Microsoft C++ 2005 is an integrated development environment (IDE) that allows developers to create, debug, and manage C++ applications efficiently using the features of the Visual Studio platform.
Here’s a simple code snippet that demonstrates a basic "Hello, World!" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is Microsoft Visual C++ 2005?
Microsoft Visual C++ 2005 is a powerful integrated development environment (IDE) that allows developers to create applications using the C++ programming language. Released as part of the Microsoft Visual Studio 2005 suite, it offers a range of tools that enhance the development process, making it easier to write, debug, and compile C++ code efficiently.
Historical Context and Release Timeline
Microsoft C++ 2005 is notable as it marked significant improvements over its predecessors. It aligned itself with the C++ standard, incorporating features that developers demanded, such as better support for Object-Oriented Programming (OOP) and enhanced debugging tools. Released in 2005, it paved the way for subsequent versions that followed, each of which built upon the advancements made in this iteration.
Key Features of the IDE
The IDE includes numerous features that streamline the coding process:
- IntelliSense: A code-completion feature that provides context-aware suggestions as you type, significantly speeding up the coding process.
- Code Snippets: Built-in templates that allow for quick insertion of reusable code blocks, enhancing productivity.
- Integrated Debugging: Robust debugging tools that help identify and troubleshoot code issues in real-time.
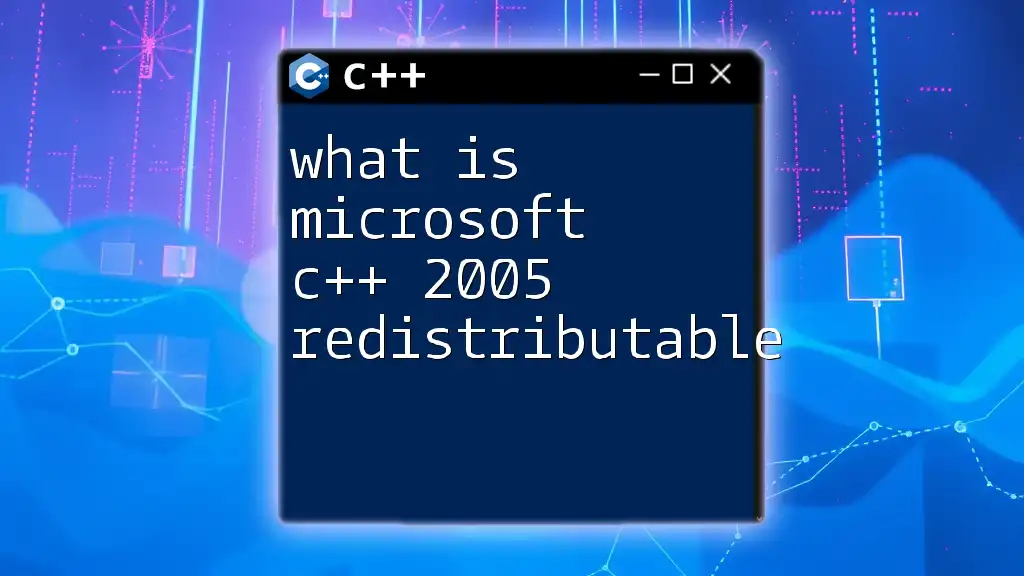
Key Features of Microsoft Visual C++ 2005
Improved Development Environment
The user interface of Microsoft Visual C++ 2005 is designed to optimize the development workflow. It offers customizable toolbars, windows, and options that help developers navigate large projects seamlessly. The introduction of IntelliSense empowers developers to write code faster and with fewer errors by providing suggestions and auto-completions based on the context of the current code.
Example of a code snippet using IntelliSense:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // Typing 'std::cout' would invoke IntelliSense
return 0;
}
Enhanced Debugging Tools
Debugging in Microsoft C++ 2005 is enhanced with several features that allow developers to quickly identify and resolve issues. The IDE allows setting breakpoints in the code where execution can pause, enabling inspection of variable states and program flow.
To set a breakpoint:
- Click in the left margin of the code window next to the line number where you want to pause execution.
- Run the program in debug mode. It will stop at the set breakpoint, allowing you to step through the code and analyze its behavior.
