The VSCode C++ debugger allows developers to efficiently debug their C++ code using breakpoints, variable inspection, and call stack navigation within a user-friendly interface.
Here’s a simple example of a C++ program that can be debugged:
#include <iostream>
int main() {
int a = 5;
int b = 0;
// Set a breakpoint on the next line
int result = a / b; // This line will cause a division by zero error
std::cout << "Result: " << result << std::endl;
return 0;
}
Setting Up VSCode for C++ Development
Installing VSCode
To start using the vscode c++ debugger, first, you need to have Visual Studio Code installed on your machine. You can download it from the [official website](https://code.visualstudio.com/). Make sure your system meets the recommended requirements for optimal performance. VSCode supports multiple operating systems, including Windows, macOS, and Linux, so choose the correct version for your environment.
Installing C++ Extension
Once VSCode is installed, the next step is to enhance its functionality by adding the C++ extension. You can find this extension by searching for "C/C++" in the Extensions Marketplace within VSCode. Developed by Microsoft, this extension not only offers intelligent code completion (intellisense) but also equips the editor with debugging features critical for handling complex C++ projects.
Setting Up the Environment
To utilize the vscode c++ debugger, it’s essential to set up your development environment correctly. Install the necessary build tools like GCC or Clang depending on your platform. For Windows users, installing MSYS2 is a common approach. After installing the tools, ensure that the installation directory is added to your system's environment variables. This allows VSCode to access the C++ compiler seamlessly.
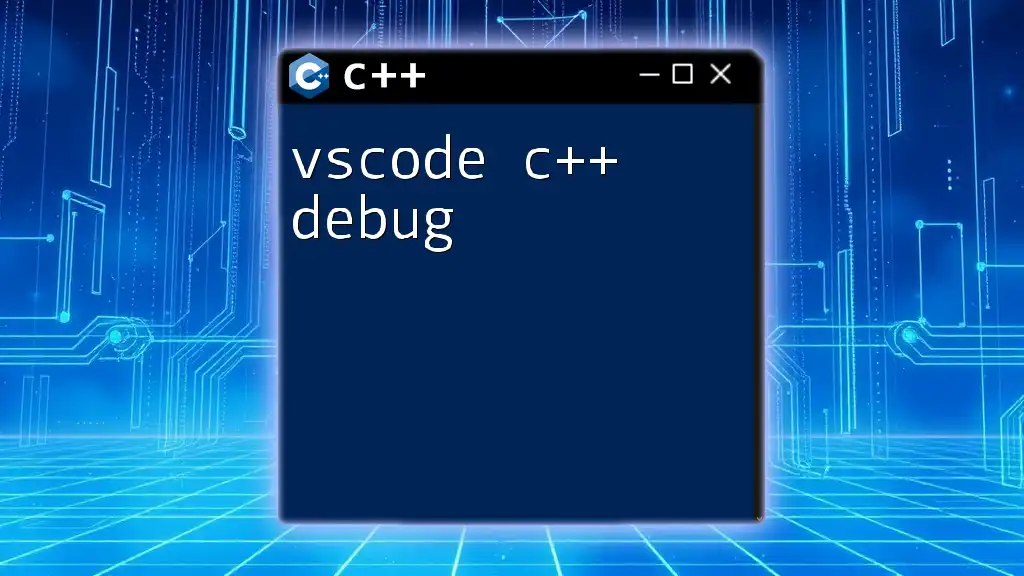
Understanding the VSCode Interface
Navigating the Debugger UI
Familiarizing yourself with the debugger UI in VSCode is crucial for effective debugging. The debugger UI consists of two primary components: the Debug sidebar, which offers an overview of breakpoints, variables, and the call stack, and the Debug toolbar, where you control the debugging session (start, stop, step over, etc.). Understanding these components will enhance your debugging efficiency and control.
Key Features of the VSCode Debugger
Breakpoints
Breakpoints act as markers in your code where the debugger will halt execution. They allow you to inspect variable values and control flow. You can set a breakpoint by clicking next to the line numbers in the editor. To manage breakpoints, you can easily remove or disable them in the Breakpoints section of the sidebar. It’s also possible to use conditional breakpoints, which pause execution only when certain conditions are met, enabling you to focus on specific scenarios.
