In this post, we will provide concise C++ code examples to illustrate fundamental commands and syntax for beginners.
Here's a simple example to demonstrate how to print "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding the Basics of C++
What is C++?
C++ is a powerful general-purpose programming language that supports procedural, object-oriented, and generic programming. Initially developed by Bjarne Stroustrup in the late 1970s as an extension of the C programming language, C++ brings the benefits of object-oriented programming (OOP) to the table.
Key Features of C++:
- Object-Oriented Programming: This enables the encapsulation of data and functions, thus simplifying code management and enhancing reusability.
- Low-level Memory Manipulation: C++ gives developers direct access to memory, enabling fine-tuning of performance, which is crucial for system-level programming or embedded systems.
C++ Syntax Overview
A basic C++ program consists of the following components:
- Preprocessor Directives: These tell the compiler to include certain files or libraries before compilation.
- Main Function: All C++ programs start executing from the `main()` function.
- Statements: The lines of code that perform operations.
- Return Statement: This signifies the end of the `main()` function and typically returns 0 to indicate success.
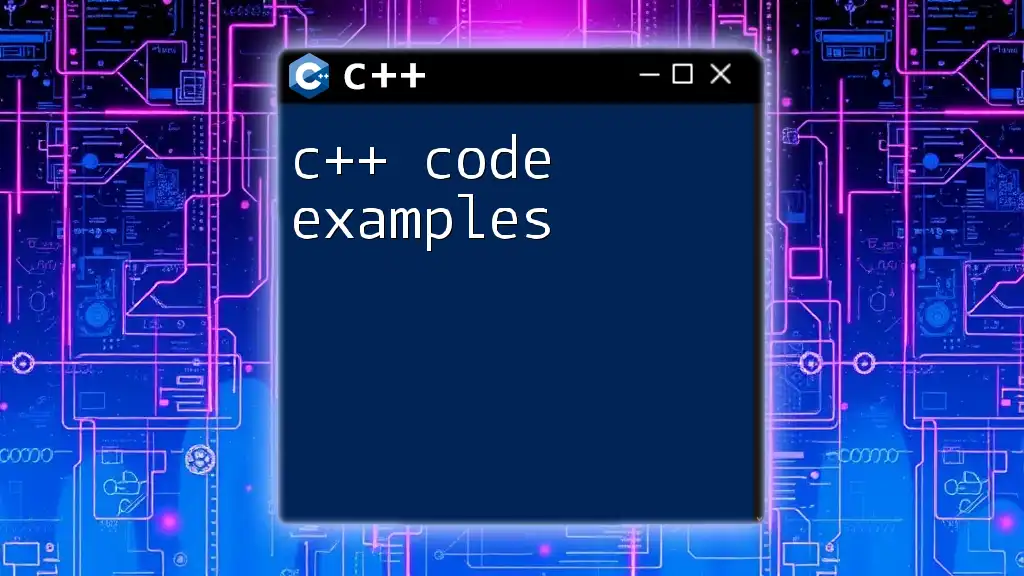
Setting Up the Development Environment
Required Software
To begin programming in C++, you'll need a compiler. For instance:
- g++: A common compiler for Linux and Windows, part of the GNU Compiler Collection.
- Microsoft Visual Studio: A robust IDE that includes a built-in C++ compiler.
Installing C++ IDEs
Various Integrated Development Environments (IDEs) can simplify coding by providing helpful features like debugging tools, syntax highlighting, and code completion:
- Code::Blocks: A free, open-source cross-platform IDE that supports multiple compilers.
- CLion: A powerful IDE from JetBrains providing extensive C++ features, although it is commercial software requiring a subscription.
