A "for loop" in C++ is a control structure that allows you to execute a block of code repeatedly with a specified number of iterations, as demonstrated in the following example:
#include <iostream>
using namespace std;
int main() {
for (int i = 0; i < 5; i++) {
cout << "Iteration: " << i << endl;
}
return 0;
}
Understanding the For Loop in C++
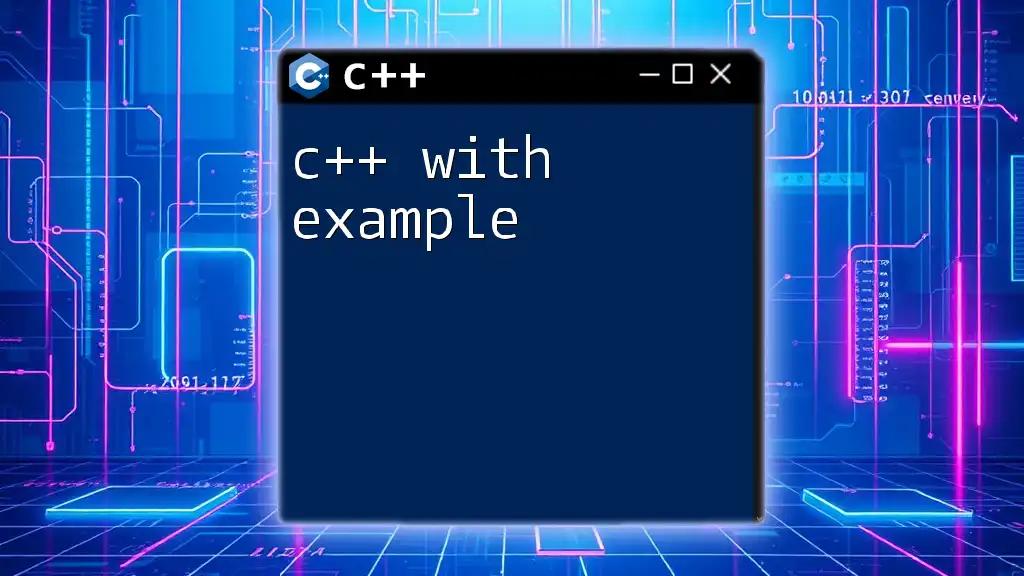
Introduction to Loops in C++
Loops are fundamental constructs in programming that allow developers to execute a block of code multiple times without the need to duplicate code manually. This capability is particularly valuable when working with repetitive tasks or large datasets. In C++, there are several types of loops available, including while loops, do-while loops, and for loops.
In this article, we will focus on the for loop, which provides a concise and structured way to iterate over a range of values, making it a popular choice among programmers.
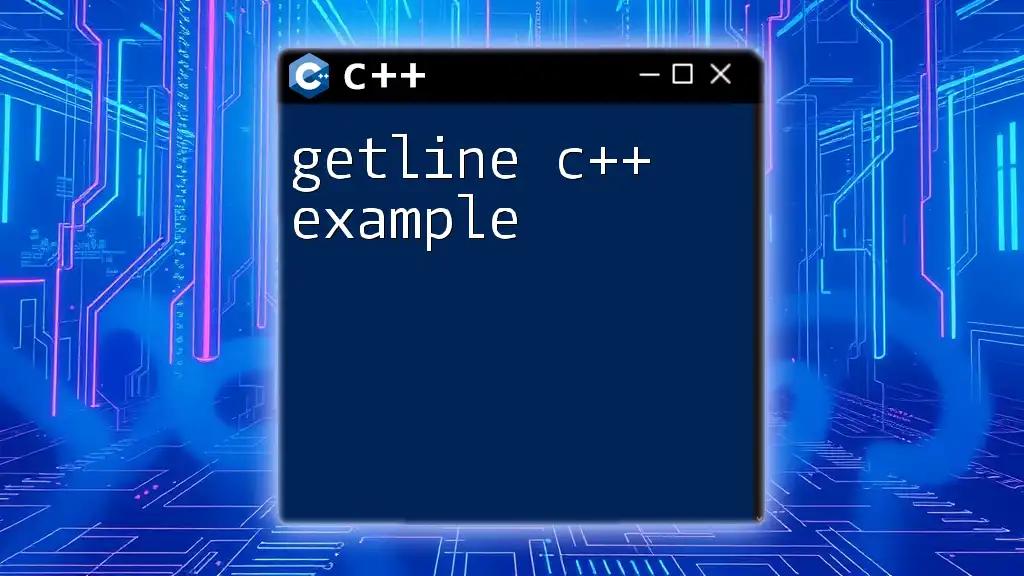
The Structure of a For Loop
The for loop in C++ is designed with a specific syntax that allows it to be easily read and understood at a glance. The basic syntax is as follows:
for (initialization; condition; increment/decrement) {
// code block to be executed
}
Explanation of Each Component
-
Initialization: This is where you declare and set the loop control variable. It typically occurs at the beginning of the loop.
