In C++, `std::string` is a part of the Standard Library that provides a flexible and convenient way to handle and manipulate strings of characters.
#include <iostream>
#include <string>
int main() {
std::string greeting = "Hello, World!";
std::cout << greeting << std::endl;
return 0;
}
Understanding std::string in C++
What is std::string?
`std::string` is a part of the C++ Standard Library, designed to represent sequences of characters more flexibly than C-style strings (`char*`). While C-style strings are arrays of characters terminated by a null character (`'\0'`), `std::string` provides a higher-level abstraction that automatically manages memory and provides a rich set of functionality.
One of the primary advantages of using `std::string` is its dynamic sizing. Unlike C-style strings, which require manual memory management, `std::string` can automatically adjust its size as needed. This feature simplifies string manipulation and reduces the risk of memory leaks and buffer overflows.
Key Features of std::string
`std::string` comes with a host of features that make it indispensable for C++ programming:
- Dynamic Sizing: Automatically adjusts to fit the content, eliminating manual sizing.
- Built-in Functions: Offers a variety of methods to manipulate and query the string.
- Operator Overloading: Supports common operations like concatenation using `+` or `+=`, making the syntax intuitive.
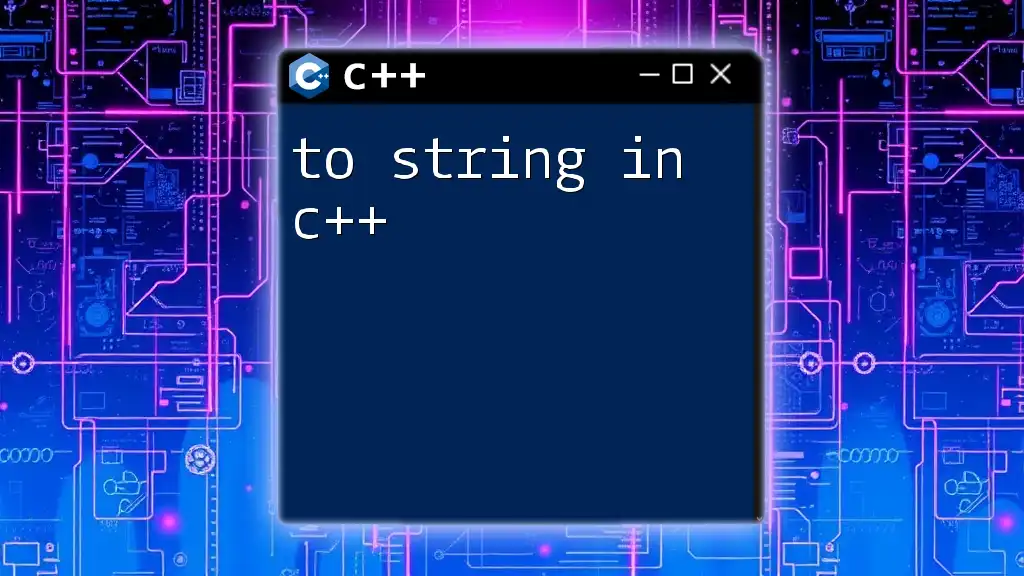
Basic Usage of std::string
Creating and Initializing std::string
Creating a `std::string` is straightforward. You can declare and initialize it in various ways:
std::string myString = "Hello, World!";
std::string anotherString; // Default initialization, empty string.
Additionally, you can also initialize a string with a specific number of characters:
std::string filledString(5, 'A'); // Results in "AAAAA"
Accessing Characters in std::string
You can access individual characters in a `std::string` using the `[]` operator or the `at()` method. While both serve similar purposes, `at()` includes bounds checking and will throw an exception if the index is out of range.
char firstChar = myString[0]; // Accessing the first character
char secondChar = myString.at(1); // Accessing the second character safely
Modifying std::string
Appending Strings
Appending to a `std::string` can be done simply using the `+` operator or the `append()` method. This allows for straightforward concatenation:
std::string newString = " Welcome!";
myString += newString;
Or using the `append()` method:
myString.append(" Have a nice day!");
Inserting and Erasing Characters
You can also insert substrings at any position or remove characters:
myString.insert(5, " Beautiful");
myString.erase(0, 5);
The `insert()` method takes an index and the substring to be inserted, while `erase()` removes characters starting from the specified index.