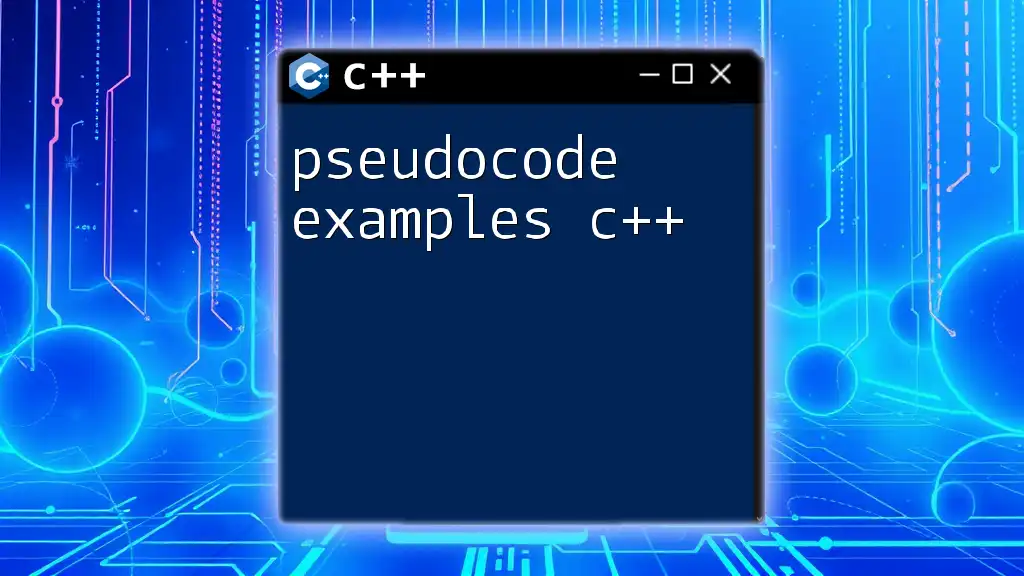
Fundamental C++ Sample Code Examples
Hello World Program
The quintessential example when learning any language is the "Hello, World!" program. It displays a simple message to the user, showcasing how to output text.
Here’s the code:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Explanation:
- `#include <iostream>` includes the input-output stream library, enabling the program to use `cout`.
- `using namespace std;` allows access to standard library features without needing to prepend `std::` every time.
- `cout` prints the message, and `return 0;` indicates the program ended successfully.
Data Types and Variables
C++ supports several data types for storing different kinds of information:
int age = 25;
double salary = 50000.50;
char gender = 'M';
Explanation:
- int for integer values, double for floating-point numbers, and char for single characters. Understanding data types is crucial for effective memory management and code efficiency.
Basic Input and Output
C++ uses `cin` and `cout` for input and output operations. Here’s how you can take user input:
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
cin >> age;
cout << "You are " << age << " years old." << endl;
return 0;
}
Explanation:
- This program prompts the user to enter their age. It captures the input using `cin` and displays the response using `cout`.
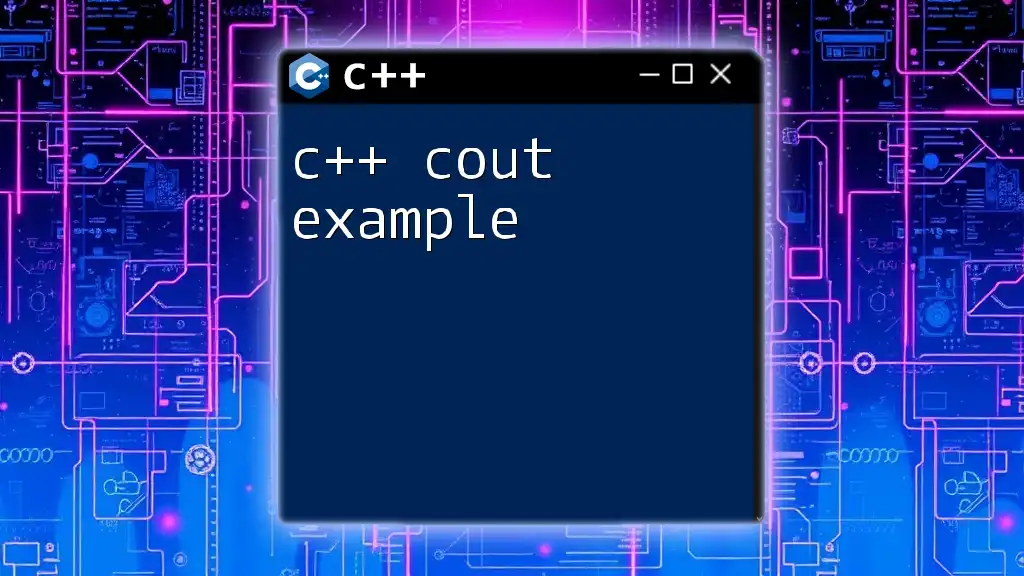
Control Structures in C++
Conditional Statements
Conditional statements allow your program to make decisions based on certain conditions. The if-else structure is the most common.
