To read a line of input from the user in C++, you can use the `std::getline` function from the `<iostream>` header to capture the entire line, including spaces, into a string variable. Here's a code snippet demonstrating this:
#include <iostream>
#include <string>
int main() {
std::string input;
std::cout << "Enter a line: ";
std::getline(std::cin, input);
std::cout << "You entered: " << input << std::endl;
return 0;
}
Understanding Input Streams
What are Input Streams?
In C++, input streams are essential components that allow the program to receive data from various sources, such as the keyboard or files. Streams abstract the process of reading input, facilitating data flow in a structured manner. When a program needs to read user input or manipulate files, understanding input streams becomes crucial.
The `std::cin` Stream
The `std::cin` stream is a fundamental part of C++ that facilitates reading input from the standard input device, usually the keyboard. It is a part of the standard input-output library and provides a straightforward way for users to interact with the program.
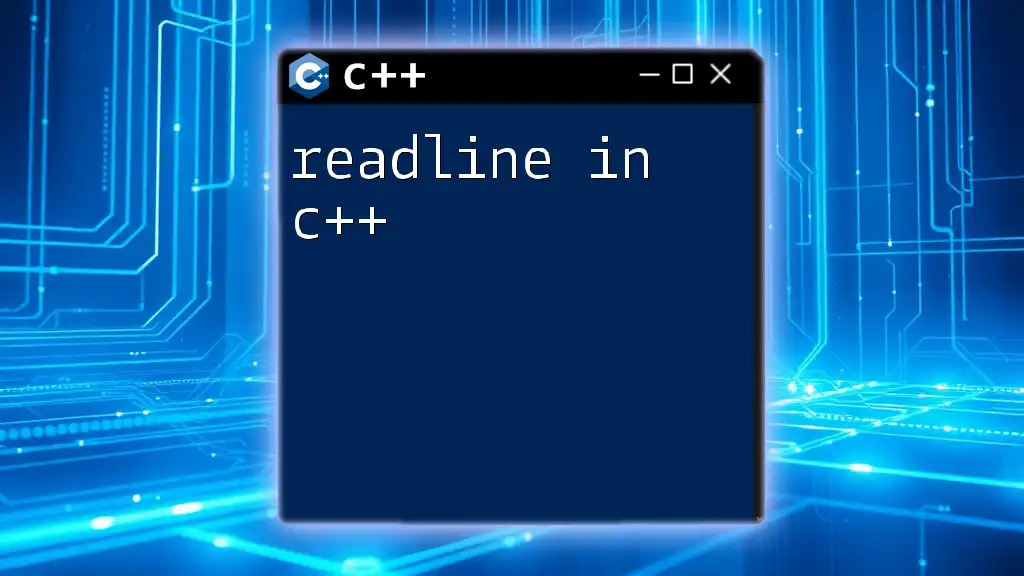
Basic Methods for Reading a Line
Using `std::getline()`
`std::getline()` is the preferred method for reading a complete line of text from an input stream. It reads characters from the input until a newline character is found, allowing for more comprehensive input handling compared to methods like `std::cin`.
Syntax and Basic Usage
The syntax of `std::getline()` is as follows:
std::getline(std::istream& is, std::string& str);
- Here, `is` refers to the input stream (like `std::cin` or an input file stream), and `str` is the string that will store the result.
Example Code
#include <iostream>
#include <string>
int main() {
std::string line;
std::cout << "Enter a line of text: ";
std::getline(std::cin, line);
std::cout << "You entered: " << line << std::endl;
return 0;
}
In this example, the program prompts the user to enter a line of text. `std::getline()` captures everything the user types until they press Enter, storing it in the `line` variable. The input is then echoed back to the user.
Handling Input with `std::cin`
While `std::cin` is commonly used, it has certain limitations when handling line inputs. It reads data up to the first whitespace character, making it unsuitable for reading full lines unless used properly.
Limitations of `std::cin`
When using `std::cin`, if the user inputs a multi-word string, only the first word will be captured. This can be a limitation in scenarios where users may need to enter more extensive text.
Example Code
#include <iostream>
int main() {
std::string word;
std::cout << "Enter a word: ";
std::cin >> word;
std::cout << "You entered: " << word << std::endl;
return 0;
}
In this example, we prompt the user for a single word. If the user types "Hello World", only "Hello" will be captured and displayed, demonstrating the constraints of `std::cin`.
