The `is_open` function in C++ is used to check whether a file stream is successfully opened for input or output operations.
#include <fstream>
#include <iostream>
int main() {
std::ifstream file("example.txt");
if (file.is_open()) {
std::cout << "File is open!" << std::endl;
} else {
std::cout << "Failed to open the file." << std::endl;
}
return 0;
}
What is `is_open` in C++?
In C++, managing files is an essential part of programming, especially when it comes to input and output operations. The `is_open` member function plays a crucial role in ensuring that your program interacts with files safely and effectively. Before performing any read or write operations, it's important to check whether a file stream is open to avoid unexpected errors.

Understanding File Streams in C++
What are File Streams?
File streams in C++ are abstractions used to connect to files on the filesystem. They serve as interfaces that allow easy reading from and writing to files. The two main types of file streams are:
- Input File Stream (`ifstream`): Used for reading data from files.
- Output File Stream (`ofstream`): Used for writing data to files.
- File Stream (`fstream`): Combines both `ifstream` and `ofstream`, allowing you to read and write to the same file.
Opening and Closing Files
To work with files in C++, you need to open them using constructors or member functions and close them when your operations are done. For instance:
std::ifstream inputFile("input.txt");
When done, always ensure to close the file:
inputFile.close();
This prevents memory leaks and potential file corruption.

The `is_open()` Member Function
Definition of `is_open()`
The `is_open()` member function checks whether a file stream is currently open. If the stream has been successfully opened, it returns `true`; otherwise, it returns `false`. This function is crucial for error handling in file operations.
How `is_open()` Works
When you invoke `is_open()` on a file stream object, you are querying its internal state. If the stream is valid and successfully linked to a file, it will respond with `true`, allowing you to proceed with reading or writing operations.
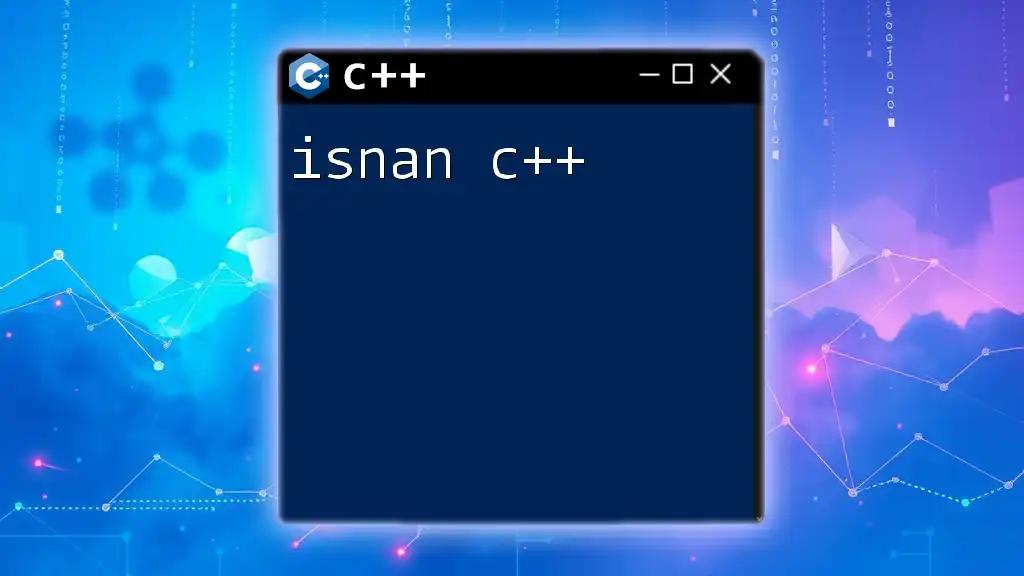
Using `is_open()` in Your Code
Basic Example
To illustrate the use of `is_open()`, consider the following simple program where we attempt to open a file for reading:
#include <iostream>
#include <fstream>
int main() {
std::ifstream file("example.txt");
if (file.is_open()) {
std::cout << "File is open!" << std::endl;
} else {
std::cout << "Unable to open file." << std::endl;
}
file.close();
return 0;
}
In this example, the program checks if "example.txt" is open. If it is, it confirms with a message; otherwise, it informs the user that the file cannot be opened. This check is vital before any further file operations to avoid crashes or undefined states.
Error Handling
Combining `is_open()` with proper error handling is a good practice. Using standard error output can highlight issues effectively:
std::ofstream outputFile("output.txt");
if (!outputFile.is_open()) {
std::cerr << "Error: could not open output.txt for writing!" << std::endl;
}
By checking the state of the file stream, you can gracefully handle errors and inform the user appropriately.

Practical Applications of `is_open()`
Checking File Availability in Programs
In user-driven applications, ensuring that files exist and can be opened is critical to providing a smooth experience. For example, you could implement functionality that allows users to select files for processing. Using `is_open()` ensures that the file is available before proceeding with operations.