Here’s a snippet:
int num;
cout << "Enter a number: ";
cin >> num;
if (num > 0) {
cout << "Positive number." << endl;
} else if (num < 0) {
cout << "Negative number." << endl;
} else {
cout << "Zero." << endl;
}
Explanation:
- Depending on the number entered, the program will determine if it is positive, negative, or zero, demonstrating how flow control works in C++.
Loops in C++
Loops are essential for executing a block of code multiple times. They come in three forms: for, while, and do-while loops.
Here’s a basic for loop:
for (int i = 0; i < 5; i++) {
cout << "Iteration " << i + 1 << endl;
}
Explanation:
- The loop iterates five times, printing the iteration number. This showcases the ability to repeat actions—an essential piece of most programs.
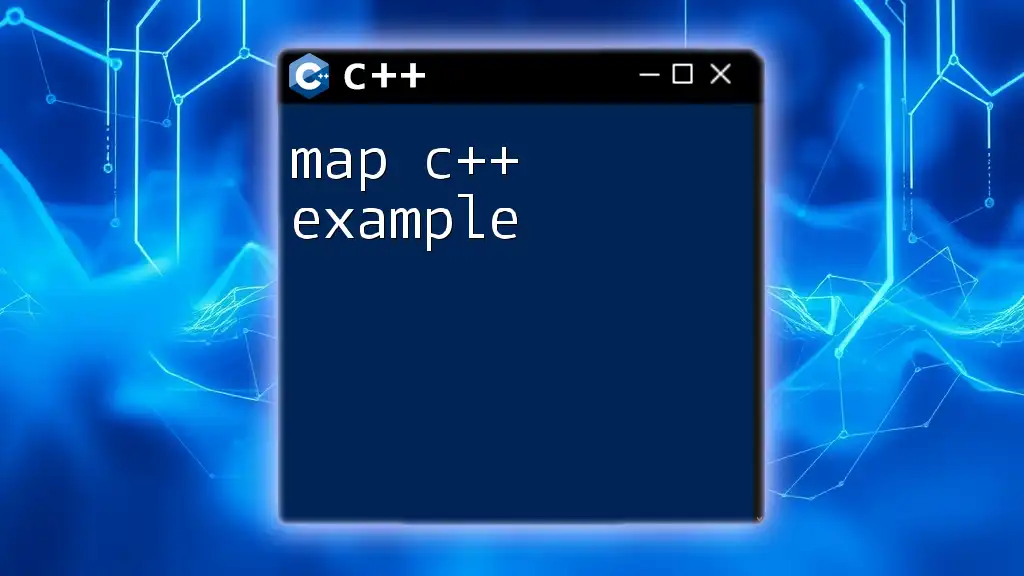
Advanced C++ Sample Code Examples
Functions
Functions allow code to be modularized by breaking it into reusable pieces. Here’s an example:
int add(int a, int b) {
return a + b;
}
int main() {
cout << "Sum: " << add(5, 3) << endl;
return 0;
}
Explanation:
- The `add` function takes two integers as arguments and returns their sum. This modular approach makes code easier to maintain and understand.
Classes and Objects
C++ employs the principles of object-oriented programming, which helps structure code into objects that represent real-world entities. Here’s an example of a simple class:
class Car {
public:
string model;
int year;
void display() {
cout << "Model: " << model << ", Year: " << year << endl;
}
};
int main() {
Car myCar;
myCar.model = "Toyota";
myCar.year = 2022;
myCar.display();
return 0;
}
Explanation:
- The `Car` class includes attributes (model and year) and a method to display this information. Creating classes fosters better organization and encapsulation of data.
