The Microsoft C++ compiler can be utilized on the Steam Deck to develop and run C++ applications, allowing users to create software for this handheld gaming device efficiently.
Here’s a simple code snippet to showcase a basic "Hello, World!" application in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Microsoft C++
What is Microsoft C++?
Microsoft C++ is a powerful programming language that serves as a cornerstone in software and game development. It provides developers with extensive libraries and tooling support through the Microsoft Visual Studio environment, enabling seamless integration with various applications. Its intuitive syntax, combined with robust functionality, makes it an industry-standard choice among developers working on diverse platforms, including gaming systems like the Steam Deck.
The Role of C++ in Game Development
The advantages of using C++ in gaming applications cannot be overstated. This language offers:
-
Performance: C++ is known for its high performance due to its close-to-hardware nature, allowing developers to write highly optimized code. This is crucial for gaming, where speed and efficiency can directly impact user experience.
-
Memory Management: Unlike many high-level programming languages, C++ allows programmers direct control over memory management. This capability is essential for creating resource-intensive games on devices like the Steam Deck.
-
Compatibility with Game Engines: Many popular game engines, such as Unreal Engine and Unity, utilize C++ as their primary language. This compatibility facilitates cross-platform game development, allowing developers to efficiently deploy games onto various devices, including the Steam Deck.
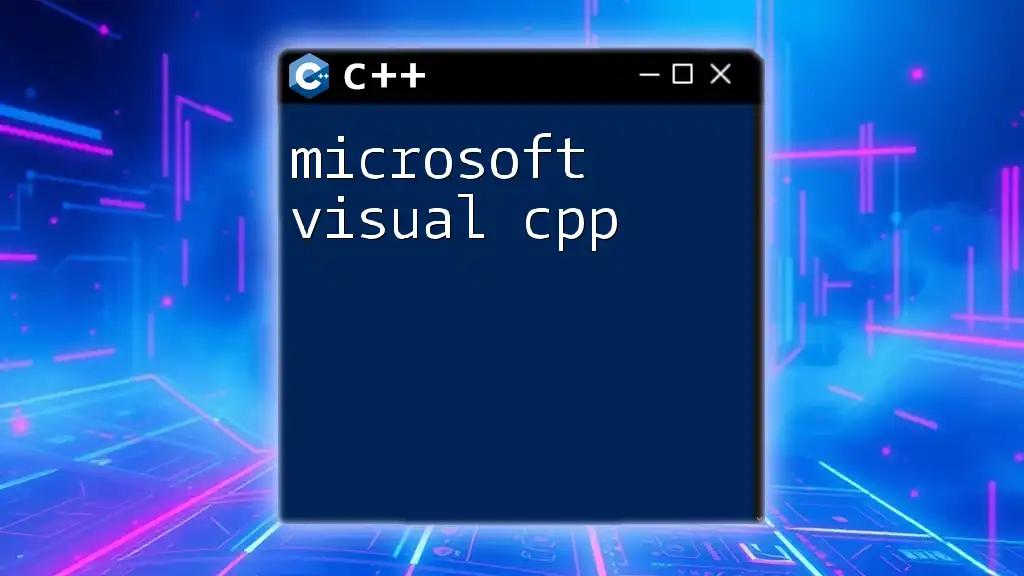
Overview of the Steam Deck
What is the Steam Deck?
The Steam Deck is a handheld gaming device developed by Valve Corporation that allows users to play their PC games on the go. With its impressive hardware specifications, including a custom AMD APU, a 7-inch touchscreen display, and a versatile controller system, the Steam Deck offers an engaging gaming experience comparable to traditional gaming consoles.
The Operating System of the Steam Deck
Powered by SteamOS, a Linux-based operating system, the Steam Deck supports a wide range of applications and is particularly friendly to C++ development. The open-source nature of Linux offers developers the flexibility to create and run C++ applications seamlessly, making the Steam Deck a versatile platform for game development.

Setting Up Your Development Environment
Installing Necessary Software
To get started with Microsoft C++ development, first, you need to install Microsoft Visual Studio. This powerful IDE (Integrated Development Environment) provides essential tools for writing, compiling, and debugging C++ code.
Here are the steps to install it:
- Navigate to the Microsoft Visual Studio website and download the installer.
- Run the installer, selecting the necessary components for C++ development, such as the C++ Desktop Development workload.
- Follow the installation prompts until completion.
Configuring the Steam Deck for C++ Programming
Enabling developer mode on the Steam Deck is a crucial step for developing C++ applications. Follow these steps to activate it:
- Go to Settings.