Debugging File Operations
When debugging, `is_open()` helps confirm that your file operations are functioning correctly. Here’s a more elaborate example where both reading and writing are involved:
std::fstream file("example.txt", std::ios::in | std::ios::out | std::ios::app);
if (!file.is_open()) {
std::cerr << "Error: Could not open example.txt" << std::endl;
return 1; // Exit the program if the file cannot be opened
}
// Perform read/write operations...
file.close();
In this scenario, the program attempts to open a file for both reading and writing. If it fails, it immediately reports the issue and exits, protecting the integrity of the application's logic.
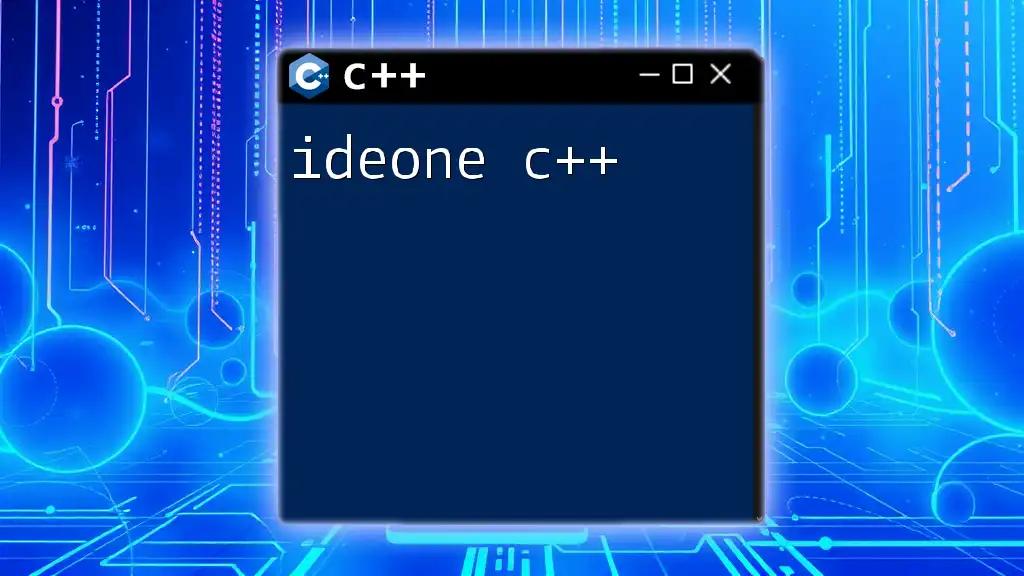
Best Practices for File Handling
Always Check `is_open()` Before Operations
Always checking with `is_open()` before performing any operations on a file stream can save you from potential problems. This ensures that your code is both robust and resilient against crashes due to file issues.
Consistent File Closure
It is essential to close files properly after your operations are complete. This practice releases system resources and prevents data corruption. Failing to close files might lead to memory leaks and other unexpected behavior.
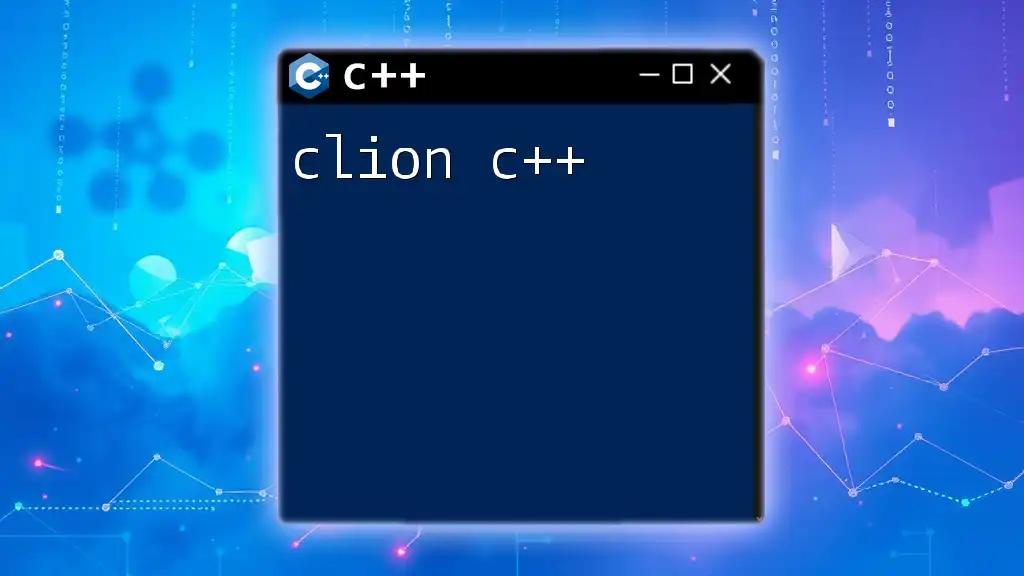
Common Mistakes with `is_open()`
Forgetting to Include the Header
One common error is neglecting to include the necessary header file:
#include <fstream> // Required for file stream operations
Without this, your program will not recognize the file stream types or the `is_open()` function, leading to compilation errors.
