A simple C++ program typically includes a basic structure that outputs a message to the console, demonstrating fundamental C++ syntax.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is a Simple C++ Program?
A simple C++ program is a compact piece of code that demonstrates fundamental programming concepts using C++. These programs are typically short, straightforward, and focus on basic command usage, making them ideal for beginners. Simple programs can serve as building blocks for more complex applications.
Characteristics of simple C++ programs include:
- Short and concise code: They typically consist of minimal lines while showcasing essential programming techniques.
- Basic command usage: They involve basic input/output operations, variable declarations, and control structures.
Examples of Simple C++ Programs
A classic example of a simple C++ program is the "Hello, World!" program, which prints a greeting to the console.
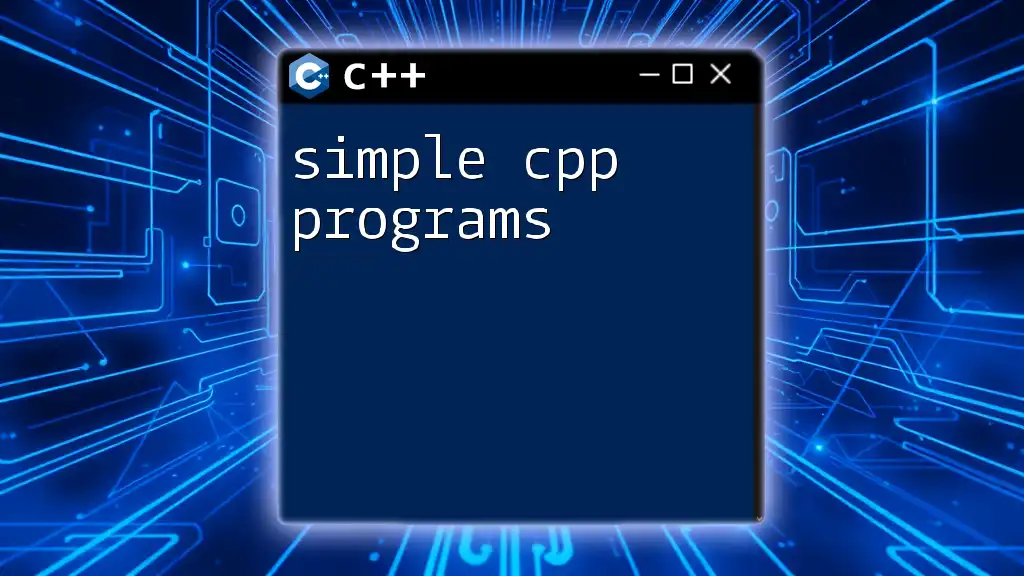
Setting Up Your C++ Environment
Installing a C++ Compiler
Before diving into writing your first simple C++ program, you'll need a C++ compiler. Here’s how to install one on various operating systems:
-
Windows: Install MinGW
- Download the MinGW installation manager from the MinGW website.
- Choose the components to install, ensuring `mingw32-base` and `mingw32-gcc-g++` are selected.
- Follow the prompts to complete the installation.
-
Linux: Install GCC via APT
sudo apt update sudo apt install g++
-
macOS: Install GCC using Homebrew
brew install gcc
After installing, verify your installation by running `g++ --version` in your command line to ensure the compiler is working correctly.
Choosing an Integrated Development Environment (IDE) or Text Editor
Selecting the right IDE or text editor can streamline your coding experience. Here are a few recommendations:
- IDEs: Visual Studio and Code::Blocks offer comprehensive environments with built-in debugging and project management features.
- Text editors: Lightweight options like Visual Studio Code and Sublime Text are great for coding without the added complexity of a full IDE.
Once you've installed your preferred option, configure it for C++ by ensuring it points to your compiler and has support for C++ file types.