- Click on System and find Developer Mode.
- Toggle it on and restart the device.
Once in developer mode, familiarize yourself with the development tools displayed in the SteamOS interface. Key resources include text editors, terminal applications, and package managers.
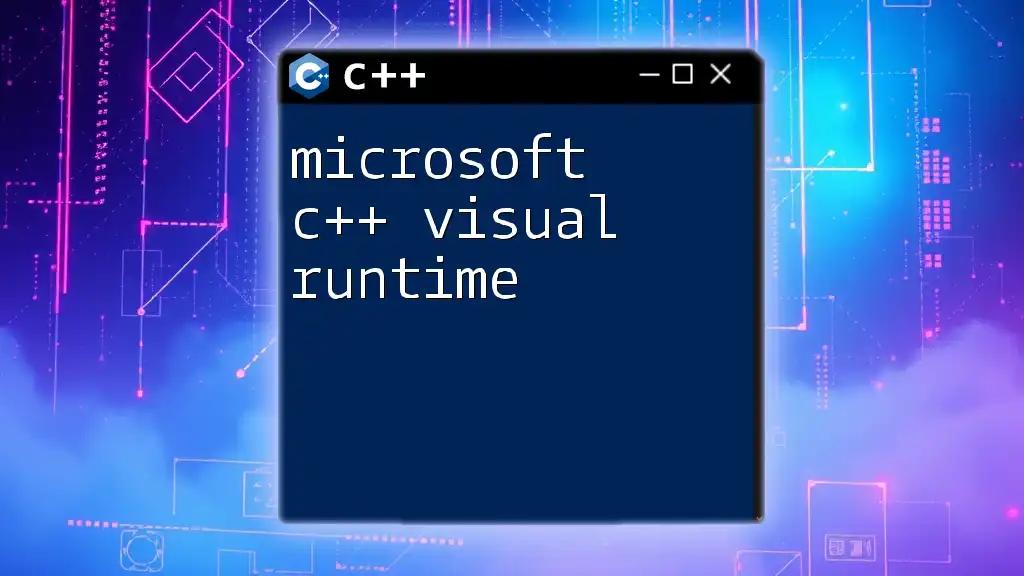
Writing Your First C++ Program for the Steam Deck
Basic C++ Syntax and Structure
Understanding the fundamental syntax and structure of C++ is essential for writing effective code. Key concepts include variables, data types, loops, and conditional statements. Below is a simple example demonstrating basic C++ syntax:
Example Code Snippet: A Simple Hello World Program
#include <iostream>
int main() {
std::cout << "Hello, Steam Deck!" << std::endl;
return 0;
}
This snippet includes the iostream library for input/output operations, defines the main function, and outputs "Hello, Steam Deck!" to the console.
Compiling and Running Your Program
To compile your C++ program on the Steam Deck, you can use the terminal:
- Save your code in a file named `hello.cpp`.
- Open the terminal and navigate to the file's directory.
- Run the following command to compile:
g++ hello.cpp -o hello
- Execute your program with:
./hello
After running these commands, you should see "Hello, Steam Deck!" displayed in the terminal, confirming that your setup is working correctly.
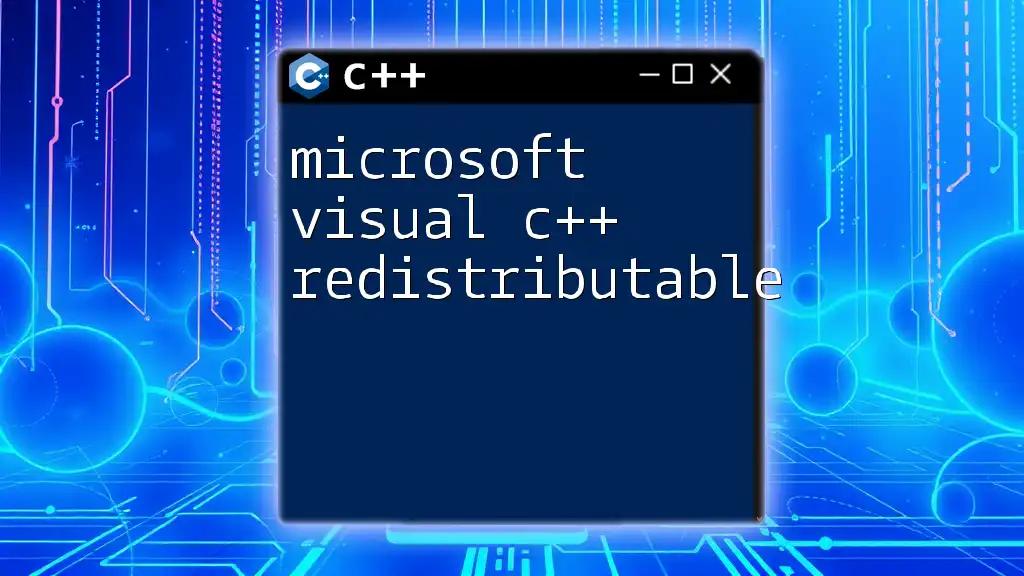
Developing Games for Steam Deck
Best Practices for Game Development in C++
For optimal performance during game development, adhering to established best practices is crucial. These include:
-
Code Optimization: Regularly profile and optimize your code. Efficient algorithms can significantly enhance game speed, leveraging the Steam Deck’s hardware capabilities.