Support for Standard C++ and Extensions
Microsoft Visual C++ 2005 adheres to the C++ standard, ensuring that developers can write portable and compatible code. Additionally, the IDE supports Microsoft-specific extensions that provide advanced functionalities, such as improved memory management and threading support.
For instance, a simple code comparison shows the use of standard versus Microsoft extensions:
// Standard C++
#include <vector>
int main() {
std::vector<int> myVector; // Uses STL
return 0;
}
// Microsoft Extension
#include <windows.h>
#include <iostream>
int main() {
MessageBox(NULL, "Hello, World!", "Greetings", MB_OK); // Windows API call
return 0;
}
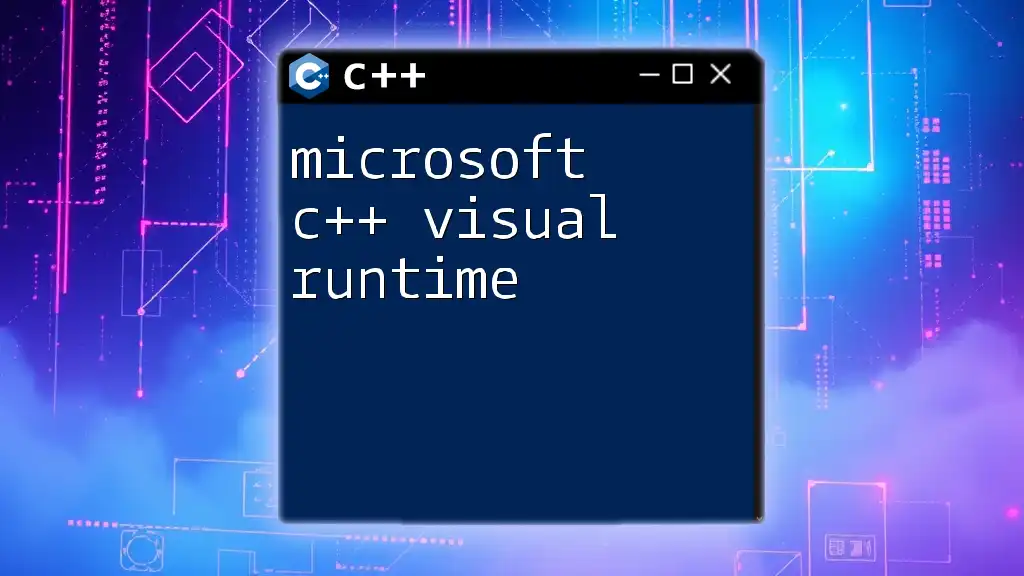
Installation and Setup
System Requirements
To ensure compatibility and optimal performance, it is essential to check the following system requirements before installation:
-
Minimum Specifications:
- Windows XP Service Pack 2 or later
- 500 MHz processor
- 256 MB of RAM
- 3 GB of available disk space
-
Recommended Specifications:
- Windows Vista or later
- 1 GHz processor
- 512 MB of RAM or more
- Additional disk space for complex projects
Downloading Microsoft Visual C++ 2005
The installer for Microsoft Visual C++ 2005 can be found on the official Microsoft website or other trusted software repositories. It's crucial to ensure you download the correct version based on your system architecture (32-bit or 64-bit).
Installing Microsoft Visual C++ 2005 Redistributable
The Microsoft Visual C++ 2005 Redistributable is essential for running applications built using this environment. It contains runtime components required for executing C++ applications.
To install it:
- Download the appropriate version (x86 or x64) from Microsoft’s official site.
- Run the installer and follow the prompts.
- Once installed, verify the installation by checking the "Programs and Features" section in the Control Panel.
