behavior.
How to Prevent Out-of-Bounds Errors:
- Use conditional statements to check if an index is within the valid range.
- For arrays, ensure the index is less than the size of the array. For vectors and strings, use the `size()` or `length()` methods.
Choosing the Right Indexing
When should you use zero-based vs. one-based indexing? Generally, for C++, zero-based indexing is preferred as it's more widely implemented and typically aligns with the language's syntax. Consider performance when accessing and manipulating elements—understanding how indices work performance-wise can significantly affect the efficiency of your code.

Conclusion
In this guide, we delved into the realm of indices in C++, exploring their foundational role in accessing and manipulating arrays, vectors, and strings. Mastering indices is a vital skill for any C++ programmer. By understanding how to access and modify elements using indices correctly, you can harness the power of data structures to build efficient and effective applications.
Now, it's time to practice using indices across various structures and enhance your understanding of this fundamental programming concept. Happy coding!