Call Stack
The call stack shows the active function calls leading to the breakpoint. This feature allows you to trace back through your function calls and see how you arrived at the current execution point. By analyzing the call stack during a debugging session, you can identify potential issues in your code flow.
Variables and Watch
The Variables panel displays the values of your current variables, while the Watch panel enables you to track specific expressions and variables in real-time. This capability is invaluable when debugging scenarios that involve changing data states, as it provides insight into how variable values evolve during execution.
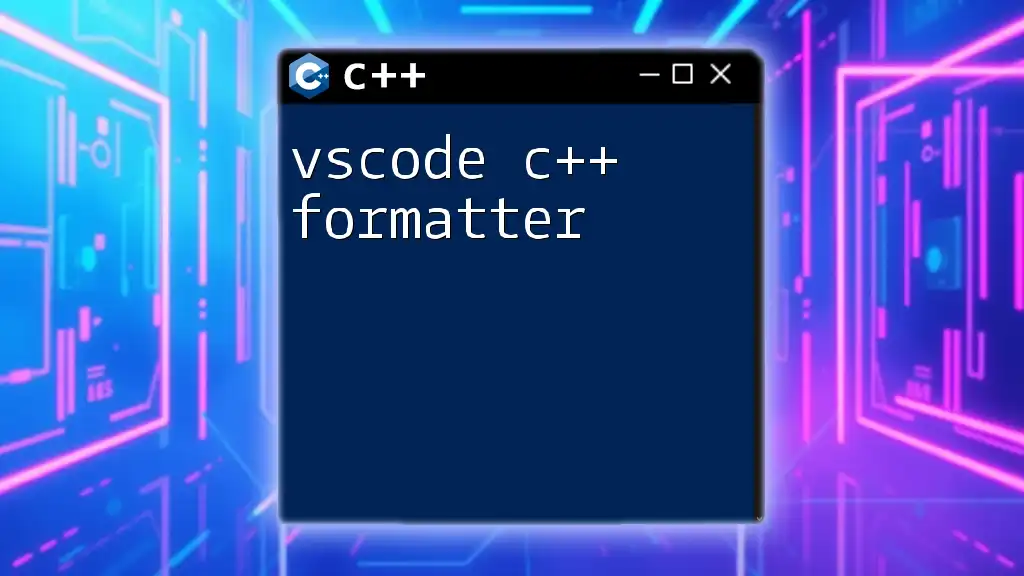
Configuring the Debugger
Launch Configuration
The `launch.json` file is where you define how your project should be executed in debug mode. This configuration file is located in the `.vscode` directory of your project. Here is a sample configuration for a simple C++ program:
{
"version": "0.2.0",
"configurations": [
{
"name": "Debug C++",
"type": "cppdbg",
"request": "launch",
"program": "${workspaceFolder}/a.out",
"args": [],
"stopAtEntry": false,
"cwd": "${workspaceFolder}",
"environment": [],
"externalConsole": false,
"MIMode": "gdb",
"setupCommands": [
{
"description": "Enable pretty-printing for gdb",
"text": "-enable-pretty-printing",
"ignoreFailures": true
}
],
"preLaunchTask": "build"
}
]
}
Make sure to adjust the `program` path based on your output filenames.
Modify Build Configurations
To build your C++ projects seamlessly from within VSCode, you should create a `tasks.json` file. This file defines how to compile your project. Here's an example of a simple build configuration:
{
"version": "2.0.0",
"tasks": [
{
"label": "build",
"type": "shell",
"command": "g++",
"args": [
"-g",
"${file}",
"-o",
"a.out"
],
"group": {
"kind": "build",
"isDefault": true
},
"problemMatcher": ["$gcc"]
}
]
}
This configuration compiles the currently active C++ file with debugging symbols.