-
Condition: This expression is evaluated before each iteration of the loop. The loop will continue as long as this condition evaluates to true. Once it is false, the loop will terminate.
-
Increment/Decrement: After the code block has executed, this step modifies the loop control variable, usually increasing or decreasing its value. This is crucial for progressing toward the termination of the loop.
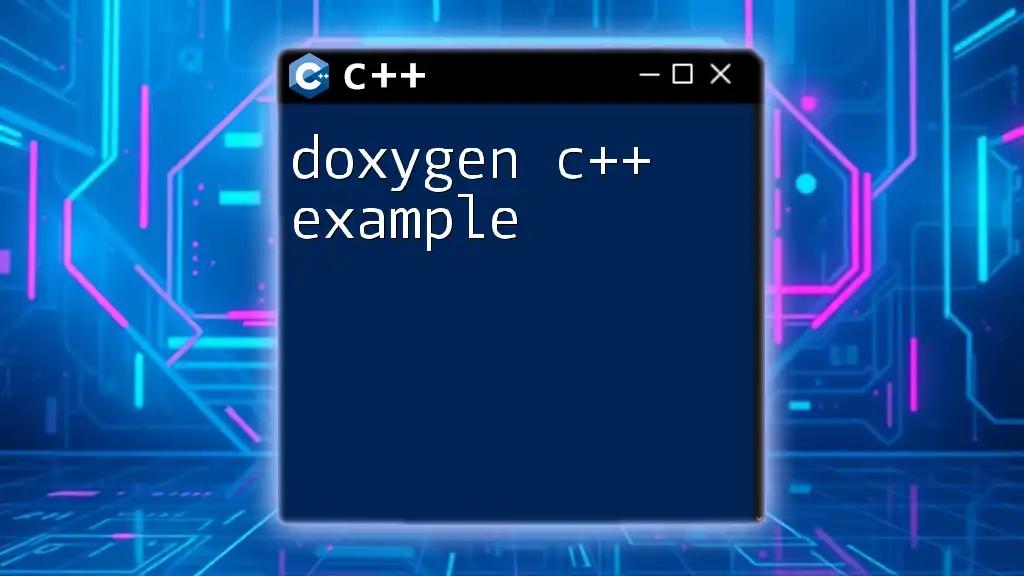
How the For Loop Works
Understanding how a for loop operates can help demystify its behavior. The execution flow consists of four key steps:
-
Initialization is performed first, establishing the value of the loop control variable.
-
Condition Evaluation occurs next. If the condition evaluates to true, the loop enters its body.
-
Code Execution takes place within the body, where developers can perform necessary computations or actions.
-
Increment/Decrement modifies the control variable, and the loop returns to the second step. This process continues until the condition evaluates to false.
This structured approach simplifies managing repetitive tasks while also ensuring that the program does not inadvertently enter into an infinite loop.
