In C++, `size_type` is a typedef that represents an unsigned integral type used to express the size of objects or the result of the `size()` function, typically found in standard containers.
Here’s an example of how to use `size_type` in a vector:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::vector<int>::size_type size = numbers.size();
std::cout << "Size of the vector: " << size << std::endl;
return 0;
}
Understanding size_type in C++
size_type is a crucial concept in C++ programming, primarily associated with the Standard Template Library (STL) containers. It represents an unsigned integral data type used to express sizes and counts. This concept is not just a syntactic preference; it embodies significant advantages that enhance type safety and coding practices.

Why Use size_type?
Purpose of size_type
Using size_type provides a robust way to express the sizes of containers. When you declare a variable to represent the size of a container, using size_type ensures that the type is consistent with the actual implementation of the container, no matter the version of C++ or the architecture you are compiling for.
Benefits of size_type
- Avoiding Overflow Issues: Given that size_type is an unsigned type, it helps prevent negative size values, which can lead to logic errors and unpredictable behavior in your program.
- Consistency Across Different Implementations: Different containers may have different internal types for their sizes, and size_type adapts accordingly. This guarantees that your code is portable and adheres to the standard STL behavior.

How size_type is Defined
Standard Template Libraries (STL) and size_type
In C++, every standard library container provides its own definition of size_type. This means that you should always express sizes and indices in terms of the specific container's size_type.
For example, in a `std::vector`, you can define size_type as follows:
using size_type = std::vector<int>::size_type; // Example
This way, the type used for indexes or sizes is consistent with the vector it refers to.
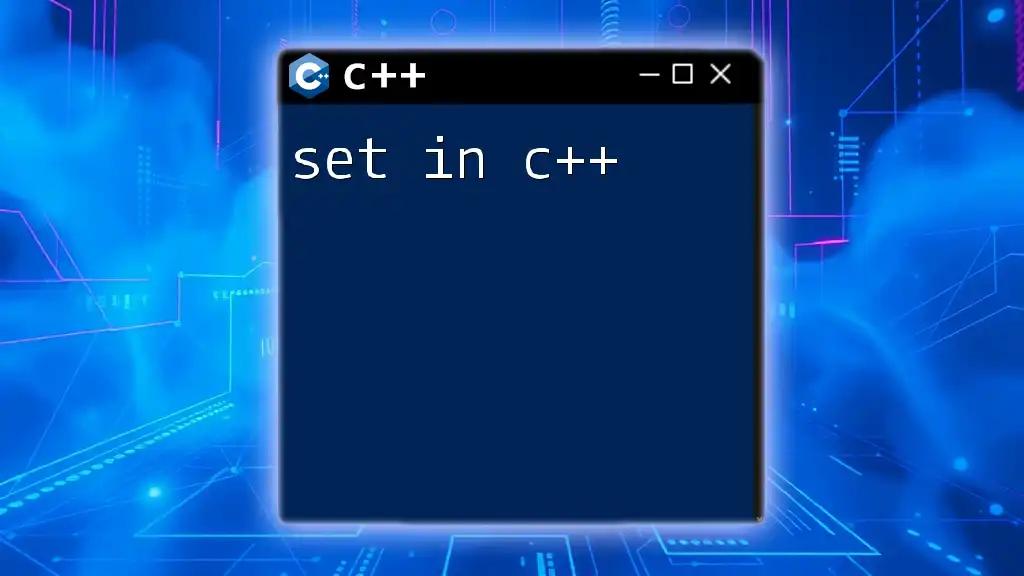
Practical Use Cases of size_type
Iterating Through Containers
When iterating through a container, using size_type for the loop counter is a best practice. This avoids potential issues related to type mismatches and overflow. For instance:
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (std::vector<int>::size_type i = 0; i < numbers.size(); ++i) {
// Accessing elements
std::cout << numbers[i] << " "; // Safely accessing elements
}
Defining Array Sizes and Indices
Using size_type for defining sizes or indices adds clarity and reduces errors. For example, in an array context:
std::array<int, 10> myArray;
std::array<int, 10>::size_type arraySize = myArray.size();
// Now arraySize is guaranteed to be of the right type
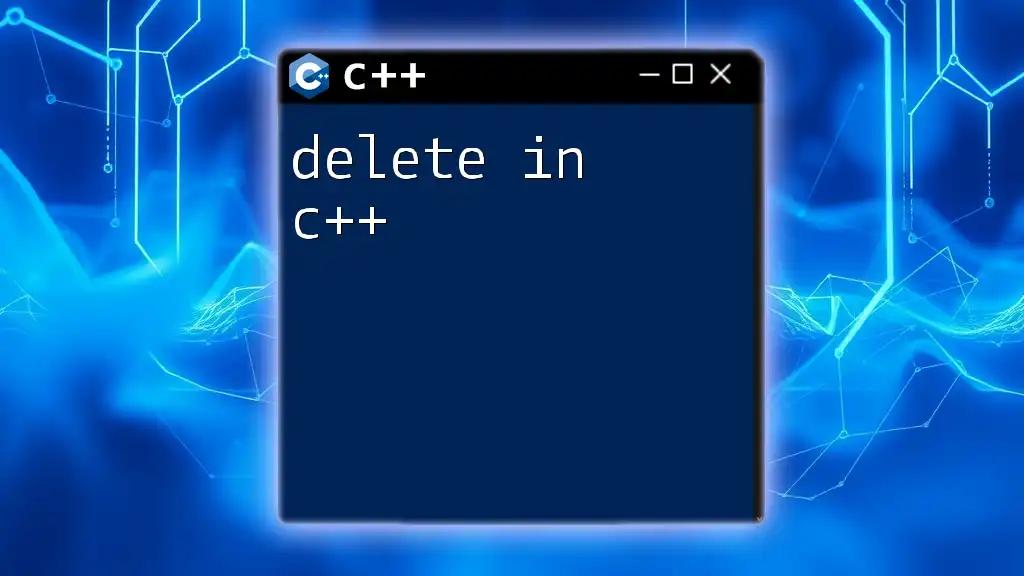
Comparison of size_type with Other Types
size_type vs int
When dealing with counts or indices, one significant advantage of size_type over a basic data type like `int` is that it inherently carries the correct unsigned nature, enhancing type safety.
For instance, if a container can hold up to 2 billion elements, using `int` might lead to overflow if you accidentally exceed that size, potentially leading to undefined behavior:
int index = 2147483647; // Potential overflow scenario
By utilizing size_type, such overflow risks are mitigated.