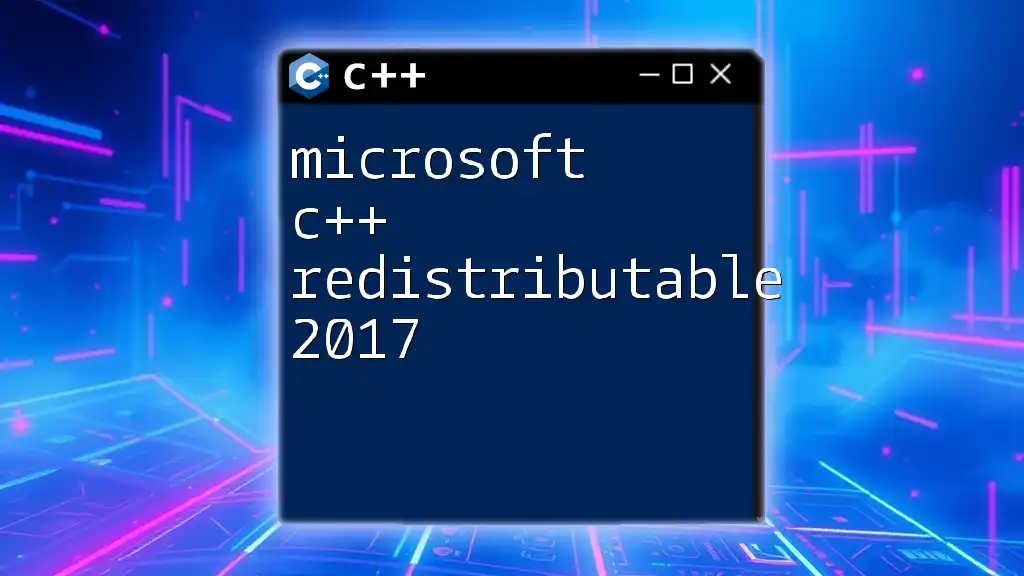
Creating a Simple Application
Your First C++ Program
Creating a simple C++ program in Visual C++ 2005 involves a series of straightforward steps:
- Open Microsoft Visual C++ 2005.
- Select File > New > Project.
- Choose a Win32 Console Application.
- Name your project and click OK.
- Follow the wizard, selecting default options, then click Finish.
A basic “Hello World” program can look like this:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // Output text to the console
return 0;
}
Building and Running the Application
After writing your code, building the project is essential. To build the project:
- Go to Build > Build Solution.
- Fix any compilation errors that appear in the output window.
To run the application:
- Simply press F5 or click the Start Debugging button.
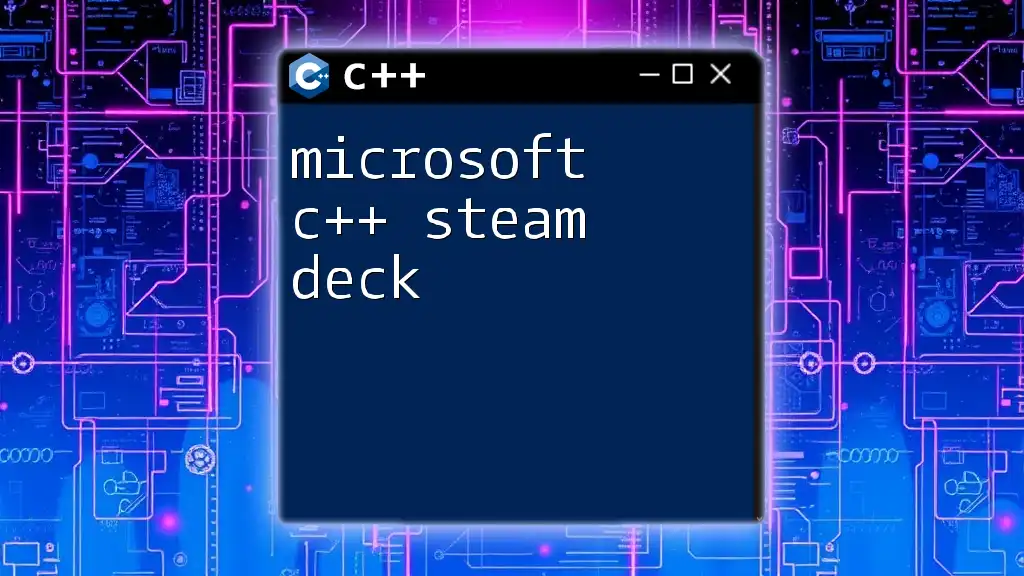
Common Challenges and Solutions
Compiling Errors
Developers often encounter compiling errors due to syntax issues or missing files. Common errors include missing semicolons or undeclared identifiers.
For instance, if you forget a semicolon:
#include <iostream>
int main()
std::cout << "Hello, World!" << std::endl // Missing semicolon will cause a compile error
return 0;
}
To address these issues, refer to the error messages in the output window that typically point to the line of syntax that caused the problem.
Runtime Errors
Runtime errors occur during program execution and can often be harder to diagnose. Common reasons include uninitialized variables or incorrect assumptions about data input.