-
Resource Management: Carefully manage memory resources to avoid leaks and crashes, particularly in a resource-constrained environment like the Steam Deck.
-
Modular Design: Structure your code into reusable components, making it easier to manage, test, and debug. This approach enhances code maintainability and accelerates the development process.
Game Engine Compatibility
When developing games for the Steam Deck, you can utilize several popular game engines that support C++. Two noteworthy mentions include:
-
Unreal Engine: Renowned for its stunning graphics capabilities, Unreal Engine allows developers to create immersive environments. By leveraging its C++ API, developers have full control over the game’s behavior and performance.
-
Unity: While Unity's core language is C#, it supports C++ through plugins. This flexibility lets developers harness C++'s performance benefits while using Unity's user-friendly features.
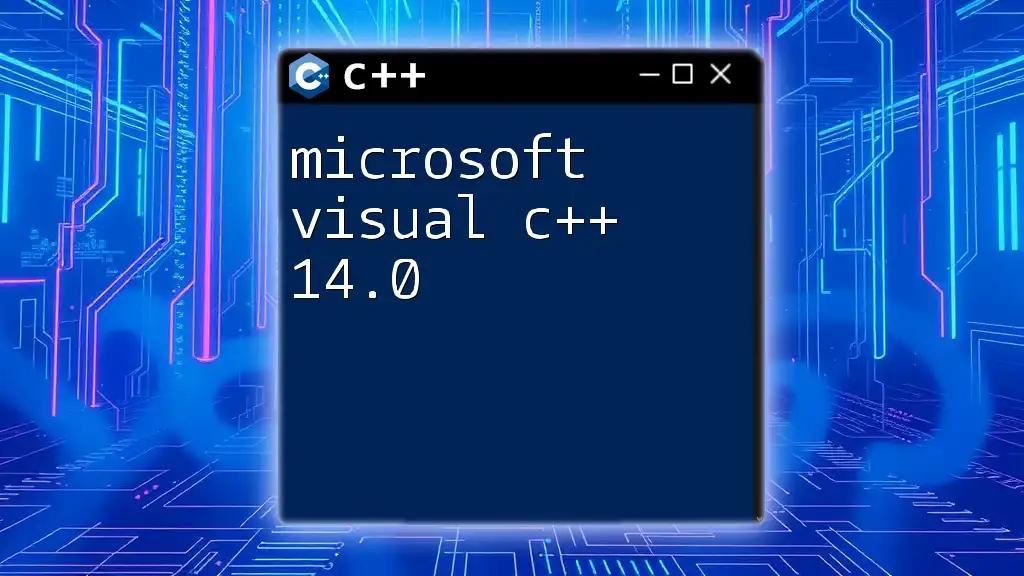
Testing and Debugging
Debugging C++ Applications
Effective debugging is critical for producing high-quality applications. Common debugging techniques in C++ include:
-
Print Statements: Using `std::cout` to output variable values can help track code execution flow and identify logical errors.
-
Integrated Debugging Tools: Utilize Visual Studio’s built-in debugger to step through your code, inspect variables, and identify breaking points.
Performance Testing on the Steam Deck
Performance testing entails evaluating the game under various conditions to ensure a smooth user experience. You can utilize tools like Gprof or Valgrind for performance profiling, which help identify bottlenecks and optimize code accordingly.