Common Mistakes to Avoid When Using size_type
Using size_type from Different Containers
One common pitfall developers face is mixing size_type between different containers. For example, using `std::vector<int>::size_type` interchangeably with `std::list<float>::size_type` can cause inconsistencies and create compiler errors. Always stick to the specific container's size_type for accurate results.
Misunderstanding Size and Capacity
It’s vital to distinguish between the size and capacity of containers. The size is the number of elements, while capacity refers to the total allocated space. Using size_type for both ensures clarity but be aware of their distinct meanings.
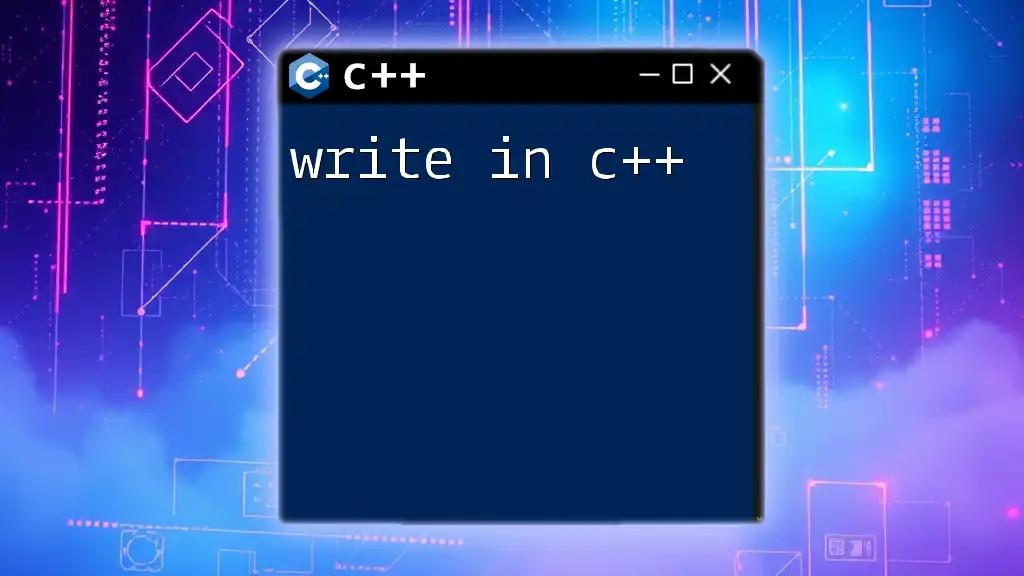
Best Practices for Using size_type in C++
Consistent Use Across Function Parameters
When designing functions dealing with container sizes, it’s beneficial to accept parameters in terms of size_type to enhance robustness and readability:
void processContainer(std::vector<int>::size_type size) {
// Function logic
}
This approach makes your functions adaptable to any STL container you may use.
Explicit Casting
When you are required to cast to size_type—for instance, while performing arithmetic operations—make sure to do it explicitly to convey your intent clearly, which will help maintain code readability and prevent silent errors.

Conclusion
In conclusion, understanding the concept of size_type in C++ is essential for any developer working with STL containers. The benefits of type safety, preventing overflow issues, and ensuring consistency enhance the reliability of your code. By following best practices around its use, you can create cleaner, more professional C++ programs that stand the test of time.