For example:
#include <iostream>
using namespace std;
int main() {
int num; // Uninitialized variable
cout << num << endl; // Using it can lead to undefined behavior
return 0;
}
Always initialize variables before use and consider adding error handling to manage exceptions smoothly.
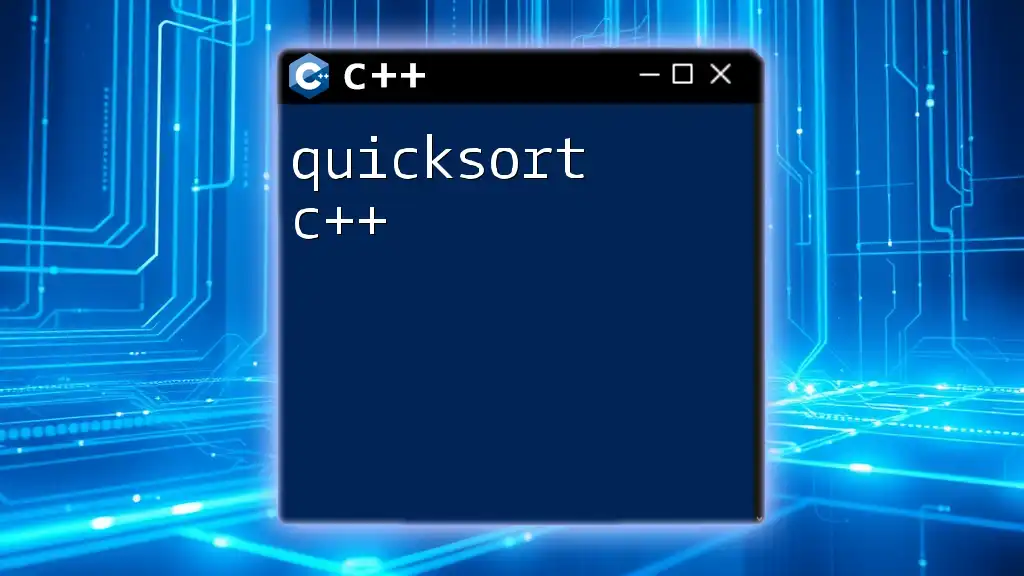
Optimizing Your C++ Code
Best Practices for Efficient Coding
Writing clean and maintainable code is crucial for long-term project success. Follow these best practices:
- Use meaningful variable names that describe their purpose.
- Comment on complex sections of your code to explain intent and logic.
- Structure your code in a way that logically separates concerns, making it easier to read and navigate.
Leveraging Performance Tools in Visual C++ 2005
Performance profiling tools in Visual C++ 2005 help identify bottlenecks in your application. To utilize these tools, access the Performance Wizard and follow the guidelines to monitor application execution time, memory usage, and more.
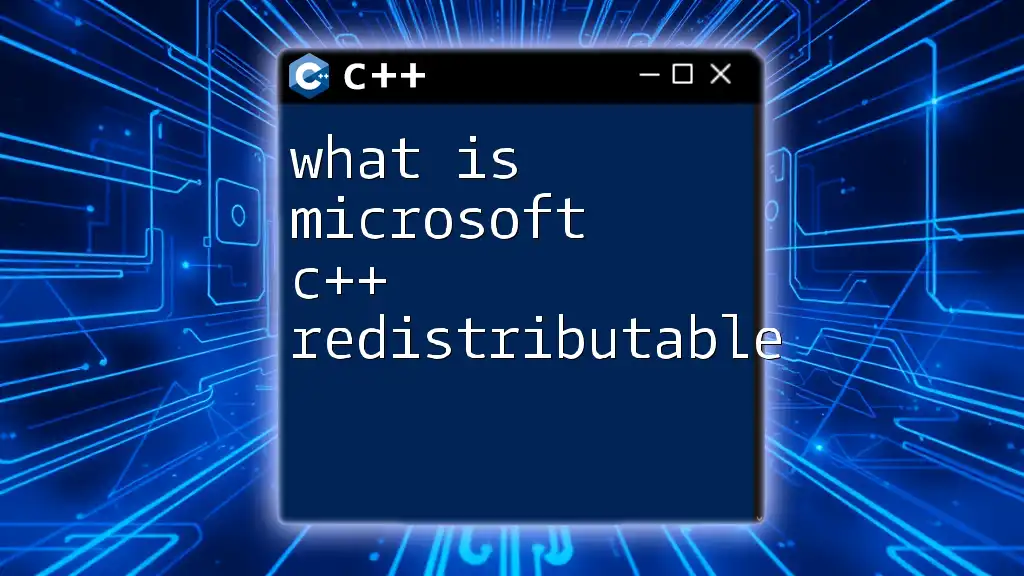
Advanced Features
STL (Standard Template Library) Support
The Standard Template Library (STL) allows developers to utilize pre-written classes and functions for common data structures and algorithms, enhancing productivity.