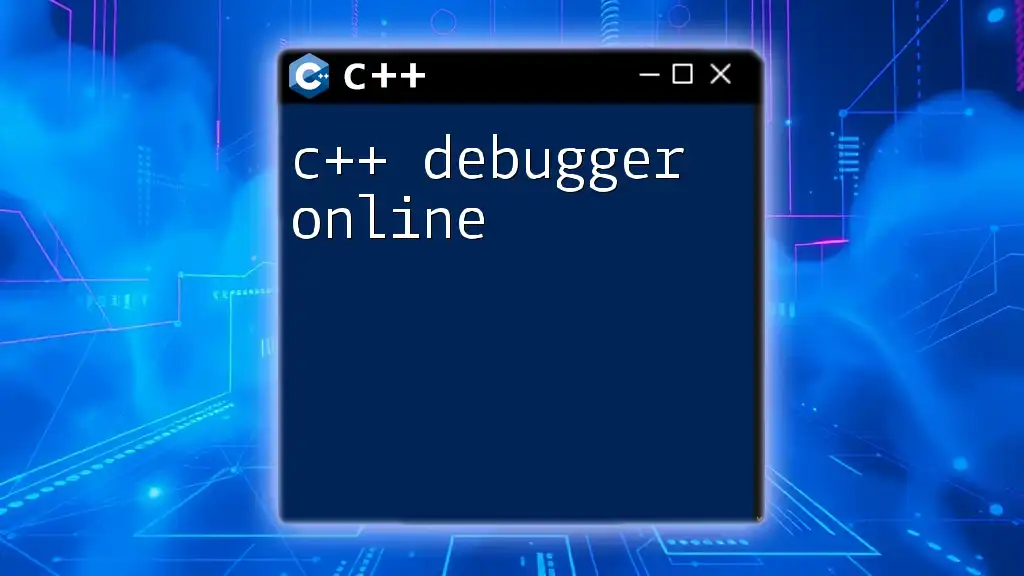
Basic Debugging Techniques
Starting a Debugging Session
To start debugging, simply press `F5`, or go to the Debug sidebar and click the green play button. If configured correctly, this will compile your code if it has not been built yet and launch the debugger. Familiarize yourself with controlling the session using the debug toolbar—this includes starting, stopping, and restarting your debugging session.
Using Breakpoints Effectively
Setting breakpoints at strategic locations in your code is key to spotting issues efficiently. Use breakpoints to pause execution before critical decisions or results to inspect the program’s state. For example, if your program encounters a segmentation fault, place a breakpoint before the suspected line and review variable states iteratively.
Inspecting Variables and Expressions
During a debugging session, hovering over a variable will display its current value, enabling quick inspections. To monitor variables actively, utilize the Watch panel by adding expressions or variables that are critical to your debugging process. This allows you to track changes to important data without modifying your code.
Step Over, Step Into, and Step Out
Utilize the various stepping commands to navigate through your program.
- Step Over (`F10`): Executes the next line of code but doesn’t enter any function calls.
- Step Into (`F11`): Enters the function at the current line to allow you to debug within that function.
- Step Out (`Shift + F11`): Completes execution of the current function and returns control to the calling function.
For example, if you want to debug a complex recursive function, using Step Into will let you closely analyze each recursive call.