Not Handling Different Stream Types
It’s also important to ensure you are using the correct stream type for your operations. For instance, using an `ifstream` where an `ofstream` is required will lead to errors.
Overlooking File Permissions
Remember that file permissions can impede your ability to open files. If a file is locked or you lack the necessary permissions, `is_open()` will return `false`. Always handle these scenarios gracefully in your code.
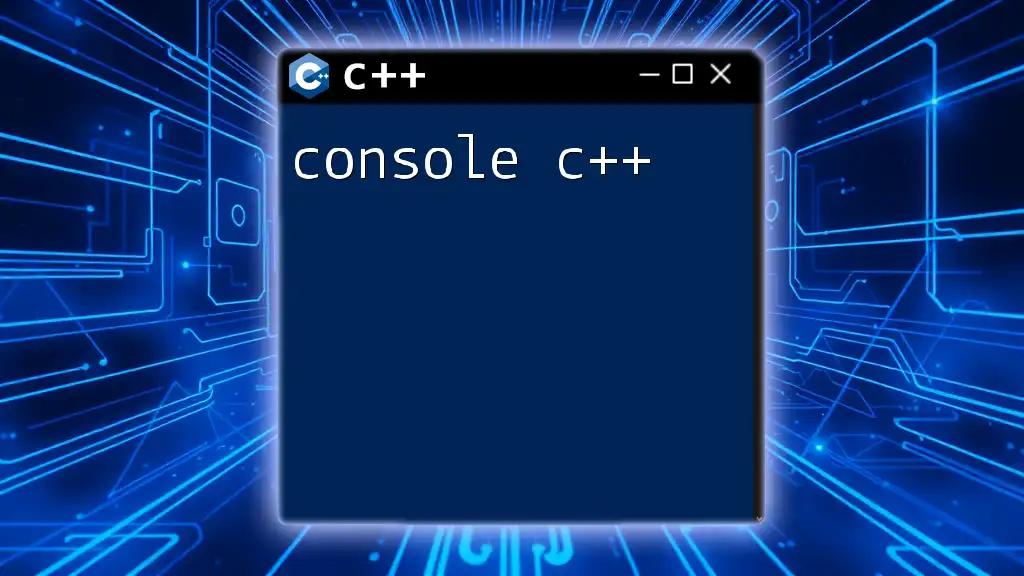
Conclusion
The `is_open()` function is an invaluable tool in C++ file handling, ensuring that you safely and effectively interact with files. By employing this function in your file operations, you can avoid many common pitfalls associated with file handling. Remember to always check if a file is open before proceeding with operations, close your file streams when done, and handle errors appropriately. This foundational knowledge will enhance your programming skills and lead to more reliable applications in C++.
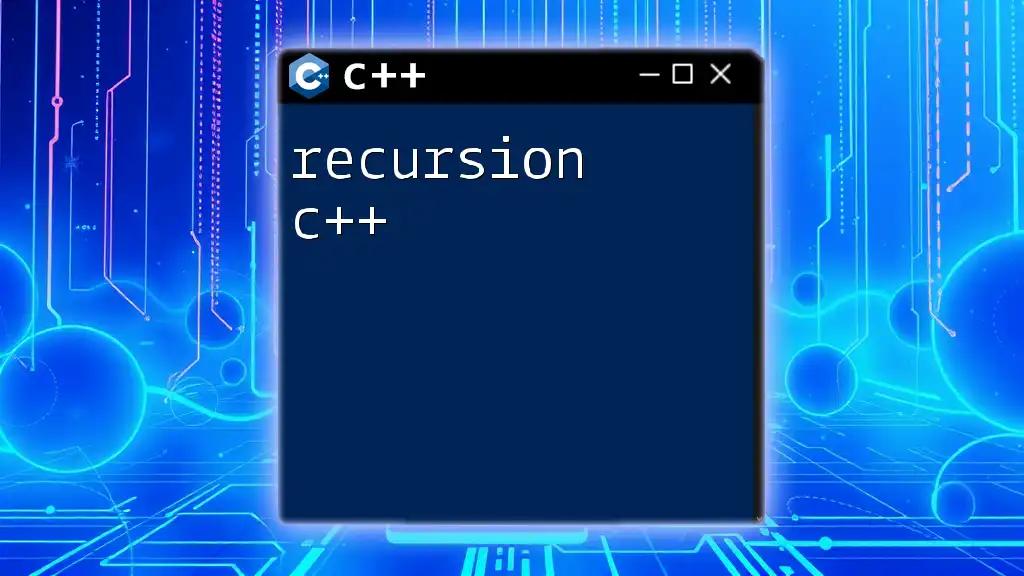
Additional Resources
For more information on file handling in C++, check out the official documentation. Consider enrolling in tutorials and courses dedicated to mastering file I/O operations, as they can provide deeper insights and broader techniques applicable in various programming scenarios.