In C++, you can obtain the size of a string using the `size()` or `length()` member functions of the `std::string` class, which return the number of characters in the string. Here's an example:
#include <iostream>
#include <string>
int main() {
std::string myString = "Hello, World!";
std::cout << "Size of the string: " << myString.size() << std::endl;
return 0;
}
Understanding Strings in C++
What is a String in C++?
A string in C++ is a sequence of characters treated as a single data type. Unlike standard character arrays, C++ strings (specifically `std::string`) provide a rich set of functionalities that facilitate easier manipulation and management. The inclusion of the `<string>` header allows programmers to work with a robust string class that takes care of size management, memory allocation, and many built-in operations.
Types of Strings in C++
Standard String (std::string)
The `std::string` type is the most commonly used string representation in C++. It offers dynamic size, automatic memory management, and a plethora of member functions to interact with the string data. C++ strings handle their own memory, minimizing the chances of memory leaks or overflows.
C-Style Strings
In contrast, C-style strings are essentially arrays of characters that end with a null terminator (`\0`). While they are more lightweight than `std::string`, they require careful handling of memory and size, as they lack built-in size management.
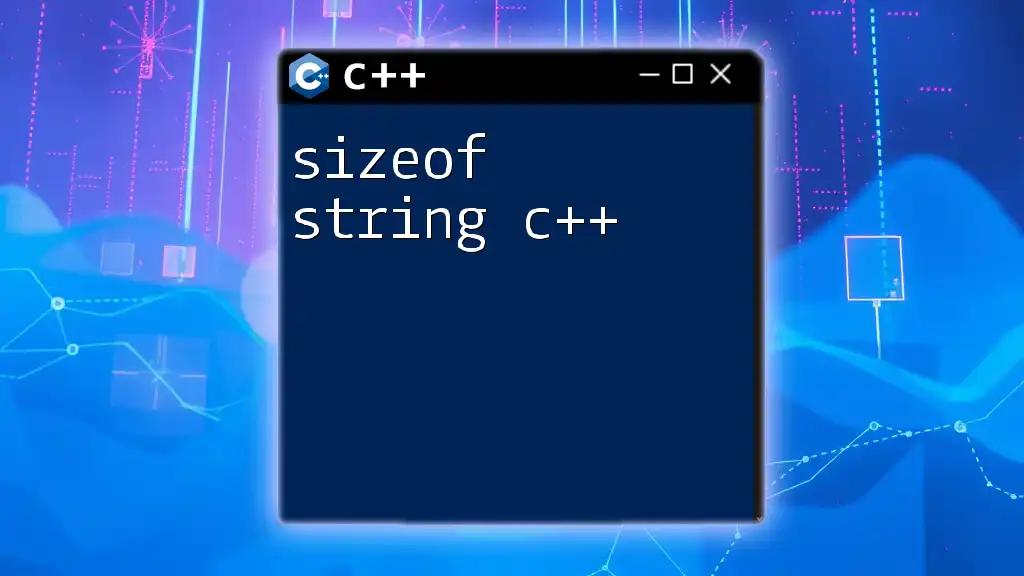
Getting the Size of a String in C++
Using std::string::size() Method
To retrieve the size of a `std::string`, you can utilize the `size()` method. This method returns the number of characters in the string.
#include <iostream>
#include <string>
int main() {
std::string text = "Hello, World!";
std::cout << "Size of the string: " << text.size() << std::endl;
return 0;
}
This code will output the size of the string as 13, which includes all characters, including spaces and punctuation.
Using std::string::length() Method
Interestingly, `std::string` also provides the `length()` method, which serves the same purpose as `size()`. Both methods return the same value, and you can use either depending on your preference.
#include <iostream>
#include <string>
int main() {
std::string text = "Hello, World!";
std::cout << "Length of the string: " << text.length() << std::endl;
return 0;
}
This will yield the same output of 13. It’s vital to note that while both methods do the same thing, `size()` is often more intuitive as it conveys a clearer meaning regarding the number of elements in a container.
Using C-Style Strings
To compute the size of a C-style string, the function `strlen()` can be used. This function takes a pointer to the first character of the string and returns the number of characters before the null terminator.
#include <iostream>
#include <cstring>
int main() {
const char* cstr = "Hello, World!";
std::cout << "Size of the C-style string: " << strlen(cstr) << std::endl;
return 0;
}
The output here will also be 13. It is crucial to remember that handling C-style strings requires special attention to the null terminator to avoid out-of-bounds errors.
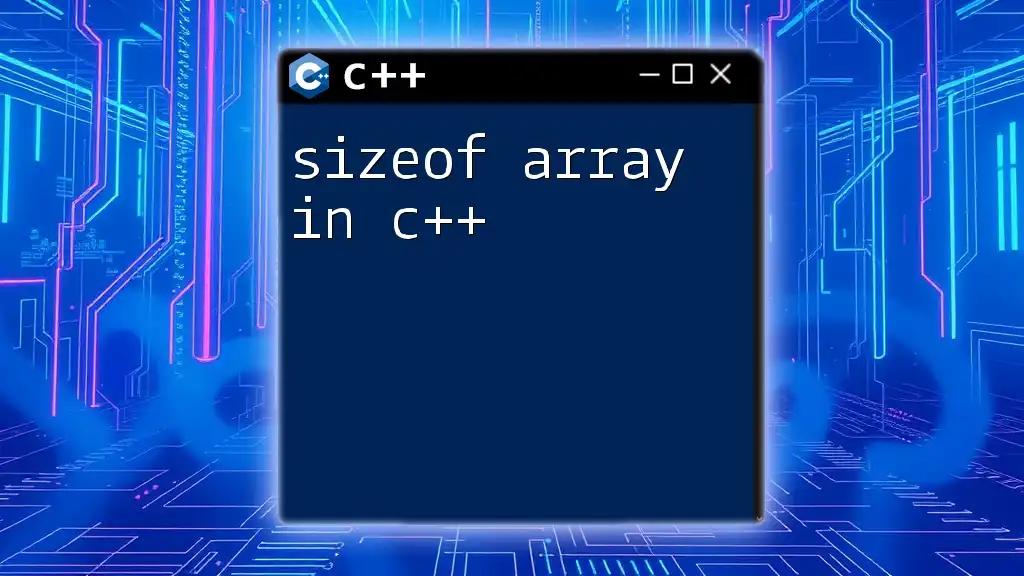
Memory Considerations
Understanding Capacity and Size
The size of a string refers to the number of characters currently held by the string, while capacity refers to the total amount of space allocated for the string. This distinction is significant as the capacity can often exceed the size to avoid frequent reallocations as the string grows. You can use the `capacity()` method to understand memory allocation:
#include <iostream>
#include <string>
int main() {
std::string text = "Hello, World!";
std::cout << "Size: " << text.size() << ", Capacity: " << text.capacity() << std::endl;
return 0;
}
The output will typically show a capacity larger than size, which enhances efficiency when you need to append more characters.
Resizing Strings
If you find it necessary to change the size of a string, you can use the `resize()` method. This method allows you to change the length of the string to the specified size. If the new size is greater, the additional characters can be initialized to a specified character:
#include <iostream>
#include <string>
int main() {
std::string text = "Hello";
text.resize(10, '!');
std::cout << "Resized string: " << text << std::endl;
return 0;
}
In this instance, after resizing, the output will be "Hello!!!!!", demonstrating how the string has been padded with exclamation marks.