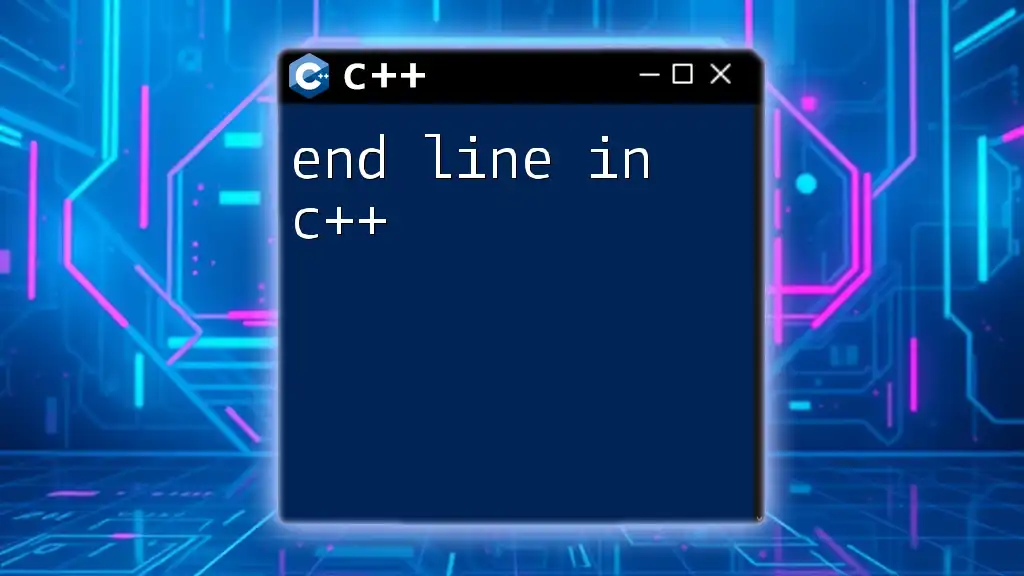
Reading from Files
Opening a File Stream
To read data from files in C++, you utilize the input file stream (`std::ifstream`). This stream provides an interface for input operations similar to how `std::cin` works for keyboard input.
Example Code
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("example.txt");
std::string line;
if (file.is_open()) {
while (std::getline(file, line)) {
std::cout << line << std::endl;
}
file.close();
} else {
std::cout << "Unable to open file." << std::endl;
}
return 0;
}
In this snippet, we attempt to open "example.txt". If successful, the program reads each line from the file using `std::getline()` and prints it until the end of the file is reached. This approach highlights the versatility of `std::getline()` for both dynamic user input and static file data.
Error Handling While Reading
When working with file input, error handling is critical. If a file cannot be opened or read, your program should notify the user appropriately.
Checking for Failures
Utilizing the error reporting capabilities of C++, you can check for stream failures. For instance:
if (file.fail()) {
std::cerr << "Error reading file!" << std::endl;
}
This code snippet serves as an error check after attempting to read from a file, ensuring that issues are caught gracefully and communicated to the user.
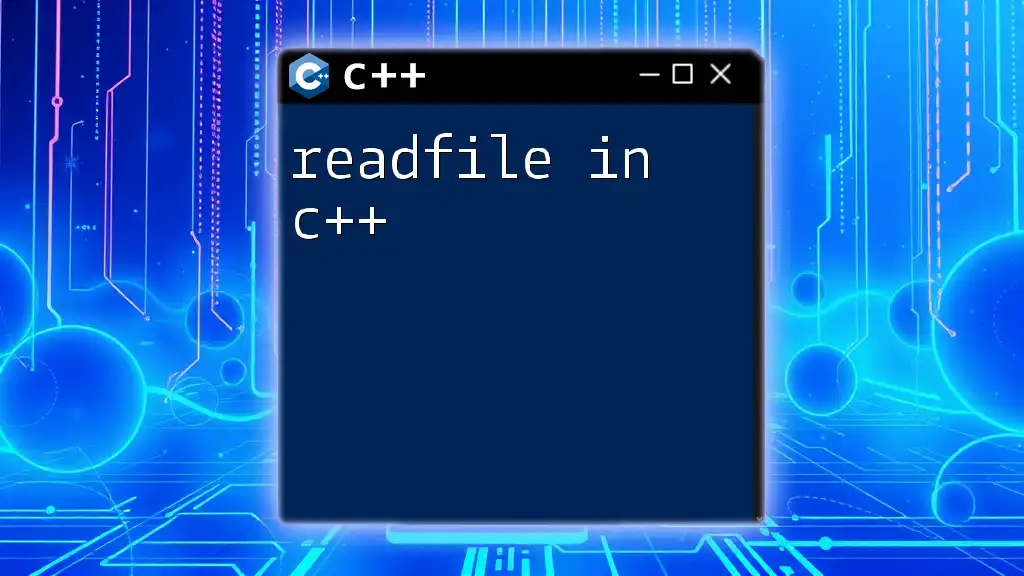
Customizing Input Delimiters
Changing the Delimiter in `std::getline()`
In many situations, you may need to read input delimited by specific characters (e.g., commas, spaces). Fortunately, `std::getline()` allows for customizing the delimiter it uses to separate input.