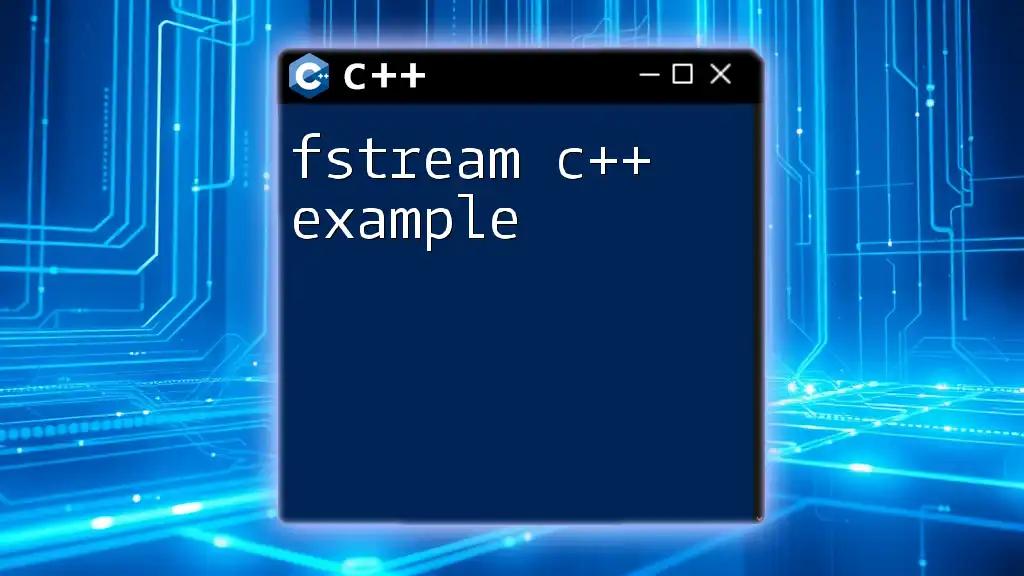
Practical Examples of For Loops in C++
Example 1: Simple For Loop
A simple for loop provides a clear demonstration of how loops function in C++. Here’s a straightforward example:
for (int i = 0; i < 5; i++) {
std::cout << "Iteration: " << i << std::endl;
}
In this example, the loop initializes the variable `i` at 0. The condition `i < 5` checks whether `i` is less than 5 before executing the block that outputs the current iteration number. After each iteration, `i` is incremented by 1. The output will display the iterations from 0 to 4, confirming that the loop executed five times, as expected.
Example 2: Using For Loop with Arrays
For loops are particularly useful for processing arrays or collections. The following code snippet illustrates this concept:
int arr[] = {2, 4, 6, 8, 10};
for (int i = 0; i < sizeof(arr)/sizeof(arr[0]); i++) {
std::cout << "Element at index " << i << ": " << arr[i] << std::endl;
}
This example demonstrates how a for loop can iterate through an array. The loop initializes `i` to 0 and continues until it reaches the size of the array. The `sizeof(arr)/sizeof(arr[0])` expression calculates the number of elements in the array. Each element is accessed and printed, showcasing the elements indexed in the array from 0 to 4.
Example 3: Nested For Loops
Nested for loops allow you to perform complex iterations, such as creating a multiplication table. The following code demonstrates this scenario:
for (int i = 1; i <= 3; i++) {
for (int j = 1; j <= 2; j++) {
std::cout << "i: " << i << ", j: " << j << std::endl;
}
}
Here, the outer loop iterates with `i` values from 1 to 3, while the inner loop iterates with `j` values from 1 to 2 for every iteration of `i`. The output will display every combination of `i` and `j`, demonstrating how nested loops can be utilized to handle more complex scenarios.
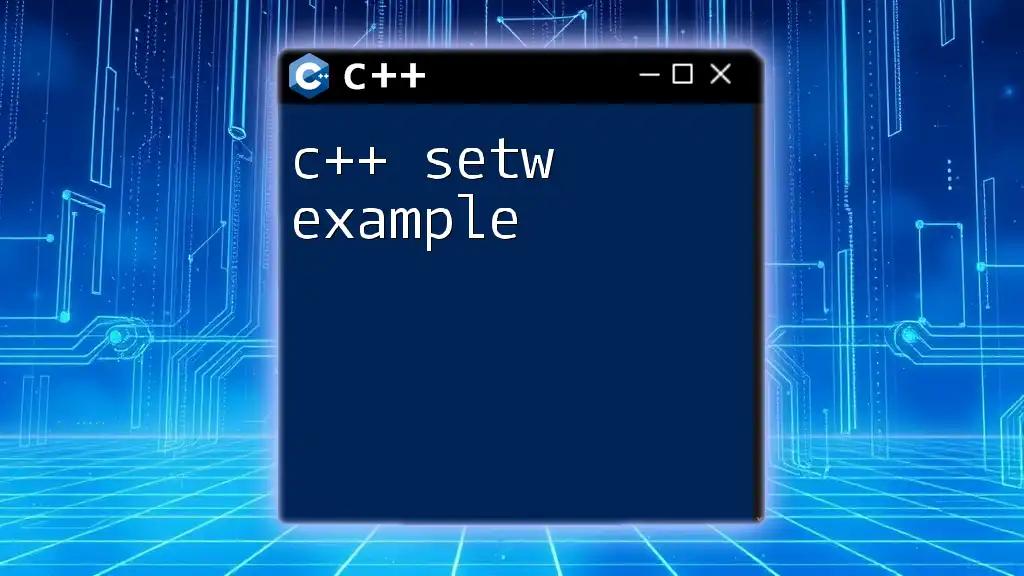
Common Mistakes to Avoid When Using For Loops
While using for loops can simplify programming tasks, several common pitfalls can lead to issues.
