An adjacency list in C++ is a data structure used to represent a graph, where each vertex has a list of its adjacent vertices, allowing for efficient storage and traversal.
Here’s a simple code snippet to illustrate an adjacency list using a vector of vectors:
#include <iostream>
#include <vector>
using namespace std;
int main() {
int V = 5; // Number of vertices
vector<vector<int>> adj(V); // Adjacency list
// Adding edges
adj[0].push_back(1);
adj[0].push_back(4);
adj[1].push_back(0);
adj[1].push_back(2);
adj[1].push_back(3);
adj[2].push_back(1);
adj[3].push_back(1);
adj[4].push_back(0);
// Displaying the adjacency list
for (int i = 0; i < V; ++i) {
cout << "Vertex " << i << ": ";
for (int j : adj[i]) {
cout << j << " ";
}
cout << endl;
}
return 0;
}
Understanding the Structure of an Adjacency List
An adjacency list is one of the most efficient ways to represent a graph in C++. It stores a collection of nodes (or vertices) where each node has a list of adjacent nodes (or vertices) to which it is connected. This structure offers significant memory advantages and is particularly suited for sparse graphs.
Components of an Adjacency List
To understand how an adjacency list works, consider a graph consisting of nodes connected by edges. Each node in the graph corresponds to a vertex, and any edges are represented as lists in C++.
For instance, if we have a graph with nodes A, B, and C with edges connecting A to B, and A to C, the adjacency list will effectively mirror this connectivity. Instead of using a matrix that potentially allocates space for every vertex pair, an adjacency list dynamically allocates space only for existing edges, making it a memory-efficient representation.
Why Choose an Adjacency List in C++?
Using an adjacency list in C++ is particularly beneficial in scenarios with a larger number of vertices and fewer edges. Here are key reasons to prefer it:
- Space Efficiency: Unlike adjacency matrices, which require space in proportion to the square of the number of vertices (O(V^2)), adjacency lists only need space proportional to the number of edges (O(E + V)).
- Ease of Traversal: The structure allows for easier traversal of adjacent nodes, enhancing certain algorithm implementations, like BFS or DFS.
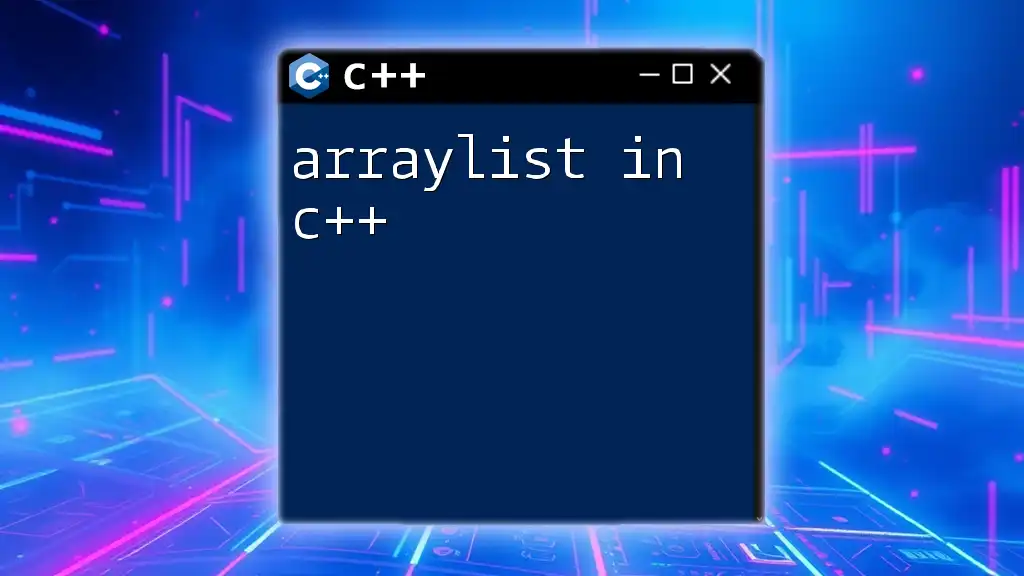
Implementing an Adjacency List in C++
To create our adjacency list in C++, we first need to set up our environment and define the graph structure.
Setting Up the Environment
Make sure you have a C++ environment ready. You'll typically need a modern compiler such as g++ or clang++. You can also utilize IDEs such as Code::Blocks, Visual Studio, or JetBrains CLion.