Here’s how you can set a custom delimiter using `std::getline()`:
Example Code
std::string line;
std::cout << "Enter text (comma-separated): ";
std::getline(std::cin, line, ',');
std::cout << "First part: " << line << std::endl;
By specifying a comma as the third argument in `std::getline()`, the program only reads input up to the first comma. This functionality is particularly useful when reading CSV (Comma-Separated Values) data or other structured input formats.
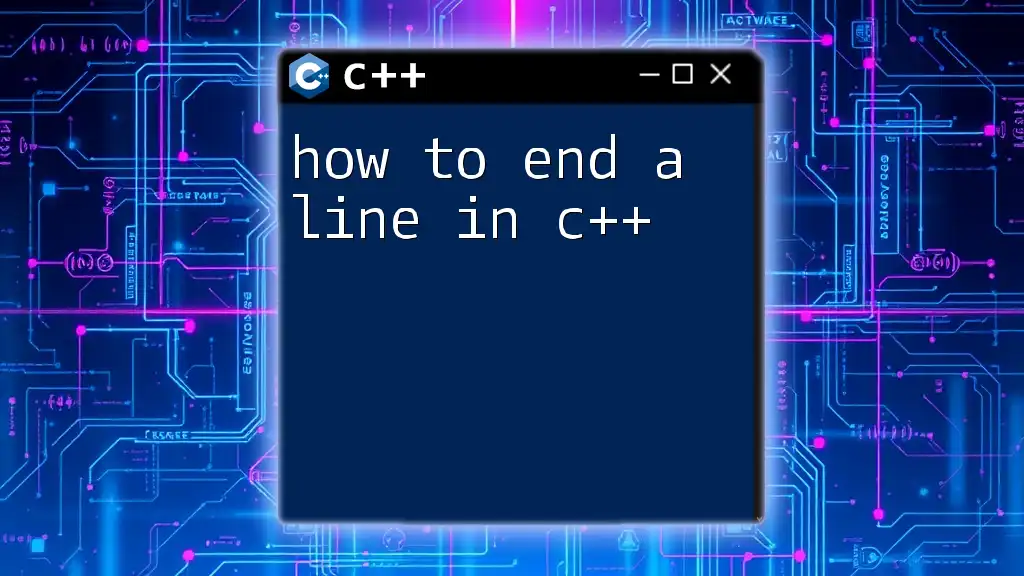
Conclusion
In this guide, we explored multiple methodologies to read a line in C++, from utilizing the robust `std::getline()` function to managing file input and customizing delimiters. By mastering these techniques, you can enhance your input handling capabilities in C++, making your programs more interactive and user-friendly.
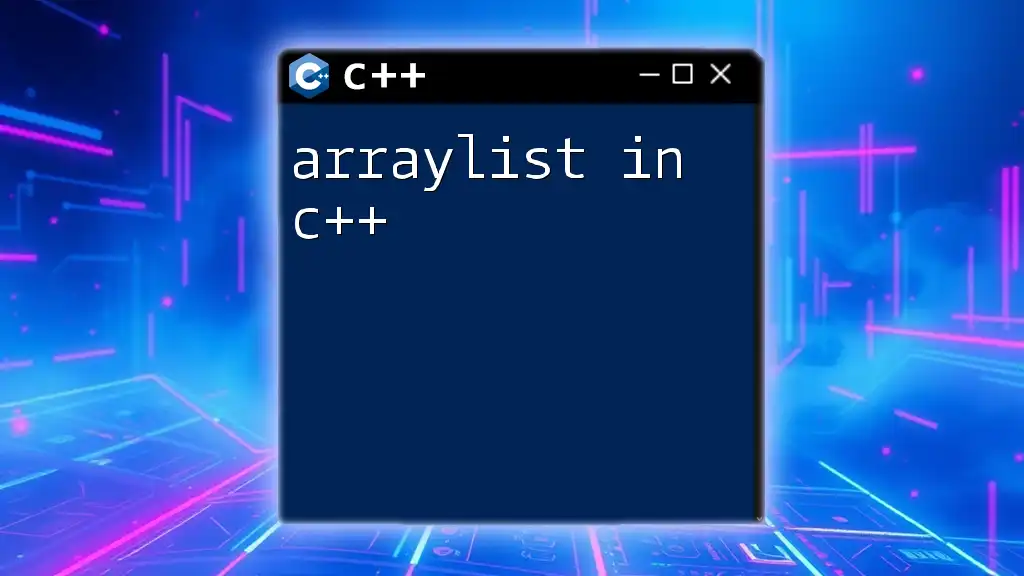
Additional Tips and Resources
For those eager to deepen their understanding of input handling in C++, numerous resources are available, including official C++ documentation, online tutorials, and coding practice sites. Engaging with these materials will expand your skills and enable you to tackle more complex input scenarios effectively.