Array lists in C++ are dynamic data structures that can hold a resizable collection of elements, enabling you to manage collections of data more flexibly than static arrays.
Here’s a simple example using `std::vector`, a commonly used array list in C++:
#include <iostream>
#include <vector>
int main() {
std::vector<int> myArrayList; // Create an empty array list
myArrayList.push_back(10); // Add elements
myArrayList.push_back(20);
myArrayList.push_back(30);
// Output elements
for (int i = 0; i < myArrayList.size(); i++) {
std::cout << myArrayList[i] << " "; // Prints: 10 20 30
}
return 0;
}
Understanding Array Lists in C++
What is an Array List?
An array list is a data structure that allows for dynamic resizing and easy management of an array. Unlike traditional arrays that have a fixed size, array lists can grow and shrink as needed. This makes them particularly useful for scenarios where the number of elements cannot be predetermined, such as user-driven applications or dynamically generated data.
Use Cases: Array lists are widely used in situations like maintaining lists of names entered by users, managing items in applications, or even handling dynamic sets of numbers resulting from calculations or data entry.
Advantages of Using Array Lists
One of the key advantages of array lists is dynamic sizing. This means that when you add elements, the array automatically grows to accommodate them, allowing you to work effectively without needing to specify a capacity up front.
Another major benefit is the ease of use. Array lists utilize simple syntax for managing elements, making them much more user-friendly compared to standard arrays, where fixed sizes often lead to complications in code.
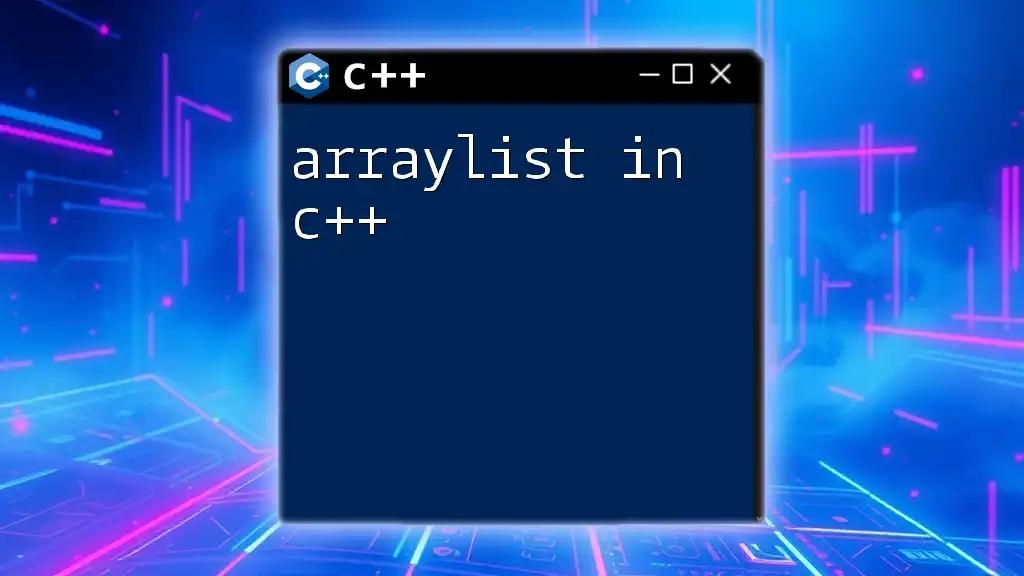
Implementing Array Lists in C++
Basic Structure of an Array List
The fundamental components of an array list include an array pointer, which points to the actual array, a size that tracks the current number of elements, and a capacity that indicates the maximum size the array can grow to.
Let’s look at a code snippet that defines a basic `ArrayList` class:
class ArrayList {
private:
int* arr; // Pointer to the array
int size; // Current number of elements
int capacity; // Maximum size of the array
public:
ArrayList(int cap);
~ArrayList();
void add(int element);
void remove(int index);
void resize(int newCapacity);
};
Memory Management in Array Lists
Effective memory management is crucial when implementing array lists. In C++, we use dynamic memory allocation to manage the memory efficiently with the `new` and `delete` keywords.
Here’s how the constructor and destructor are defined:
ArrayList::ArrayList(int cap) {
capacity = cap;
size = 0;
arr = new int[capacity]; // Dynamic allocation
}
ArrayList::~ArrayList() {
delete[] arr; // Free allocated memory
}
Essential Operations on Array Lists
Adding Elements to the Array List
Adding elements is a vital operation for any array list. This method checks if the current size exceeds the array's capacity before adding a new element. If it does, the array resizes to accommodate more elements.
Here’s how the `add` method is implemented:
void ArrayList::add(int element) {
if (size >= capacity) {
resize(capacity * 2); // Double the capacity
}
arr[size++] = element; // Add element and increment size
}
Removing Elements from the Array List
Removing elements involves shifting elements to the left to fill the gap created by the removed item. This operation requires careful validation of the index.
Here’s a code snippet for the `remove` method:
void ArrayList::remove(int index) {
if (index < 0 || index >= size) {
throw out_of_range("Index out of range");
}
for (int i = index; i < size - 1; ++i) {
arr[i] = arr[i + 1]; // Shift elements left
}
size--;
}
Resizing the Array List
Resizing is a crucial feature that allows the array to increase its capacity as necessary. This typically happens when adding a new element results in exceeding the existing capacity.
Let’s see how the `resize` method works:
void ArrayList::resize(int newCapacity) {
int* newArr = new int[newCapacity]; // New larger array
for (int i = 0; i < size; ++i) {
newArr[i] = arr[i]; // Copy elements to new array
}
delete[] arr; // Release old array
arr = newArr; // Reassign
capacity = newCapacity;
}

Iterating Over an Array List
Implementing Iterators in Array Lists
To enhance usability, implementing iterators allows for users to traverse the array list easily. An iterator hides the inner workings of the array list and permits sequential access to its elements without exposing the underlying data structure.
Here’s a simple example of an iterator class:
class ArrayListIterator {
private:
ArrayList& list;
int index;
public:
ArrayListIterator(ArrayList& lst) : list(lst), index(0) {}
bool hasNext() { return index < list.size; }
int next() { return list.arr[index++]; }
};

Conclusion
To summarize, array lists in C++ offer a flexible way to manage collections of data that can grow and shrink dynamically. With operations like adding, removing, and resizing elements easily accessible, they prove to be powerful tools in the C++ programmer's toolkit.
FAQs on Array Lists in C++
What is the difference between array lists and linked lists?
Array lists are stored in contiguous memory, allowing random access, while linked lists consist of nodes that are distributed throughout memory, making them more efficient for insertions and deletions but less efficient for searching.
When should I use an array list over a vector in C++?
While both are used for dynamic arrays, `std::vector` is part of the C++ Standard Library, offering built-in safety and convenience features. Consider using an array list for educational purposes or a specific requirement when you seek control over implementation.
Are array lists thread-safe?
By default, array lists are not thread-safe. If you intend to use them in a multithreaded context, you must implement synchronization mechanisms to ensure safe concurrent access.