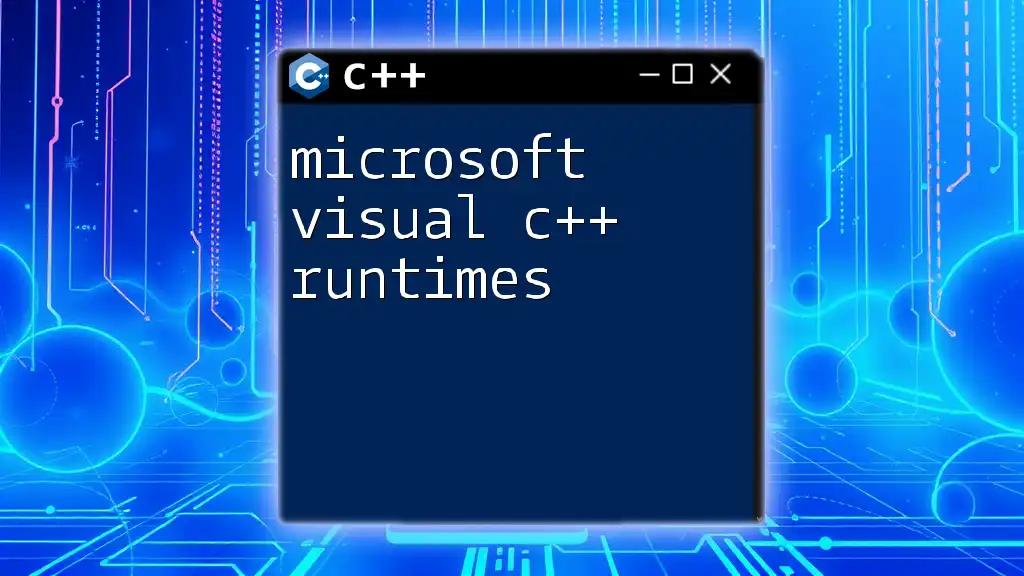
Deploying Applications on Steam Deck
Creating Install Files
Once your game is complete, packaging it for distribution is the next step. Use tools like CMake to generate installation files, which can be run directly on the Steam Deck. Ensure that you handle file permissions correctly to facilitate smooth execution.
Publishing Your Game on Steam
Publishing on Steam involves a few essential steps:
- Create a Steamworks account and set up your game’s page.
- Upload your packaged game files and fill out necessary information.
- Once approved, your game will be live for players, allowing you to gather feedback and make improvements.
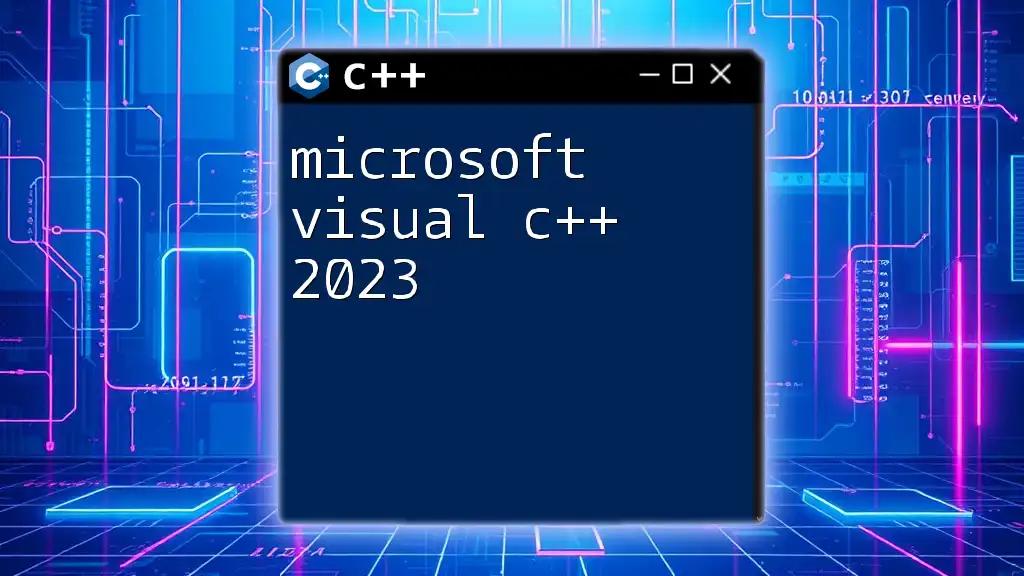
Troubleshooting Common Issues
Installation Errors
When setting up C++, you may encounter common error messages. Issues like "missing dependencies" can typically be resolved by ensuring that all necessary libraries are installed and that your environment is properly configured.
Performance Issues
For performance-related problems, analyze your code for inefficient algorithms. Use profiling tools to collect data on resource usage and optimize identified bottlenecks.