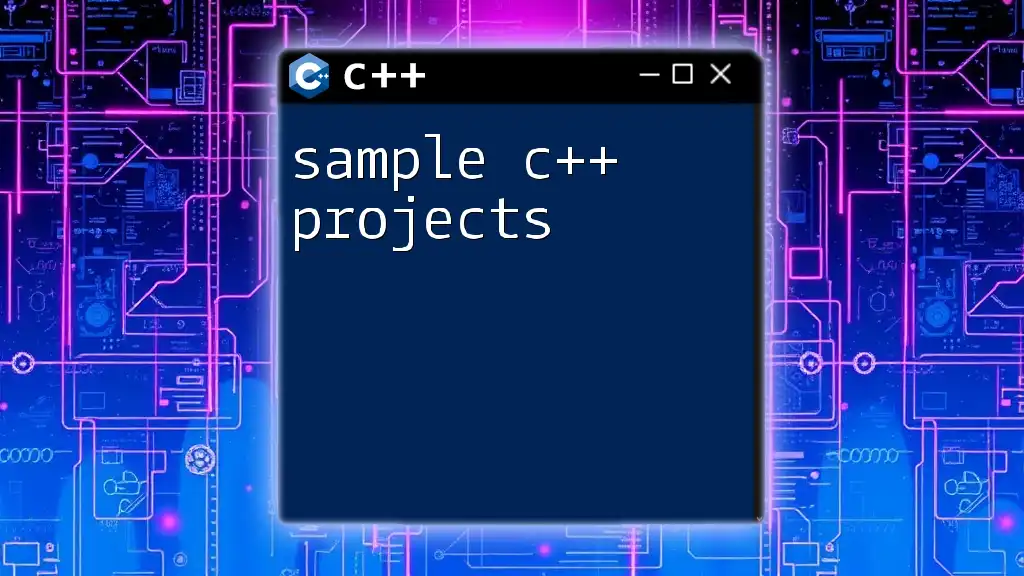
Structure of a Simple C++ Program
Basic Components
Understanding the structure of a simple C++ program is critical. The core elements include:
-
Header Files: These are included at the top using the `#include` directive, which allows you to use libraries. For example, including the iostream library permits input and output operations:
#include <iostream>
-
The main() Function: This is where the execution of your program begins. It is essential for every C++ program.
-
Return Type: The `main` function usually returns an integer. This signifies the exit status of the program:
int main() { return 0; // The program completed successfully }
Example of a Simple C++ Program
Here’s a basic program that illustrates these components:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this example, `#include <iostream>` allows the use of the standard input/output stream. The `main()` function is defined, and inside it, the program prints "Hello, World!" to the console.
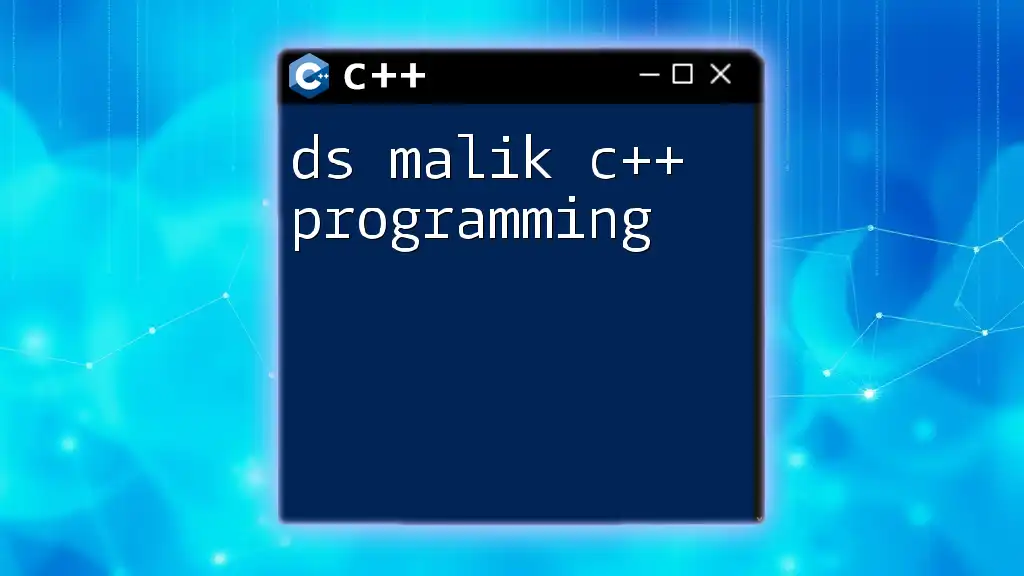
Common Features of Simple C++ Programs
Input and Output Operations
In C++, you can perform input and output operations using `std::cout` (for output) and `std::cin` (for input). Here’s an example demonstrating basic user input:
#include <iostream>
int main() {
int number;
std::cout << "Enter a number: ";
std::cin >> number;
std::cout << "You entered: " << number << std::endl;
return 0;
}
This program prompts the user to enter a number and displays it back, illustrating the flow of input and output in a simple C++ program.