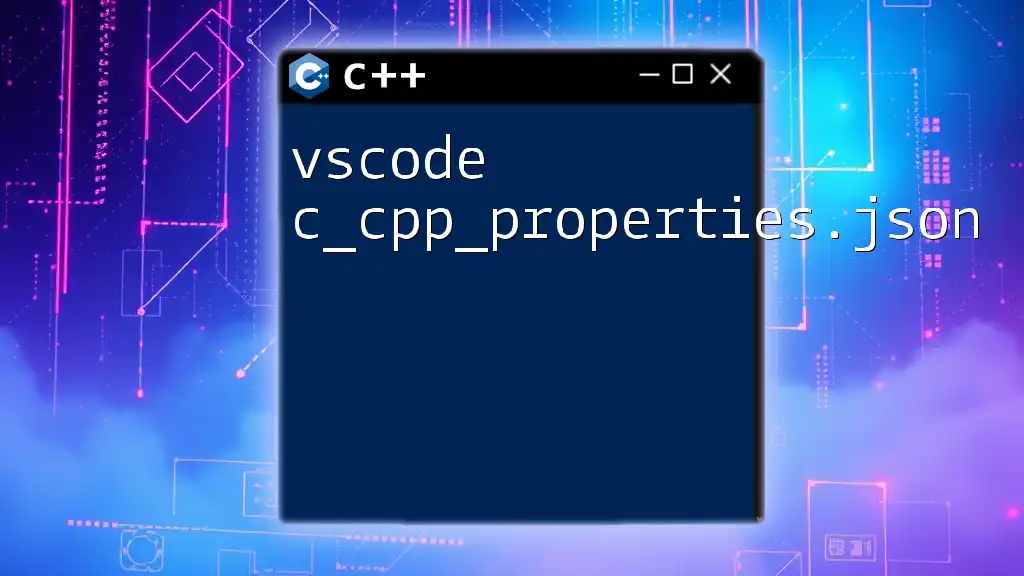
Advanced Debugging Techniques
Conditional Breakpoints
Conditional breakpoints can be created by right-clicking on an existing breakpoint and selecting "Edit Breakpoint." You can specify conditions (e.g., `x == 5`) that must be true for the debugger to pause execution. This technique is especially useful for examining loops where a particular state is of interest.
Debugging with the Integrated Terminal
The integrated terminal in VSCode allows you to run terminal commands while debugging. You can utilize this for logging outputs or executing debug commands without leaving the debug environment. Using `cout` statements for debugging along with the integrated terminal can provide real-time feedback during execution.
Exception Breakpoints
Setting breakpoints on exceptions will halt execution whenever an exception occurs, providing a chance to analyze the situation. In the debug panel, you can configure exception breakpoints by clicking the gear icon next to the Breakpoints section. This capability is crucial to catching runtime errors and understanding their context when they happen.
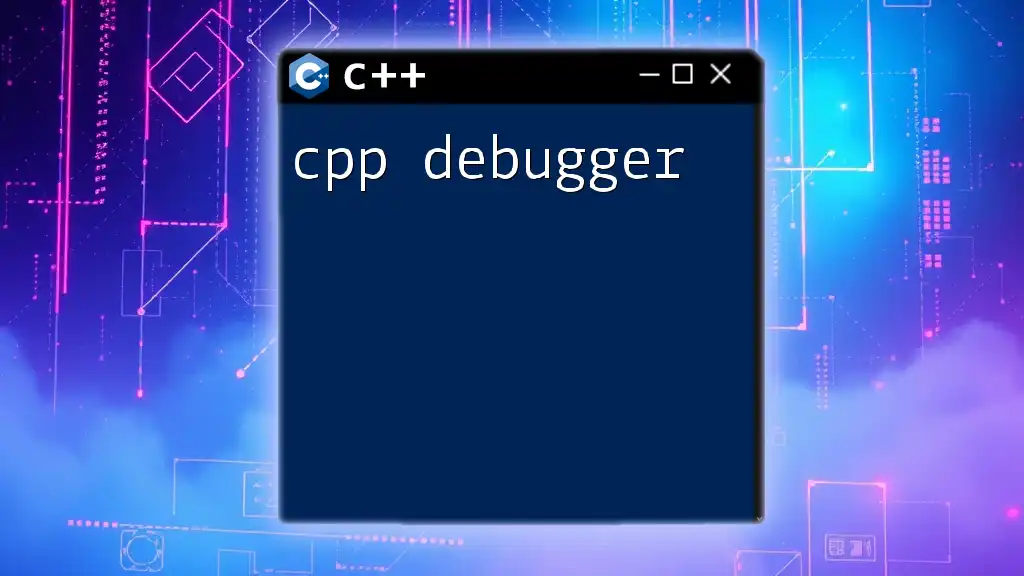
Debugging Tips and Best Practices
Frequently Used Shortcuts
Mastering keyboard shortcuts is critical for efficient debugging in VSCode. Familiarize yourself with the following:
- F5: Start/Continue
- F10: Step Over
- F11: Step Into
- Shift+F11: Step Out
- Ctrl+Shift+B: Build the project
You can also customize shortcuts in the keyboard shortcuts settings to fit your workflow better.