Basic Structure of an Adjacency List
The basic organization of an adjacency list in C++ can be implemented using a class. Here’s a code snippet that shows how to get started:
#include <iostream>
#include <vector>
using namespace std;
class Graph {
public:
int V; // Number of vertices
vector<vector<int>> adjList; // Adjacency List
Graph(int vertices) {
V = vertices;
adjList.resize(V); // Resize the vector to the number of vertices
}
};
This code defines a `Graph` class with a constructor that initializes the number of vertices and resizes the adjacency list to hold empty vectors for each vertex.
Building the Adjacency List
An adjacency list is built by adding edges. The following function demonstrates how to add edges between nodes:
void addEdge(int u, int v) {
adjList[u].push_back(v); // For directed graphs
adjList[v].push_back(u); // Uncomment for undirected graphs
}
In this code, each vertex has a dynamic list of its adjacent vertices, allowing you to add connections as needed.
Visualizing the Adjacency List
To get a clearer picture of how the adjacency list looks in action, you can print it out with a simple function:
void printGraph() {
for (int i = 0; i < V; ++i) {
cout << "Node " << i << ": ";
for (int j : adjList[i]) {
cout << j << " ";
}
cout << endl;
}
}
When you call this function post populating the graph with edges, it will display the connections clearly for each node.
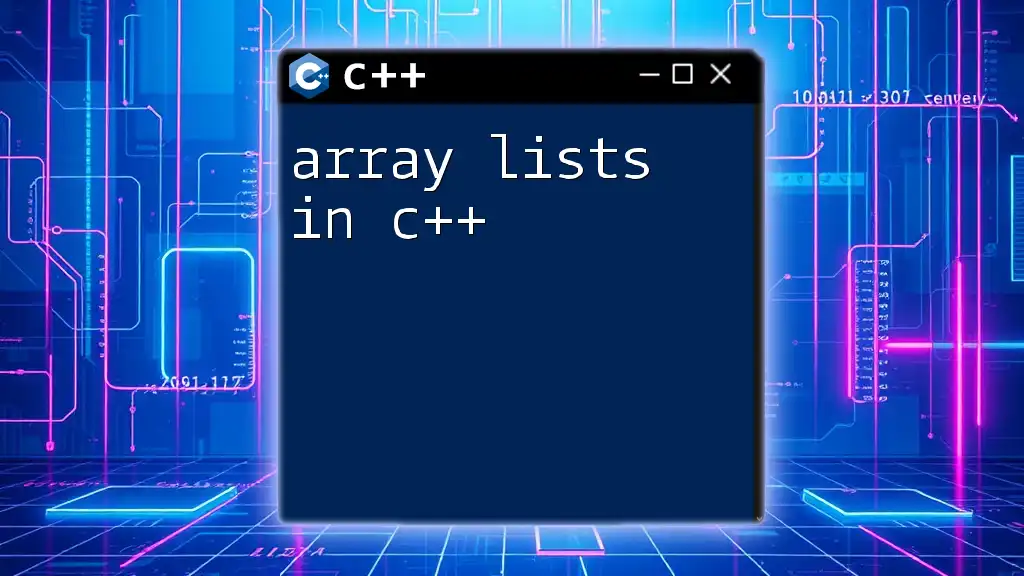
Traversing the Graph Using Adjacency List
One of the key advantages of the adjacency list is how effectively it allows for graph traversal. You can implement different algorithms, such as Depth-First Search (DFS) and Breadth-First Search (BFS).
Depth-First Search (DFS) Implementation
DFS explores as far as possible down a branch before backtracking. Here’s how you can implement it:
void DFSUtil(int v, vector<bool>& visited) {
visited[v] = true; // Mark the current node as visited
cout << v << " "; // Print the current node
for (int i : adjList[v]) {
if (!visited[i]) {
DFSUtil(i, visited); // Visit adjacent nodes
}
}
}
void DFS(int start) {
vector<bool> visited(V, false); // Track visited nodes
DFSUtil(start, visited); // Start DFS from the given node
}
Calling `DFS(0);` for a graph starting from node 0 would print all reachable nodes in a depth-first order.
Breadth-First Search (BFS) Implementation
On the other hand, BFS explores neighbor nodes before moving on to the next level. Here's how it can be implemented:
void BFS(int start) {
vector<bool> visited(V, false); // Track visited nodes
queue<int> q; // Queue for BFS
visited[start] = true; // Mark the start node
q.push(start); // Push starting node into the queue
while (!q.empty()) {
int v = q.front();
q.pop(); // Pop the front of the queue
cout << v << " "; // Print the current node
for (int i : adjList[v]) {
if (!visited[i]) {
visited[i] = true; // Mark as visited
q.push(i); // Enqueue adjacent nodes
}
}
}
}
Initiating BFS with `BFS(0);` will show all nodes level-wise when starting from node 0.