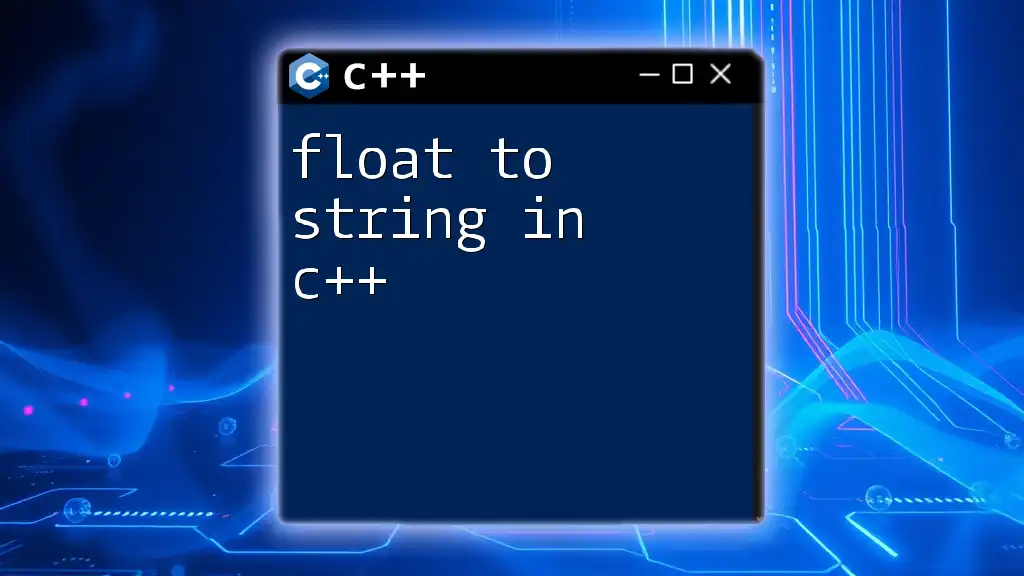
Advanced Features of std::string
String Comparison
Comparing strings in C++ is intuitive with `std::string`. You can use various operators such as `==`, `!=`, `<`, `>`, etc.:
if (myString == anotherString) {
// Do something when strings are equal
}
Substrings and Searching
Extracting substrings can be done easily with the `substr()` method:
std::string subStr = myString.substr(0, 5); // Extracts the first five characters
Searching within a string can be done with the `find()` and `rfind()` methods, allowing you to locate a substring's position. If not found, `find()` returns `std::string::npos`:
size_t pos = myString.find("World");
if (pos != std::string::npos) {
// "World" was found, handle accordingly
}
Finding Length and Capacity
To get the size of a string, you can use either `length()` or `size()`, which return the number of characters in the string. To understand memory allocation better, `capacity()` shows how much memory has been allocated for future expansion:
size_t len = myString.length(); // Get the length
size_t cap = myString.capacity(); // Get the capacity
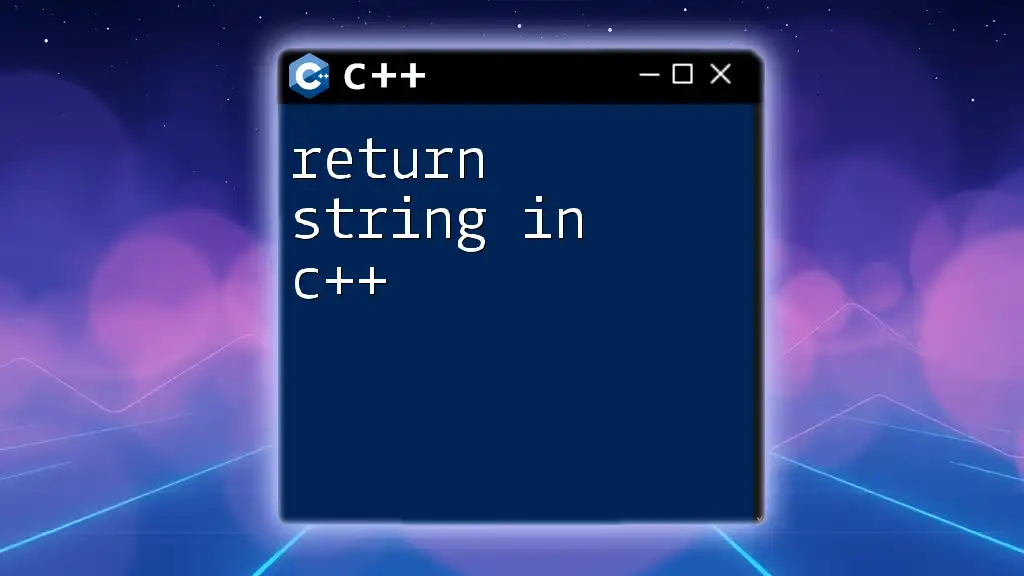
Formatting with std::string