Basic Data Types
C++ supports various data types. The most common ones include `int`, `float`, and `char`. Here’s an example of declaring and using variables, showcasing basic data types:
#include <iostream>
int main() {
int age = 25; // An integer
float height = 5.9; // A floating-point number
char initial = 'A'; // A character
std::cout << "Age: " << age << ", Height: " << height << ", Initial: " << initial << std::endl;
return 0;
}
Understanding how to declare variables of different data types is vital for constructing simple C++ programs.
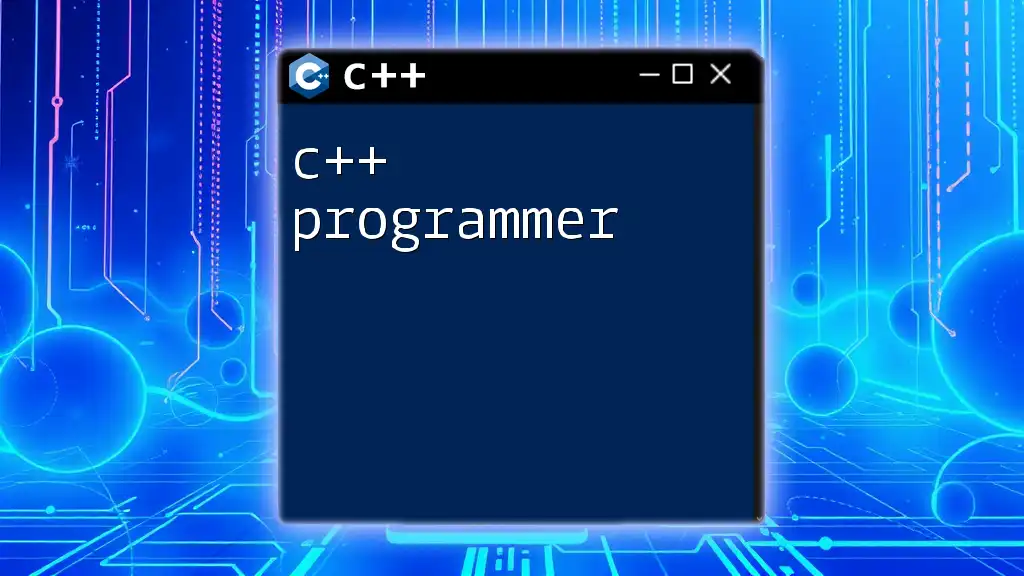
Control Structures in Simple C++ Programs
Conditional Statements
Conditional statements, like `if`, `else`, and `switch`, allow your program to make decisions. Here is an example using an `if` statement:
#include <iostream>
int main() {
int age;
std::cout << "Enter your age: ";
std::cin >> age;
if (age < 18) {
std::cout << "You are a minor." << std::endl;
} else {
std::cout << "You are an adult." << std::endl;
}
return 0;
}
This code checks the user's age and responds accordingly, demonstrating how to implement conditional logic in a simple C++ program.