Best Practices
Choosing Between std::string and C-Style Strings
When deciding between `std::string` and C-style strings, always prefer `std::string` unless performance considerations dictate otherwise. The safety and ease of use that `std::string` offers make it an ideal choice for most applications. C-style strings can lead to memory management issues and might not be as intuitive to work with.
Performance Considerations
When it comes to performance, `std::string` has overhead due to its dynamic nature, but it is generally more efficient for string manipulation when considering functionality and safety. Understanding copy vs. move semantics can also inform string usage. When passing `std::string` parameters, consider passing by reference to avoid unnecessary copies.
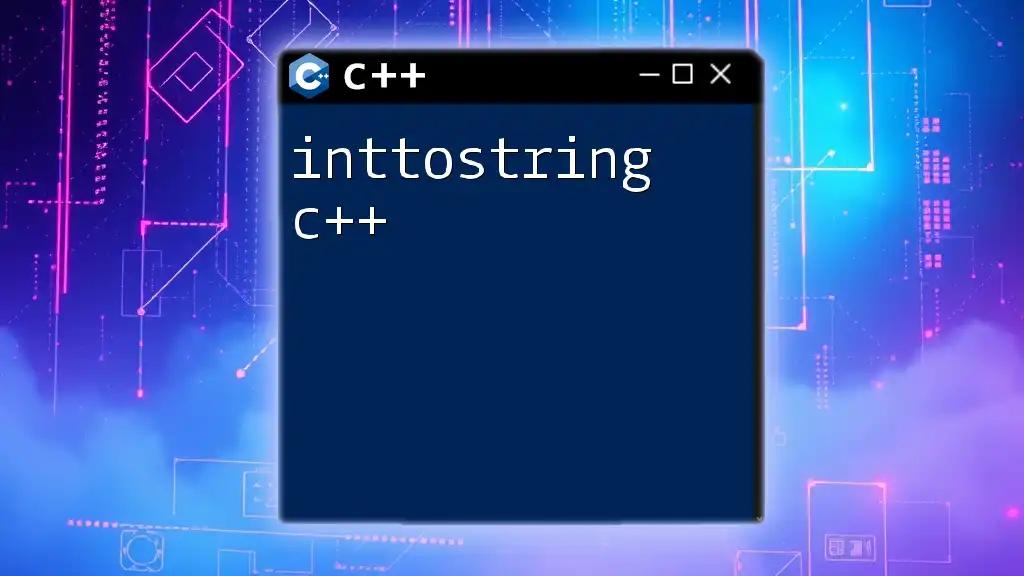
Common Mistakes
Not Accounting for Null Terminators
One of the frequent pitfalls when working with C-style strings is neglecting to manage the null terminator. Failing to include or improperly handling the null terminator can lead to buffer overflows and memory access violations.
Misunderstanding String Mutability
Another common mistake arises from the misunderstanding of string mutability. For example, `std::string` allows modification of strings after creation, but one must be mindful when performing operations that might unintentionally alter or invalidate other strings.

Conclusion
Understanding the size of string in C++ is fundamental for efficient programming. By mastering the various methods for retrieving string sizes and managing string memory, you can write safer and more efficient code. Whether you choose `std::string` or C-style strings will largely depend on the needs of your application, but always prioritize readability and safety in your designs.

Further Reading
To deepen your understanding of strings in C++, consider exploring recommended books, online resources, and community forums. Participating in coding challenges will further cement your knowledge of string management in C++.