Off-by-One Errors
Off-by-one errors occur when a loop iterates one time too many or one time too few. A classic example is using `i <= n` instead of `i < n`, resulting in an extra iteration and potential out-of-bounds access.
Infinite Loops
An infinite loop arises when the termination condition is never met. For instance:
for (int i = 0; i < 5; ) {
std::cout << "Infinite Loop!" << std::endl; // Missed increment step
}
Here, because there’s no incrementing step included, the loop continues indefinitely, causing your program to hang.
Forgotten Initialization or Increment Steps
Neglecting to properly set up the initialization or increment steps can lead to broken loops or infinite iterations. Always remember that every part of the for loop structure is crucial for its proper function.
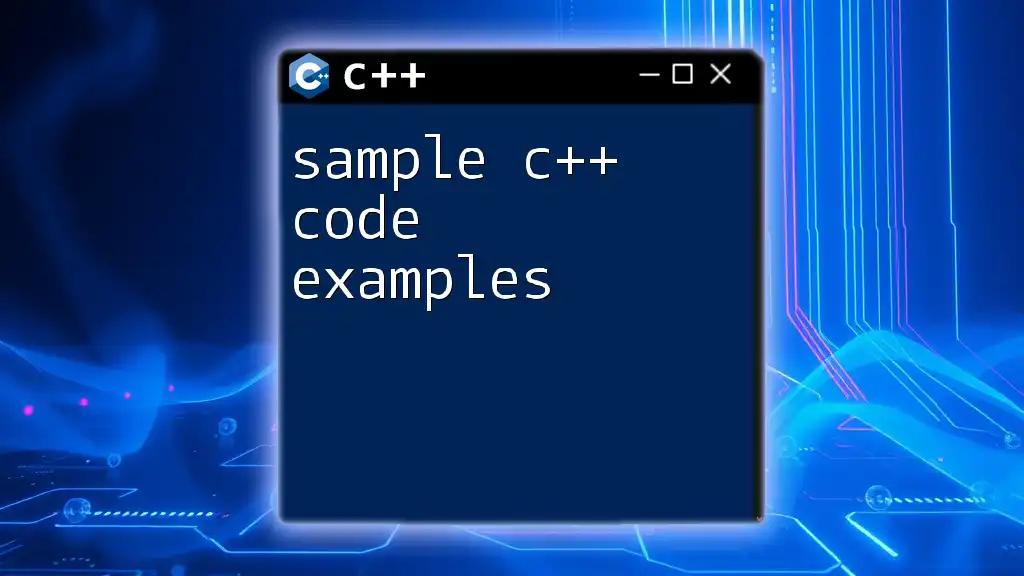
Best Practices for Using For Loops
To write effective and maintainable for loops, consider the following best practices:
Keep the Code Clear and Concise
Avoid overly complex expressions in the loop conditions or using multiple variables in the initialization. Clean, readable code improves maintainability and understanding.
Choosing the Right Loop Type
Identify when a for loop is the most suitable choice versus while or do-while loops. For loops are ideal for situations where the number of iterations is known in advance.
Using Comments for Clarity
Documenting your code with comments helps clarify the intent of the loop, especially for more complex logic or nested loops. This practice serves both the original developer and future maintainers of the code.