Looping Constructs
C++ provides several looping constructs, including `for`, `while`, and `do-while` loops. Here’s an example using a `for` loop to count from 1 to 5:
#include <iostream>
int main() {
for (int i = 1; i <= 5; ++i) {
std::cout << "Counting: " << i << std::endl;
}
return 0;
}
This loop iterates five times, displaying a count each time, which is critical for repetitive tasks in programming.
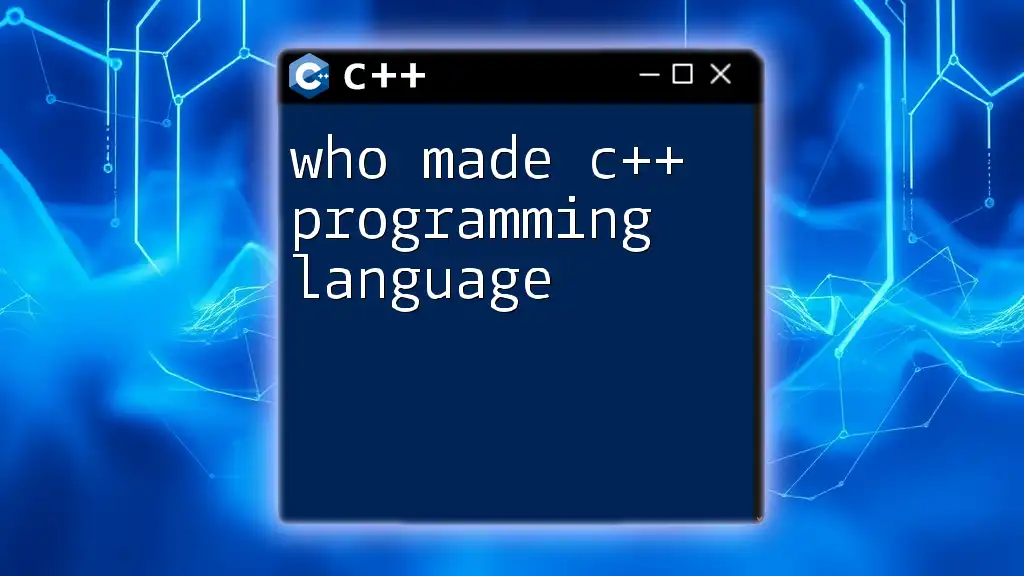
Best Practices for Writing Simple C++ Programs
Code Readability
Writing readable code is essential. Use clear variable names and include comments to explain your code. This makes it easier for others (or yourself in the future) to understand your thought process. Maintain consistent formatting and indentation to enhance clarity further.
Error Handling
Handling input errors is crucial in programming. Always check for potential errors when accepting input. Here is an example of how to manage simple error handling:
#include <iostream>
int main() {
int number;
std::cout << "Enter an integer: ";
while (!(std::cin >> number)) {
std::cout << "Invalid input. Please enter an integer: ";
std::cin.clear(); // clear the error flag
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n'); // discard invalid input
}
std::cout << "You entered: " << number << std::endl;
return 0;
}
In this snippet, the program prompts the user until valid input is received, demonstrating effective input validation.
