In C++, the `<limits>` header provides standardized information about the properties of fundamental data types, such as their minimum and maximum representable values.
Here's a code snippet demonstrating how to use the `std::numeric_limits` template to find the limits of an `int` type:
#include <iostream>
#include <limits>
int main() {
std::cout << "Minimum int value: " << std::numeric_limits<int>::min() << std::endl;
std::cout << "Maximum int value: " << std::numeric_limits<int>::max() << std::endl;
return 0;
}
What are Limits in C++?
In C++, limits refer to the boundaries that define the range of values that a particular data type can store. Each built-in data type in C++ has specific limits regarding its maximum and minimum values that programmers must be aware of for efficient programming. Understanding these limits is crucial as it helps in preventing errors related to overflow and underflow during computation, leading to robust and safer code.

The Standard Library `<limits>` Header
Introduction to `<limits>`
The `<limits>` header in C++ provides a standardized way to query the properties and limits of fundamental data types. It contains the `std::numeric_limits` template class, which serves to directly access these properties. By including this header, programmers can easily obtain the maximum, minimum, and other characteristics of various data types.
Using the `<limits>` header
To utilize the `<limits>` library, you must include it at the beginning of your code:
#include <limits>
This inclusion allows you to call properties on the `std::numeric_limits` class to retrieve specific information about data types.
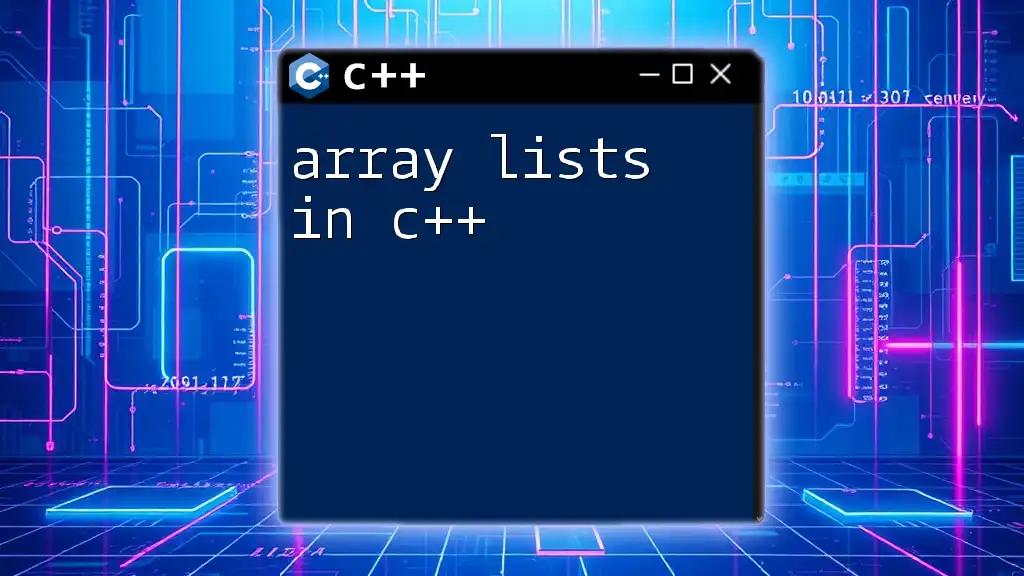
Common Data Types and Their Limits
Integral Types
Integer Limits
- int: The `int` data type typically has a range from `-2,147,483,648` to `2,147,483,647`. To find these limits, use:
#include <iostream>
#include <limits>
int main() {
std::cout << "int max: " << std::numeric_limits<int>::max() << std::endl;
std::cout << "int min: " << std::numeric_limits<int>::min() << std::endl;
return 0;
}
- short: Usually has a smaller range compared to `int`, typically from `-32,768` to `32,767`.
- long: Offers a range from `-2,147,483,648` to `2,147,483,647` (on 32-bit), but can expand on 64-bit systems.
- long long: Provides a range from `-9,223,372,036,854,775,808` to `9,223,372,036,854,775,807`.
Floating Point Types
Floating Point Limits
- float: This data type usually provides around `1.2E-38` to `3.4E+38` in terms of range but has precision limitations to about 7 decimal places.
- double: It has greater range and precision (up to 15 decimal places) compared to `float`. Use it when higher precision is necessary.
- long double: Even larger than `double`, it is generally used when working with high precision calculations and can provide more than 15 decimal places.
Character Types
Character Limits
- char: Typically represents a single character and ranges from `-128` to `127` for signed `char`. For `unsigned char`, it ranges from `0` to `255`.
- wchar_t: Used for wide characters, it can store larger character sets, particularly useful in internationalization.