Using STL, a typical operation might look as follows:
#include <vector>
#include <algorithm>
#include <iostream>
int main() {
std::vector<int> myNumbers = {5, 2, 8, 3, 1};
std::sort(myNumbers.begin(), myNumbers.end()); // Sorts the vector
for(int num : myNumbers) {
std::cout << num << " "; // Outputs sorted numbers
}
return 0;
}
Working with Windows API
For those developing applications specifically for Windows, leveraging the Windows API can enhance functionality. This API allows developers to interact directly with the operating system to handle everything from file operations to GUI functions.
A basic example of utilizing the Windows API to show a message box:
#include <windows.h>
int main() {
MessageBox(NULL, "Hello, World!", "Greetings", MB_OK); // Displays a message box
return 0;
}
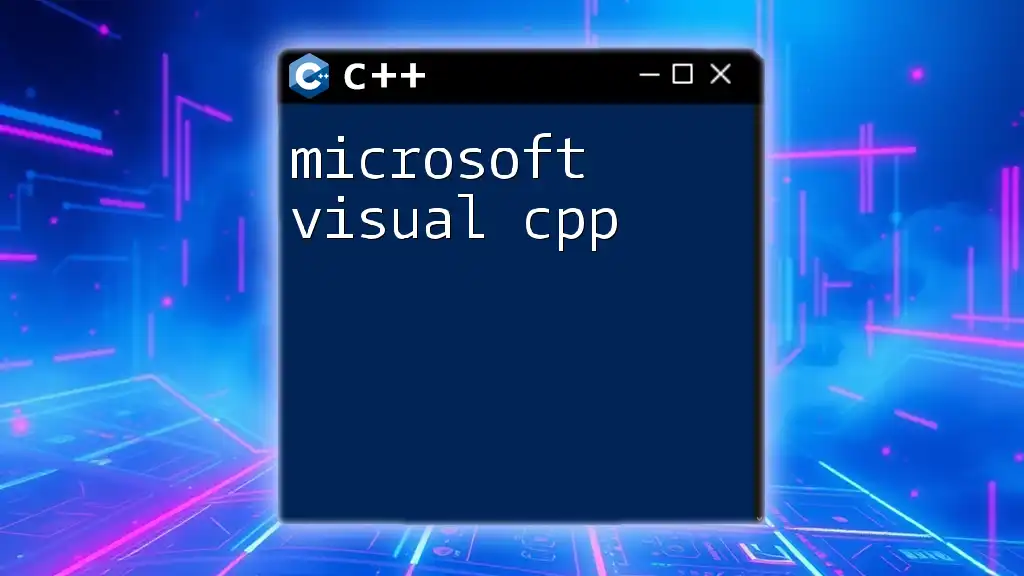
Resources for Further Learning
Official Microsoft Documentation
The official Microsoft documentation provides a wealth of materials, including tutorials, API references, and best practices for C++. Learning how to navigate this resource can significantly enhance your understanding and utilization of Microsoft C++ 2005.
Online Communities and Forums
Participating in online programming communities, such as Stack Overflow and other dedicated forums, is an excellent way to stay updated with the latest developments and troubleshooting tips. Engaging with others can also provide inspiration and examples for your learning journey.