Common Debugging Pitfalls
During debugging, it’s common to overlook simple mistakes. Be aware of pitfalls like:
- Missing initialization of variables, leading to undefined behavior.
- Incorrect assumptions about object lifetimes.
- Not removing final breakpoints after debugging a section, which can lead to confusion on subsequent runs.
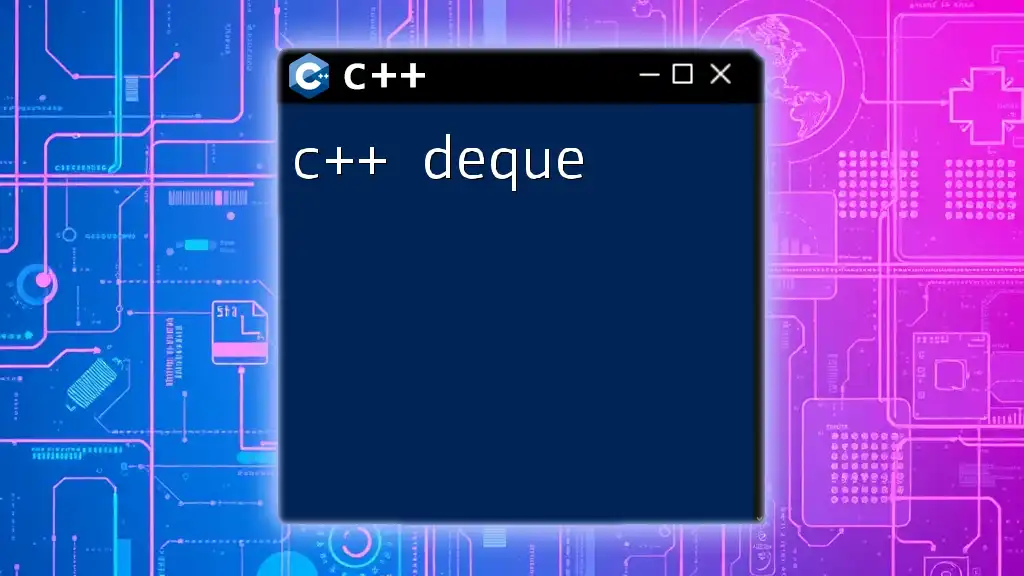
Case Study: Debugging a Sample C++ Program
Overview of the Sample Program
Let’s consider a simple program that calculates the factorial of a number. Here’s the code snippet:
#include <iostream>
int factorial(int n) {
if (n < 0) return -1; // Error case
if (n == 0) return 1;
return n * factorial(n - 1);
}
int main() {
int num;
std::cout << "Enter a number: ";
std::cin >> num;
int result = factorial(num);
std::cout << "Factorial: " << result << std::endl;
return 0;
}
Step-by-Step Debugging Walkthrough
- Start by setting a breakpoint at the beginning of the `factorial` function.
- Run the debugger. Upon hitting the breakpoint, inspect the value of `n`.
- Use Step Into to analyze recursive calls. You can confirm whether the recursion is functioning as expected.
- Check edge cases like when `n` is `0` or negative. Use conditional breakpoints to pause when a negative value is encountered.
- Observe the returned values to validate correctness throughout the recursion.
By diligently following these steps, you can pinpoint any logical flaws while grasping the recursion conceptively.