Conversion Between Types
When you need to convert other data types to strings, `std::to_string()` is your go-to method. It simplifies the task of transforming various types into string representations:
int number = 42;
std::string strNumber = std::to_string(number);
String Formatting Libraries
For more complex string formatting needs, consider using `std::ostringstream` from the `<sstream>` library. This enables you to create formatted strings easily:
#include <sstream>
std::ostringstream oss;
oss << "The number is: " << number;
std::string formattedString = oss.str();
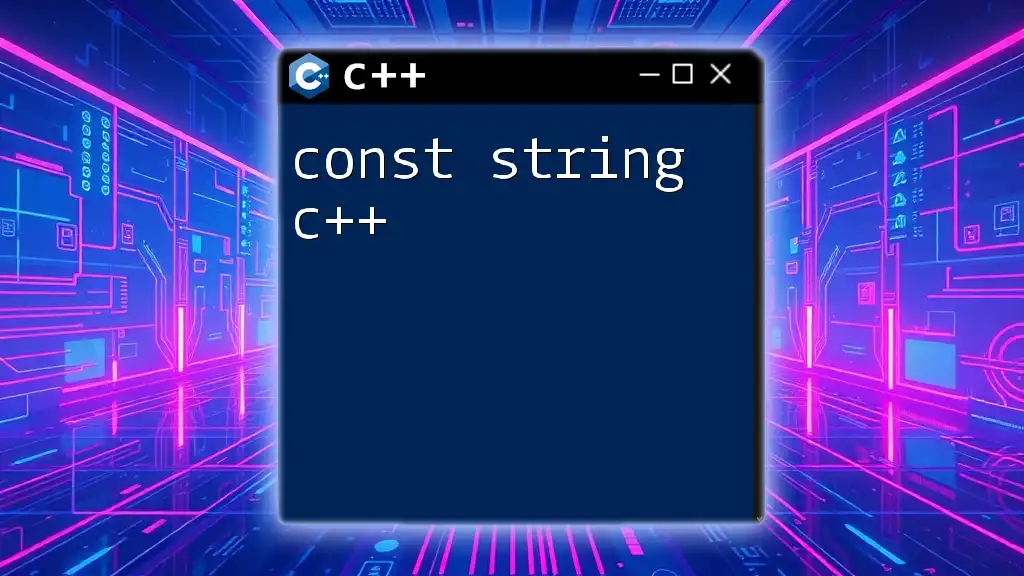
Common Pitfalls When Using std::string
Memory Management Issues
While `std::string` simplifies memory management, it’s essential to understand that copying strings or creating references to them can lead to unexpected behavior. Modifying a referenced string affects the original.
Performance Considerations
`std::string` is versatile, but it's crucial to be aware of performance implications in specific contexts. For operations requiring frequent modifications, consider alternatives like `std::string_view`, which provides a lightweight reference without ownership.