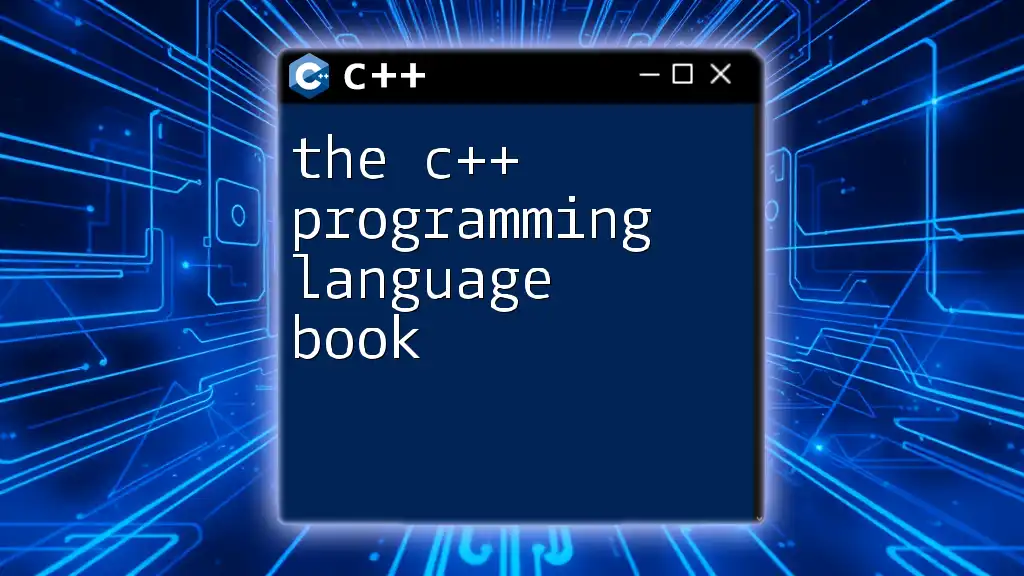
Conclusion
In this guide on simple C++ programs, we covered the fundamental concepts and structures that form the basis of C++ programming. With a focus on clarity, we discussed the essential components, the setup of your development environment, and the common characteristics that make C++ an enduring language.
Now, with a solid understanding of simple programming principles, you are encouraged to explore more complex topics and continue your journey in the world of C++. Remember, practice is key to mastering programming. Visit our website for more resources and tutorials catered to your learning journey!
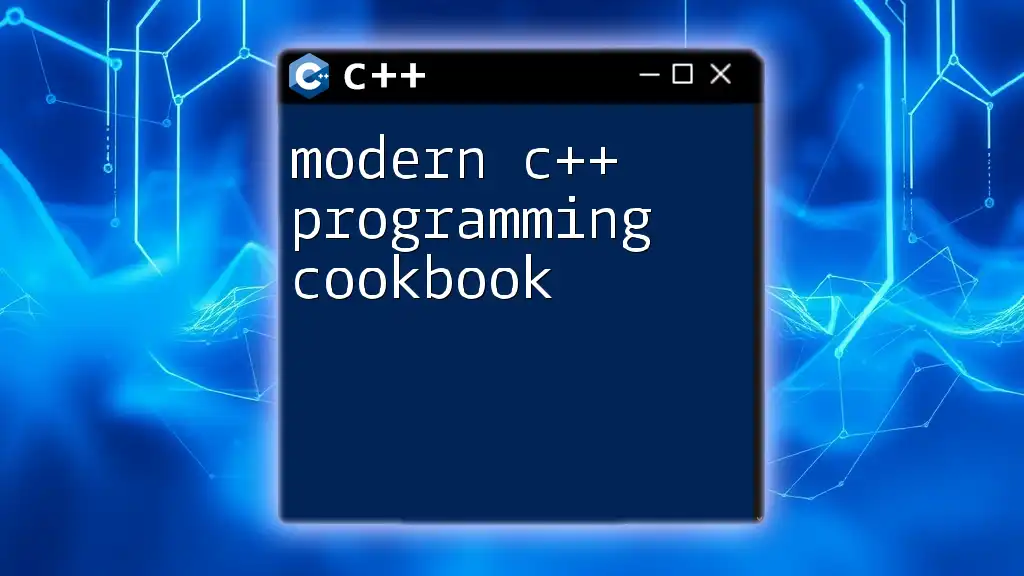
Additional Resources
For further reading and learning opportunities, consider exploring:
- Documentation: Sites like cppreference.com and the official C++ documentation.
- Books: Look for beginner-friendly C++ books that can guide you through various concepts.
- Communities: Engage in forums such as Stack Overflow and Reddit’s r/cpp to connect with fellow learners and experienced developers.
With the right knowledge and resources, you can become proficient in C++ and tackle even the most challenging programming projects!