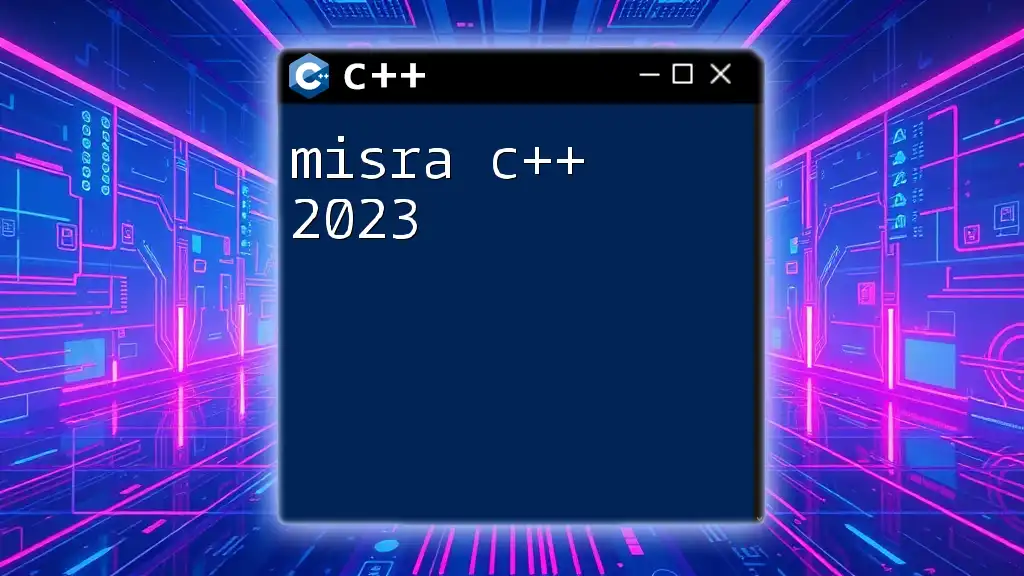
Conclusion
Microsoft C++ 2005 remains an essential tool for developers looking to create efficient software applications. By understanding its features, capabilities, and best practices, you can leverage this powerful IDE to enhance your programming skills. Experiment with the examples provided, optimize your code, and take advantage of the community resources available. Your journey to mastering Microsoft C++ 2005 begins now!
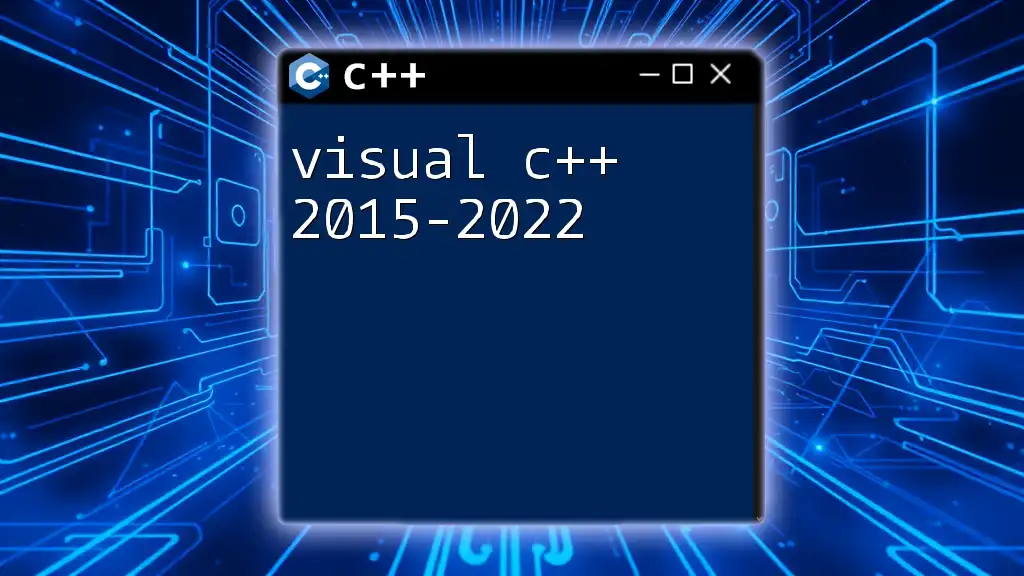
FAQs
What is the difference between Microsoft Visual C++ 2005 and later versions?
Later versions of Microsoft Visual C++ introduced new features and improvements, especially in terms of compatibility with modern programming standards, enhanced debugging tools, and more integrated support for libraries and frameworks.
Can I use Microsoft C++ 2005 for modern applications?
While Microsoft C++ 2005 is robust, modern applications might benefit from newer versions due to additional features and support for the latest C++ standards. However, it can still be used for learning, prototyping, and legacy software development.