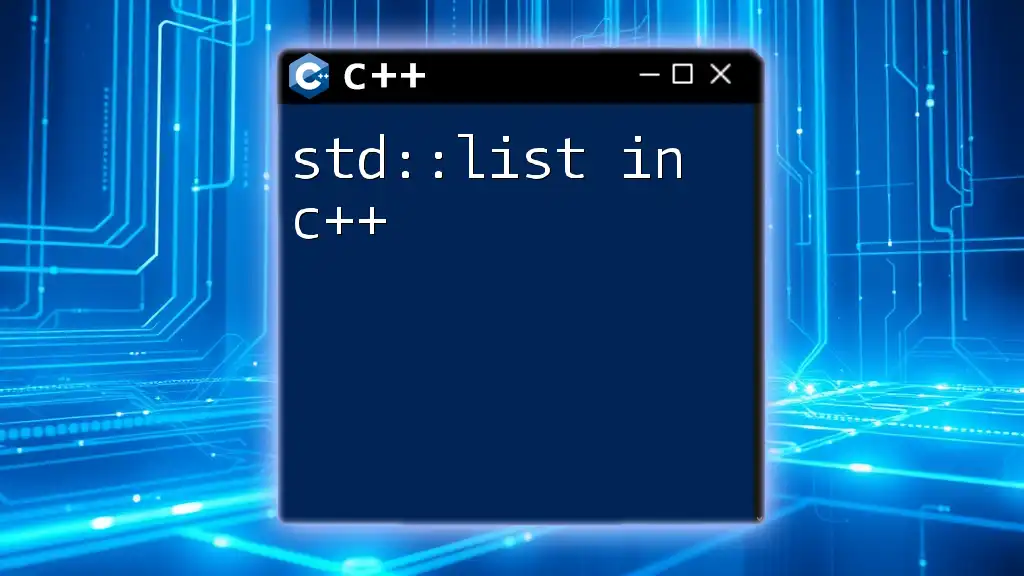
Use Cases and Applications of Adjacency List in C++
The adjacency list in C++ finds applications across a variety of domains.
For instance, in social networks, users can be represented by nodes, while friendships act as edges between them. In routing algorithms for network optimization, you can model computers as nodes and connections as edges.
Comparison with Other Data Structures
While the adjacency list is efficient for sparse graphs, it may not be the best choice for dense graphs (where every vertex is connected to many others). In such cases, an adjacency matrix may provide quicker access time at the cost of increased space.
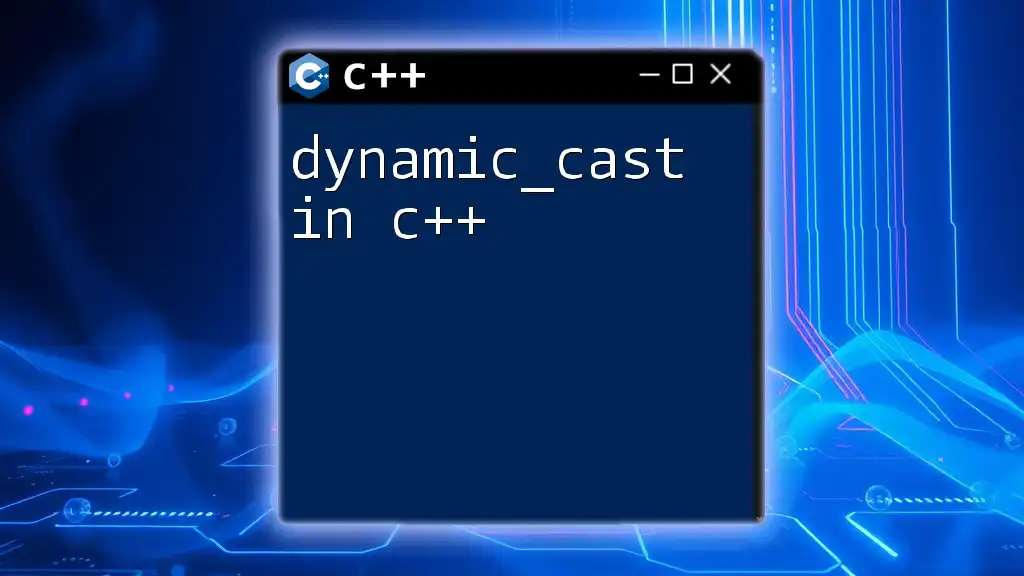
Best Practices in Using Adjacency Lists
To maximize the benefits of using an adjacency list, here are some considerations:
Memory Management
Since adjacency lists dynamically allocate space, ensure you are regularly cleaning up memory, especially when using raw pointers or creating complex objects in your graph.