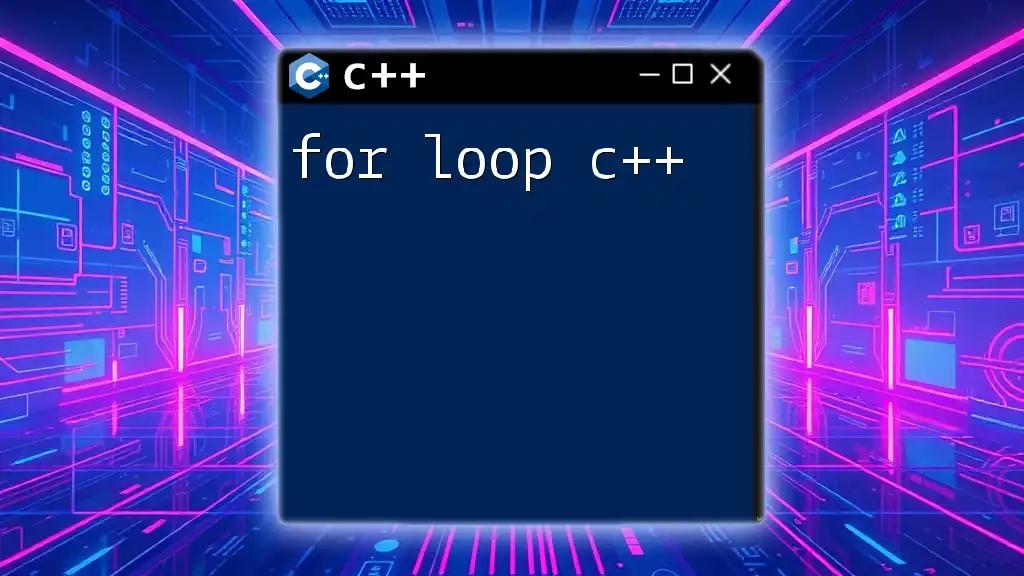
Conclusion
In summary, the for loop in C++ with example showcases a powerful and flexible way to handle repetitive tasks effectively. By understanding its structure and behavior, as well as avoiding common pitfalls, you can harness the full potential of for loops in your programming endeavors. So, take the time to practice these concepts through coding challenges and exercises, solidifying your knowledge and developing proficiency with loops in C++.
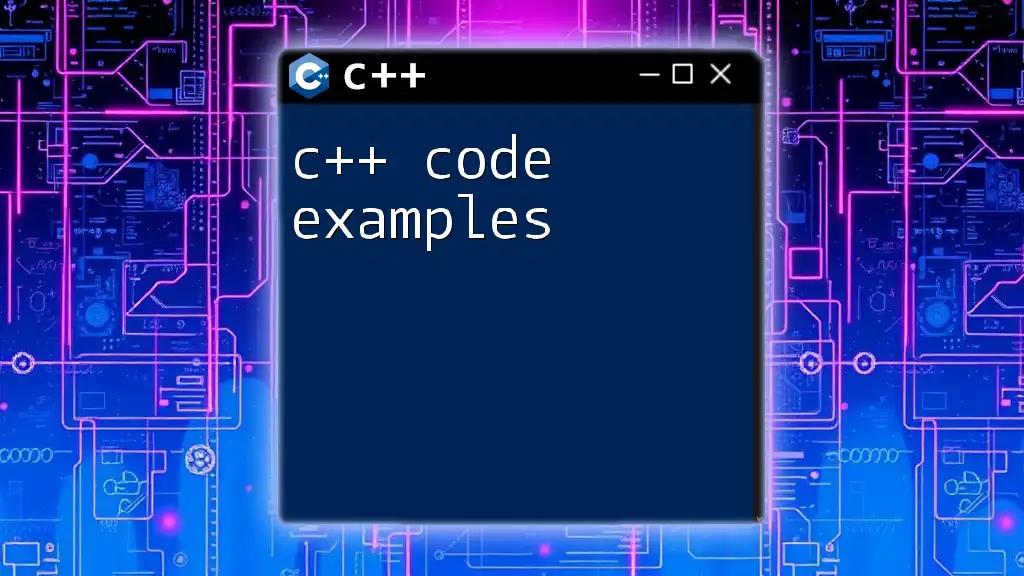
Additional Resources
To further enhance your understanding of C++ loops, consider exploring official documentation and online tutorials. Recommended books and guides on C++ programming can also provide deeper insights into effective coding practices and advanced techniques.