C++ is a powerful programming language that supports object-oriented programming and allows developers to create efficient and high-performance applications, as illustrated in the following example demonstrating a simple "Hello, World!" program.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++
What is C++?
C++ is a powerful general-purpose programming language that was developed in the early 1980s as an extension of the C programming language. It adds object-oriented features, thus allowing programmers to work with complex systems more intuitively. Key features of C++ include:
- Object-oriented programming: C++ allows for encapsulation, inheritance, and polymorphism, enabling better code management and reuse.
- Low-level manipulation: The language gives programmers direct access to memory, making it suitable for system-level programming.
- Rich library support: C++ comes with a comprehensive Standard Library that provides numerous functionalities.
Why Use C++?
C++ is widely used in various domains, including system/software development, game development, and performance-critical applications. Here are some of its major benefits:
- Performance: C++ is known for its high performance due to its close-to-hardware functionality.
- Portability: Written C++ code can be compiled on many platforms with minimal changes.
- Control: The language provides fine control over system resources and memory management.
When compared to languages like Python or Java, C++ can offer faster execution times and more granular control over system resources, making it a valuable tool in a programmer's toolkit.
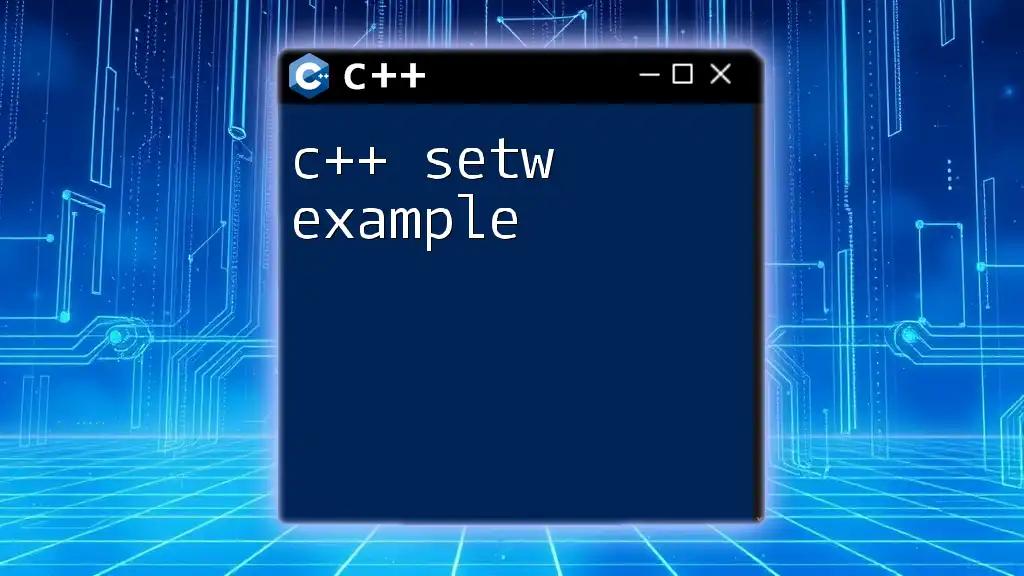
Getting Started with C++
Setting Up Your Environment
Before you can start writing C++ programs, you'll need to set up a development environment. Here are some popular IDEs you can consider:
- Visual Studio: Ideal for Windows users, it provides robust features and debugging tools.
- Code::Blocks: A lightweight option that supports multiple operating systems.
- CLion: A powerful cross-platform IDE that's part of JetBrains.
To run C++ code, you'll also need a compiler like g++ or clang. Installation instructions vary depending on your operating system, so check the specific guidelines for the environment you choose.
Writing Your First C++ Program
Let's dive into the structure of a basic C++ program. Here’s a classic example of a simple program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Explanation of the code:
- `#include <iostream>`: This preprocessor directive includes the Input/Output stream library, which is essential for console operations.
- `int main()`: This line defines the main function where program execution begins.
- `std::cout`: An object used to output data to the console. Here, it’s used to print the string "Hello, World!".
- `return 0;`: This statement indicates the program has finished executing successfully.