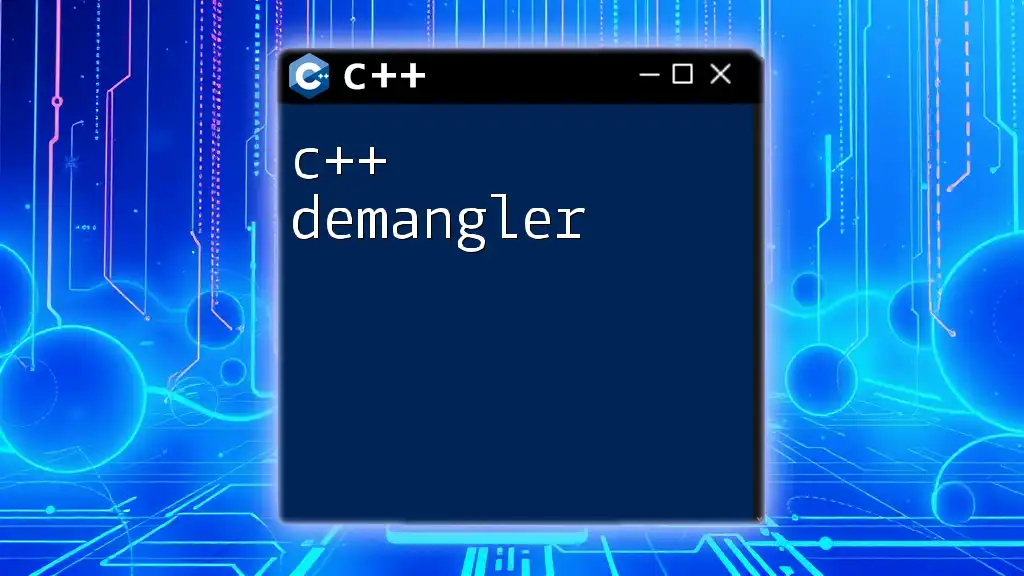
Conclusion
Utilizing the vscode c++ debugger streamlines your debugging process significantly. With a robust set of features, such as breakpoints, call stack examination, and the ability to inspect variables, debugging becomes a manageable task rather than an overwhelming challenge. Practicing these techniques will enhance your programming skills and enable you to develop robust C++ applications with confidence.
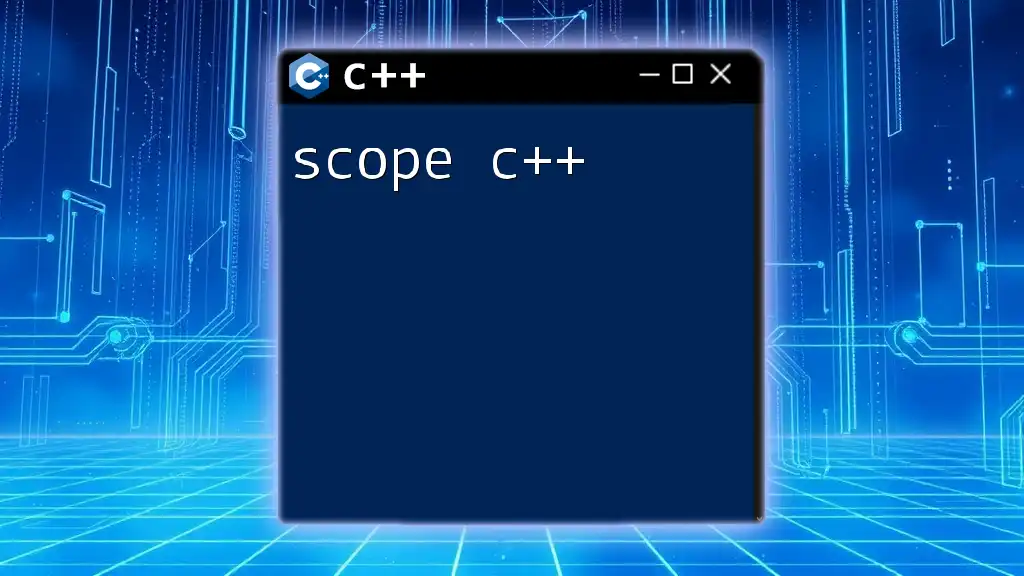
Additional Resources
To further deepen your understanding, consider exploring community forums, official documentation, and tutorials specific to C++ debugging. Engaging with other developers can provide new insights and techniques that enhance your debugging prowess.