Exploring the Characteristics of Data Types
Characteristics Explained
Is signed/unsigned
You can determine if a type is signed or unsigned using:
std::cout << "Is int signed? " << std::numeric_limits<int>::is_signed << std::endl;
This reveals whether the integer type can represent negative values, which is crucial for preventing errors in calculations involving negative numbers.
Precision and Digits
Understanding the precision of floating-point types is essential in computations. For instance, you can check the precision and digits of a `float` like so:
std::cout << "Float precision: " << std::numeric_limits<float>::digits10 << std::endl;
Examples in Practice
Practical scenarios highlight the importance of data type limits. Overflow occurs when a computation exceeds the maximum limits of a data type, while underflow occurs when it falls below the minimum limits.
For example, an overflow situation with an `int` could look like this:
#include <iostream>
#include <limits>
int main() {
int maxInt = std::numeric_limits<int>::max();
std::cout << "Max int: " << maxInt << std::endl;
std::cout << "Overflowing max int: " << (maxInt + 1) << std::endl; // This will overflow
return 0;
}
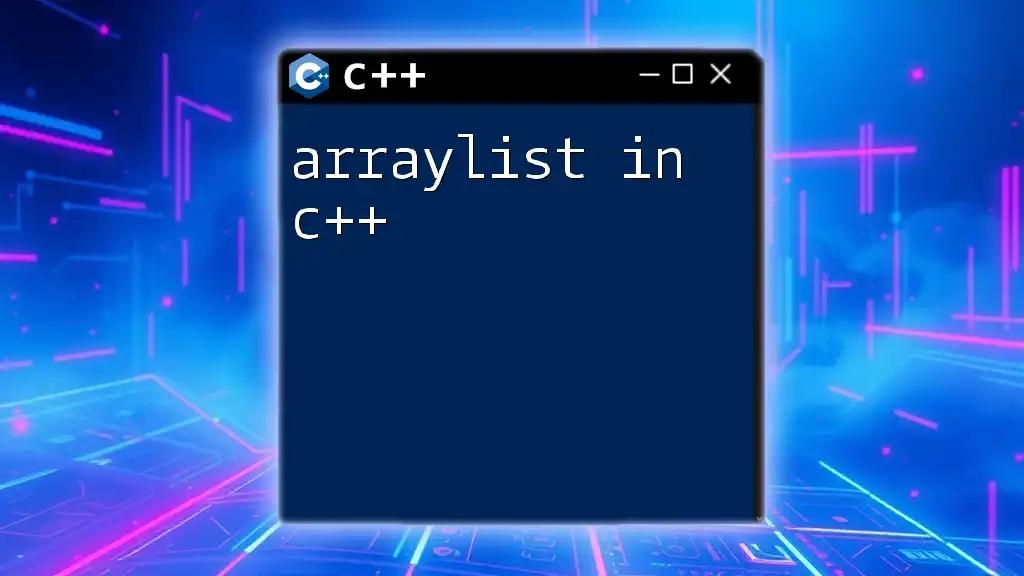
Checking for Limits and Validating Data
Writing Functions for Limit Checking
Creating functions to check if a value exceeds defined limits can be a great practice, particularly in critical applications. For example:
#include <limits>
bool isWithinIntLimits(int value) {
return value >= std::numeric_limits<int>::min() && value <= std::numeric_limits<int>::max();
}
This function can help prevent issues before they arise, adding an extra layer of validation to your code logic.
Exception Handling
When limits are exceeded, handling exceptions is vital. Implementing error handling around your limits checks can help manage these incidents gracefully. For instance, using try-catch blocks can effectively capture unexpected behavior related to data limits.
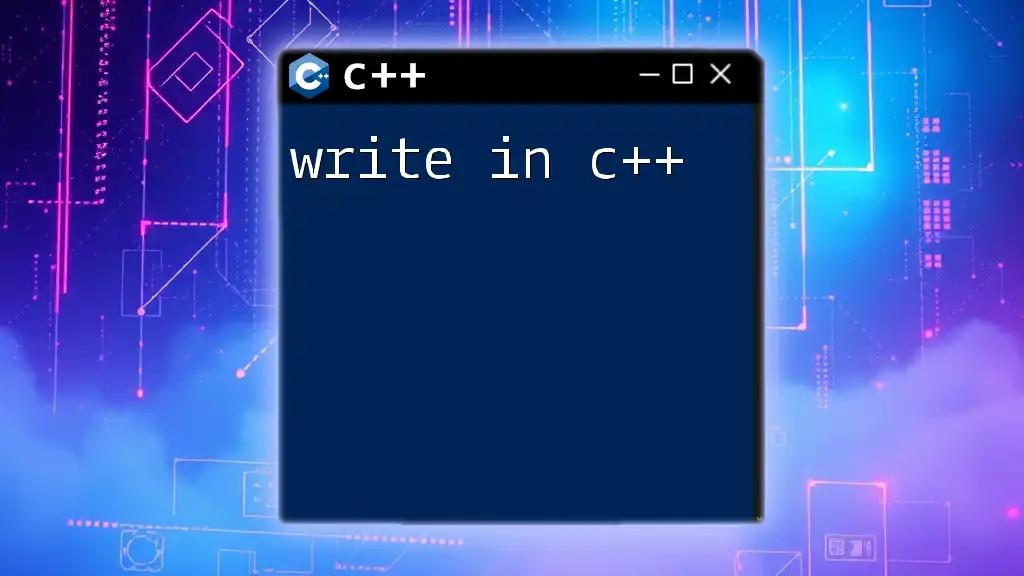
Real-World Applications of Limits in C++
Understanding limits significantly impacts real-world programming tasks. For instance, when dealing with user input, ensuring that values stay within the defined limits helps to prevent potential errors in computations later on.
Performance Considerations should also be taken into account. Depending on the chosen data types and their limits, algorithms may run faster or slower. Ensuring that you select the appropriate type not only affects memory usage but also execution time.

Conclusion
In summary, understanding limits in C++ is critical for writing efficient, safe, and effective code. Knowing the boundaries of data types helps prevent errors related to overflow and underflow, ensures data integrity, and enhances overall performance in your applications. As you explore further in your programming journey, don’t forget to implement limit checks for a more robust codebase.

Additional Resources
To deepen your understanding of limits in C++, consider exploring these resources: documentation on the `<limits>` header, online C++ tutorials, and textbooks focused on C++ programming principles.

FAQ Section
If you have further questions about limits in C++, common queries such as how limits affect custom data types or complex structures can be researched or discussed with peers in programming communities. Engaging with other programmers enhances your understanding and keeps you abreast of best practices in programming.