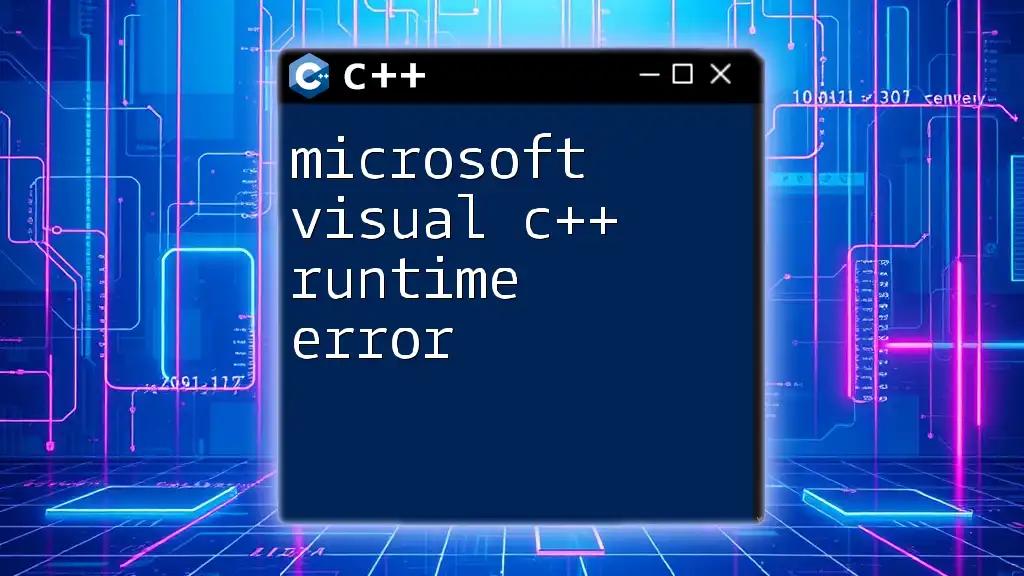
Additional Resources
Online Documentation and Communities
Make use of resources like the official Microsoft C++ documentation, GitHub repositories, and forums like Stack Overflow. Engaging with these communities can provide invaluable insights and solutions to challenges you encounter.
Tutorials and Learning Resources
Consider exploring platforms like Coursera or Udemy for tutorials on C++ and game development. Books such as "C++ Primer" offer in-depth understanding, while online communities often share valuable content and projects.
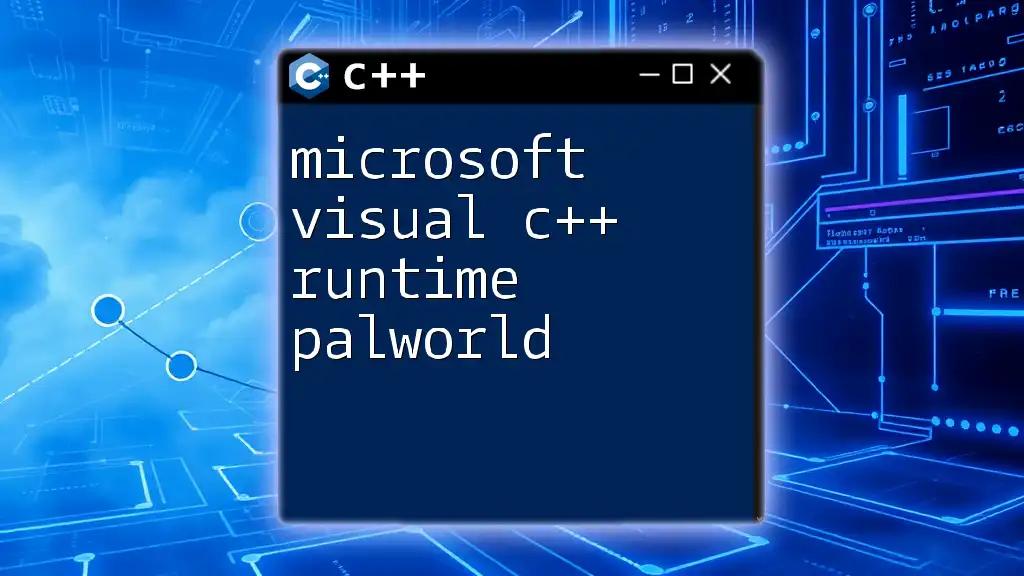
Conclusion
Utilizing Microsoft C++ for the Steam Deck opens an array of opportunities for aspiring game developers. By mastering C++ syntax, effective game engine usage, and following industry best practices, you can create compelling applications tailored to this versatile platform. Start developing today, and don’t hesitate to share your projects and experiences with the growing community of Steam Deck developers.