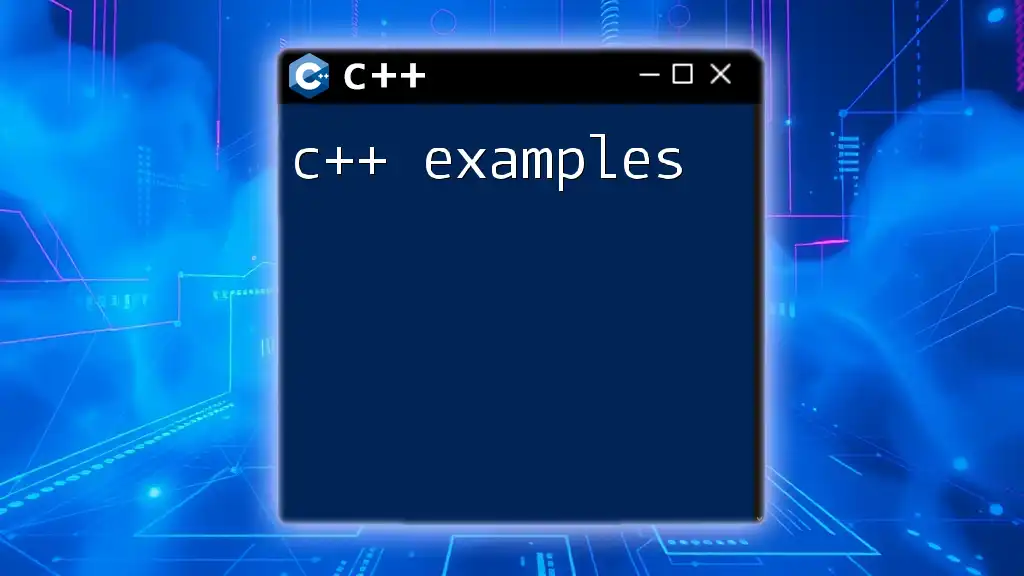
Efficient Coding Practices
Using Good Naming Conventions
Adopting meaningful and consistent naming conventions significantly enhances code readability and maintainability. Variables should reflect their purpose. For instance, `totalAmount` is preferable over `ta`.
Commenting Your Code
Providing comments within your code helps others (and yourself) understand its logic. C++ supports single-line and multi-line comments:
// This is a single-line comment
/*
This is a
multi-line comment
*/
Explanation:
- Comments clarify the intended functionality, improving code comprehension and future updates.
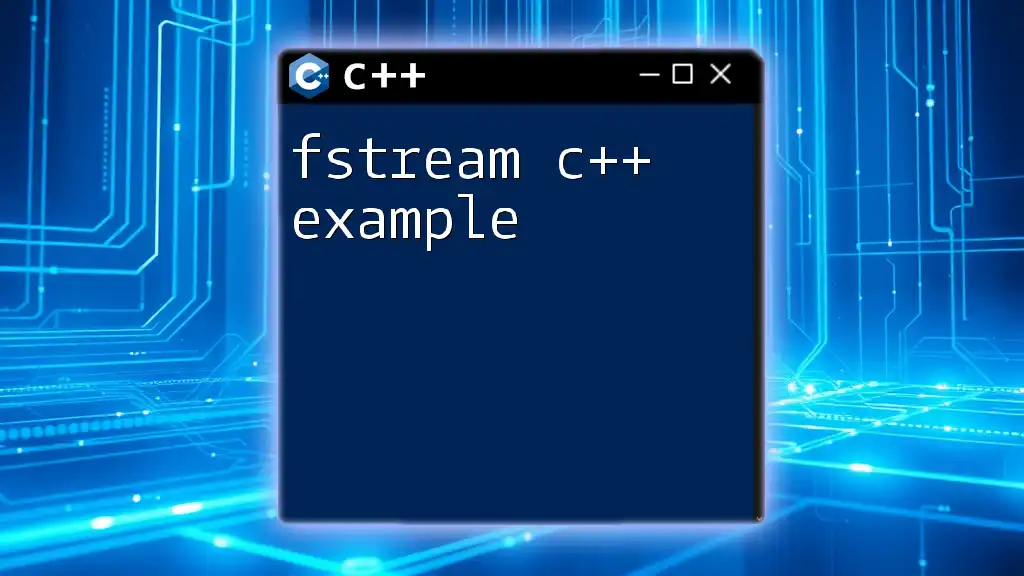
Conclusion
Sample C++ code examples serve as invaluable resources for both beginners and experienced programmers. Learning through real-world examples not only solidifies your understanding of syntax and semantics but also encourages practical application of concepts. By practicing these examples and exploring new ones, you can enhance your C++ programming skills dramatically.
Additional Resources
Educate yourself further by exploring a variety of resources, including:
- Books on C++ programming
- Online courses and tutorials dedicated to C++
- Documentation provided by the C++ standard library
- Community forums where you can ask questions and share knowledge
Delve into the world of C++ programming with these sample code examples, and let your coding journey thrive!