Performance Considerations
The structure of your adjacency list may significantly affect performance. Always consider the growth of your list operations; if inserting or deleting edges becomes frequent, optimizing your data structure can lead to significant performance improvements.
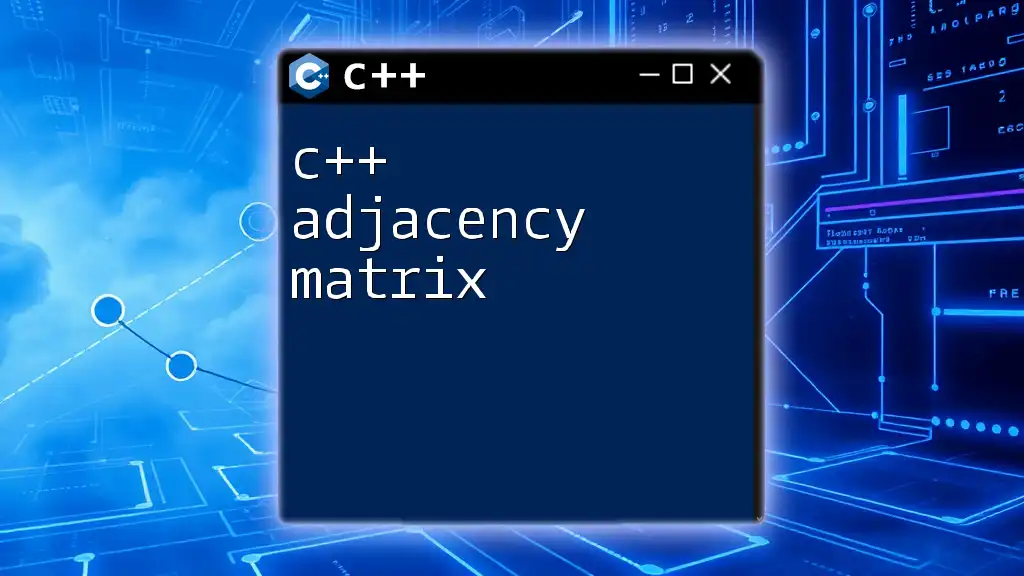
Conclusion
In conclusion, employing an adjacency list in C++ is a fundamental technique for representing graphs effectively, especially when concerned with memory efficiency and ease of traversal. By practicing these implementations and understanding the underlying concepts, you can harness the full power of graph data structures in your projects. Now, take the opportunity to create your own graphs and explore the various functionalities of the adjacency list to solidify your understanding!
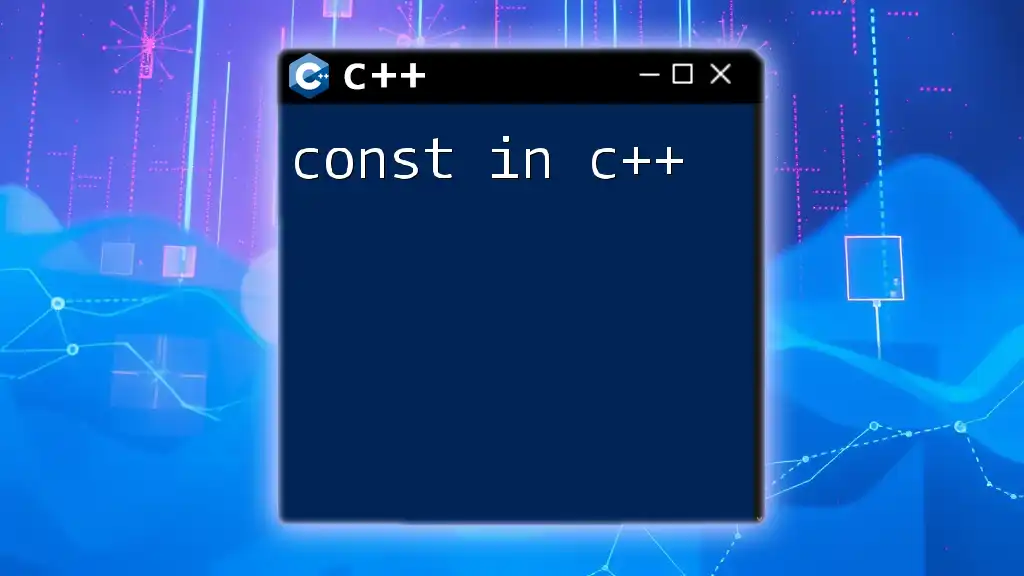
Additional Resources
For further exploration, consider checking out additional literature on graph theory and the implementation of advanced algorithms. Libraries such as Boost Graph Library and GLib can also provide valuable insights into sophisticated graph operations in C++.