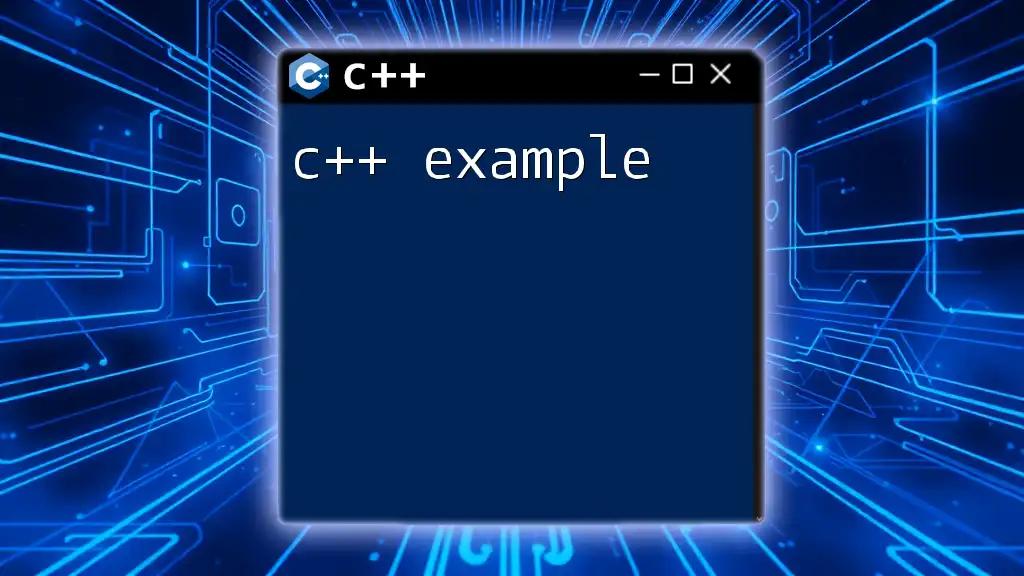
Key Components of C++
Variables and Data Types
In C++, variables are used to store data that can be changed during program execution. Here are some common data types:
- int: For integers (e.g., `int age = 25;`)
- float: For decimal numbers (e.g., `float salary = 3000.50;`)
- char: For single characters (e.g., `char grade = 'A';`)
- bool: For boolean values (e.g., `bool isEmployed = true;`)
Type safety is a crucial aspect of C++. It only allows certain operations for each data type, reducing the risk of errors. Additionally, C++ supports type inference, allowing you to declare variables without explicitly stating their types:
auto avg = 25.5; // Automatically inferred as double
Control Structures
Conditional Statements
C++ provides conditional statements to execute different portions of code based on certain conditions. The most common are `if`, `else if`, and `else`. Here’s an example:
if (age >= 18) {
std::cout << "You are an adult." << std::endl;
} else {
std::cout << "You are a minor." << std::endl;
}
In this snippet, the output will determine if the person is an adult or a minor based on their age.
Loops
Loops allow you to execute a section of code repeatedly. C++ supports several types of loops, including `for`, `while`, and `do-while`. Here's an example using a `for` loop:
for (int i = 0; i < 5; i++) {
std::cout << "Iteration: " << i << std::endl;
}
In this case, the loop iterates from 0 to 4, displaying each iteration in the console.
Functions
Functions in C++ enable code organization into reusable blocks. Here's a fundamental function that adds two numbers together:
int add(int a, int b) {
return a + b;
}
int main() {
std::cout << "Sum: " << add(5, 10) << std::endl;
return 0;
}
Explanation: The `add` function takes two integers, sums them, and returns the result. The `main` function demonstrates calling the `add` function and printing the result to the console.
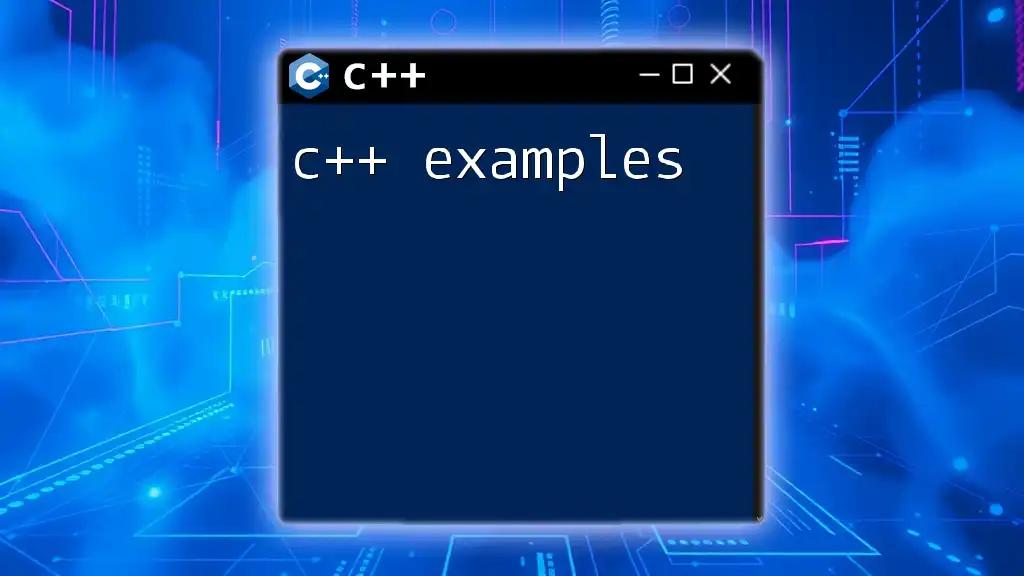
Advanced Concepts
Object-Oriented Programming in C++
C++ is well-known for its object-oriented programming (OOP) capabilities, allowing developers to model real-world entities in a more intuitive way. The three main principles of OOP in C++ include Encapsulation, Inheritance, and Polymorphism.
Here’s a basic class definition:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
int main() {
Dog myDog;
myDog.bark();
return 0;
}
Here, we define a `Dog` class with a public member function `bark`. The `main` function creates an instance of `Dog` and invokes `bark`.