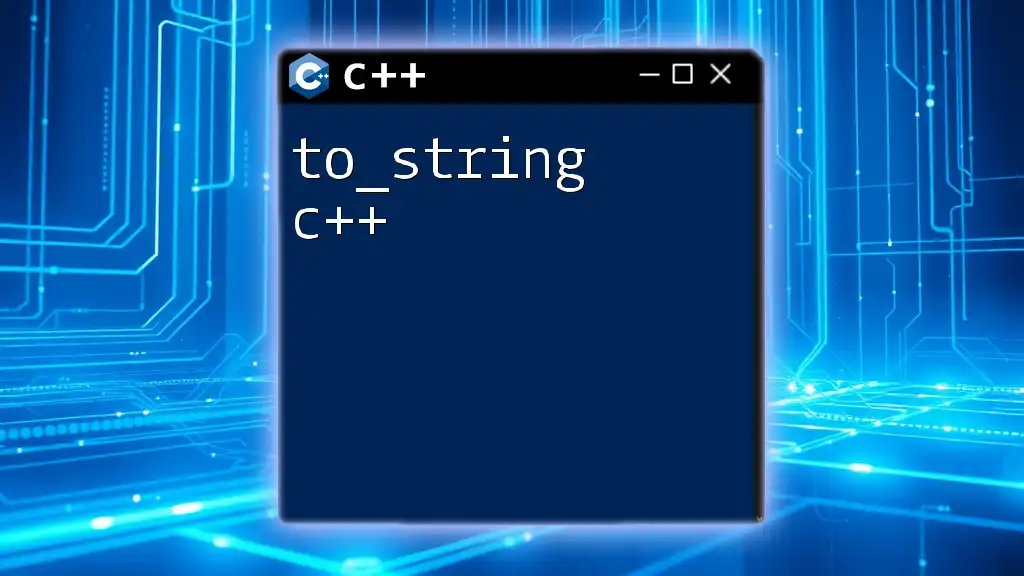
Conclusion
In summary, `std::string` in C++ offers a powerful and flexible way to handle strings. Its dynamic sizing, built-in methods, and ease of use distinguish it from C-style strings. Gaining mastery over `std::string` will not only enhance your programming skills but also make your code cleaner and more efficient. Exploring more advanced string manipulation techniques will further broaden your proficiency in C++.
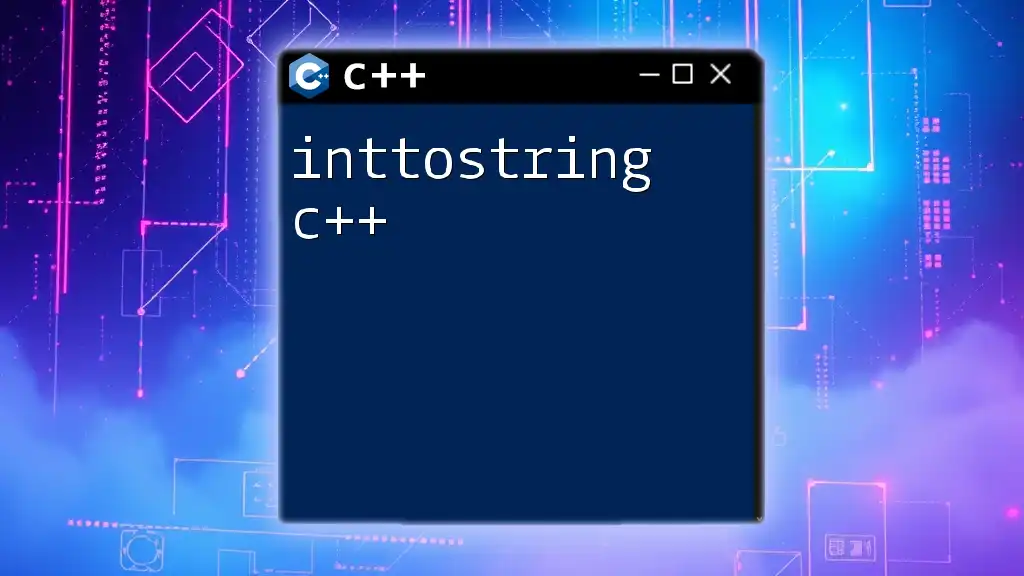
Additional Resources
To deepen your understanding, check the official C++ documentation on `std::string`, explore recommended books, or engage in community forums where you can ask questions and share insights.