Error Handling
Effective error handling can enhance the reliability of C++ applications. The `try` and `catch` blocks are used to catch exceptions. Here’s a simple example:
try {
int x = 10;
if (x < 0) {
throw std::runtime_error("Negative value");
}
} catch (const std::exception& e) {
std::cout << "Error: " << e.what() << std::endl;
}
In this code, an exception is thrown if `x` is less than zero. The `catch` block then handles the error by printing an error message.
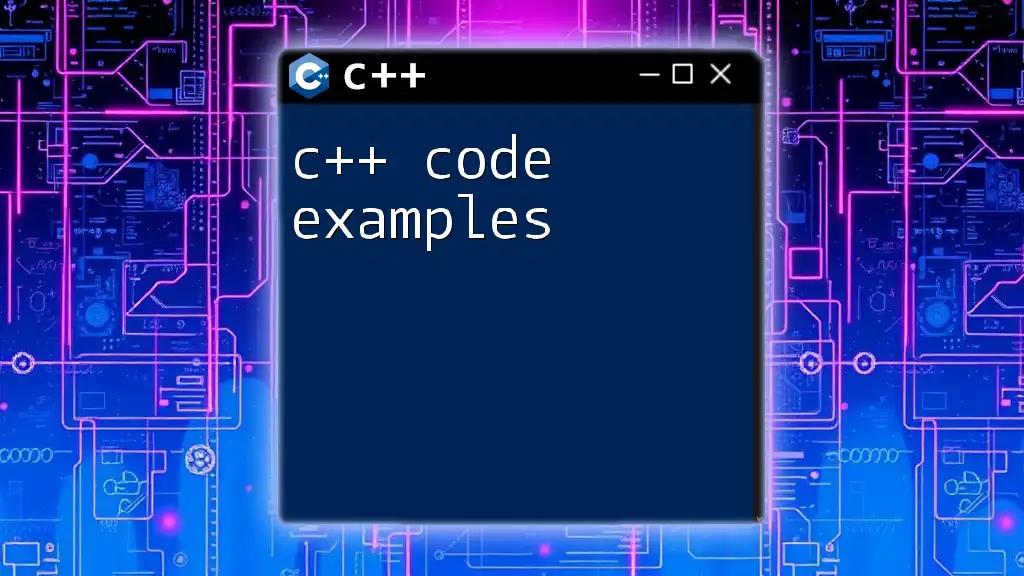
Best Practices
When programming in C++, adhering to best practices can significantly improve code quality:
- Comment Your Code: Comments help explain complex code segments, making them easier to understand and maintain.
- Consistent Naming Conventions: Using a consistent naming scheme increases readability and aids in collaboration among developers.
- Avoiding Common Pitfalls: Be cautious of memory leakage and always free resources when they are no longer needed.
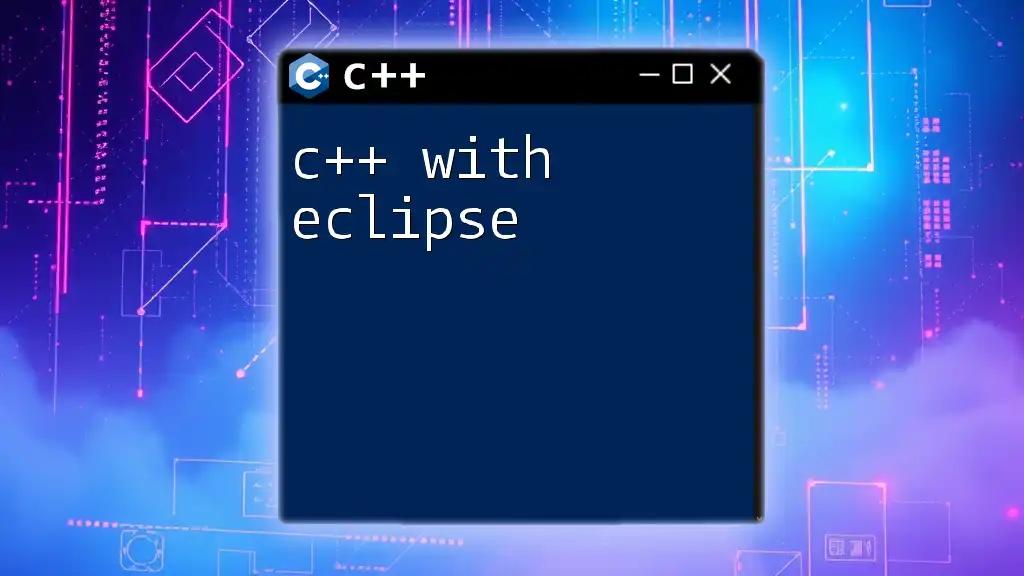
Conclusion
C++ is a versatile programming language that balances performance and functionality. With a foundational understanding of C++ through examples, you can begin building more complex applications and delve into advanced topics.
Additional Resources
To continue your journey in C++, explore books such as "The C++ Programming Language" by Bjarne Stroustrup, online courses on platforms like Coursera or Udemy, and community forums like Stack Overflow for guidance and support.